Coding Parkour: Taking the Most Efficient Path Through Technical Interview Obstacles
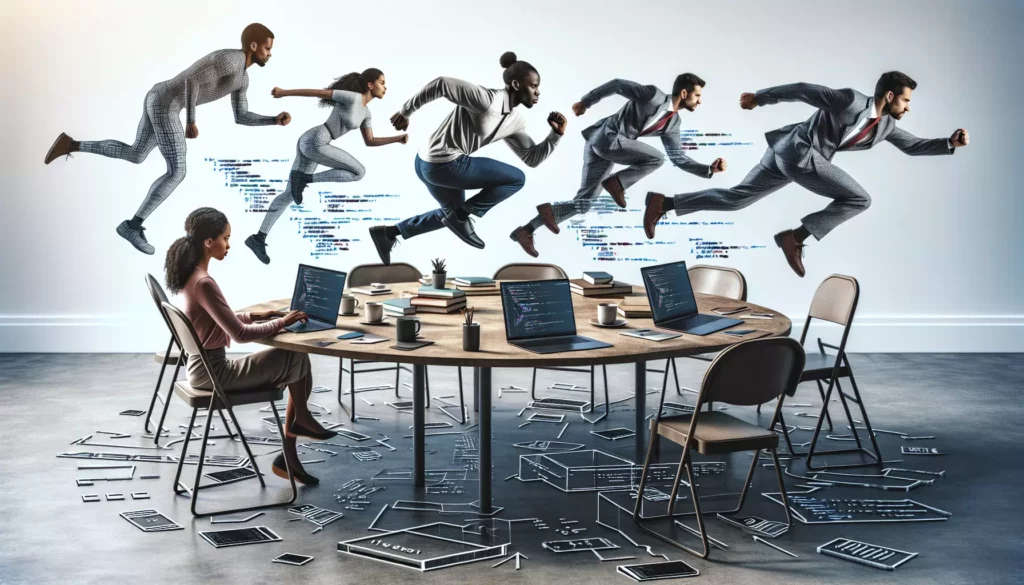
In the world of software development, technical interviews can feel like an intense obstacle course. Just as parkour practitioners navigate urban landscapes with grace and efficiency, successful candidates must swiftly and skillfully maneuver through complex coding challenges. This article will explore the art of “Coding Parkour” – the ability to take the most efficient path through technical interview obstacles, helping you land that dream job at top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google).
Understanding the Technical Interview Landscape
Before we dive into specific strategies, it’s crucial to understand the terrain you’ll be traversing. Technical interviews, especially at major tech companies, typically consist of several key components:
- Algorithmic problem-solving
- Data structure manipulation
- System design questions
- Coding on a whiteboard or in a shared editor
- Behavioral questions
Each of these elements presents its own set of challenges, much like the various obstacles in a parkour course. Your goal is to navigate them all with confidence and agility.
Mastering Algorithmic Thinking
At the heart of coding parkour is the ability to think algorithmically. This means breaking down complex problems into smaller, manageable steps and identifying efficient solutions. Here are some key strategies to enhance your algorithmic thinking:
1. Practice, Practice, Practice
Just as parkour athletes train relentlessly to perfect their moves, aspiring developers should solve coding problems regularly. Platforms like AlgoCademy offer a wealth of interactive coding tutorials and challenges to help you hone your skills.
2. Learn to Recognize Patterns
Many coding problems share similar patterns. By familiarizing yourself with common algorithmic patterns, you’ll be better equipped to tackle new challenges. Some essential patterns include:
- Two-pointer technique
- Sliding window
- Dynamic programming
- Depth-first search (DFS) and Breadth-first search (BFS)
- Binary search
3. Analyze Time and Space Complexity
Understanding the efficiency of your solutions is crucial. Learn to analyze the time and space complexity of your algorithms using Big O notation. This will help you optimize your code and impress your interviewers.
Navigating Data Structures with Ease
Data structures are the building blocks of efficient algorithms. Like a parkour artist who knows how to use every surface to their advantage, a skilled programmer must be familiar with various data structures and their applications.
1. Master the Fundamentals
Ensure you have a solid grasp of basic data structures such as:
- Arrays and strings
- Linked lists
- Stacks and queues
- Trees and graphs
- Hash tables
2. Understand Trade-offs
Each data structure has its strengths and weaknesses. Learn when to use one over another based on the problem requirements and performance considerations.
3. Implement from Scratch
Practice implementing data structures from scratch. This deep understanding will give you the confidence to manipulate and optimize them during interviews.
Tackling Coding Challenges with Finesse
When faced with a coding challenge in an interview, approach it like a parkour run – with a clear plan and smooth execution.
1. The UMPIRE Method
Use the UMPIRE method to structure your approach:
- Understand the problem
- Match the problem to known patterns
- Plan your approach
- Implement the solution
- Review your code
- Evaluate alternative solutions
2. Communicate Clearly
Explain your thought process as you work through the problem. This gives the interviewer insight into your problem-solving skills and allows them to provide hints if needed.
3. Start with a Brute Force Solution
Begin with a simple, working solution before optimizing. This demonstrates your ability to solve the problem and provides a foundation for improvement.
