Comprehensive Guide to SpaceX Technical Interview Prep: Mastering Algorithms and Problem-Solving
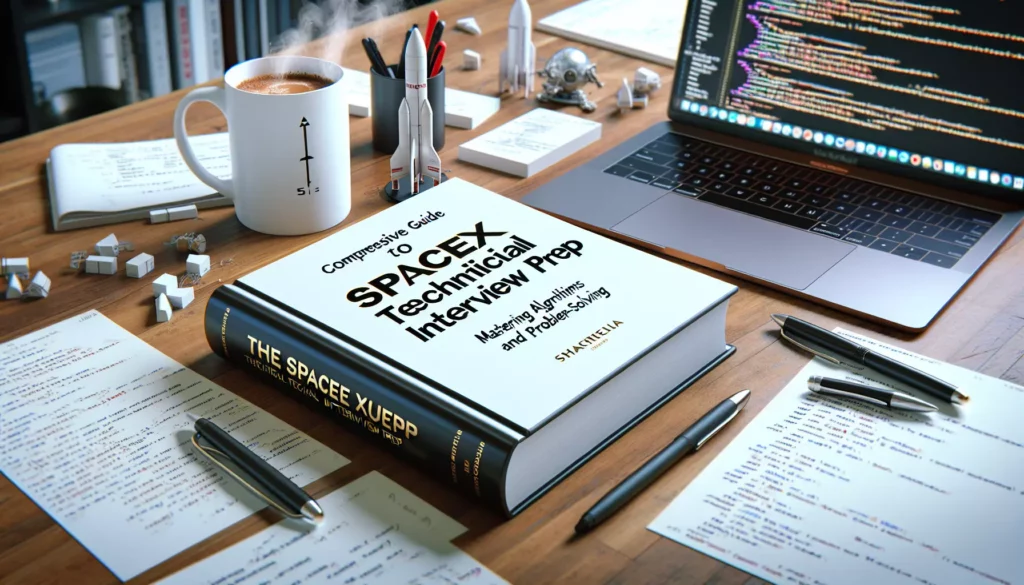
Are you dreaming of launching your career at SpaceX, one of the most innovative aerospace companies in the world? Landing a technical role at SpaceX requires not only a passion for space exploration but also a solid foundation in computer science, problem-solving skills, and the ability to think on your feet. In this comprehensive guide, we’ll walk you through the essential steps to prepare for a SpaceX technical interview, focusing on the key areas that interviewers are likely to assess.
Table of Contents
- Understanding SpaceX and Its Technical Needs
- The SpaceX Interview Process
- Core Computer Science Concepts to Master
- Essential Data Structures for SpaceX Interviews
- Key Algorithms and Problem-Solving Techniques
- System Design and Scalability
- Coding Practice and Resources
- Soft Skills and Behavioral Preparation
- Conducting Mock Interviews
- Final Tips for Success
1. Understanding SpaceX and Its Technical Needs
Before diving into the technical preparation, it’s crucial to understand SpaceX’s mission and the types of challenges their engineers face. SpaceX is known for pushing the boundaries of space technology, with projects ranging from reusable rockets to satellite internet systems.
Key areas of focus for SpaceX include:
- Rocket propulsion systems
- Guidance, navigation, and control systems
- Avionics and flight software
- Satellite communications
- Mission control software
- Data analysis and machine learning for optimizing operations
Understanding these areas will help you tailor your preparation and demonstrate your passion for the company’s goals during the interview process.
2. The SpaceX Interview Process
The SpaceX interview process typically consists of several rounds:
- Initial Phone Screen: A brief conversation with a recruiter to assess your background and interest in the position.
- Technical Phone Interview: A more in-depth discussion with an engineer, often including coding questions or problem-solving exercises.
- Take-Home Assignment: Some positions may require you to complete a coding project or technical assessment.
- On-Site Interviews: A series of face-to-face interviews (or virtual equivalents) with team members and potential colleagues, involving whiteboard coding, system design discussions, and behavioral questions.
Each stage is designed to evaluate your technical skills, problem-solving abilities, and cultural fit within the SpaceX team.
3. Core Computer Science Concepts to Master
To excel in a SpaceX technical interview, you should have a strong grasp of fundamental computer science concepts. Focus on the following areas:
- Time and Space Complexity Analysis: Understanding Big O notation and being able to analyze the efficiency of your algorithms is crucial.
- Object-Oriented Programming (OOP): Familiarize yourself with concepts like encapsulation, inheritance, polymorphism, and abstraction.
- Memory Management: Understand how memory allocation works, especially in languages like C and C++, which are commonly used at SpaceX.
- Concurrency and Multithreading: Given the real-time nature of many SpaceX systems, knowledge of parallel processing is valuable.
- Networking Fundamentals: Understand TCP/IP, HTTP, and other protocols used in distributed systems.
- Database Systems: Familiarity with both SQL and NoSQL databases can be beneficial.
4. Essential Data Structures for SpaceX Interviews
Mastery of data structures is fundamental to performing well in technical interviews. Be prepared to implement and discuss the following:
- Arrays and Strings: Understand operations, searching, and sorting techniques.
- Linked Lists: Implement singly and doubly linked lists, and solve related problems.
- Stacks and Queues: Know their applications and be able to implement them.
- Trees: Understand binary trees, binary search trees, and balanced trees like AVL or Red-Black trees.
- Graphs: Be familiar with representation methods and traversal algorithms.
- Hash Tables: Understand hash functions, collision resolution, and implement a basic hash table.
- Heaps: Know how to implement and use min-heaps and max-heaps.
