Coding Without Numbers: An Experiment in Qualitative Programming
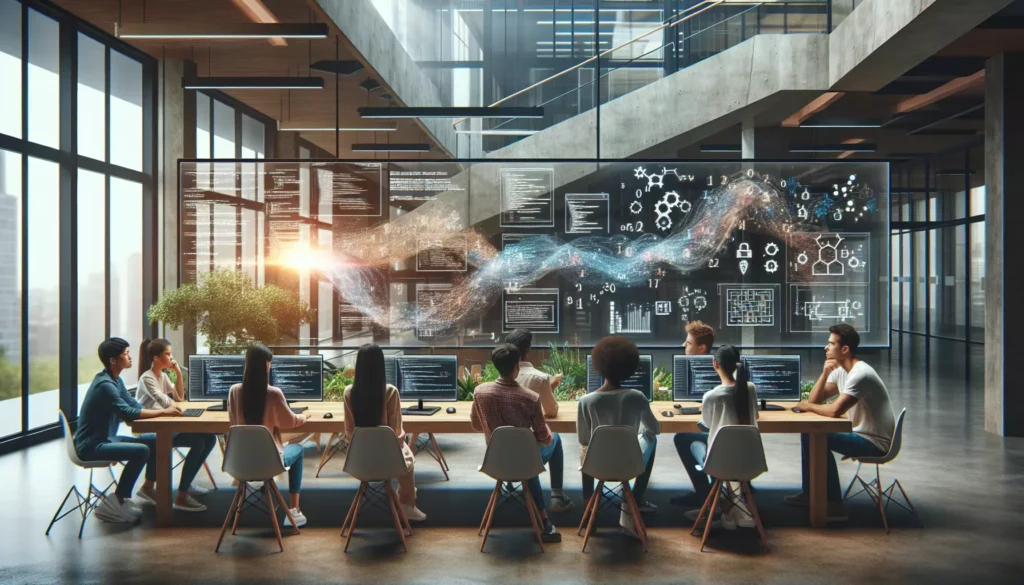
In the vast landscape of programming, numbers often take center stage. From loop counters to array indices, from mathematical calculations to performance metrics, digits seem to be an inseparable part of coding. But what if we challenge this notion? What if we embark on a journey to explore programming from a different perspective – one that doesn’t rely on numerical values? Welcome to the intriguing world of coding without numbers, a concept that pushes the boundaries of traditional programming and opens up new avenues for creative problem-solving.
The Concept of Qualitative Programming
Qualitative programming is an approach that focuses on the descriptive and categorical aspects of data and processes, rather than quantitative measurements. It emphasizes the use of non-numeric representations and logic to solve problems and create algorithms. This paradigm shift encourages programmers to think beyond numerical constructs and explore alternative ways of expressing computations and relationships.
While it might seem counterintuitive at first, qualitative programming can lead to more intuitive and human-readable code in certain scenarios. It can also help in developing a deeper understanding of programming concepts by forcing us to think about problems in a different light.
Why Experiment with Qualitative Programming?
There are several compelling reasons to explore coding without numbers:
- Enhanced Problem-Solving Skills: By removing the crutch of numerical representations, programmers are forced to approach problems from new angles, potentially leading to more creative and efficient solutions.
- Improved Code Readability: Qualitative representations can often be more descriptive and self-explanatory, leading to code that is easier to understand and maintain.
- Better Abstraction: Working with qualitative concepts can help in developing stronger abstraction skills, which are crucial for designing scalable and modular software systems.
- Alignment with Natural Language: Qualitative programming can bridge the gap between natural language and code, making it easier for non-technical stakeholders to understand the logic behind programs.
- Preparation for Emerging Paradigms: As AI and machine learning continue to evolve, understanding qualitative programming concepts can be beneficial for working with more abstract and fuzzy logic systems.
Techniques for Coding Without Numbers
Let’s explore some techniques and approaches that can be used to implement qualitative programming:
1. Enumerations and Named Constants
Instead of using numeric values to represent states or options, we can use enumerations or named constants. This not only makes the code more readable but also adds semantic meaning to the values.
For example, instead of using numbers to represent days of the week:
// Traditional approach
int day = 1; // 1 represents Monday
// Qualitative approach
enum Day {
MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY, SUNDAY
}
Day today = Day.MONDAY;
2. Boolean Logic and Predicates
Boolean logic can be a powerful tool for qualitative programming. By using predicates (functions that return true or false) and combining them with logical operators, we can create complex conditions without relying on numeric comparisons.
