Compiler Theory and Design: Unveiling the Magic Behind Programming Languages
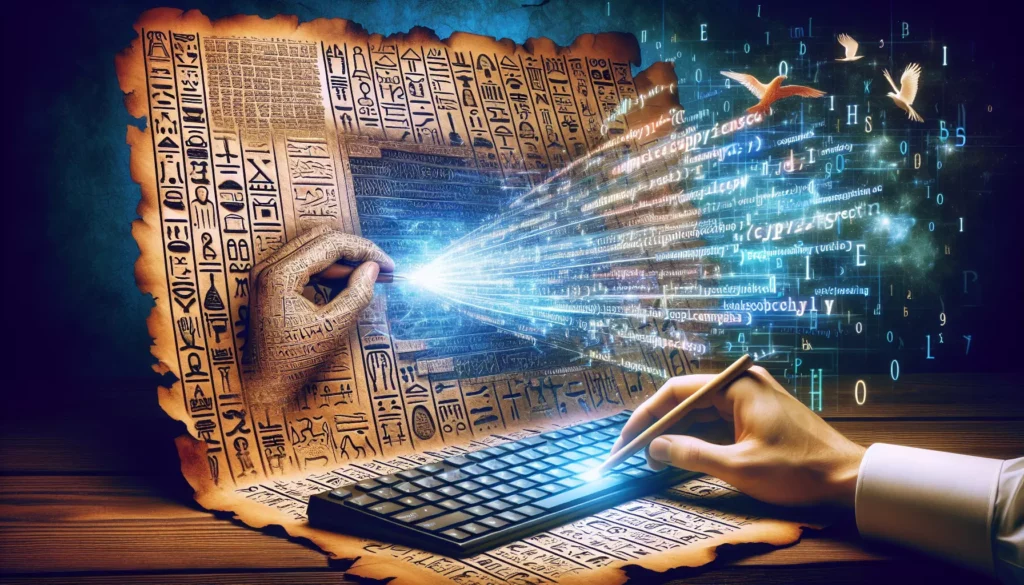
In the vast realm of computer science, few areas are as fundamental and intriguing as compiler theory and design. This field serves as the bridge between human-readable programming languages and the machine code that computers can execute. For anyone serious about mastering the art of programming, understanding how compilers work is not just beneficial—it’s essential. In this comprehensive guide, we’ll dive deep into the world of compiler theory and design, exploring how programming languages are transformed into executable code and why this knowledge is crucial for every aspiring programmer.
What is Compiler Theory?
Compiler theory is the study of how programming languages are translated into executable machine code. It encompasses the principles, techniques, and algorithms used in the design and implementation of compilers. A compiler is a special program that processes statements written in a particular programming language and turns them into machine language or “code” that a computer’s processor uses.
The importance of compiler theory extends far beyond just creating compilers. It provides insights into:
- Language design principles
- Code optimization techniques
- Error detection and handling
- The intersection of theory and practice in computer science
The Compilation Process: From Source to Executable
To understand compiler theory, it’s crucial to grasp the steps involved in the compilation process. Let’s break it down:
1. Lexical Analysis
The first step in compilation is lexical analysis, also known as scanning or tokenization. During this phase, the compiler breaks down the source code into a series of tokens. Each token represents a string of characters that has a collective meaning, such as keywords, identifiers, or operators.
For example, consider the following line of C code:
int main() { return 0; }
The lexical analyzer would break this down into tokens like:
int
(keyword)main
(identifier)(
(left parenthesis))
(right parenthesis){
(left brace)return
(keyword)0
(integer literal);
(semicolon)}
(right brace)
2. Syntax Analysis
Once the source code has been tokenized, the syntax analyzer (also called a parser) takes over. This component checks if the sequence of tokens adheres to the grammatical rules of the programming language. It typically constructs an Abstract Syntax Tree (AST) that represents the structure of the program.
If we continue with our previous example, the parser would verify that the tokens form a valid function definition according to C language rules. It would create an AST that might look something like this (simplified):
Function Declaration
├── Return Type: int
├── Name: main
├── Parameters: none
└── Body
└── Return Statement
└── Value: 0
3. Semantic Analysis
The semantic analyzer checks the meaning of the program. It ensures that the code makes sense logically. This phase includes tasks such as:
- Type checking
- Scope resolution
- Checking for undeclared variables
- Verifying that function calls match their definitions
In our simple example, the semantic analyzer would verify that the return
statement is valid within the main
function and that returning an integer is consistent with the function’s declared return type.
4. Intermediate Code Generation
After semantic analysis, many compilers generate an intermediate representation of the code. This representation is closer to machine code but is not tied to any specific machine architecture. Common forms of intermediate representation include Three-Address Code (TAC) or Quadruples.
Our example might be translated to something like:
FUNCTION main
RETURN 0
END FUNCTION
5. Code Optimization
The optimization phase aims to improve the intermediate code to make it more efficient. This can involve various techniques such as:
- Dead code elimination
- Constant folding
- Loop unrolling
- Common subexpression elimination
Our simple example doesn’t offer much room for optimization, but in more complex programs, this phase can significantly improve performance.
6. Code Generation
The final phase translates the optimized intermediate code into the target machine code. This involves selecting appropriate machine instructions, allocating registers, and handling machine-specific details.
