Common Interview Prep Errors That Slow Your Progress
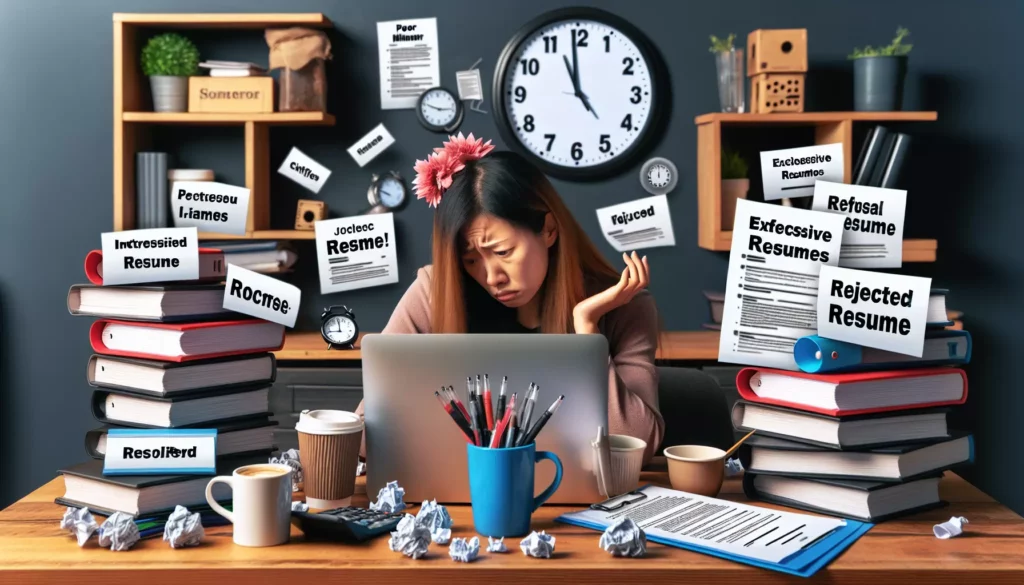
Preparing for technical interviews, especially for positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), can be a daunting task. Many aspiring developers and computer science graduates spend months honing their skills, practicing algorithms, and solving coding problems. However, despite their best efforts, some candidates find themselves struggling to make significant progress or feeling unprepared when the interview day arrives. In this comprehensive guide, we’ll explore common interview preparation errors that can slow down your progress and provide actionable strategies to overcome them.
1. Neglecting Fundamentals
One of the most critical mistakes that candidates make is rushing into complex algorithms and data structures without having a solid grasp of the fundamentals. While it’s tempting to jump straight into advanced topics, neglecting the basics can lead to gaps in your understanding and make it difficult to solve more challenging problems.
Why it’s a problem:
- Weak foundation leads to difficulty in understanding and implementing advanced concepts
- Increases the likelihood of making simple mistakes during interviews
- Reduces confidence when faced with unexpected questions or variations of familiar problems
How to fix it:
- Review core computer science concepts, including time and space complexity analysis
- Practice implementing basic data structures from scratch (e.g., arrays, linked lists, stacks, queues)
- Ensure you have a solid understanding of object-oriented programming principles
- Brush up on essential algorithms like sorting and searching
Remember, even the most complex algorithms are built upon fundamental concepts. By strengthening your foundation, you’ll be better equipped to tackle more advanced problems and adapt to new challenges during interviews.
2. Memorizing Solutions Instead of Understanding Concepts
Another common pitfall is the tendency to memorize solutions to specific problems rather than focusing on understanding the underlying concepts and problem-solving techniques. While memorization might help you solve familiar questions, it fails to prepare you for the diverse range of problems you may encounter in a real interview.
Why it’s a problem:
- Limited ability to solve variations of known problems
- Difficulty in applying learned concepts to new, unfamiliar challenges
- Increased stress when faced with unexpected questions
- Reduced capacity for on-the-spot problem-solving and algorithmic thinking
How to fix it:
- Focus on understanding the core principles behind each algorithm and data structure
- Practice explaining solutions in your own words, as if teaching someone else
- Solve multiple variations of similar problems to reinforce concept application
- Analyze and compare different approaches to solving the same problem
By prioritizing conceptual understanding over rote memorization, you’ll develop the critical thinking skills necessary to tackle a wide range of interview questions and real-world programming challenges.
