Coding Without Loops: A Week-Long Challenge in Recursive Thinking
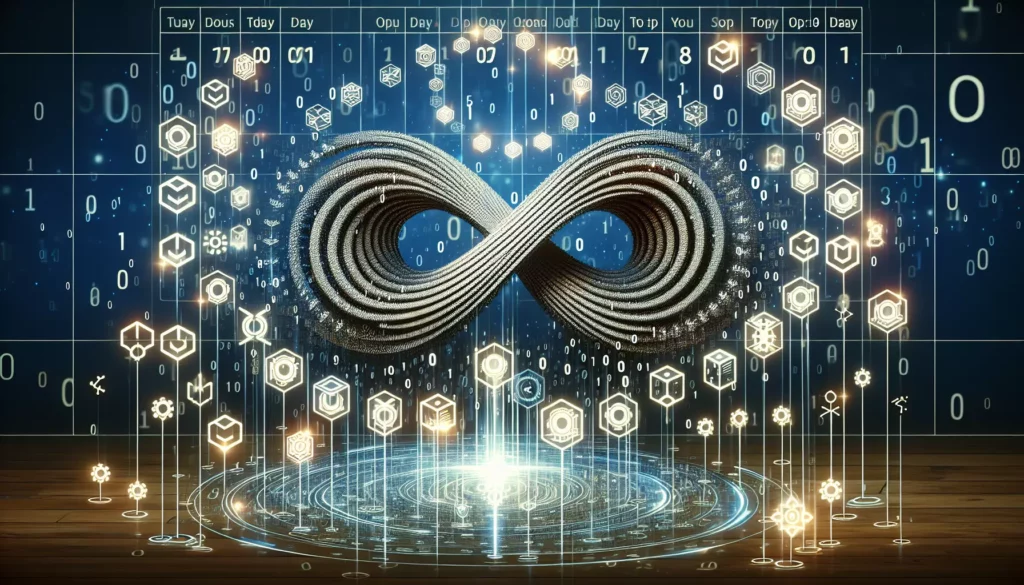
Welcome, coding enthusiasts! Today, we’re embarking on an exciting journey that will challenge your programming paradigms and push your problem-solving skills to new heights. For the next week, we’re going to explore the fascinating world of recursive thinking by taking on a unique challenge: coding without loops.
This challenge is not just about avoiding for
and while
loops; it’s about embracing a different way of approaching problems. Recursion, often seen as a complex and mysterious concept, can be an incredibly powerful tool when understood and applied correctly. By the end of this week-long challenge, you’ll have a deeper appreciation for recursive solutions and a new set of skills to add to your programming toolkit.
Why Take on This Challenge?
Before we dive into the daily challenges, let’s discuss why this exercise is valuable for programmers of all levels:
- Enhances Problem-Solving Skills: Recursive thinking often requires breaking down problems into smaller, manageable pieces, which is a crucial skill in programming.
- Improves Code Readability: Well-written recursive solutions can be more elegant and easier to understand than their iterative counterparts.
- Prepares for Technical Interviews: Many technical interviews, especially for top tech companies, include questions that are best solved using recursion.
- Expands Your Coding Toolkit: By mastering recursion, you add a powerful technique to your programming arsenal.
- Challenges Conventional Thinking: This exercise pushes you out of your comfort zone and encourages creative problem-solving.
The Rules of the Challenge
To make the most of this week-long journey, we’ll adhere to the following rules:
- No use of explicit loops (
for
,while
,do-while
, etc.). - Recursion must be used to solve each problem.
- Built-in methods that use loops internally (like
map
,reduce
,filter
) are also off-limits. - Focus on writing clean, readable, and efficient recursive solutions.
- Test your solutions with various inputs to ensure they work correctly.
Day 1: Summing an Array
Let’s start with a simple problem to warm up our recursive thinking muscles. Your task is to write a function that calculates the sum of all elements in an array without using any loops.
Problem Statement:
Write a recursive function sumArray(arr)
that takes an array of numbers as input and returns the sum of all elements in the array.
Example:
Input: [1, 2, 3, 4, 5]
Output: 15
Input: [-1, 0, 1]
Output: 0
Input: [10]
Output: 10
Solution:
Here’s a recursive solution to this problem:
function sumArray(arr) {
// Base case: if the array is empty, return 0
if (arr.length === 0) {
return 0;
}
// Recursive case: sum the first element with the sum of the rest of the array
return arr[0] + sumArray(arr.slice(1));
}
Explanation:
This recursive solution works as follows:
- We define a base case: if the array is empty, we return 0 (the sum of an empty array is 0).
