Communicating the Why: Explaining Your Design Choices and Trade-offs During a Coding Interview
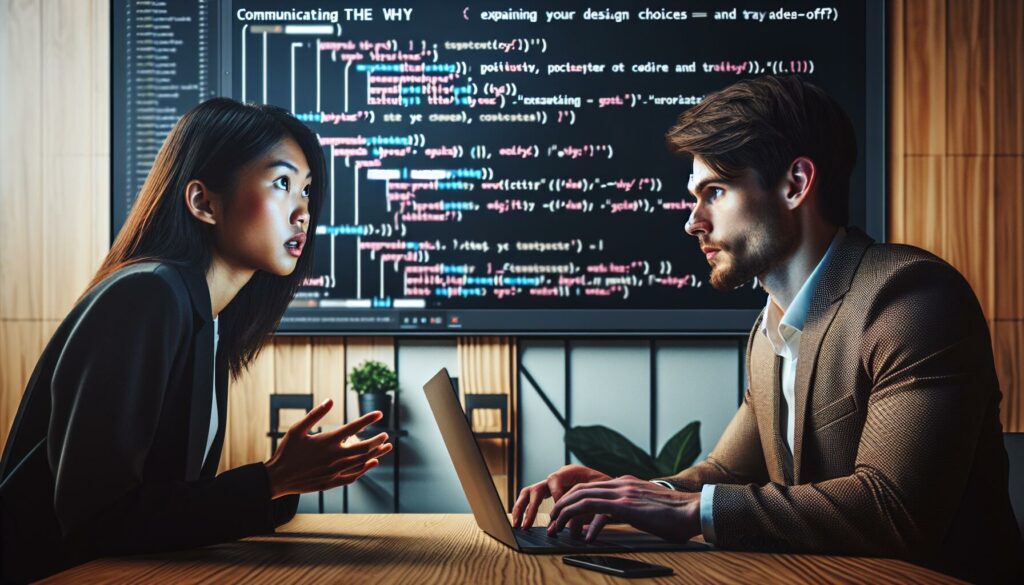
In the world of software development, technical prowess is just one piece of the puzzle. The ability to effectively communicate your thought process, design choices, and trade-offs is equally crucial, especially during coding interviews. This skill can set you apart from other candidates and demonstrate not just your coding abilities, but also your problem-solving approach and decision-making skills. In this comprehensive guide, we’ll explore how to articulate your reasoning behind design decisions, explain trade-offs, and showcase your critical thinking skills during a coding interview.
The Importance of Communicating Design Choices
Before diving into the specifics of how to communicate your design choices, it’s essential to understand why this skill is so valuable:
- Demonstrates problem-solving skills: By explaining your thought process, you show interviewers how you approach and solve complex problems.
- Highlights critical thinking: Articulating trade-offs and alternatives demonstrates your ability to consider multiple perspectives and make informed decisions.
- Shows collaboration potential: Clear communication of technical concepts is crucial for working effectively in a team environment.
- Reveals depth of knowledge: Explaining the pros and cons of different approaches showcases your understanding of various algorithms and data structures.
- Provides insight into your working style: How you communicate your choices gives interviewers a glimpse into how you might interact with colleagues and stakeholders.
Preparing to Explain Your Design Choices
Before your interview, it’s helpful to prepare a framework for explaining your design choices. Here’s a step-by-step approach you can follow:
- Understand the problem thoroughly: Before jumping into a solution, make sure you fully grasp the problem requirements, constraints, and goals.
- Consider multiple approaches: Think about different ways to solve the problem, even if you quickly settle on one.
- Analyze trade-offs: For each approach, consider the pros and cons in terms of time complexity, space complexity, readability, and scalability.
- Choose and justify: Select the approach that best fits the problem requirements and be prepared to explain why.
- Be ready to discuss alternatives: Even if not asked, be prepared to discuss why you didn’t choose other approaches.
Explaining Algorithm Choices
When it comes to explaining why you chose a particular algorithm, focus on these key aspects:
1. Time and Space Complexity
Always be prepared to discuss the time and space complexity of your chosen algorithm. Use Big O notation to express these complexities and explain what they mean in practical terms.
