Comprehensive Guide to Apple Technical Interview Preparation
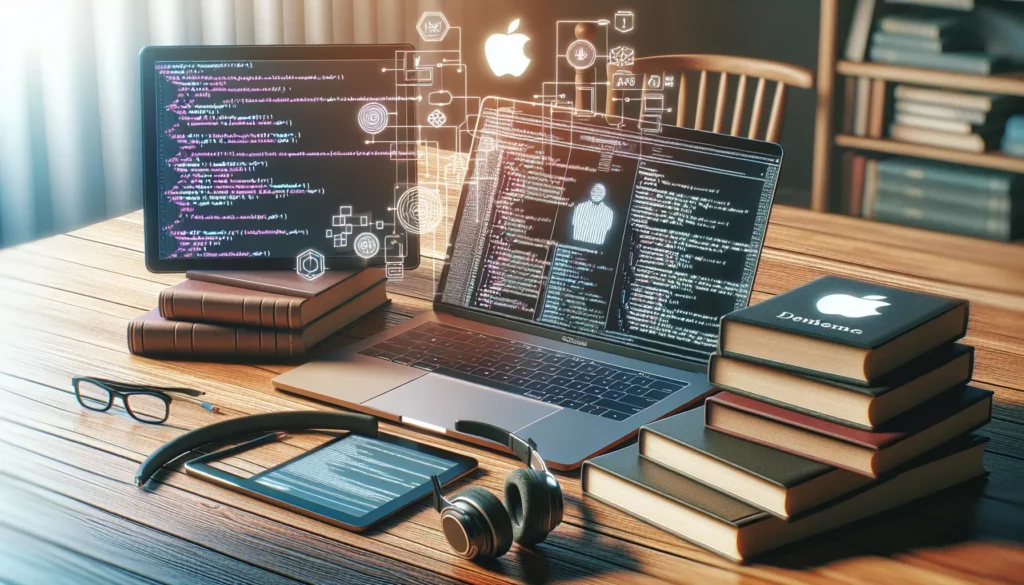
Are you dreaming of landing a coveted position at Apple, one of the world’s most innovative tech giants? The journey to becoming an Apple employee is exciting, but it also requires thorough preparation, especially for the technical interview. This comprehensive guide will walk you through the essential steps and strategies to ace your Apple technical interview.
Table of Contents
- Understanding Apple’s Interview Process
- Technical Skills to Master
- Coding Languages to Focus On
- Data Structures and Algorithms
- System Design and Architecture
- Problem-Solving Techniques
- Behavioral Questions and Soft Skills
- Conducting Mock Interviews
- Recommended Resources and Practice Platforms
- Day of the Interview: Tips and Tricks
- Post-Interview Follow-up
1. Understanding Apple’s Interview Process
Before diving into the technical preparation, it’s crucial to understand Apple’s interview process. Typically, it involves several stages:
- Initial Screening: Usually a phone call with a recruiter to discuss your background and the role.
- Technical Phone Screen: A 45-60 minute interview focusing on basic coding and problem-solving skills.
- On-site Interviews: A series of 4-6 interviews, including coding challenges, system design discussions, and behavioral questions.
- Final Decision: The hiring committee reviews all feedback to make a decision.
Apple values innovation, attention to detail, and a user-centric approach. Keep these core values in mind throughout your preparation and during the interviews.
2. Technical Skills to Master
Apple looks for candidates with a strong foundation in computer science and practical coding skills. Here are key areas to focus on:
- Data Structures and Algorithms
- Object-Oriented Programming
- Operating Systems Concepts
- Networking Fundamentals
- Database Management
- Mobile Development (iOS)
- Web Technologies
- Version Control (Git)
Ensure you have a solid understanding of these concepts and can apply them practically in coding scenarios.