- For the recursive case, we add the first element of the array (
arr[0]
) to the sum of the rest of the array. - We calculate the sum of the rest of the array by making a recursive call to
sumArray
with a new array that excludes the first element (arr.slice(1)
). - This process continues until we reach the base case (an empty array).
This solution elegantly breaks down the problem into smaller subproblems, solving it recursively without any loops.
Day 2: Reversing a String
For our second day, let’s tackle a classic programming problem: reversing a string. This task is often solved using loops, but we’ll approach it recursively to flex our newfound skills.
Problem Statement:
Write a recursive function reverseString(str)
that takes a string as input and returns the reversed string.
Example:
Input: "hello"
Output: "olleh"
Input: "recursion"
Output: "noisrucer"
Input: "a"
Output: "a"
Solution:
Here’s a recursive solution to reverse a string:
function reverseString(str) {
// Base case: if the string is empty or has only one character, return it
if (str.length <= 1) {
return str;
}
// Recursive case: reverse the substring excluding the first character,
// then append the first character at the end
return reverseString(str.slice(1)) + str[0];
}
Explanation:
This recursive solution works as follows:
- We define a base case: if the string is empty or has only one character, we return it as is (reversing an empty string or a single character doesn’t change anything).
- For the recursive case, we do two things:
- We make a recursive call to
reverseString
with a substring that excludes the first character (str.slice(1)
). - We append the first character of the original string (
str[0]
) to the end of the reversed substring.
- We make a recursive call to
- This process continues, effectively moving each character from the front to the back until we reach the base case.
This solution demonstrates how recursion can be used to break down the problem of reversing a string into smaller, more manageable steps.
Day 3: Fibonacci Sequence
As we reach the midpoint of our challenge, let’s tackle a problem that’s often used to introduce recursion: generating the Fibonacci sequence. While this problem can be solved more efficiently using dynamic programming or iteration, implementing it recursively will help solidify our understanding of recursive thinking.
Problem Statement:
Write a recursive function fibonacci(n)
that returns the nth number in the Fibonacci sequence. Recall that the Fibonacci sequence is defined as follows:
- F(0) = 0
- F(1) = 1
- F(n) = F(n-1) + F(n-2) for n > 1
Example:
Input: 0
Output: 0
Input: 1
Output: 1
Input: 6
Output: 8 (The sequence is 0, 1, 1, 2, 3, 5, 8)
Solution:
Here’s a recursive solution to generate the nth Fibonacci number:
function fibonacci(n) {
// Base cases: F(0) = 0, F(1) = 1
if (n === 0) return 0;
if (n === 1) return 1;
// Recursive case: F(n) = F(n-1) + F(n-2)
return fibonacci(n - 1) + fibonacci(n - 2);
}
Explanation:
This recursive solution follows the mathematical definition of the Fibonacci sequence:
- We define two base cases:
- If n is 0, we return 0
- If n is 1, we return 1
- For the recursive case (n > 1), we return the sum of the two preceding Fibonacci numbers:
- We make a recursive call to
fibonacci(n - 1)
to get F(n-1) - We make another recursive call to
fibonacci(n - 2)
to get F(n-2) - We sum these two values to get F(n)
- We make a recursive call to
While this solution is elegant and closely mirrors the mathematical definition, it’s worth noting that it’s not the most efficient implementation for large values of n due to the repeated calculations. In a real-world scenario, you might want to use memoization or dynamic programming to optimize this solution.
Day 4: Binary Search
As we enter the second half of our challenge, let’s tackle a more complex problem: implementing binary search recursively. Binary search is an efficient algorithm for finding an item from a sorted list of items. It works by repeatedly dividing in half the portion of the list that could contain the item, until you’ve narrowed down the possible locations to just one.
Problem Statement:
Write a recursive function binarySearch(arr, target, low, high)
that performs a binary search on a sorted array arr
to find the index of target
. If the target is not found, return -1. The low
and high
parameters represent the current search range.
