Common Logic Puzzles and How to Solve Them: Sharpening Your Coding Skills
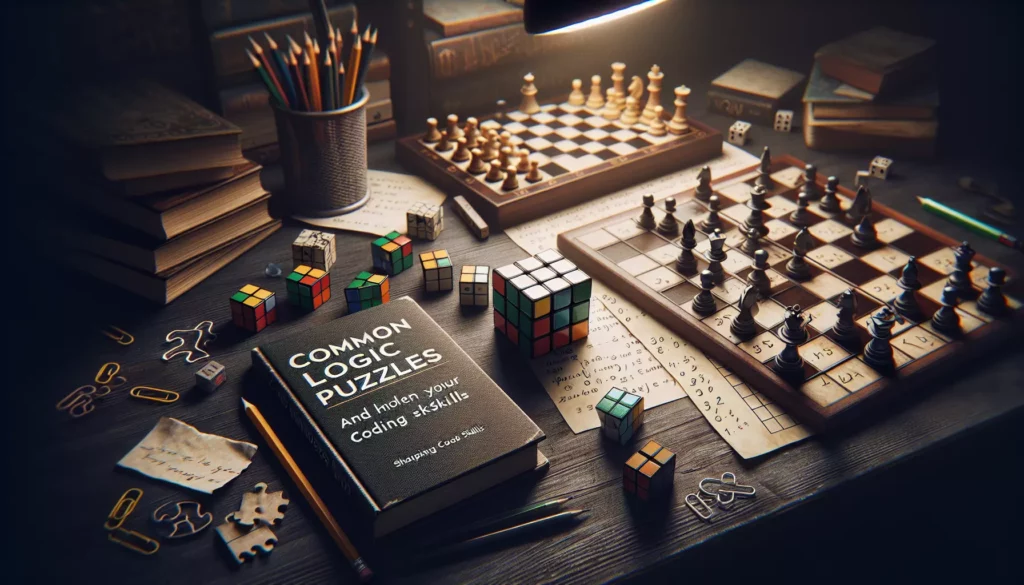
In the world of coding and programming, logical thinking is a fundamental skill that separates great developers from the rest. Whether you’re a beginner just starting your journey or an experienced coder preparing for technical interviews at top tech companies, honing your problem-solving abilities through logic puzzles can significantly boost your performance. In this comprehensive guide, we’ll explore common logic puzzles, their relevance to coding, and strategies to solve them effectively.
Why Logic Puzzles Matter in Coding
Before diving into specific puzzles, it’s crucial to understand why logic puzzles are so valuable for programmers:
- Algorithmic Thinking: Logic puzzles train your mind to break down complex problems into smaller, manageable steps – a key skill in algorithm design.
- Pattern Recognition: Many coding challenges involve recognizing patterns, which is a common element in logic puzzles.
- Efficiency: Solving puzzles efficiently often requires optimizing your approach, mirroring the need for efficient code in programming.
- Interview Preparation: Tech giants like FAANG (Facebook, Amazon, Apple, Netflix, Google) often use logic puzzles in their interview processes.
Common Types of Logic Puzzles
1. The River Crossing Puzzle
The Puzzle: A farmer needs to cross a river with a fox, a chicken, and a sack of grain. The boat can only carry the farmer and one item at a time. If left alone, the fox will eat the chicken, and the chicken will eat the grain. How can the farmer get everything across safely?
Solution Strategy:
- Start by identifying the constraints and rules.
- Visualize the problem as a state machine, where each state represents the items on each side of the river.
- Use a tree or graph to map out possible moves and their consequences.
- Implement a depth-first search or breadth-first search algorithm to find the solution.
Coding Connection: This puzzle relates to graph traversal algorithms and state space search, common in pathfinding problems and AI.
2. The Tower of Hanoi
The Puzzle: You have three rods and a number of disks of different sizes which can slide onto any rod. The puzzle starts with the disks in a neat stack in ascending order of size on one rod, the smallest at the top. The objective is to move the entire stack to another rod, obeying the following rules:
- Only one disk can be moved at a time.
- Each move consists of taking the upper disk from one of the stacks and placing it on top of another stack or on an empty rod.
- No larger disk may be placed on top of a smaller disk.
Solution Strategy:
- Understand the recursive nature of the problem.
- Break down the problem into smaller sub-problems.
- Identify the base case (moving one disk).
- Develop a recursive algorithm to solve for n disks.
Coding Connection: The Tower of Hanoi is a classic example of recursive problem-solving, essential in many algorithms and data structures.
3. The Eight Queens Puzzle
The Puzzle: Place eight chess queens on an 8×8 chessboard so that no two queens threaten each other; thus, a solution requires that no two queens share the same row, column, or diagonal.
Solution Strategy:
- Understand the constraints of queen movements in chess.
- Use a backtracking algorithm to try different configurations.
- Implement checks for row, column, and diagonal conflicts.
- Optimize by recognizing symmetries and eliminating redundant searches.
Coding Connection: This puzzle introduces concepts of backtracking and constraint satisfaction problems, widely used in scheduling and resource allocation algorithms.
4. The Monty Hall Problem
The Puzzle: You’re on a game show, and you’re given the choice of three doors. Behind one door is a car; behind the others, goats. You pick a door, say No. 1, and the host, who knows what’s behind the doors, opens another door, say No. 3, which has a goat. He then says to you, “Do you want to pick door No. 2?” Is it to your advantage to switch your choice?
Solution Strategy:
- Understand the probabilities involved.
- Recognize that the host’s action provides additional information.
- Calculate the probability of winning by staying vs. switching.
- Use simulation to verify the counterintuitive result.
Coding Connection: This problem relates to probability theory and statistical analysis, crucial in machine learning and data science applications.
