Coding Without Nouns: A Challenge in Verb-Centric Programming
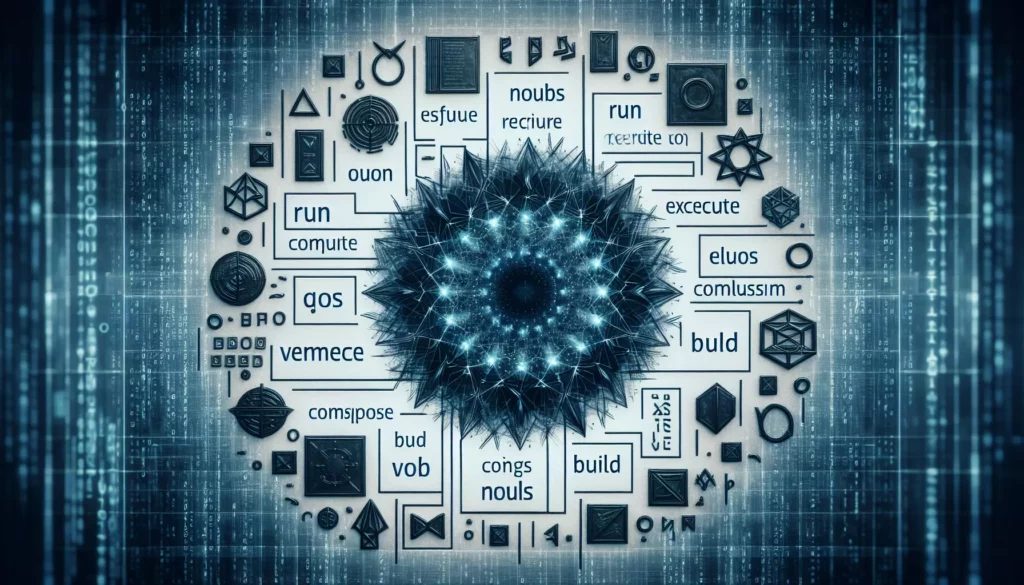
In the vast landscape of programming paradigms and coding challenges, a unique and thought-provoking concept has emerged: coding without nouns. This intriguing approach to software development pushes programmers to think differently about how they structure their code, focusing primarily on actions and behaviors rather than objects and entities. In this comprehensive exploration, we’ll dive deep into the world of verb-centric programming, examining its principles, benefits, challenges, and potential applications in modern software development.
Understanding Verb-Centric Programming
Verb-centric programming, also known as action-oriented programming, is an unconventional approach that emphasizes the use of verbs (actions) over nouns (objects) in code structure and design. This paradigm shift challenges the traditional object-oriented programming (OOP) model, which has been a cornerstone of software development for decades.
In traditional OOP, we often start by identifying the objects or entities in our problem domain and then define their properties and behaviors. For example, in a banking application, we might have classes like Account
, Customer
, and Transaction
. These nouns form the backbone of our code structure.
In contrast, verb-centric programming flips this approach on its head. Instead of focusing on objects, we prioritize actions and behaviors. We ask ourselves, “What does the program do?” rather than “What objects does the program manipulate?” This shift in perspective can lead to some interesting and potentially more efficient code structures.
The Challenge of Coding Without Nouns
Coding without nouns presents a unique set of challenges for programmers accustomed to object-oriented thinking. Here are some of the main hurdles:
- Rethinking Data Representation: Without nouns to encapsulate data, we need to find alternative ways to represent and manage information.
- Handling State: Object-oriented programming makes it easy to maintain state within objects. In a verb-centric approach, managing state becomes more challenging.
- Organizing Code: Traditional code organization often revolves around classes and objects. Without these, we need new strategies for structuring our codebase.
- Maintaining Clarity: Nouns often provide clear abstractions that make code more readable. Verb-centric code might require extra effort to maintain clarity.
- Adapting Design Patterns: Many established design patterns are built around object-oriented principles. These need to be reimagined in a verb-centric context.
Advantages of Verb-Centric Programming
Despite the challenges, coding without nouns offers several potential benefits:
- Focus on Behavior: By emphasizing actions, we naturally focus more on what the program does, potentially leading to more efficient and purpose-driven code.
- Reduced Complexity: Eliminating the need for complex class hierarchies and inheritance structures can simplify code in some scenarios.