Example:
Input: arr = [1, 3, 5, 7, 9, 11, 13, 15], target = 7
Output: 3
Input: arr = [1, 3, 5, 7, 9, 11, 13, 15], target = 6
Output: -1
Input: arr = [1, 3, 5, 7, 9, 11, 13, 15], target = 15
Output: 7
Solution:
Here’s a recursive implementation of binary search:
function binarySearch(arr, target, low = 0, high = arr.length - 1) {
// Base case: if low > high, the target is not in the array
if (low > high) return -1;
// Calculate the middle index
const mid = Math.floor((low + high) / 2);
// If the middle element is the target, return its index
if (arr[mid] === target) return mid;
// If the target is less than the middle element, search the left half
if (target < arr[mid]) return binarySearch(arr, target, low, mid - 1);
// If the target is greater than the middle element, search the right half
return binarySearch(arr, target, mid + 1, high);
}
Explanation:
This recursive binary search algorithm works as follows:
- We define a base case: if
low
becomes greater thanhigh
, it means we’ve searched the entire range without finding the target, so we return -1. - We calculate the middle index
mid
of the current range. - If the middle element is the target, we’ve found it, so we return its index.
- If the target is less than the middle element, we recursively search the left half of the range by calling
binarySearch
withhigh
set tomid - 1
. - If the target is greater than the middle element, we recursively search the right half of the range by calling
binarySearch
withlow
set tomid + 1
.
This recursive implementation elegantly expresses the divide-and-conquer nature of the binary search algorithm without using any loops.
Day 5: Tree Traversal
As we approach the end of our week-long challenge, let’s explore a problem where recursion truly shines: tree traversal. Trees are hierarchical data structures that are naturally suited for recursive algorithms. Today, we’ll implement an in-order traversal of a binary tree.
Problem Statement:
Write a recursive function inorderTraversal(root)
that performs an in-order traversal of a binary tree and returns an array of node values in the order they were visited. In an in-order traversal, we visit the left subtree, then the root, and finally the right subtree.
For this problem, assume we have a TreeNode
class defined as follows:
class TreeNode {
constructor(val = 0, left = null, right = null) {
this.val = val;
this.left = left;
this.right = right;
}
}
Example:
Input:
1
/ \
2 3
/ \
4 5
Output: [4, 2, 5, 1, 3]
Solution:
Here’s a recursive implementation of in-order traversal:
function inorderTraversal(root) {
const result = [];
function traverse(node) {
if (node === null) return;
// Traverse left subtree
traverse(node.left);
// Visit the root
result.push(node.val);
// Traverse right subtree
traverse(node.right);
}
traverse(root);
return result;
}
Explanation:
This recursive in-order traversal works as follows:
- We define an inner helper function
traverse
that does the actual traversal. - In
traverse
:- We first check if the current node is null. If it is, we return (this is our base case).
- We recursively traverse the left subtree.
- We visit the current node by pushing its value to the
result
array. - We recursively traverse the right subtree.
- We call
traverse
with the root node to start the traversal. - Finally, we return the
result
array containing the traversal order.
This solution demonstrates how recursion can elegantly handle tree-like structures, visiting each node exactly once in the correct order without any explicit loops.
Day 6: Quick Sort
As we near the end of our challenge, let’s tackle one of the most famous recursive algorithms: Quick Sort. This efficient, comparison-based sorting algorithm uses a divide-and-conquer strategy and is an excellent example of how recursion can be used to solve complex problems.
Problem Statement:
Implement the Quick Sort algorithm recursively. Write a function quickSort(arr)
that takes an unsorted array as input and returns a new sorted array. Remember, you cannot use any built-in sorting methods or loops.
Example:
Input: [64, 34, 25, 12, 22, 11, 90]
Output: [11, 12, 22, 25, 34, 64, 90]
Input: [5, 2, 9, 1, 7, 6, 3]
Output: [1, 2, 3, 5, 6, 7, 9]
Solution:
Here’s a recursive implementation of Quick Sort:
function quickSort(arr) {
// Base case: arrays with 0 or 1 element are already sorted
if (arr.length <= 1) return arr;
// Choose the first element as the pivot
const pivot = arr[0];
// Partition the array
const left = arr.slice(1).filter(x => x < pivot);
const right = arr.slice(1).filter(x => x >= pivot);
// Recursively sort the partitions and combine the result
return [...quickSort(left), pivot, ...quickSort(right)];
}
Explanation:
This recursive Quick Sort implementation works as follows:
- We define our base case: if the array has 0 or 1 elements, it’s already sorted, so we return it as is.
- We choose the first element of the array as our pivot. In a more optimized version, we might choose a random element or the median of the first, middle, and last elements.