5. The Josephus Problem
The Puzzle: N people stand in a circle. Starting from a specified point, you count off k people clockwise and eliminate the k-th person. The process is repeated with the remaining people, starting with the person immediately clockwise of the eliminated person, until only one person remains. Find the position of the survivor.
Solution Strategy:
- Understand the circular nature of the problem.
- Recognize the recursive pattern in the eliminations.
- Develop a recursive formula to solve for N people and k count.
- Implement an efficient solution using modular arithmetic.
Coding Connection: The Josephus Problem introduces concepts of circular data structures and modular arithmetic, often used in memory management and scheduling algorithms.
Strategies for Solving Logic Puzzles
While each puzzle type has its specific solving techniques, there are general strategies that can help you approach any logic puzzle:
1. Understand the Problem
Before diving into solutions, make sure you fully grasp the puzzle’s constraints and objectives. Restate the problem in your own words to ensure comprehension.
2. Visualize the Problem
Many logic puzzles benefit from visual representation. Draw diagrams, use tables, or create graphs to map out the problem space.
3. Start with Simple Cases
If the puzzle involves numbers or multiple elements, start by solving for the simplest case. This can often reveal patterns or approaches for more complex scenarios.
4. Look for Patterns
Many puzzles have underlying patterns. Identifying these can lead to efficient solutions or mathematical formulas.
5. Break It Down
Divide the problem into smaller, manageable sub-problems. This divide-and-conquer approach is fundamental in algorithm design.
6. Use Logical Reasoning
Apply deductive and inductive reasoning. Eliminate impossible scenarios and build upon known facts to reach conclusions.
7. Consider Edge Cases
Think about extreme or unusual scenarios. These often reveal flaws in initial assumptions or lead to more robust solutions.
8. Iterate and Optimize
Once you have a working solution, look for ways to improve its efficiency or simplify the approach.
Implementing Solutions in Code
Translating a logic puzzle solution into code is an essential skill for programmers. Here’s a general approach:
1. Choose the Right Data Structures
Select appropriate data structures to represent the puzzle elements. For example, use arrays for linear puzzles, graphs for network problems, or stacks for recursive solutions.
2. Plan Your Algorithm
Outline your solution in pseudocode before writing actual code. This helps clarify your thinking and catch logical errors early.
3. Implement Step by Step
Start with a basic implementation and gradually add complexity. This incremental approach helps in debugging and understanding the solution’s evolution.
4. Test with Examples
Use the examples provided in the puzzle description to test your implementation. Then, create additional test cases to cover edge cases and verify robustness.
5. Optimize for Efficiency
Once you have a working solution, analyze its time and space complexity. Look for opportunities to optimize, such as using more efficient algorithms or data structures.
Example: Implementing the Tower of Hanoi
Here’s a Python implementation of the Tower of Hanoi puzzle:
def tower_of_hanoi(n, source, destination, auxiliary):
if n == 1:
print(f"Move disk 1 from {source} to {destination}")
return
tower_of_hanoi(n-1, source, auxiliary, destination)
print(f"Move disk {n} from {source} to {destination}")
tower_of_hanoi(n-1, auxiliary, destination, source)
# Solve for 3 disks
tower_of_hanoi(3, 'A', 'C', 'B')
This recursive implementation demonstrates the elegance of solving complex problems with simple, well-structured code.
Logic Puzzles in Technical Interviews
Major tech companies, especially FAANG (Facebook, Amazon, Apple, Netflix, Google), often use logic puzzles in their interview processes. Here’s how to prepare:
1. Practice Regularly
Solve logic puzzles frequently to familiarize yourself with common patterns and problem-solving techniques.
2. Verbalize Your Thought Process
During interviews, explain your reasoning out loud. This gives interviewers insight into your problem-solving approach.
3. Ask Clarifying Questions
Don’t hesitate to ask for clarification on puzzle details or constraints. This shows attention to detail and ensures you’re solving the right problem.
4. Consider Multiple Approaches
Even if you see an immediate solution, consider alternative approaches. This demonstrates flexibility and depth of thinking.
5. Analyze Time and Space Complexity
Be prepared to discuss the efficiency of your solution in terms of time and space complexity. This is crucial for optimizing algorithms.
Beyond Puzzles: Applying Logic to Real-World Coding
While logic puzzles are excellent for sharpening your problem-solving skills, it’s essential to bridge the gap between puzzles and real-world coding challenges. Here are some ways to apply puzzle-solving skills to practical programming:
1. Algorithm Design
Use the problem-breaking and pattern-recognition skills from puzzles to design efficient algorithms for complex programming tasks.
2. Debugging
Apply logical reasoning to trace through code and identify bugs. The systematic approach used in puzzle-solving is invaluable in debugging.
3. Optimization
Like optimizing puzzle solutions, look for ways to improve the efficiency of your code, both in terms of time and space complexity.
4. System Design
Use the big-picture thinking required in puzzles to approach system design challenges, considering various components and their interactions.
5. Data Structure Selection
Choose appropriate data structures for your programming tasks, just as you would select the right approach for different types of puzzles.
Conclusion
Logic puzzles are more than just brain teasers; they are powerful tools for developing the critical thinking and problem-solving skills essential in coding. By regularly engaging with puzzles like the River Crossing, Tower of Hanoi, Eight Queens, Monty Hall, and Josephus problems, you’re not just preparing for technical interviews – you’re cultivating a mindset that will serve you well throughout your programming career.
Remember, the goal isn’t just to solve the puzzle but to understand the underlying principles and apply them to a wide range of coding challenges. As you continue your journey in programming, whether you’re using platforms like AlgoCademy or preparing for interviews with top tech companies, let logic puzzles be your companions in honing your algorithmic thinking and coding prowess.
Keep puzzling, keep coding, and watch as your problem-solving skills reach new heights!