3. Coding Languages to Focus On
While Apple uses various programming languages, some are more prevalent than others. Focus on mastering:
- Swift: Apple’s primary language for iOS development
- Objective-C: Still relevant for maintaining legacy iOS code
- C/C++: Used in system-level programming and performance-critical applications
- Python: Often used for scripting and backend development
- Java: Useful for cross-platform development and backend services
Here’s a simple example of a Swift function to reverse a string:
func reverseString(_ s: String) -> String {
return String(s.reversed())
}
// Usage
let original = "Hello, Apple!"
let reversed = reverseString(original)
print(reversed) // Output: !elppA ,olleH
Practice implementing common algorithms and data structures in these languages to build your proficiency.
4. Data Structures and Algorithms
A strong grasp of data structures and algorithms is crucial for Apple’s technical interviews. Focus on:
Data Structures:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
Algorithms:
- Sorting (Quick Sort, Merge Sort, Heap Sort)
- Searching (Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic Programming
- Greedy Algorithms
- Recursion and Backtracking
Here’s an example of implementing a binary search algorithm in Python:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
# Usage
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15]
target = 7
result = binary_search(sorted_array, target)
print(f"Target {target} found at index: {result}") # Output: Target 7 found at index: 3
Practice implementing these data structures and algorithms from scratch to deeply understand their operations and time complexities.
5. System Design and Architecture
For more senior positions, Apple often includes system design questions in their interviews. These assess your ability to design scalable, efficient, and robust systems. Key areas to study include:
- Scalability and Load Balancing
- Database Sharding
- Caching Mechanisms
- Microservices Architecture
- API Design
- Data Storage Solutions
- Content Delivery Networks (CDNs)
Practice designing systems like:
- A distributed file storage system (like iCloud)
- A real-time messaging application
- A video streaming service
- An app store backend
When approaching system design questions, remember to:
- Clarify requirements and constraints
- Outline a high-level design
- Deep dive into critical components
- Discuss trade-offs and potential improvements
6. Problem-Solving Techniques
Apple values candidates who can approach problems methodically. Develop a structured approach to problem-solving:
- Understand the Problem: Clarify any ambiguities and constraints.
- Plan Your Approach: Outline your solution before coding.
- Implement the Solution: Write clean, efficient code.
- Test and Debug: Consider edge cases and potential errors.
- Optimize: Improve time and space complexity if possible.
Practice verbalizing your thought process as you solve problems. This skill is crucial during interviews to demonstrate your problem-solving abilities.
Here’s an example of how you might approach a problem to find the longest palindromic substring:
def longest_palindrome(s: str) -> str:
if not s:
return ""
start, max_len = 0, 1
def expand_around_center(left: int, right: int) -> int:
while left >= 0 and right < len(s) and s[left] == s[right]:
left -= 1
right += 1
return right - left - 1
for i in range(len(s)):
len1 = expand_around_center(i, i)
len2 = expand_around_center(i, i + 1)
length = max(len1, len2)
if length > max_len:
start = i - (length - 1) // 2
max_len = length
return s[start:start + max_len]
# Usage
s = "babad"
result = longest_palindrome(s)
print(f"Longest palindromic substring: {result}") # Output: Longest palindromic substring: bab
This solution demonstrates efficient problem-solving by using the expand around center technique, which is more space-efficient than dynamic programming for this particular problem.
7. Behavioral Questions and Soft Skills
While technical skills are crucial, Apple also values candidates who align with their culture and possess strong soft skills. Prepare for behavioral questions that assess:
- Teamwork and Collaboration
- Problem-solving in real-world scenarios
- Adaptability and Learning Agility
- Leadership and Initiative
- Passion for Technology and Innovation
Use the STAR method (Situation, Task, Action, Result) to structure your responses. Here’s an example:
Question: “Tell me about a time when you had to work on a challenging project with a tight deadline.”
Answer:
- Situation: “In my previous role, we were tasked with developing a new feature for our mobile app with only three weeks until the release date.”
- Task: “My responsibility was to implement the backend API and ensure it integrated smoothly with the frontend.”