- We partition the array into two parts:
left
: all elements less than the pivotright
: all elements greater than or equal to the pivot
- We recursively apply quickSort to both
left
andright
partitions. - Finally, we combine the sorted left partition, the pivot, and the sorted right partition to get the final sorted array.
Note that while we’re using filter
here, which internally uses a loop, we’re doing so to keep the example concise. In a strict interpretation of our “no loops” rule, we would implement the partitioning step recursively as well.
Day 7: Solving the Tower of Hanoi
For the final day of our challenge, let’s tackle a classic problem that’s perfectly suited for recursive thinking: the Tower of Hanoi. This mathematical game consists of three rods and a number of disks of different sizes which can slide onto any rod. The puzzle starts with the disks in a neat stack in ascending order of size on one rod, the smallest at the top. The objective of the puzzle is to move the entire stack to another rod, obeying the following simple rules:
- Only one disk can be moved at a time.
- Each move consists of taking the upper disk from one of the stacks and placing it on top of another stack or on an empty rod.
- No larger disk may be placed on top of a smaller disk.
Problem Statement:
Write a recursive function towerOfHanoi(n, source, auxiliary, target)
that solves the Tower of Hanoi puzzle. The function should print out the steps to move n disks from the source rod to the target rod, using the auxiliary rod as needed.
Example:
Input: n = 3, source = 'A', auxiliary = 'B', target = 'C'
Output:
Move disk 1 from rod A to rod C
Move disk 2 from rod A to rod B
Move disk 1 from rod C to rod B
Move disk 3 from rod A to rod C
Move disk 1 from rod B to rod A
Move disk 2 from rod B to rod C
Move disk 1 from rod A to rod C
Solution:
Here’s a recursive implementation of the Tower of Hanoi solution:
function towerOfHanoi(n, source, auxiliary, target) {
// Base case: if there's only one disk, move it directly from source to target
if (n === 1) {
console.log(`Move disk 1 from rod ${source} to rod ${target}`);
return;
}
// Move n-1 disks from source to auxiliary, using target as the auxiliary rod
towerOfHanoi(n - 1, source, target, auxiliary);
// Move the nth disk from source to target
console.log(`Move disk ${n} from rod ${source} to rod ${target}`);
// Move the n-1 disks from auxiliary to target, using source as the auxiliary rod
towerOfHanoi(n - 1, auxiliary, source, target);
}
Explanation:
This recursive solution to the Tower of Hanoi puzzle works as follows:
- We define our base case: if there’s only one disk (n = 1), we simply move it from the source rod to the target rod.
- For the recursive case (n > 1), we break down the problem into three steps:
- Move n-1 disks from the source rod to the auxiliary rod, using the target rod as temporary storage.
- Move the nth (largest) disk from the source rod to the target rod.
- Move the n-1 disks from the auxiliary rod to the target rod, using the source rod as temporary storage.
- Each of these steps is either a single move (for the largest disk) or a recursive call to
towerOfHanoi
with n-1 disks.
This elegant recursive solution demonstrates how complex problems can be broken down into simpler sub-problems, which is the essence of recursive thinking.
Conclusion
Congratulations on completing this week-long challenge of coding without loops! Through these seven days, we’ve explored a variety of problems and solved them using recursive thinking. From simple array operations to complex algorithms like Quick Sort and the Tower of Hanoi, we’ve seen how recursion can be a powerful tool in a programmer’s toolkit.
Here are some key takeaways from this challenge:
- Problem Decomposition: Recursion excels at breaking down complex problems into smaller, more manageable sub-problems.
- Elegance and Readability: Many recursive solutions are more concise and easier to understand than their iterative counterparts.
- Natural Fit for Certain Problems: Some problems, particularly those involving tree-like structures or divide-and-conquer strategies, are naturally suited to recursive solutions.
- Importance of Base Cases: Properly defining base cases is crucial in recursive programming to prevent infinite recursion.
- Trade-offs: While recursive solutions can be elegant, they may not always be the most efficient in terms of memory usage or performance.
As you continue your programming journey, remember that recursion is a powerful technique, but it’s not always the best solution for every problem. The skills you’ve developed this week in thinking recursively will serve you well in problem-solving, whether you end up using a recursive or iterative approach.
Keep practicing, keep challenging yourself, and most importantly, keep coding! The world of programming is vast and exciting, and there’s always more to learn and explore.