Coding Without a Safety Net: A Month Without Stack Overflow
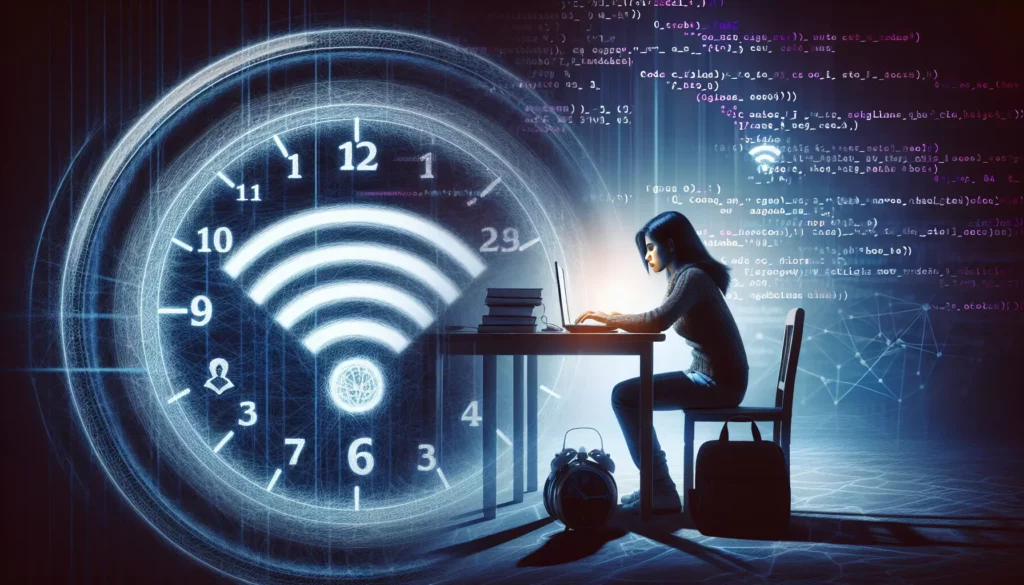
In the ever-evolving world of programming, Stack Overflow has become an indispensable resource for developers of all levels. It’s the go-to platform for quick solutions, code snippets, and expert advice. But what happens when you remove this safety net? What could a developer learn by deliberately avoiding Stack Overflow for an entire month? This blog post explores the challenges, insights, and potential growth that comes from coding without this ubiquitous resource.
The Challenge: Why Go Without Stack Overflow?
Before we dive into the experience, let’s consider why a developer might choose to forgo Stack Overflow:
- To build problem-solving skills
- To gain a deeper understanding of programming concepts
- To challenge oneself and break out of comfort zones
- To discover alternative learning resources
- To simulate real-world scenarios where quick answers aren’t always available
This challenge aligns perfectly with AlgoCademy’s mission of fostering algorithmic thinking and problem-solving skills. By removing the crutch of Stack Overflow, developers are forced to think more critically and develop a deeper understanding of the code they write.
Week 1: The Withdrawal
The first week without Stack Overflow was, unsurprisingly, the most challenging. Here’s a breakdown of the experience:
Day 1-3: The Panic
The initial reaction was one of panic. Simple tasks that would typically take minutes now stretched into hours. The muscle memory of opening a new tab and typing “Stack Overflow” had to be actively resisted.
Day 4-7: Finding Alternatives
As the week progressed, alternative resources began to emerge:
- Official documentation: Often overlooked, but incredibly valuable
- Books: Both physical and digital programming books
- Online courses: Platforms like AlgoCademy offer structured learning paths
- Community forums: Less instant than Stack Overflow, but often more in-depth
- Experimentation: Trial and error became a significant part of the process
Key Takeaway from Week 1
The most significant realization was how dependent we’ve become on quick answers. Without Stack Overflow, there was a forced slowdown in coding pace, but also a noticeable increase in understanding the ‘why’ behind solutions.
Week 2: The Adaptation
As the challenge continued into its second week, a new rhythm began to emerge:
Embracing the Documentation
Official documentation, once seen as dry and intimidating, became a treasure trove of information. For instance, when working with Python’s datetime module, instead of searching for a quick Stack Overflow answer, diving into the official docs revealed nuances and features that might have otherwise been missed.