Here’s a simple example of implementing a stack in Python:
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
if not self.is_empty():
return self.items.pop()
def peek(self):
if not self.is_empty():
return self.items[-1]
def is_empty(self):
return len(self.items) == 0
def size(self):
return len(self.items)
# Usage example
stack = Stack()
stack.push(1)
stack.push(2)
stack.push(3)
print(stack.pop()) # Output: 3
print(stack.peek()) # Output: 2
print(stack.size()) # Output: 2
5. Key Algorithms and Problem-Solving Techniques
SpaceX interviewers will expect you to be well-versed in various algorithmic approaches. Focus on understanding and implementing the following:
- Sorting Algorithms: Quick Sort, Merge Sort, Heap Sort, and their time/space complexities.
- Searching Algorithms: Binary Search, Depth-First Search (DFS), Breadth-First Search (BFS).
- Dynamic Programming: Understand how to break down problems into subproblems and memoize results.
- Greedy Algorithms: Recognize when a greedy approach is applicable and implement common greedy algorithms.
- Divide and Conquer: Understand how to solve problems by breaking them into smaller, manageable parts.
- Backtracking: Implement solutions for problems like N-Queens or Sudoku solver.
- Graph Algorithms: Dijkstra’s algorithm, Bellman-Ford, Minimum Spanning Tree algorithms.
Here’s an example of implementing a binary search algorithm in C++:
#include <iostream>
#include <vector>
int binarySearch(const std::vector<int>& arr, int target) {
int left = 0;
int right = arr.size() - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1; // Target not found
}
int main() {
std::vector<int> arr = {1, 3, 5, 7, 9, 11, 13, 15};
int target = 7;
int result = binarySearch(arr, target);
if (result != -1) {
std::cout << "Target found at index: " << result << std::endl;
} else {
std::cout << "Target not found in the array." << std::endl;
}
return 0;
}
6. System Design and Scalability
For more senior positions or roles involving distributed systems, you may encounter system design questions. Prepare to discuss:
- Distributed Systems: Understand concepts like load balancing, caching, and data partitioning.
- Scalability: Know how to design systems that can handle increasing loads efficiently.
- Fault Tolerance: Discuss strategies for making systems resilient to failures.
- Real-Time Systems: Given SpaceX’s focus on real-time control systems, understand the principles of designing for low latency and high reliability.
- Data Processing Pipelines: Be familiar with designing systems for ingesting, processing, and analyzing large volumes of data.
When approaching system design questions, follow these steps:
- Clarify requirements and constraints
- Outline the high-level design
- Deep dive into critical components
- Discuss trade-offs and potential bottlenecks
- Propose scaling strategies
7. Coding Practice and Resources
Consistent practice is key to performing well in technical interviews. Utilize the following resources to hone your skills:
- LeetCode: Solve problems regularly, focusing on medium and hard difficulty levels.
- HackerRank: Participate in coding challenges and competitions.
- CodeSignal: Practice with timed coding assessments similar to those used by many companies.
- Project Euler: Tackle mathematically-oriented programming problems.
- GitHub: Contribute to open-source projects to gain practical experience and showcase your skills.
Additionally, consider implementing projects that align with SpaceX’s areas of focus, such as:
- A simple flight simulator
- A satellite tracking application
- A data visualization tool for rocket telemetry
8. Soft Skills and Behavioral Preparation
While technical skills are crucial, don’t neglect the importance of soft skills and behavioral preparation. SpaceX values team players who can communicate effectively and handle high-pressure situations. Prepare for questions about:
- Your past projects and experiences
- How you handle challenges and setbacks
- Your ability to work in a fast-paced, dynamic environment
- Your passion for space exploration and innovation
- Your approach to problem-solving and learning new technologies
Use the STAR method (Situation, Task, Action, Result) to structure your responses to behavioral questions effectively.
9. Conducting Mock Interviews
Practice makes perfect, especially when it comes to technical interviews. Set up mock interviews to simulate the real experience:
- Use platforms like Pramp or interviewing.io to practice with peers.
- Ask friends or mentors in the industry to conduct mock interviews with you.
- Record yourself solving problems out loud to improve your verbal communication skills.
- Time your problem-solving sessions to get comfortable working under pressure.
After each mock interview, reflect on your performance and identify areas for improvement.
10. Final Tips for Success
As you prepare for your SpaceX technical interview, keep these final tips in mind:
- Stay Updated: Follow SpaceX news and developments to show your genuine interest in the company.
- Be Prepared to Code: Practice writing code on a whiteboard or in a simple text editor without auto-completion.
- Think Aloud: Communicate your thought process clearly during problem-solving exercises.
- Ask Questions: Don’t hesitate to seek clarification on problems or requirements.
- Handle Edge Cases: Consider and address edge cases in your solutions to demonstrate thoroughness.
- Optimize Your Solutions: After solving a problem, discuss potential optimizations and trade-offs.
- Show Enthusiasm: Let your passion for space technology and innovation shine through.
Remember, preparation is key, but so is staying calm and confident during the interview. Trust in your abilities and the hard work you’ve put into preparing.
Conclusion
Preparing for a SpaceX technical interview is a challenging but exciting journey. By focusing on strong fundamentals in computer science, mastering key data structures and algorithms, and honing your problem-solving skills, you’ll be well-equipped to tackle whatever questions come your way. Remember that SpaceX is looking for not just technical proficiency, but also individuals who are passionate about pushing the boundaries of space exploration and technology.
As you work through your preparation, leverage the resources and strategies outlined in this guide. Practice consistently, stay curious, and don’t be afraid to dive deep into complex problems. With dedication and the right approach, you’ll be well on your way to launching your career at SpaceX.
Good luck with your interview preparation, and may your coding skills take you to new heights – perhaps even to the stars!