Optimizing Your Code
Like a parkour artist refining their movements, you should always look for ways to optimize your code. Here are some techniques to consider:
1. Space-Time Trade-offs
Sometimes, you can improve time complexity by using more memory, or vice versa. Understand these trade-offs and be prepared to discuss them.
2. Preprocessing
For problems involving repeated operations on static data, consider preprocessing the data to improve overall efficiency.
3. Caching and Memoization
Use techniques like caching and memoization to avoid redundant computations in recursive or repetitive algorithms.
Mastering System Design Questions
System design questions test your ability to architect large-scale systems. This is where your parkour skills truly come into play, as you navigate complex landscapes of distributed systems and scalability challenges.
1. Understand the Requirements
Start by clarifying the system’s functional and non-functional requirements. This sets the foundation for your design.
2. Break It Down
Divide the system into manageable components. This might include:
- Client-side considerations
- API design
- Data storage
- Caching mechanisms
- Load balancing
3. Scale It Up
Discuss how your system would handle increased load. Consider techniques like:
- Horizontal vs. vertical scaling
- Database sharding
- Caching strategies
- Content delivery networks (CDNs)
Whiteboard and Shared Editor Strategies
Coding on a whiteboard or in a shared editor can be daunting. Here’s how to approach it with the grace of a parkour artist:
1. Practice Writing Code by Hand
Get comfortable writing code without the aid of an IDE. This will help you focus on the logic rather than syntax highlighting or auto-completion.
2. Use Pseudocode
Start with pseudocode to outline your approach before diving into the actual implementation. This helps organize your thoughts and catch logical errors early.
3. Leave Room for Edits
Whether on a whiteboard or in a text editor, leave space between lines and sections. This allows for easy insertion of forgotten details or optimizations.
Navigating Behavioral Questions
While technical skills are crucial, behavioral questions are equally important. They assess your ability to work in a team, handle conflicts, and align with the company culture.
1. Use the STAR Method
When answering behavioral questions, use the STAR method:
- Situation: Set the context
- Task: Describe your responsibility
- Action: Explain what you did
- Result: Share the outcome
2. Prepare Stories
Have a repertoire of stories that showcase your problem-solving skills, leadership abilities, and teamwork. Tailor these to the company’s values and culture.
3. Show Growth Mindset
Demonstrate your ability to learn from challenges and your enthusiasm for continuous improvement.
Tools and Resources for Your Coding Parkour Journey
To excel in your coding parkour journey, leverage these tools and resources:
1. Online Platforms
Utilize platforms like AlgoCademy that offer interactive coding tutorials, AI-powered assistance, and a vast array of practice problems.
2. Coding Books
Invest in classic coding interview books such as “Cracking the Coding Interview” by Gayle Laakmann McDowell or “Elements of Programming Interviews” by Adnan Aziz, Tsung-Hsien Lee, and Amit Prakash.
3. Mock Interviews
Practice with peers or use services that offer mock technical interviews with experienced professionals.
Putting It All Together: A Sample Coding Challenge
Let’s walk through a sample coding challenge to demonstrate the coding parkour approach in action. Consider the following problem:
Given an array of integers, find the contiguous subarray with the largest sum.
This is the classic “Maximum Subarray” problem. Let’s apply our coding parkour skills:
1. Understand the Problem
We need to find a contiguous sequence of numbers in the array that adds up to the largest possible sum.
2. Match to Known Patterns
This problem can be solved using dynamic programming or the Kadane’s algorithm, which is an efficient way to solve the maximum subarray problem.
3. Plan the Approach
We’ll use Kadane’s algorithm, which involves iterating through the array once, keeping track of the maximum sum seen so far and the current sum.
4. Implement the Solution
Here’s a Python implementation of Kadane’s algorithm:
def max_subarray(nums):
max_sum = float('-inf')
current_sum = 0
for num in nums:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
# Test the function
arr = [-2, 1, -3, 4, -1, 2, 1, -5, 4]
result = max_subarray(arr)
print(f"The maximum subarray sum is: {result}")
5. Review the Code
Let’s break down the solution:
- We initialize
max_sum
to negative infinity to handle cases where all numbers are negative. current_sum
keeps track of the sum of the current subarray we’re considering.- We iterate through each number in the array.
- At each step, we decide whether to start a new subarray (if
current_sum
becomes negative) or extend the current one. - We update
max_sum
if thecurrent_sum
is larger.
6. Evaluate Alternatives
While this solution is optimal with O(n) time complexity and O(1) space complexity, we could discuss alternative approaches:
- Brute force approach: Check all possible subarrays (O(n^2) time complexity)
- Divide and conquer approach: Similar to merge sort, divide the array and solve recursively (O(n log n) time complexity)
Conclusion: Mastering the Art of Coding Parkour
Navigating technical interviews requires a combination of skills, much like the discipline of parkour. By mastering algorithmic thinking, data structures, problem-solving techniques, and communication skills, you can gracefully overcome the obstacles in your path to landing your dream job at top tech companies.
Remember, the key to success lies in consistent practice and a growth mindset. Platforms like AlgoCademy offer the perfect training ground to hone your skills and prepare for the challenges ahead. With dedication and the right approach, you’ll be performing coding parkour like a pro in no time, impressing interviewers and securing your place in the tech industry.
So, gear up, start practicing, and get ready to take the most efficient path through your next technical interview. Your coding parkour journey begins now!