Common Mistakes Candidates Make in Whiteboard Interviews
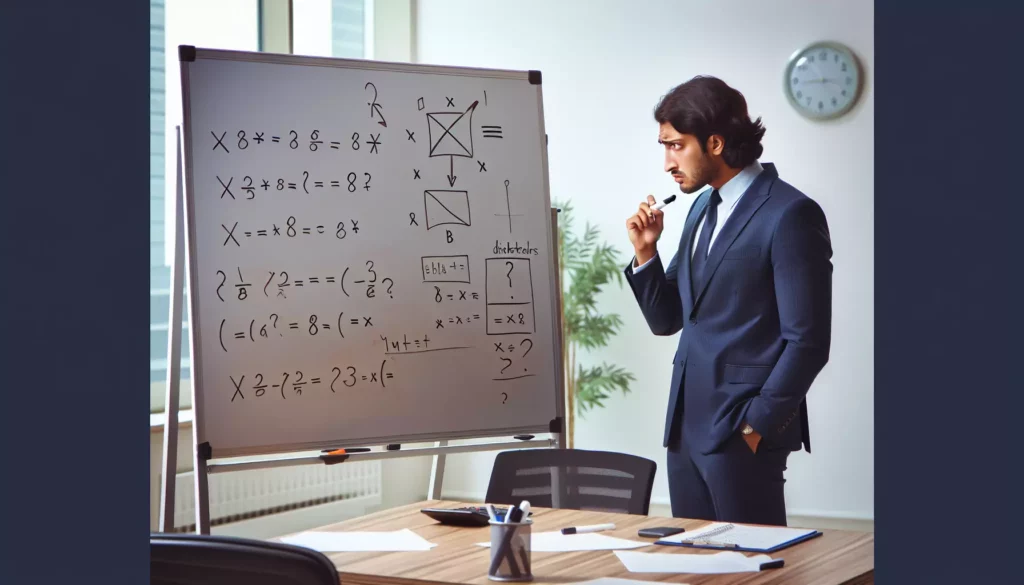
Whiteboard interviews are a crucial part of the technical interview process, especially for software engineering positions at major tech companies. These interviews test a candidate’s problem-solving skills, coding abilities, and communication under pressure. However, many candidates make common mistakes that can significantly impact their performance and chances of landing their dream job. In this comprehensive guide, we’ll explore the most frequent errors candidates make during whiteboard interviews and provide actionable tips on how to avoid them.
1. Failing to Clarify the Problem
One of the most critical mistakes candidates make is diving into coding without fully understanding the problem at hand. This often leads to wasted time and incorrect solutions.
Why it’s a problem:
- Misinterpreting the question can lead to solving the wrong problem entirely.
- Overlooking important details or constraints can result in an incomplete or incorrect solution.
- It demonstrates poor communication skills and a lack of attention to detail.
How to avoid it:
- Take time to read the problem carefully and ask clarifying questions.
- Repeat the problem statement back to the interviewer to ensure understanding.
- Discuss any assumptions you’re making about the problem.
- Ask about input constraints, expected output format, and edge cases.
2. Not Planning Before Coding
Many candidates feel pressured to start writing code immediately, without taking the time to plan their approach. This can lead to disorganized solutions and difficulty explaining their thought process.
Why it’s a problem:
- Lack of planning often results in inefficient or overly complicated solutions.
- It becomes harder to explain your thought process to the interviewer.
- Making significant changes mid-solution can be time-consuming and error-prone.
How to avoid it:
- Spend a few minutes outlining your approach before writing any code.
- Discuss your high-level strategy with the interviewer.
- Break down the problem into smaller, manageable steps.
- Consider multiple approaches and discuss trade-offs with the interviewer.
3. Poor Time Management
Whiteboard interviews often have time constraints, and poor time management can lead to incomplete solutions or rushed explanations.
Why it’s a problem:
- Spending too much time on one aspect of the problem can leave insufficient time for others.
- Rushing through the solution increases the likelihood of errors.
- Poor time management can create unnecessary stress and anxiety.
How to avoid it:
- Ask the interviewer about the expected time frame for solving the problem.
- Set mental checkpoints for different stages of your solution.