// Traditional approach
if (age >= 18 && age < 65) {
// Logic for working-age adults
}
// Qualitative approach
boolean isAdult(Person p) {
return p.hasCompletedSchool() && !p.isRetired();
}
if (isAdult(person)) {
// Logic for working-age adults
}
3. String Manipulation and Pattern Matching
Strings and pattern matching can be used to represent and manipulate qualitative data. Regular expressions, in particular, are a powerful tool for working with text-based information.
// Traditional approach
if (phoneNumber.length() == 10 && phoneNumber.startsWith("555")) {
// Valid phone number logic
}
// Qualitative approach
if (phoneNumber.matches("^555\\d{7}$")) {
// Valid phone number logic
}
4. Object-Oriented Concepts
Object-oriented programming naturally lends itself to qualitative approaches. By using inheritance, polymorphism, and encapsulation, we can create rich, descriptive models of our problem domain without relying heavily on numbers.
// Traditional approach
int vehicleType = 1; // 1 for car, 2 for truck, 3 for motorcycle
// Qualitative approach
abstract class Vehicle {
abstract void drive();
}
class Car extends Vehicle {
void drive() {
System.out.println("Driving a car");
}
}
class Truck extends Vehicle {
void drive() {
System.out.println("Driving a truck");
}
}
5. Functional Programming Concepts
Functional programming emphasizes the use of functions and immutable data, which can be leveraged for qualitative programming. Higher-order functions, in particular, can be used to create expressive and flexible code without relying on numeric operations.
// Traditional approach
int[] numbers = {1, 2, 3, 4, 5};
int sum = 0;
for (int i = 0; i < numbers.length; i++) {
sum += numbers[i];
}
// Qualitative approach (using Java streams)
List<String> words = Arrays.asList("Hello", "World", "Qualitative", "Programming");
String concatenated = words.stream()
.reduce("", (a, b) -> a + " " + b)
.trim();
Real-World Applications of Qualitative Programming
While coding without numbers might seem like an academic exercise, there are several real-world scenarios where qualitative programming approaches can be particularly beneficial:
1. Natural Language Processing (NLP)
NLP systems often deal with textual data and linguistic concepts that are inherently qualitative. Techniques like named entity recognition, sentiment analysis, and text classification rely heavily on qualitative programming approaches.
// Example of sentiment analysis using a qualitative approach
enum Sentiment {
POSITIVE, NEUTRAL, NEGATIVE
}
Sentiment analyzeSentiment(String text) {
if (text.toLowerCase().contains("happy") || text.toLowerCase().contains("great")) {
return Sentiment.POSITIVE;
} else if (text.toLowerCase().contains("sad") || text.toLowerCase().contains("angry")) {
return Sentiment.NEGATIVE;
} else {
return Sentiment.NEUTRAL;
}
}
2. Expert Systems and Rule Engines
Expert systems and rule engines often work with qualitative knowledge representations. These systems use logical rules and inference mechanisms to make decisions, which align well with qualitative programming techniques.
// Simplified example of a rule engine using qualitative concepts
class RuleEngine {
List<Rule> rules = new ArrayList<>();
void addRule(Rule rule) {
rules.add(rule);
}
void evaluateRules(Fact fact) {
for (Rule rule : rules) {
if (rule.condition(fact)) {
rule.action(fact);
}
}
}
}
interface Rule {
boolean condition(Fact fact);
void action(Fact fact);
}
class Fact {
String name;
String value;
}
3. State Machines and Workflow Management
State machines and workflow systems often deal with discrete states and transitions, which can be effectively modeled using qualitative programming techniques. This approach can lead to more intuitive and maintainable code for complex workflows.
// Example of a simple state machine for an order processing system
enum OrderState {
NEW, PROCESSING, SHIPPED, DELIVERED, CANCELLED
}
class Order {
private OrderState state = OrderState.NEW;
void process() {
if (state == OrderState.NEW) {
// Process the order
state = OrderState.PROCESSING;
} else {
throw new IllegalStateException("Order cannot be processed in " + state + " state");
}
}
void ship() {
if (state == OrderState.PROCESSING) {
// Ship the order
state = OrderState.SHIPPED;
} else {
throw new IllegalStateException("Order cannot be shipped in " + state + " state");
}
}
// Other methods for different state transitions
}
4. Configuration Management
Qualitative programming can be particularly useful in configuration management systems, where complex relationships between different configuration options need to be modeled and enforced.