Example: “I chose to use a hash table for this problem because it provides O(1) average-case time complexity for insertions and lookups, which is crucial given the large dataset we’re working with. The trade-off is that it requires O(n) space complexity, but in this case, the speed benefit outweighs the additional memory usage.”
2. Problem Constraints
Explain how your chosen algorithm addresses the specific constraints of the problem.
Example: “Given that the problem requires us to find the k-th largest element in an unsorted array, I opted for the QuickSelect algorithm. It has an average-case time complexity of O(n), which is more efficient than sorting the entire array (O(n log n)) when we only need to find a single element. The trade-off is that it modifies the input array, which might not be suitable if we needed to preserve the original order.”
3. Scalability
Discuss how your solution would perform with larger inputs or in a distributed environment.
Example: “I chose a divide-and-conquer approach for this problem because it naturally lends itself to parallelization. While a simple iterative solution might be faster for small inputs, this approach scales better for large datasets that could be processed across multiple machines.”
Explaining Data Structure Choices
When explaining your choice of data structure, consider the following points:
1. Access Patterns
Explain how the chosen data structure aligns with the required access patterns of the problem.
Example: “I decided to use a linked list for this task because we frequently need to insert and delete elements at the beginning of the list. These operations are O(1) for a linked list, whereas they would be O(n) for an array due to the need for shifting elements.”
2. Memory Usage
Discuss the memory implications of your chosen data structure.
Example: “For this problem, I chose to use a bit vector instead of a boolean array. While both would work, the bit vector is significantly more memory-efficient, using about 8 times less space. The trade-off is slightly more complex bit manipulation operations, but the space savings are worth it for large inputs.”
3. Built-in Functionality
Explain how the inherent properties of a data structure benefit your solution.
Example: “I opted for a priority queue (implemented as a max heap) for this task because it automatically maintains the elements in sorted order as we insert them. This gives us O(log n) insertion time and O(1) access to the maximum element, which is ideal for our streaming data scenario.”
Communicating Trade-offs
Being able to articulate trade-offs is a crucial skill that demonstrates your ability to think critically about different solutions. Here are some common trade-offs you might need to explain:
1. Time vs. Space
This is one of the most common trade-offs in algorithm design. Be prepared to explain when you prioritize one over the other.
Example: “In this solution, I used dynamic programming with memoization. This approach trades space for time, using additional memory to store computed results and avoid redundant calculations. The time complexity improves from O(2^n) to O(n), but at the cost of O(n) additional space. Given that the problem emphasizes speed and the input size is manageable, I believe this is an acceptable trade-off.”
2. Simplicity vs. Efficiency
Sometimes, a more complex solution might be more efficient. Be ready to justify when you choose one over the other.
Example: “For this problem, I chose a simple iterative solution over a more efficient but complex recursive approach. While the recursive solution has a slightly better time complexity, the iterative version is more readable and easier to debug. Given that the performance difference is negligible for the expected input sizes, I prioritized code simplicity and maintainability.”
3. Flexibility vs. Specialization
Discuss when you choose a more general solution versus a specialized one.
Example: “I implemented a general-purpose sorting algorithm rather than a counting sort, even though counting sort would be faster for this specific input range. The trade-off is that this solution is more flexible and can handle a wider range of inputs, which could be beneficial if the requirements change in the future.”
Practical Tips for Communicating During the Interview
Now that we’ve covered what to communicate, let’s discuss how to effectively convey your thoughts during the interview:
1. Think Aloud
Verbalize your thought process as you work through the problem. This gives the interviewer insight into your problem-solving approach and allows them to provide hints if needed.
Example: “I’m considering two approaches here: a hash table for O(1) lookups or a balanced binary search tree for maintaining sorted order. Let me think through the trade-offs…”
2. Use Clear and Concise Language
Avoid jargon unless you’re sure the interviewer is familiar with it. Explain concepts in simple terms.
