Common Types of Questions Asked in Technical Interviews
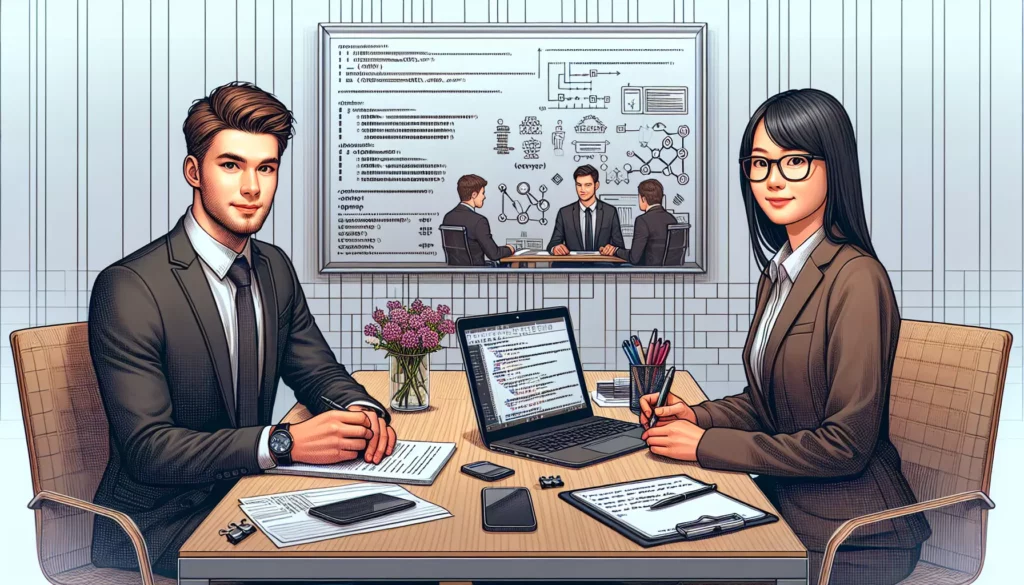
Technical interviews are a crucial part of the hiring process for software engineering and other tech-related positions. These interviews are designed to assess a candidate’s technical skills, problem-solving abilities, and overall fit for the role. As you prepare for your next technical interview, it’s essential to understand the common types of questions you might encounter. In this comprehensive guide, we’ll explore the various categories of questions typically asked in technical interviews, providing examples and tips to help you succeed.
1. Algorithmic Problem-Solving Questions
Algorithmic problem-solving questions are among the most common and important types of questions asked in technical interviews. These questions assess your ability to think critically, analyze problems, and develop efficient solutions using data structures and algorithms.
Examples of Algorithmic Questions:
- Implement a function to reverse a linked list
- Find the kth largest element in an unsorted array
- Determine if a given string is a palindrome
- Implement a binary search algorithm
- Design an algorithm to find the longest common subsequence of two strings
When tackling algorithmic questions, it’s crucial to:
- Clarify the problem and any constraints
- Consider edge cases and input validation
- Discuss your approach before diving into coding
- Analyze the time and space complexity of your solution
- Optimize your solution if possible
2. Data Structure Questions
Data structure questions focus on your understanding and implementation of various data structures. These questions often overlap with algorithmic problems, as efficient solutions often rely on choosing the right data structure.
Common Data Structures to Study:
- Arrays and Dynamic Arrays
- Linked Lists (Singly and Doubly Linked)
- Stacks and Queues
- Hash Tables
- Trees (Binary Trees, Binary Search Trees, AVL Trees)
- Heaps
- Graphs
Example Question: “Implement a stack using two queues.”
class Stack:
def __init__(self):
self.queue1 = []
self.queue2 = []
def push(self, x):
self.queue1.append(x)
def pop(self):
if not self.queue1 and not self.queue2:
return None
while len(self.queue1) > 1:
self.queue2.append(self.queue1.pop(0))
result = self.queue1.pop(0)
self.queue1, self.queue2 = self.queue2, self.queue1
return result
def top(self):
if not self.queue1 and not self.queue2:
return None
while len(self.queue1) > 1:
self.queue2.append(self.queue1.pop(0))
result = self.queue1[0]
self.queue2.append(self.queue1.pop(0))
self.queue1, self.queue2 = self.queue2, self.queue1
return result
def empty(self):
return len(self.queue1) == 0 and len(self.queue2) == 0
3. System Design Questions
System design questions are particularly common in interviews for more senior positions or at larger tech companies. These questions assess your ability to design scalable, efficient, and robust systems.
Examples of System Design Questions:
- Design a URL shortening service like bit.ly
- How would you design Twitter’s trending topics feature?
- Design a distributed key-value store
- How would you implement a real-time chat application?
- Design a system for a parking lot
When answering system design questions, consider the following aspects:
- Requirements gathering and clarification
- System constraints and scale
- High-level architecture design
- Data model and storage
- API design
- Scalability and performance optimizations
- Fault tolerance and reliability
4. Object-Oriented Design Questions
Object-Oriented Design (OOD) questions test your ability to apply object-oriented programming principles to design software systems. These questions often involve designing classes and their relationships for real-world scenarios.