3. Neglecting Time Management and Problem-Solving Strategies
Many candidates focus solely on coding skills and neglect the equally important aspects of time management and problem-solving strategies. Technical interviews often have time constraints, and your ability to approach problems systematically and efficiently is just as crucial as your coding proficiency.
Why it’s a problem:
- Inability to complete all interview questions within the given time frame
- Increased stress and pressure during the interview
- Difficulty in breaking down complex problems into manageable steps
- Reduced ability to communicate your thought process effectively
How to fix it:
- Practice solving problems under timed conditions to improve your pace
- Develop a structured approach to problem-solving (e.g., understand, plan, implement, test)
- Learn to quickly identify and communicate trade-offs between different solutions
- Practice explaining your thought process out loud while solving problems
By honing your time management and problem-solving strategies, you’ll be better prepared to handle the pressure of technical interviews and demonstrate your ability to think critically under constraints.
4. Overlooking the Importance of Code Quality and Best Practices
While solving the problem correctly is crucial, many candidates underestimate the importance of code quality, readability, and adherence to best practices. Interviewers not only evaluate your ability to solve problems but also assess how well you can write clean, maintainable code.
Why it’s a problem:
- Difficulty in explaining or modifying your code during the interview
- Negative impression on interviewers, even if the solution is correct
- Missed opportunity to demonstrate professional coding skills
- Increased likelihood of introducing bugs or errors in your code
How to fix it:
- Practice writing clean, well-documented code for every problem you solve
- Learn and apply coding style guidelines for your preferred programming language
- Refactor your solutions to improve readability and efficiency
- Familiarize yourself with common design patterns and when to apply them
By prioritizing code quality and best practices in your preparation, you’ll not only improve your chances of success in technical interviews but also develop habits that will serve you well throughout your professional career.
5. Failing to Practice Communication and Collaboration Skills
Technical skills are essential, but many candidates overlook the importance of communication and collaboration in the interview process. Your ability to articulate your thoughts, explain your approach, and work effectively with others is crucial for success in both interviews and real-world software development.
Why it’s a problem:
- Difficulty in explaining your thought process and solution to interviewers
- Missed opportunities to demonstrate teamwork and collaboration skills
- Inability to effectively seek clarification or ask questions during the interview
- Reduced ability to handle behavioral interview questions
How to fix it:
- Practice explaining your solutions out loud, as if presenting to an interviewer
- Participate in mock interviews or coding sessions with peers
- Join online coding communities or local meetups to discuss and share ideas
- Work on open-source projects to gain experience in collaborative development
By developing strong communication and collaboration skills alongside your technical abilities, you’ll be better prepared for the multifaceted nature of technical interviews and increase your chances of success.
6. Ignoring the Importance of System Design and Scalability
As you progress in your career and aim for more senior positions, system design and scalability become increasingly important topics in technical interviews. Many candidates focus solely on algorithmic problems and neglect these crucial aspects of software engineering.
Why it’s a problem:
- Inability to handle system design questions in interviews for senior positions
- Lack of understanding of real-world software architecture challenges
- Missed opportunity to demonstrate broader technical knowledge
- Difficulty in discussing trade-offs and design decisions for large-scale systems
How to fix it:
- Study common system design patterns and architectures
- Practice designing scalable systems for various scenarios (e.g., URL shortener, social media platform)
- Learn about distributed systems, load balancing, and caching strategies
- Familiarize yourself with cloud computing concepts and services
By incorporating system design and scalability into your interview preparation, you’ll be better equipped to handle a wider range of technical questions and demonstrate your ability to think at a higher level of abstraction.
7. Not Leveraging Online Resources and Coding Platforms Effectively
With the abundance of online resources available for interview preparation, failing to utilize them effectively can significantly slow down your progress. Many candidates either overwhelm themselves with too many resources or stick to a single platform, missing out on the benefits of a diverse learning approach.