Example: Instead of saying “This algorithm has O(n) time complexity,” you might say, “This algorithm’s running time grows linearly with the input size, meaning it scales well for larger inputs.”
3. Use Visual Aids
If possible, use diagrams or pseudocode to illustrate your ideas. This can make complex concepts easier to understand.
Example: “Let me draw out how this binary tree would look after we’ve inserted the first few elements…”
4. Invite Discussion
Engage the interviewer in your thought process. This shows you’re open to feedback and collaboration.
Example: “I’m leaning towards using a heap for this part of the solution. What are your thoughts on that approach?”
5. Be Honest About Uncertainties
If you’re not sure about something, it’s better to admit it than to try to bluff your way through.
Example: “I’m not entirely certain about the worst-case time complexity of this algorithm. My initial thought is O(n log n), but I’d want to analyze it more carefully to be sure.”
Example Scenarios
Let’s look at a couple of example scenarios to see how you might communicate your design choices and trade-offs:
Scenario 1: Implementing a Cache
Interviewer: “Design and implement a cache with get and put operations, both running in O(1) time.”
Your Response: “For this problem, I’m going to use a combination of a hash map and a doubly linked list. Here’s my reasoning:
The hash map will give us O(1) access time for both get and put operations, which meets our time complexity requirement. The linked list will help us implement a Least Recently Used (LRU) eviction policy efficiently.
The trade-off here is that we’re using more memory by having both data structures. An alternative would be to use just a hash map, which would use less memory but wouldn’t allow for efficient implementation of an eviction policy.
I chose this approach because:
- It meets the O(1) time requirement for both operations.
- It allows for efficient implementation of an LRU policy, which is a common and effective caching strategy.
- The additional memory usage is justified by the performance benefits and added functionality.
One downside to consider is that this implementation is more complex than a simple hash map-only solution. However, I believe the benefits outweigh this drawback for a production-level cache implementation.”
Scenario 2: Finding the Kth Largest Element
Interviewer: “Implement a function to find the kth largest element in an unsorted array.”
Your Response: “For this problem, I’m going to use the QuickSelect algorithm. Here’s my thought process:
We could solve this by sorting the array and then accessing the kth element from the end, but that would have a time complexity of O(n log n). QuickSelect, on the other hand, has an average-case time complexity of O(n), which is more efficient.
The trade-offs to consider are:
- Time Complexity: QuickSelect is O(n) on average, but O(n^2) in the worst case. However, the worst case is rare with good pivot selection.
- Space Complexity: QuickSelect uses O(1) extra space, which is better than some alternatives like using a heap, which would require O(k) space.
- Input Modification: QuickSelect modifies the input array, which might not be acceptable in all scenarios.
I chose QuickSelect because:
- It’s more efficient than sorting for this specific problem.
- It uses minimal extra space.
- The average-case performance is excellent, and we can mitigate the worst-case scenario with good pivot selection.
An alternative approach would be using a min-heap of size k, which would guarantee O(n log k) time complexity. This might be preferable if we needed to find the kth largest element frequently in a stream of data, but for a one-time operation on a static array, QuickSelect is generally more efficient.”
Conclusion
Effectively communicating your design choices and trade-offs during a coding interview is a skill that can significantly improve your performance and showcase your problem-solving abilities. By clearly articulating your thought process, explaining the reasoning behind your decisions, and demonstrating an understanding of alternative approaches, you provide interviewers with valuable insights into your technical knowledge and critical thinking skills.
Remember, the goal is not just to solve the problem, but to demonstrate how you approach complex challenges, make informed decisions, and communicate technical concepts clearly. Practice explaining your choices out loud as you solve coding problems, and don’t hesitate to discuss trade-offs even if not explicitly asked.
As you continue to develop your coding skills on platforms like AlgoCademy, make it a habit to verbalize your thought process and consider multiple approaches to each problem. This practice will not only prepare you for coding interviews but also make you a more effective communicator and collaborator in your future software development career.
By mastering the art of communicating the ‘why’ behind your code, you’ll set yourself apart as a thoughtful, articulate, and skilled developer ready to tackle the challenges of modern software engineering.