Coinbase Technical Interview Prep: A Comprehensive Guide
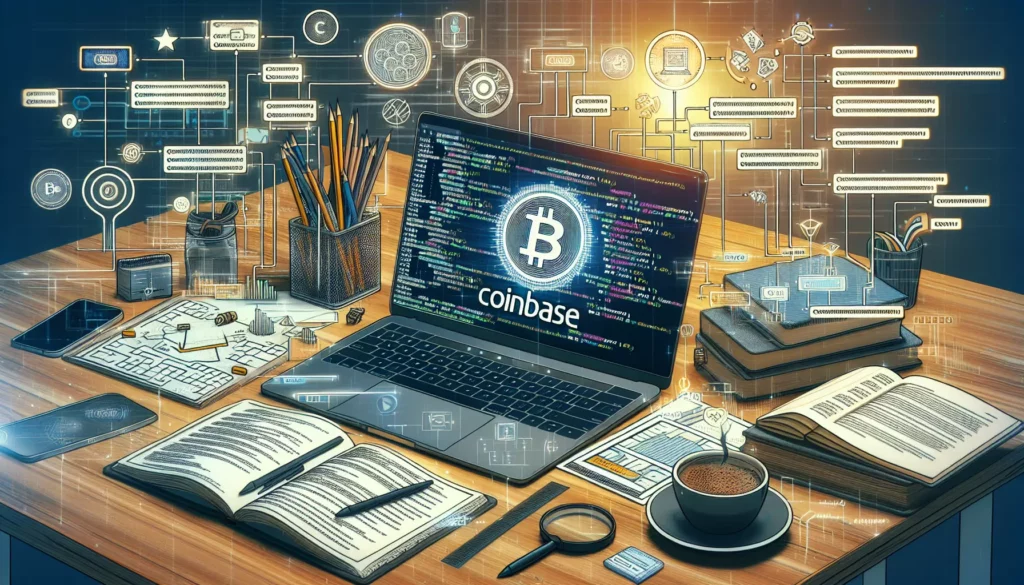
As the cryptocurrency industry continues to grow and evolve, Coinbase remains at the forefront of digital asset exchanges. With its reputation for innovation and security, Coinbase attracts top talent from around the world. If you’re aspiring to join their team, you’ll need to be well-prepared for their rigorous technical interview process. In this comprehensive guide, we’ll explore everything you need to know to ace your Coinbase technical interview.
Table of Contents
- Understanding Coinbase and Its Technology Stack
- The Coinbase Interview Process
- Core Concepts to Master
- Common Coding Challenges and How to Approach Them
- System Design for Cryptocurrency Exchanges
- Essential Blockchain Knowledge
- Security Best Practices in Cryptocurrency
- Behavioral Questions and Company Culture
- Resources for Further Preparation
- Tips and Tricks for Interview Success
1. Understanding Coinbase and Its Technology Stack
Before diving into interview preparation, it’s crucial to understand Coinbase’s role in the cryptocurrency ecosystem and the technologies they use. Coinbase is more than just a cryptocurrency exchange; it’s a platform that provides infrastructure, services, and tools for the digital currency economy.
Key Technologies at Coinbase:
- Programming Languages: Ruby, Python, Go, JavaScript (Node.js)
- Frameworks: Ruby on Rails, React, Redux
- Databases: PostgreSQL, DynamoDB
- Cloud Services: AWS
- Blockchain Technologies: Bitcoin, Ethereum, and other major cryptocurrencies
Familiarizing yourself with these technologies will give you a solid foundation for your interview prep. While you don’t need to be an expert in all of them, having a working knowledge of several will be beneficial.
2. The Coinbase Interview Process
The Coinbase interview process typically consists of several stages:
- Initial Phone Screen: A brief call with a recruiter to discuss your background and the role.
- Technical Phone Interview: A coding interview conducted over the phone or video call, usually lasting 45-60 minutes.
- Take-Home Assignment: Some positions may require a take-home coding project.
- On-Site Interviews: A series of in-person or virtual interviews, including:
- Coding interviews
- System design discussions
- Behavioral interviews
- Domain-specific knowledge assessments
- Final Decision: The hiring team reviews all feedback and makes a decision.
Each stage is designed to assess different aspects of your skills and fit for the role. Let’s explore how to prepare for each component.