- If you’re stuck, communicate with the interviewer and ask for hints if necessary.
- Practice solving problems under timed conditions before the actual interview.
4. Neglecting to Think Aloud
Some candidates work silently, making it difficult for the interviewer to understand their thought process and problem-solving approach.
Why it’s a problem:
- Interviewers can’t assess your problem-solving skills if they can’t follow your thinking.
- Silent candidates miss opportunities to demonstrate their communication skills.
- It’s harder for interviewers to provide guidance or hints when needed.
How to avoid it:
- Verbalize your thoughts as you work through the problem.
- Explain your reasoning for choosing specific data structures or algorithms.
- Discuss trade-offs and alternative approaches you’re considering.
- Ask for feedback or confirmation from the interviewer as you progress.
5. Ignoring Edge Cases and Error Handling
Many candidates focus solely on the happy path, neglecting to consider edge cases or potential errors in their code.
Why it’s a problem:
- Real-world code needs to handle various scenarios, including unexpected inputs.
- Failing to consider edge cases can lead to bugs and system failures.
- It demonstrates a lack of thoroughness and attention to detail.
How to avoid it:
- After implementing the main solution, systematically consider edge cases.
- Discuss potential error scenarios with the interviewer.
- Implement basic error handling and input validation in your code.
- Test your solution with various inputs, including edge cases.
6. Writing Messy or Unreadable Code
In the pressure of the interview, some candidates write code that is difficult to read or understand, even if it’s functionally correct.
Why it’s a problem:
- Unreadable code is harder to debug and maintain.
- It makes it difficult for the interviewer to follow your logic.
- Poor code organization can lead to mistakes and oversights.
How to avoid it:
- Use clear and consistent indentation.
- Write descriptive variable and function names.
- Break down complex logic into smaller, well-named functions.
- Add comments to explain non-obvious parts of your code.
7. Not Testing the Solution
Some candidates consider their job done once they’ve written the code, without taking the time to test and validate their solution.
Why it’s a problem:
- Untested solutions may contain bugs or logical errors.
- It shows a lack of attention to quality and correctness.
- Missed opportunities to demonstrate debugging skills.
How to avoid it:
- Always test your solution with at least one or two sample inputs.
- Walk through your code step-by-step to verify its logic.
- Consider edge cases and test them if time allows.
- If you find bugs, demonstrate your debugging process to the interviewer.
8. Giving Up Too Easily
When faced with a challenging problem or a roadblock, some candidates become discouraged and give up instead of persevering.
Why it’s a problem:
- It demonstrates a lack of problem-solving resilience.
- Missed opportunities to showcase your ability to work through challenges.
- Interviewers want to see how you handle difficult situations.
How to avoid it:
- Stay calm and maintain a positive attitude when facing difficulties.
- Break down the problem into smaller, more manageable parts.
- Communicate your thought process and ask for hints if you’re truly stuck.
- Practice solving diverse problems to build confidence and resilience.
9. Neglecting to Optimize the Solution
Some candidates stop at the first working solution without considering potential optimizations or more efficient approaches.
Why it’s a problem:
- Inefficient solutions may not scale well with larger inputs.
- Missed opportunities to demonstrate knowledge of algorithms and data structures.
- It can indicate a lack of critical thinking about performance and scalability.
How to avoid it:
- After implementing a working solution, discuss its time and space complexity.
- Consider alternative approaches that might be more efficient.
- Discuss trade-offs between different solutions with the interviewer.
- If time allows, implement optimizations or discuss how you would optimize the solution.
10. Poor Communication Skills
Some candidates struggle to articulate their thoughts clearly or fail to engage effectively with the interviewer.
Why it’s a problem:
- Clear communication is crucial for teamwork in software development.
- Inability to explain your approach can lead to misunderstandings.
- Poor communication can overshadow strong technical skills.
How to avoid it:
- Practice explaining technical concepts to non-technical friends or family.
- Use analogies or diagrams to illustrate complex ideas.
- Actively listen to the interviewer’s questions and feedback.
- Seek clarification if you don’t understand something.