// Example of a configuration management system using qualitative concepts
class ConfigurationManager {
private Map<String, String> config = new HashMap<>();
void setConfig(String key, String value) {
if (isValidConfig(key, value)) {
config.put(key, value);
} else {
throw new IllegalArgumentException("Invalid configuration: " + key + " = " + value);
}
}
boolean isValidConfig(String key, String value) {
switch (key) {
case "logLevel":
return Arrays.asList("DEBUG", "INFO", "WARN", "ERROR").contains(value.toUpperCase());
case "maxConnections":
return value.matches("\\d+") && Integer.parseInt(value) > 0;
// Other configuration validations
default:
return true;
}
}
}
Challenges and Limitations of Qualitative Programming
While qualitative programming offers many benefits, it’s important to acknowledge its challenges and limitations:
1. Performance Considerations
In some cases, qualitative approaches may introduce performance overhead compared to numeric operations. For example, string comparisons are generally slower than integer comparisons. When working on performance-critical systems, careful consideration should be given to the impact of qualitative techniques.
2. Increased Complexity
For certain problems, especially those that are inherently mathematical, qualitative approaches may introduce unnecessary complexity. It’s important to choose the right tool for the job and not force qualitative solutions where quantitative approaches are more appropriate.
3. Learning Curve
Programmers who are accustomed to traditional, number-heavy coding styles may find it challenging to adapt to qualitative programming techniques. There might be a learning curve involved in thinking about problems in a more abstract, qualitative manner.
4. Tool and Library Support
Many existing programming tools and libraries are designed with numerical operations in mind. When adopting qualitative programming techniques, you may find less off-the-shelf support for certain operations, requiring more custom implementations.
5. Precision and Accuracy
In scenarios where precise numerical calculations are required (e.g., financial systems, scientific computing), qualitative approaches may not provide the necessary level of accuracy. It’s crucial to recognize when quantitative precision is non-negotiable.
Best Practices for Qualitative Programming
To make the most of qualitative programming techniques, consider the following best practices:
1. Start with Clear Abstractions
Before diving into implementation, spend time defining clear, meaningful abstractions for your problem domain. This will help you identify the qualitative concepts and relationships that are central to your solution.
2. Leverage Type Systems
Use your programming language’s type system to create rich, expressive types that capture the qualitative aspects of your domain. This can help catch errors at compile-time and make your code more self-documenting.
// Example of using custom types for qualitative programming
class Temperature {
private final double value;
private final TemperatureUnit unit;
// Constructor, getters, etc.
enum TemperatureUnit {
CELSIUS, FAHRENHEIT, KELVIN
}
boolean isFreezing() {
switch (unit) {
case CELSIUS:
return value <= 0;
case FAHRENHEIT:
return value <= 32;
case KELVIN:
return value <= 273.15;
default:
throw new IllegalStateException("Unknown temperature unit");
}
}
}
3. Write Descriptive Tests
Qualitative programming often leads to more descriptive and readable code. Extend this principle to your tests, writing them in a way that clearly describes the expected behavior using qualitative terms.
// Example of a descriptive test for qualitative programming
@Test
void testOrderProcessing() {
Order order = new Order();
assertTrue(order.isNew(), "New order should be in NEW state");
order.process();
assertTrue(order.isProcessing(), "Processed order should be in PROCESSING state");
order.ship();
assertTrue(order.isShipped(), "Shipped order should be in SHIPPED state");
assertThrows(IllegalStateException.class, order::process,
"Cannot process an order that has already been shipped");
}
4. Balance Qualitative and Quantitative Approaches
While exploring qualitative programming, remember that it’s not about completely eliminating numbers, but rather about finding the right balance. Use qualitative approaches where they add clarity and maintainability, and quantitative approaches where precision and performance are critical.
5. Document Your Approach
When using qualitative programming techniques, especially in a team setting, it’s important to document your approach and the reasoning behind it. This will help other developers understand and maintain the code, and can also serve as a valuable learning resource.
Conclusion
Coding without numbers, or qualitative programming, is more than just an intellectual exercise. It’s a powerful paradigm that can lead to more expressive, maintainable, and intuitive code. By shifting our focus from numerical representations to qualitative concepts, we open up new ways of thinking about and solving problems in software development.
While it’s not a silver bullet and may not be appropriate for every situation, incorporating qualitative programming techniques into your toolkit can significantly enhance your problem-solving skills and lead to more robust and flexible software designs. As you continue your journey in programming, challenge yourself to look beyond the numbers and explore the rich world of qualitative concepts that underlie the problems you’re solving.
Remember, the goal is not to completely eliminate numbers from your code, but to find the right balance between qualitative and quantitative approaches. By doing so, you’ll be better equipped to tackle a wide range of programming challenges and create software that is not just functional, but also intuitive and aligned with the way humans naturally think about problems.
So, the next time you sit down to code, ask yourself: “Can I express this without using numbers?” You might be surprised by the creative and elegant solutions that emerge when you embrace the principles of qualitative programming.