3. Core Concepts to Master
To excel in Coinbase’s technical interviews, you should have a strong grasp of the following core computer science and software engineering concepts:
Data Structures:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
Algorithms:
- Sorting (QuickSort, MergeSort, HeapSort)
- Searching (Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic Programming
- Recursion
- Graph Algorithms (Shortest Path, Minimum Spanning Tree)
Time and Space Complexity Analysis:
Understanding Big O notation and being able to analyze the efficiency of your solutions is crucial. Coinbase deals with large-scale systems, so optimizing for performance is key.
Object-Oriented Programming:
Be prepared to discuss OOP concepts such as encapsulation, inheritance, polymorphism, and abstraction. Coinbase’s codebase heavily utilizes OOP principles.
Concurrency and Multithreading:
Given the real-time nature of cryptocurrency trading, understanding concurrent programming is essential. Be familiar with concepts like race conditions, deadlocks, and thread synchronization.
4. Common Coding Challenges and How to Approach Them
During your technical interviews, you’ll likely face coding challenges similar to those found on platforms like LeetCode or HackerRank. Here are some types of problems you should be prepared to solve:
String Manipulation:
Example: Implement a function to find the longest palindromic substring in a given string.
def longest_palindrome(s):
if not s:
return ""
start, max_len = 0, 1
for i in range(len(s)):
# Check for odd-length palindromes
len1 = expand_around_center(s, i, i)
# Check for even-length palindromes
len2 = expand_around_center(s, i, i + 1)
length = max(len1, len2)
if length > max_len:
start = i - (length - 1) // 2
max_len = length
return s[start:start + max_len]
def expand_around_center(s, left, right):
while left >= 0 and right < len(s) and s[left] == s[right]:
left -= 1
right += 1
return right - left - 1
Array Manipulation:
Example: Given an array of integers, find two numbers such that they add up to a specific target number.
def two_sum(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
Tree and Graph Traversal:
Example: Implement a function to find the lowest common ancestor of two nodes in a binary tree.
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def lowest_common_ancestor(root, p, q):
if not root or root == p or root == q:
return root
left = lowest_common_ancestor(root.left, p, q)
right = lowest_common_ancestor(root.right, p, q)
if left and right:
return root
return left if left else right
Dynamic Programming:
Example: Calculate the maximum profit from buying and selling stocks with a cooldown period.
def max_profit_with_cooldown(prices):
if not prices:
return 0
n = len(prices)
buy = [0] * n
sell = [0] * n
cooldown = [0] * n
buy[0] = -prices[0]
for i in range(1, n):
buy[i] = max(buy[i-1], cooldown[i-1] - prices[i])
sell[i] = max(sell[i-1], buy[i-1] + prices[i])
cooldown[i] = max(cooldown[i-1], sell[i-1])
return max(sell[-1], cooldown[-1])
When approaching these challenges, remember to:
- Clarify the problem and ask questions if needed.
- Think out loud and explain your thought process.
- Start with a brute force solution, then optimize.
- Analyze the time and space complexity of your solution.
- Test your code with various inputs, including edge cases.
5. System Design for Cryptocurrency Exchanges
System design questions are a crucial part of Coinbase’s interview process, especially for more senior positions. You should be prepared to design large-scale systems that can handle the complexities of a cryptocurrency exchange. Key areas to focus on include:
Scalability:
Design systems that can handle millions of transactions per second and store vast amounts of data. Consider techniques like sharding, load balancing, and caching.
Consistency and Availability:
Understand the trade-offs between consistency and availability in distributed systems. Coinbase needs to ensure accurate account balances and transaction histories while maintaining high availability.
Real-time Processing:
Design systems that can process and match orders in real-time, update order books, and broadcast price changes to users instantly.
Security:
Incorporate security measures at every level of the system design, including encryption, secure key management, and protection against various types of attacks.
Example Design Question:
“Design a system to handle real-time cryptocurrency price updates and order matching for millions of users.”
Approach:
- Clarify requirements (e.g., number of users, transactions per second, latency requirements)
- Design the high-level architecture (e.g., microservices for order processing, price updates, user management)
- Choose appropriate databases (e.g., PostgreSQL for user data, Redis for caching, Time-series DB for price history)
- Implement a message queue system (e.g., Kafka) for handling real-time updates
- Design the order matching engine for high performance
- Implement a WebSocket server for real-time communication with clients
- Discuss scaling strategies and potential bottlenecks
6. Essential Blockchain Knowledge
While not all positions at Coinbase require deep blockchain expertise, having a solid understanding of blockchain technology and cryptocurrency concepts will give you an edge in your interviews. Key areas to focus on include:
Blockchain Fundamentals:
- Distributed ledger technology
- Consensus mechanisms (Proof of Work, Proof of Stake)
- Block structure and chain formation
- Public key cryptography and digital signatures
Smart Contracts:
Understand the basics of smart contract development, especially on platforms like Ethereum. Familiarity with Solidity (Ethereum’s smart contract language) can be beneficial.