Here’s an example of how one might use the datetime module, discovered through documentation rather than a quick online search:
from datetime import datetime, timedelta
# Get current date
current_date = datetime.now()
# Add 30 days to current date
future_date = current_date + timedelta(days=30)
print(f"Current date: {current_date.strftime('%Y-%m-%d')}")
print(f"Date after 30 days: {future_date.strftime('%Y-%m-%d')}")
Rediscovering Books
Programming books, both old and new, became valuable companions. They offered not just solutions, but context and deeper explanations. Books like “Clean Code” by Robert C. Martin and “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein provided insights that went beyond simple code snippets.
Leveraging IDE Features
Without the crutch of Stack Overflow, there was a newfound appreciation for IDE features. Code completion, inline documentation, and debugging tools became more integral to the coding process.
Key Takeaway from Week 2
The pace of coding remained slower, but the quality of understanding improved dramatically. Solutions were not just implemented, but truly comprehended.
Week 3: The Revelation
As the challenge entered its third week, some surprising benefits began to emerge:
Improved Problem-Solving Skills
Without the ability to quickly search for solutions, problem-solving skills were put to the test. Breaking down complex problems into smaller, manageable parts became second nature. This aligns perfectly with AlgoCademy’s emphasis on algorithmic thinking.
More Efficient Debugging
Debugging skills improved significantly. Instead of immediately searching for error messages online, there was a forced engagement with the code, leading to a better understanding of the errors and how to fix them.
Creative Solutions
Without the influence of Stack Overflow’s most popular answers, solutions became more creative and tailored to specific needs. For example, when faced with a problem of efficient data storage, instead of using a common solution found on Stack Overflow, a custom data structure was developed:
class EfficientStorage:
def __init__(self):
self.data = {}
self.timestamp = {}
def insert(self, key, value):
self.data[key] = value
self.timestamp[key] = datetime.now()
def get(self, key):
if key in self.data:
# Update timestamp on each access
self.timestamp[key] = datetime.now()
return self.data[key]
return None
def remove_oldest(self, n):
# Remove n oldest entries
sorted_keys = sorted(self.timestamp, key=self.timestamp.get)
for key in sorted_keys[:n]:
del self.data[key]
del self.timestamp[key]
Key Takeaway from Week 3
The most significant revelation was the boost in confidence. Solving problems independently led to a greater trust in personal abilities and instincts.
Week 4: The New Normal
In the final week of the challenge, a new approach to coding had solidified:
Structured Learning
Instead of relying on fragmented information from Stack Overflow, there was a shift towards more structured learning. Online courses, particularly those offered by platforms like AlgoCademy, became invaluable. These courses provided a comprehensive understanding of topics, rather than just quick fixes.
Community Engagement
Engaging with programming communities in a more meaningful way became the norm. Instead of just consuming information, there was more active participation in discussions, leading to deeper insights and networking opportunities.
Code Review and Pair Programming
Without Stack Overflow as a crutch, there was more reliance on peers for code reviews and pair programming sessions. This not only improved code quality but also enhanced communication skills and collaborative problem-solving abilities.
Building a Personal Knowledge Base
Throughout the month, a habit of documenting solutions and insights emerged. This personal knowledge base became a valuable resource, often more relevant and tailored than generic Stack Overflow answers.
Key Takeaway from Week 4
The final week demonstrated that while Stack Overflow is a valuable tool, it’s not irreplaceable. A more holistic approach to learning and problem-solving can lead to greater growth as a developer.
The Aftermath: Lessons Learned
After a month without Stack Overflow, several important lessons emerged:
1. The Importance of First Principles Thinking
Without quick answers at our fingertips, there was a natural gravitation towards first principles thinking. This approach, which involves breaking down complex problems into their most basic elements and then reassembling them, led to more robust and innovative solutions.
2. The Value of Struggle
The struggle to find solutions independently, while initially frustrating, ultimately led to deeper understanding and retention of knowledge. This aligns with cognitive science research that suggests that effort and difficulty in learning can lead to stronger neural connections and better long-term recall.