- Action: “I broke down the project into smaller, manageable tasks and prioritized them. I also set up daily stand-ups with the frontend team to ensure alignment and quick problem resolution.”
- Result: “We successfully launched the feature on time, and it received positive user feedback, increasing our app’s engagement by 15%.”
Practice answering behavioral questions to showcase your experiences and align them with Apple’s values.
8. Conducting Mock Interviews
Mock interviews are an excellent way to prepare for the real thing. They help you:
- Practice articulating your thoughts clearly
- Get comfortable with the interview format
- Receive feedback on your performance
- Identify areas for improvement
Consider these options for mock interviews:
- Peer Practice: Partner with a friend or colleague in the tech industry.
- Online Platforms: Use services like Pramp or InterviewBit that offer free peer-to-peer mock interviews.
- Professional Services: Consider paid services with experienced interviewers for more targeted feedback.
- Coding Challenges: Participate in timed coding challenges on platforms like LeetCode or HackerRank to simulate interview pressure.
After each mock interview, reflect on your performance and create an action plan to address any weaknesses.
9. Recommended Resources and Practice Platforms
To supplement your preparation, leverage these resources:
Books:
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “Elements of Programming Interviews” by Adnan Aziz, Tsung-Hsien Lee, and Amit Prakash
- “System Design Interview” by Alex Xu
Online Platforms:
- LeetCode: For coding practice and interview preparation
- HackerRank: Offers a wide range of programming challenges
- AlgoExpert: Provides curated coding interview questions with video explanations
- Educative.io: Offers courses on system design and coding interviews
Websites and Blogs:
- GeeksforGeeks: Comprehensive resource for computer science topics
- Apple Developer Documentation: Familiarize yourself with Apple’s technologies
- Stack Overflow: For practical coding problems and solutions
Remember to focus on understanding concepts deeply rather than memorizing solutions. Apple values candidates who can apply their knowledge to solve novel problems.
10. Day of the Interview: Tips and Tricks
As the big day approaches, keep these tips in mind:
- Get a Good Night’s Sleep: Ensure you’re well-rested and alert.
- Arrive Early: For on-site interviews, arrive at least 15 minutes early. For virtual interviews, test your setup in advance.
- Dress Appropriately: While Apple has a relaxed dress code, opt for smart casual attire to make a good impression.
- Bring Necessities: Have a notebook, pen, and copies of your resume handy.
- Stay Calm: Take deep breaths and remember that nervousness is normal.
- Listen Carefully: Pay attention to the details in each question.
- Think Aloud: Share your thought process as you work through problems.
- Ask Questions: Demonstrate your interest by asking thoughtful questions about the role and team.
- Be Yourself: Show your personality and passion for technology.
During coding interviews, remember to:
- Clarify requirements before starting to code
- Consider edge cases and potential optimizations
- Write clean, well-commented code
- Test your solution with sample inputs
11. Post-Interview Follow-up
After the interview:
- Send a Thank You Email: Within 24 hours, send a brief email thanking the interviewer(s) for their time.
- Reflect on the Experience: Note down the questions asked and areas where you felt confident or struggled.
- Follow Up if Necessary: If you don’t hear back within the timeframe provided, it’s appropriate to send a polite follow-up email.
- Prepare for Next Steps: If invited for further rounds, continue your preparation focusing on areas that were emphasized in the previous interview.
Remember, regardless of the outcome, each interview is a learning experience that will help you grow as a professional.
Conclusion
Preparing for an Apple technical interview requires dedication, thorough knowledge of computer science fundamentals, and the ability to apply this knowledge to solve complex problems. By following this guide and consistently practicing, you’ll be well-equipped to showcase your skills and land that dream job at Apple.
Remember that the journey doesn’t end with the interview. The tech industry is constantly evolving, so maintain a habit of continuous learning and stay updated with the latest trends and technologies in your field.
Good luck with your Apple interview! With the right preparation and mindset, you’re one step closer to joining one of the world’s most innovative tech companies.