Cryptocurrency Wallets:
Know the different types of wallets (hot, cold, hardware) and how they work to store and manage private keys.
Token Standards:
Be familiar with common token standards like ERC-20 for fungible tokens and ERC-721 for non-fungible tokens (NFTs).
Blockchain Scalability Solutions:
Understand layer 2 scaling solutions like Lightning Network for Bitcoin and rollups for Ethereum.
7. Security Best Practices in Cryptocurrency
Security is paramount in the cryptocurrency industry, and Coinbase places a strong emphasis on it. Be prepared to discuss:
Cryptographic Principles:
- Symmetric vs. asymmetric encryption
- Hash functions and their properties
- Digital signatures and message authentication
Secure Key Management:
Understand best practices for storing and managing cryptographic keys, including hardware security modules (HSMs) and multi-signature schemes.
Common Attack Vectors:
Be familiar with potential attacks on cryptocurrency systems, such as:
- 51% attacks
- Double-spending
- Sybil attacks
- Phishing and social engineering
Secure Coding Practices:
Demonstrate knowledge of writing secure code, including:
- Input validation and sanitization
- Proper error handling and logging
- Secure API design
- Protection against common vulnerabilities (e.g., SQL injection, XSS)
8. Behavioral Questions and Company Culture
Coinbase not only assesses technical skills but also looks for candidates who align with their company culture and values. Be prepared to answer behavioral questions that demonstrate your problem-solving abilities, teamwork, and alignment with Coinbase’s mission.
Example Behavioral Questions:
- Describe a time when you had to make a difficult technical decision. How did you approach it?
- Tell me about a project where you had to learn a new technology quickly. How did you manage the learning process?
- How do you stay updated with the latest developments in cryptocurrency and blockchain technology?
- Describe a situation where you had to explain a complex technical concept to a non-technical stakeholder.
Coinbase’s Cultural Values:
Familiarize yourself with Coinbase’s core values and be prepared to discuss how you embody them:
- Clear Communication
- Positive Energy
- Efficient Execution
- Continuous Learning
9. Resources for Further Preparation
To help you prepare for your Coinbase technical interview, consider using the following resources:
Coding Practice:
- LeetCode: Focus on medium to hard difficulty problems in algorithms and data structures.
- HackerRank: Offers a wide range of programming challenges and contests.
- AlgoExpert: Provides curated coding interview questions with video explanations.
System Design:
- “Designing Data-Intensive Applications” by Martin Kleppmann
- System Design Primer (GitHub repository)
- Grokking the System Design Interview (online course)
Blockchain and Cryptocurrency:
- “Mastering Bitcoin” by Andreas M. Antonopoulos
- “Mastering Ethereum” by Andreas M. Antonopoulos and Gavin Wood
- Coinbase Learn: Educational resources provided by Coinbase
Coinbase-specific Resources:
- Coinbase Engineering Blog
- Coinbase’s GitHub repositories
- Coinbase API documentation
10. Tips and Tricks for Interview Success
As you prepare for your Coinbase technical interview, keep these final tips in mind:
- Practice, practice, practice: Regularly solve coding problems and participate in mock interviews to build confidence.
- Stay current: Keep up with the latest developments in cryptocurrency, blockchain, and financial technology.
- Communicate clearly: Explain your thought process clearly during coding and system design questions.
- Ask clarifying questions: Don’t hesitate to ask for more information or clarification during the interview.
- Show enthusiasm: Demonstrate your passion for cryptocurrency and Coinbase’s mission.
- Be honest: If you don’t know something, admit it and explain how you would go about finding the answer.
- Follow up: After the interview, send a thank-you note and reiterate your interest in the position.
Preparing for a Coinbase technical interview requires a combination of strong computer science fundamentals, blockchain knowledge, and an understanding of large-scale system design. By focusing on these areas and utilizing the resources provided, you’ll be well-equipped to showcase your skills and land your dream job at Coinbase.
Remember, the key to success is not just about having the right answers, but also demonstrating your problem-solving approach, your ability to learn quickly, and your passion for the cryptocurrency space. Good luck with your Coinbase interview preparation!