- Improved Testability: With a focus on actions, it can be easier to write and maintain unit tests that cover specific behaviors.
- Enhanced Modularity: Verb-centric code often leads to smaller, more focused functions, which can improve modularity and reusability.
- Novel Problem-Solving Approaches: This paradigm encourages thinking about problems in new ways, potentially leading to innovative solutions.
Practical Examples of Verb-Centric Programming
Let’s explore some practical examples to illustrate how verb-centric programming might look in practice. We’ll use JavaScript for these examples, as its functional programming capabilities make it well-suited for this approach.
Example 1: A Simple To-Do List
In a traditional object-oriented approach, we might have a Task
class and a ToDoList
class. In a verb-centric approach, we focus on the actions:
// Verb-centric To-Do List
// Actions (verbs)
const add = (list, task) => [...list, task];
const remove = (list, index) => list.filter((_, i) => i !== index);
const complete = (list, index) => list.map((task, i) =>
i === index ? { ...task, completed: true } : task
);
const display = (list) => list.forEach((task, index) =>
console.log(`${index + 1}. [${task.completed ? 'X' : ' '}] ${task.text}`)
);
// Usage
let myList = [];
myList = add(myList, { text: "Buy groceries", completed: false });
myList = add(myList, { text: "Write code", completed: false });
myList = complete(myList, 0);
display(myList);
myList = remove(myList, 1);
display(myList);
In this example, we define actions like add
, remove
, complete
, and display
as pure functions that operate on a list of tasks. The state (the list itself) is managed externally and passed to these functions as needed.
Example 2: A Simple Banking System
Now, let’s consider a more complex example: a basic banking system. In an object-oriented approach, we might have Account
and Transaction
classes. Here’s how we could approach this in a verb-centric way:
// Verb-centric Banking System
// Actions (verbs)
const createAccount = (id, balance = 0) => ({ id, balance });
const deposit = (account, amount) => ({
...account,
balance: account.balance + amount
});
const withdraw = (account, amount) => {
if (account.balance < amount) {
throw new Error("Insufficient funds");
}
return {
...account,
balance: account.balance - amount
};
};
const transfer = (fromAccount, toAccount, amount) => {
const updatedFromAccount = withdraw(fromAccount, amount);
const updatedToAccount = deposit(toAccount, amount);
return [updatedFromAccount, updatedToAccount];
};
const getBalance = (account) => account.balance;
// Usage
let account1 = createAccount("A001", 1000);
let account2 = createAccount("A002", 500);
console.log("Initial balances:");
console.log(`Account 1: ${getBalance(account1)}`);
console.log(`Account 2: ${getBalance(account2)}`);
account1 = deposit(account1, 200);
console.log("\nAfter depositing 200 to Account 1:");
console.log(`Account 1: ${getBalance(account1)}`);
try {
account2 = withdraw(account2, 700);
} catch (error) {
console.log("\nWithdrawal failed:", error.message);
}
[account1, account2] = transfer(account1, account2, 300);
console.log("\nAfter transferring 300 from Account 1 to Account 2:");
console.log(`Account 1: ${getBalance(account1)}`);
console.log(`Account 2: ${getBalance(account2)}`);
In this verb-centric approach, we define actions like createAccount
, deposit
, withdraw
, transfer
, and getBalance
. These functions operate on simple account objects, focusing on the actions performed rather than the objects themselves.
Challenges and Considerations
While the examples above demonstrate the potential of verb-centric programming, they also highlight some of the challenges:
- State Management: In larger applications, managing state without the encapsulation provided by objects can become complex. Techniques from functional programming, such as using immutable data structures and pure functions, can help mitigate this.
- Data Organization: Without classes to group related data and behavior, we need to carefully consider how to structure our data. In some cases, simple objects (as seen in the banking example) can serve as lightweight data containers.
- Performance Considerations: Creating new objects for every operation (as in the
deposit
andwithdraw
functions) can have performance implications in large-scale applications. Immutable data structure libraries can help optimize this approach. - Familiarity and Learning Curve: For developers accustomed to object-oriented programming, adapting to a verb-centric mindset can be challenging and may require significant practice.
Verb-Centric Programming in the Real World
While pure verb-centric programming as described in this article is relatively rare in production environments, many of its principles are finding their way into modern software development practices:
1. Functional Programming
Many concepts from verb-centric programming align closely with functional programming paradigms. Languages like Haskell, Clojure, and F# emphasize functions over objects and promote immutability and pure functions.