3. The Power of Documentation
Official documentation, once overlooked in favor of quick Stack Overflow answers, revealed itself to be an incredibly powerful resource. Not only did it provide solutions, but it also offered a comprehensive understanding of the tools and libraries being used.
4. The Benefit of Diverse Learning Resources
The challenge forced an exploration of diverse learning resources. From books and documentation to online courses and community forums, each resource offered unique benefits. This multi-faceted approach to learning led to a more well-rounded understanding of programming concepts.
5. The Growth in Problem-Solving Skills
Perhaps the most significant outcome was the growth in problem-solving skills. Without the ability to quickly find solutions online, there was a forced development of critical thinking and analytical skills. This improvement in problem-solving ability is particularly valuable in the context of technical interviews and real-world programming challenges.
Implementing the Lessons: A New Approach to Coding
Based on the insights gained from this month-long experiment, here’s a proposed new approach to coding and learning:
1. Start with the Documentation
Before searching for quick answers, make it a habit to consult the official documentation first. This not only provides accurate information but also helps in understanding the broader context and capabilities of the tool or library you’re using.
2. Embrace the Problem-Solving Process
When faced with a challenging problem, resist the urge to immediately search for a solution. Instead, break down the problem, attempt to solve it independently, and only then seek external input if needed. This process might look like this:
- Clearly define the problem
- Break it down into smaller, manageable parts
- Attempt to solve each part independently
- If stuck, consult documentation or other learning resources
- Implement and test the solution
- Reflect on the process and document the learning
3. Build a Personal Knowledge Base
Start documenting your learning journey, solutions to problems, and insights gained. This could be in the form of a personal wiki, a blog, or even detailed comments in your code. Here’s an example of how you might document a solution:
def fibonacci(n):
"""
Calculate the nth Fibonacci number using dynamic programming.
Time Complexity: O(n)
Space Complexity: O(1)
Learning Notes:
- Initially attempted a recursive solution, but it was inefficient for large n.
- Discovered the benefits of dynamic programming through this problem.
- Key insight: We only need to keep track of the last two numbers in the sequence.
@param n: The position of the Fibonacci number to calculate (0-indexed)
@return: The nth Fibonacci number
"""
if n <= 1:
return n
a, b = 0, 1
for _ in range(2, n + 1):
a, b = b, a + b
return b
4. Engage in Structured Learning
Complement your day-to-day coding with structured learning. This could involve:
- Taking online courses (like those offered by AlgoCademy) to build a strong foundation in algorithms and data structures
- Reading programming books to gain deeper insights into best practices and design patterns
- Participating in coding challenges to sharpen problem-solving skills
- Engaging in open-source projects to gain real-world experience and collaborate with other developers
5. Use Stack Overflow Wisely
While the challenge involved avoiding Stack Overflow completely, the goal isn’t to never use it again. Instead, use it more judiciously:
- Try to solve problems independently first
- When you do use Stack Overflow, don’t just copy-paste solutions. Understand the reasoning behind the answers
- Contribute back to the community by answering questions and sharing your own insights
Conclusion: The Path Forward
The month without Stack Overflow was challenging, enlightening, and ultimately transformative. It revealed the immense capacity for growth and learning that exists when we step out of our comfort zones and challenge our established patterns.
This experience aligns closely with the philosophy of platforms like AlgoCademy, which emphasize the importance of deep understanding, algorithmic thinking, and robust problem-solving skills. By focusing on these fundamental aspects of programming, rather than quick fixes, we can become more competent, confident, and creative developers.
As we move forward, the goal isn’t to abandon resources like Stack Overflow entirely, but to use them more mindfully as part of a broader, more holistic approach to learning and problem-solving. By combining independent thinking, diverse learning resources, and judicious use of community knowledge, we can continue to grow and evolve as developers, ready to tackle the complex challenges of modern software development.
Remember, the journey of a developer is one of continuous learning and improvement. Challenges like this one serve as reminders of our capacity for growth and the importance of stepping out of our comfort zones. So, whether you’re a beginner just starting your coding journey or an experienced developer looking to refine your skills, consider setting yourself similar challenges. You might be surprised by what you can achieve when you push your boundaries and code without a safety net.