Examples of OOD Questions:
- Design a parking lot system
- Implement a deck of cards for a card game
- Design a library management system
- Create a class hierarchy for different types of vehicles
- Design an elevator system for a building
When tackling OOD questions, keep in mind the following principles:
- Encapsulation
- Inheritance
- Polymorphism
- Abstraction
- SOLID principles (Single Responsibility, Open-Closed, Liskov Substitution, Interface Segregation, Dependency Inversion)
5. Database and SQL Questions
Database and SQL questions assess your understanding of database concepts, query optimization, and your ability to write efficient SQL queries.
Examples of Database and SQL Questions:
- Write a SQL query to find the second highest salary in a table
- Explain the difference between INNER JOIN and LEFT JOIN
- How would you optimize a slow-running query?
- Describe the ACID properties in database transactions
- Write a query to find duplicate records in a table
Example SQL Query: Find the second highest salary
SELECT MAX(Salary) AS SecondHighestSalary
FROM Employee
WHERE Salary < (SELECT MAX(Salary) FROM Employee);
6. Coding Implementation Questions
Coding implementation questions require you to write actual code to solve a given problem. These questions test your ability to translate your thoughts into working code and your familiarity with the syntax and features of a specific programming language.
Examples of Coding Implementation Questions:
- Implement a function to check if a number is prime
- Write a program to find the factorial of a number
- Implement a basic calculator that can perform addition, subtraction, multiplication, and division
- Write a function to remove duplicates from a sorted array in-place
- Implement a simple string compression algorithm
Example: Implementing a function to check if a number is prime
def is_prime(n):
if n < 2:
return False
for i in range(2, int(n**0.5) + 1):
if n % i == 0:
return False
return True
# Test the function
print(is_prime(17)) # True
print(is_prime(4)) # False
print(is_prime(2)) # True
print(is_prime(1)) # False
7. Behavioral Questions
While not strictly technical, behavioral questions are often included in technical interviews to assess your soft skills, problem-solving approach, and cultural fit within the company.
Examples of Behavioral Questions:
- Describe a challenging project you worked on and how you overcame obstacles
- How do you handle disagreements with team members?
- Tell me about a time when you had to learn a new technology quickly
- How do you prioritize tasks when working on multiple projects?
- Describe a situation where you had to explain a complex technical concept to a non-technical person
When answering behavioral questions, use the STAR method:
- Situation: Describe the context
- Task: Explain your role or responsibility
- Action: Describe the steps you took
- Result: Share the outcome and what you learned
8. Operating System and Concurrency Questions
Questions about operating systems and concurrency concepts are common in technical interviews, especially for roles that involve system-level programming or distributed systems.
Examples of OS and Concurrency Questions:
- Explain the difference between a process and a thread
- What is a deadlock, and how can it be prevented?
- Describe the concept of virtual memory
- How does a context switch work?
- Implement a basic producer-consumer problem using semaphores
Example: Implementing a simple producer-consumer problem in Python
import threading
import queue
import time
import random
# Shared queue
buffer = queue.Queue(maxsize=10)
# Producer function
def producer():
while True:
item = random.randint(1, 100)
buffer.put(item)
print(f"Produced: {item}")
time.sleep(random.random())
# Consumer function
def consumer():
while True:
item = buffer.get()
print(f"Consumed: {item}")
time.sleep(random.random())
# Create and start threads
producer_thread = threading.Thread(target=producer)
consumer_thread = threading.Thread(target=consumer)
producer_thread.start()
consumer_thread.start()
# Wait for threads to complete (they won't in this case)
producer_thread.join()
consumer_thread.join()
9. Networking and Security Questions
For roles that involve network programming or security-related tasks, you might encounter questions about networking protocols, security concepts, and best practices.
Examples of Networking and Security Questions:
- Explain the difference between TCP and UDP
- How does HTTPS work?
- What is a man-in-the-middle attack?
- Describe the OSI model and its layers
- What are some common web application security vulnerabilities?
10. Language-Specific Questions
Depending on the role and the technologies used by the company, you may be asked questions specific to certain programming languages or frameworks.
Examples of Language-Specific Questions:
- Python: Explain the difference between a list and a tuple
- Java: What is the difference between an interface and an abstract class?
- JavaScript: Describe the event loop and how asynchronous operations work
- C++: What is the rule of three (or rule of five in modern C++)?
- Ruby: Explain the difference between blocks, procs, and lambdas
Conclusion
Technical interviews can be challenging, but with proper preparation and understanding of the common types of questions, you can significantly improve your chances of success. Remember to:
- Practice regularly using platforms like AlgoCademy, LeetCode, or HackerRank
- Review fundamental computer science concepts and data structures
- Stay up-to-date with industry trends and best practices
- Work on your problem-solving skills and ability to communicate your thought process
- Prepare examples from your past projects to illustrate your skills and experience
By familiarizing yourself with these common types of questions and honing your skills in each area, you’ll be well-prepared to tackle technical interviews with confidence. Remember that the goal of these interviews is not just to test your knowledge but also to assess your problem-solving approach and how you think through complex challenges. Good luck with your upcoming interviews!