Why it’s a problem:
- Inefficient use of study time and resources
- Limited exposure to different problem types and difficulty levels
- Missed opportunities to learn from varied explanations and approaches
- Lack of structured learning path and progress tracking
How to fix it:
- Use platforms like AlgoCademy for structured learning and practice
- Participate in coding challenges on platforms like LeetCode, HackerRank, or CodeForces
- Follow curated learning paths designed for interview preparation
- Utilize AI-powered coding assistants for personalized feedback and guidance
By leveraging online resources effectively, you can create a well-rounded and efficient interview preparation strategy that covers all aspects of technical interviews.
8. Neglecting to Review and Learn from Past Mistakes
One of the most valuable aspects of interview preparation is learning from your mistakes and continuously improving. Many candidates solve problems but fail to review their past errors or analyze areas for improvement, leading to repeated mistakes and slower progress.
Why it’s a problem:
- Repeated errors in similar problem types
- Slower improvement in problem-solving skills
- Missed opportunities to identify and address weak areas
- Lack of awareness about personal progress and areas needing focus
How to fix it:
- Keep a log of problems solved, including mistakes made and lessons learned
- Regularly review past solutions and look for ways to improve them
- Analyze patterns in the types of problems or concepts you struggle with
- Seek feedback from peers or mentors on your problem-solving approach
By implementing a systematic review process and learning from your mistakes, you can accelerate your progress and avoid repeating common errors during actual interviews.
9. Underestimating the Importance of Mock Interviews
While solving problems independently is crucial, many candidates overlook the value of mock interviews in their preparation. Simulating the actual interview experience can provide invaluable insights and help you become more comfortable with the interview process.
Why it’s a problem:
- Lack of experience with real-time problem-solving under pressure
- Unfamiliarity with the interview format and expectations
- Missed opportunity to practice communication skills in an interview setting
- Limited feedback on your overall interview performance
How to fix it:
- Participate in mock interviews with peers, mentors, or professional interview prep services
- Record your mock interviews to review and analyze your performance
- Practice with a variety of interviewers to experience different styles and expectations
- Seek detailed feedback on both technical skills and soft skills after each mock interview
By incorporating regular mock interviews into your preparation routine, you’ll build confidence, improve your performance under pressure, and identify areas for improvement before the actual interview.
10. Neglecting to Prepare for Behavioral and Cultural Fit Questions
Technical skills are crucial, but many candidates underestimate the importance of behavioral and cultural fit questions in the interview process. These questions assess your ability to work in a team, handle challenges, and align with the company’s values and culture.
Why it’s a problem:
- Inability to effectively communicate your experiences and soft skills
- Missed opportunity to demonstrate your alignment with the company’s culture
- Difficulty in providing concrete examples of your problem-solving and teamwork abilities
- Reduced chances of success in holistic interview processes
How to fix it:
- Research common behavioral interview questions and prepare thoughtful responses
- Use the STAR (Situation, Task, Action, Result) method to structure your answers
- Reflect on your past experiences and identify relevant examples to share
- Practice articulating your career goals and how they align with the company’s mission
By preparing for behavioral and cultural fit questions alongside your technical preparation, you’ll present a well-rounded profile that appeals to both technical and non-technical interviewers.
Conclusion
Preparing for technical interviews, especially for positions at top tech companies, requires a multifaceted approach that goes beyond simply solving coding problems. By avoiding these common preparation errors and implementing the suggested fixes, you can significantly enhance your interview readiness and increase your chances of success.
Remember that effective interview preparation is a continuous process of learning, practicing, and refining your skills. Utilize resources like AlgoCademy to structure your learning, practice with a variety of problems, and receive personalized guidance. With dedication, a strategic approach, and attention to both technical and soft skills, you’ll be well-equipped to tackle even the most challenging technical interviews and launch your career in the tech industry.
Stay persistent, embrace the learning process, and don’t be discouraged by setbacks. Every problem you solve and every mistake you learn from brings you one step closer to achieving your goals. Good luck with your interview preparation!