11. Forgetting to Analyze Time and Space Complexity
Many candidates overlook discussing the time and space complexity of their solutions, which is often a crucial part of the interview evaluation.
Why it’s a problem:
- Understanding complexity is essential for writing efficient, scalable code.
- It demonstrates a deeper understanding of algorithms and data structures.
- Interviewers often use this to gauge a candidate’s analytical skills.
How to avoid it:
- Always analyze and discuss the time and space complexity of your solution.
- Consider best-case, average-case, and worst-case scenarios.
- Be prepared to explain how you arrived at your complexity analysis.
- Discuss how the complexity might change with different inputs or constraints.
12. Not Asking for Help When Needed
Some candidates struggle silently instead of asking for help or clarification when they’re stuck.
Why it’s a problem:
- It can lead to wasted time and incomplete solutions.
- Missed opportunities for the interviewer to guide or assess problem-solving skills.
- It doesn’t reflect real-world scenarios where developers often seek help from colleagues.
How to avoid it:
- Don’t hesitate to ask for clarification or hints if you’re truly stuck.
- Explain what you’ve tried and where you’re having difficulty.
- Frame your questions in a way that shows you’ve put thought into the problem.
- Remember that asking thoughtful questions can demonstrate good communication and collaboration skills.
13. Overlooking the Importance of Code Reusability and Modularity
In the rush to solve the problem, some candidates write monolithic, hard-to-maintain code instead of focusing on modularity and reusability.
Why it’s a problem:
- Lack of modularity makes code harder to understand and maintain.
- It misses an opportunity to demonstrate software design skills.
- Monolithic code is often more prone to bugs and harder to test.
How to avoid it:
- Break down your solution into smaller, reusable functions.
- Consider how your code might be extended or modified in the future.
- Discuss potential refactoring opportunities with the interviewer.
- Demonstrate your understanding of clean code principles.
14. Failing to Manage Stress and Anxiety
The pressure of a whiteboard interview can cause some candidates to experience high levels of stress and anxiety, negatively impacting their performance.
Why it’s a problem:
- Stress can impair cognitive function and problem-solving abilities.
- Anxiety may lead to rushed or careless mistakes.
- It can negatively affect communication and overall interview performance.
How to avoid it:
- Practice whiteboard-style problems regularly to build confidence.
- Use relaxation techniques like deep breathing before and during the interview.
- Remember that it’s okay to take a moment to collect your thoughts.
- View the interview as a collaborative problem-solving session rather than a test.
15. Not Leveraging Previous Experience or Knowledge
Some candidates fail to draw upon their past experiences or relevant knowledge when approaching interview problems.
Why it’s a problem:
- Missed opportunities to demonstrate the breadth of your skills and experience.
- Failure to apply lessons learned from past projects or challenges.
- It can make solutions less robust or well-rounded.
How to avoid it:
- Reflect on your past projects and how they might relate to the problem at hand.
- Don’t hesitate to mention relevant experiences or knowledge that inform your approach.
- Consider how industry best practices or design patterns might apply to the problem.
- Use analogies from your experience to explain your thinking or approach.
Conclusion
Whiteboard interviews can be challenging, but by being aware of these common mistakes and actively working to avoid them, you can significantly improve your performance. Remember that the goal of these interviews is not just to solve the problem, but to demonstrate your problem-solving process, communication skills, and technical abilities.
Key takeaways for succeeding in whiteboard interviews include:
- Thoroughly understand the problem before coding
- Plan your approach and communicate your thought process
- Manage your time effectively
- Write clean, readable code
- Consider edge cases and test your solution
- Analyze and discuss time and space complexity
- Stay calm and don’t hesitate to ask for help if needed
- Leverage your experience and knowledge
By focusing on these areas and practicing regularly, you can build the confidence and skills necessary to excel in whiteboard interviews and increase your chances of landing your dream job in the competitive world of software engineering.
Remember, the journey to becoming proficient in whiteboard interviews is a process. Platforms like AlgoCademy offer valuable resources, interactive coding tutorials, and AI-powered assistance to help you prepare effectively. By combining consistent practice with the strategies outlined in this guide, you’ll be well-equipped to tackle even the most challenging whiteboard interviews with confidence and skill.