The resulting machine code might look something like this (in x86 assembly):
main:
mov eax, 0
ret
Interpreters vs. Compilers
While we’ve focused on compilers, it’s worth noting the distinction between compilers and interpreters. Both are tools for translating high-level programming languages, but they work differently:
Compilers
- Translate the entire source code into machine code before execution
- Produce a standalone executable file
- Generally offer better performance for the final program
- Examples: C, C++, Rust compilers
Interpreters
- Translate and execute the source code line by line
- Do not produce a separate executable file
- Offer more flexibility and ease of debugging
- Examples: Python, Ruby, JavaScript interpreters
Some modern language implementations use a hybrid approach, compiling to an intermediate bytecode which is then interpreted or just-in-time (JIT) compiled. Java and C# are prime examples of this approach.
Advanced Topics in Compiler Theory
As you delve deeper into compiler theory, you’ll encounter several advanced topics that are crucial for building efficient and powerful compilers:
1. Type Systems
Type systems are a fundamental part of programming language design and compiler theory. They define how a language classifies values and expressions into types, how it manipulates those types, and how they interact. Understanding type systems is crucial for:
- Ensuring program correctness
- Enabling compiler optimizations
- Providing abstraction and documentation
Different languages implement various type systems, from static typing in languages like C++ and Java to dynamic typing in Python and Ruby. Some modern languages like Haskell and Rust implement advanced type systems with features like algebraic data types and affine types.
2. Static Analysis
Static analysis is the process of analyzing code without executing it. It’s a powerful technique used in modern compilers and development tools to:
- Detect potential bugs and security vulnerabilities
- Enforce coding standards
- Optimize code
- Generate documentation
Tools like lint for C, or more modern alternatives like ESLint for JavaScript, are examples of static analysis in action.
3. Code Optimization Techniques
Code optimization is a vast field within compiler theory. Some advanced optimization techniques include:
- Data flow analysis: Analyzing how data is used throughout a program
- Loop optimizations: Techniques like loop unrolling, fusion, and vectorization
- Interprocedural optimizations: Optimizing across function boundaries
- Just-In-Time (JIT) compilation: Compiling code at runtime based on actual usage patterns
4. Parallel and Distributed Compilation
As programs grow larger and multi-core processors become ubiquitous, parallel and distributed compilation techniques are becoming increasingly important. These involve:
- Distributing compilation tasks across multiple cores or machines
- Managing dependencies between different parts of the program
- Optimizing for parallel execution on the target hardware
5. Domain-Specific Languages (DSLs)
DSLs are languages tailored to specific application domains. Compiler theory plays a crucial role in designing and implementing DSLs, which can offer significant productivity gains in their target domains. Examples include:
- SQL for database queries
- Regular expressions for text processing
- Verilog for hardware description
The Relevance of Compiler Theory in Modern Programming
You might wonder why studying compiler theory is relevant if you’re not planning to build a compiler. The truth is, understanding compiler theory has far-reaching benefits for any programmer:
1. Writing More Efficient Code
When you understand how compilers work, you can write code that’s easier for the compiler to optimize. This knowledge allows you to:
- Avoid constructs that are difficult to optimize
- Leverage compiler-friendly patterns
- Make informed decisions about language features and their performance implications
2. Debugging and Troubleshooting
Compiler errors can sometimes be cryptic. With a solid understanding of compiler theory, you can:
- Better interpret error messages
- Understand the root causes of compilation issues
- More effectively use debugging tools
3. Language Design and Evolution
As programming languages evolve, understanding compiler theory allows you to:
- Appreciate the rationale behind new language features
- Contribute to language design discussions
- Create your own DSLs when needed
4. Working with New Technologies
Many modern development technologies are built on compiler theory principles:
- Transpilers (like Babel for JavaScript)
- Build tools (like webpack)
- Static type checkers (like TypeScript)
Understanding the underlying principles makes working with these tools more intuitive and effective.
Getting Started with Compiler Theory
If you’re intrigued by compiler theory and want to dive deeper, here are some steps you can take:
1. Study the Fundamentals
Start with the basics of formal languages, automata theory, and parsing techniques. Resources like “Introduction to the Theory of Computation” by Michael Sipser can provide a solid foundation.
2. Learn About Parsing Techniques
Understanding different parsing techniques is crucial. Study top-down and bottom-up parsing, and familiarize yourself with tools like Lex and Yacc (or their modern equivalents like Flex and Bison).
3. Explore Existing Compilers
Many compilers are open-source. Exploring the source code of compilers like GCC, LLVM, or even simpler compilers can provide valuable insights.
4. Build a Simple Compiler
Nothing beats hands-on experience. Try building a simple compiler for a subset of a programming language. This project will solidify your understanding of the compilation process.
5. Keep Up with Research
Compiler theory is an active area of research. Following academic papers and attending conferences like PLDI (Programming Language Design and Implementation) can keep you updated on the latest developments.
Conclusion
Compiler theory and design form the backbone of modern programming languages and tools. By understanding how programming languages are interpreted or compiled into executable code, you gain insights that can dramatically improve your skills as a programmer. Whether you’re optimizing code, debugging complex issues, or even designing your own language features, a solid grasp of compiler theory will serve you well.
As you continue your journey in computer science and software development, remember that compiler theory is not just for those building compilers. It’s a fundamental skill that can elevate your understanding of programming languages, improve your coding practices, and open up new avenues for innovation in your software development career.
So, dive in, explore the fascinating world of compiler theory, and watch as it transforms your approach to programming. The knowledge you gain will be an invaluable asset in your toolkit as a software developer, empowering you to write better, more efficient code and to tackle complex programming challenges with confidence.