2. React and Redux
In the frontend world, libraries like React (especially when combined with Redux) encourage a more action-oriented approach to state management. Redux, in particular, uses actions (verbs) to describe all changes to the application state.
3. Microservices Architecture
The trend towards microservices in backend development often leads to more action-oriented service designs, where each microservice is responsible for a specific set of actions rather than representing a particular object or entity.
4. Event-Driven Programming
Event-driven systems, which are becoming increasingly popular in distributed systems and real-time applications, naturally lend themselves to a more verb-centric approach, focusing on actions and reactions rather than static object representations.
Learning from Verb-Centric Programming
Even if we don’t adopt a purely verb-centric approach, there are valuable lessons we can learn from this paradigm:
- Focus on Behavior: Regularly ask yourself, “What does this code do?” rather than just “What objects does it manipulate?”
- Embrace Functional Concepts: Incorporate functional programming concepts like pure functions and immutability into your code where appropriate.
- Rethink Object Design: When designing objects, focus more on their behaviors and less on their properties. This can lead to more action-oriented and potentially more reusable code.
- Modular Thinking: Break down complex operations into smaller, focused functions. This aligns with the verb-centric approach and often results in more modular and testable code.
- Consider Alternative Paradigms: Regularly expose yourself to different programming paradigms. Even if you don’t fully adopt them, they can provide new perspectives and problem-solving approaches.
Implementing Verb-Centric Principles in AlgoCademy
As we consider the principles of verb-centric programming in the context of AlgoCademy, a platform focused on coding education and programming skills development, several interesting applications and teaching opportunities emerge:
1. Problem-Solving Exercises
AlgoCademy could introduce a series of coding challenges that specifically require students to solve problems using a verb-centric approach. This would encourage learners to think about problems in terms of actions and behaviors, potentially leading to more efficient and focused solutions.
2. Algorithm Design
When teaching algorithm design, emphasizing the actions and transformations involved in an algorithm, rather than focusing solely on data structures, could provide a fresh perspective. This approach might help students better understand the core logic of algorithms.
3. Functional Programming Modules
Introducing modules on functional programming concepts, which align closely with verb-centric thinking, could broaden students’ programming paradigm knowledge. This could include topics like pure functions, immutability, and function composition.
4. Refactoring Exercises
Create exercises where students are given object-oriented code and asked to refactor it into a more verb-centric style. This practice can help develop skills in code organization and alternative problem-solving approaches.
5. State Management Tutorials
Develop tutorials on state management in verb-centric programming, addressing one of its key challenges. This could cover techniques from functional programming and modern frontend frameworks like Redux.
6. Comparative Programming Paradigms
Offer lessons that compare different programming paradigms, including object-oriented, functional, and verb-centric approaches. This can help students understand the strengths and weaknesses of each approach and when to apply them.
7. Real-World Application Examples
Provide examples of how verb-centric principles are applied in real-world scenarios, such as in event-driven systems, microservices architectures, or functional reactive programming in frontend development.
Conclusion
Coding without nouns, or verb-centric programming, presents an intriguing challenge to traditional programming paradigms. While it may not be suitable as a complete replacement for object-oriented or other established approaches, it offers valuable insights and alternative problem-solving strategies.
By focusing on actions and behaviors rather than objects and entities, verb-centric programming encourages developers to think differently about code structure, state management, and program design. This can lead to more modular, testable, and potentially more efficient code in certain scenarios.
As with any programming paradigm, the key is to understand its principles, recognize its strengths and limitations, and apply it judiciously. Verb-centric programming serves as a reminder that there’s always room for new perspectives in the ever-evolving world of software development.
For platforms like AlgoCademy, incorporating elements of verb-centric programming into their curriculum can provide students with a broader understanding of programming paradigms and problem-solving approaches. This diversity of knowledge can ultimately make them more versatile and effective programmers, better prepared for the challenges of modern software development.
Whether you fully embrace verb-centric programming or simply incorporate some of its principles into your coding practice, the exercise of thinking about programming from this unique perspective can be invaluable. It challenges our assumptions, broadens our problem-solving toolkit, and reminds us that in the world of programming, there’s always more than one way to approach a problem.