Coding Interviews: The Hidden Benefit of Learning How to Manage Stress
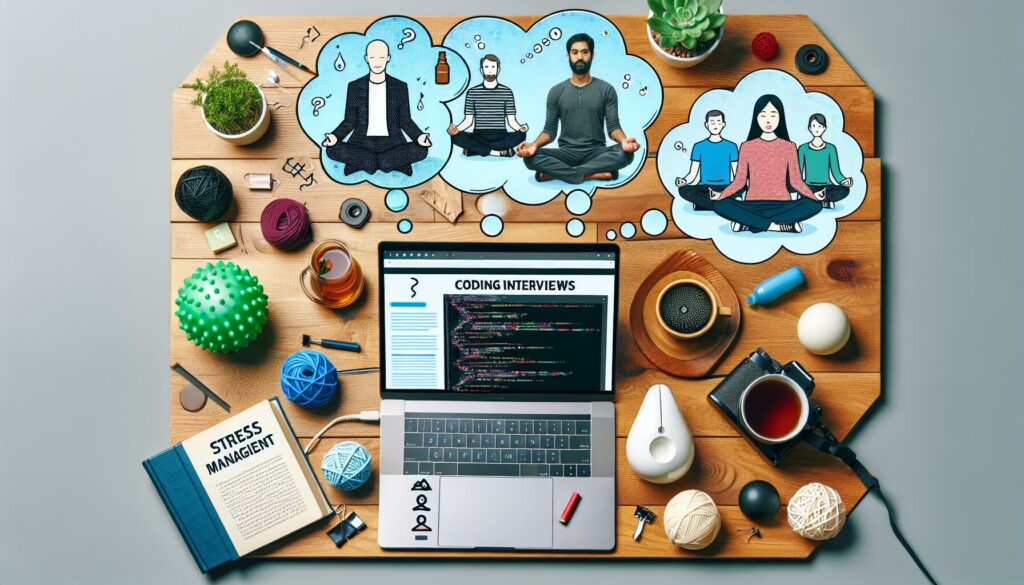
As aspiring software developers prepare for coding interviews, they often focus solely on mastering algorithms, data structures, and problem-solving techniques. While these technical skills are undoubtedly crucial, there’s a hidden benefit to the interview preparation process that’s often overlooked: learning how to manage stress. In this article, we’ll explore why stress management is a vital skill for both coding interviews and your future career in tech, and provide practical strategies to help you stay calm under pressure.
The Pressure Cooker of Coding Interviews
Coding interviews, especially those conducted by major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google), are notoriously challenging. They’re designed to test not only your technical prowess but also your ability to perform under pressure. Here’s why coding interviews can be particularly stressful:
- Time constraints: You’re often given complex problems to solve in a limited timeframe.
- Live coding: You have to write code in real-time while explaining your thought process.
- High stakes: Your performance can determine whether you land your dream job.
- Unfamiliar environment: You may be interviewing in an unfamiliar setting or via video call.
- Imposter syndrome: You might feel like you don’t belong or aren’t qualified enough.
Given these factors, it’s no wonder that even the most technically proficient candidates can falter under the weight of interview anxiety. This is where stress management becomes a game-changer.
Why Stress Management Matters in Tech Careers
Learning to manage stress during coding interviews isn’t just about landing the job; it’s a skill that will serve you throughout your career in tech. Here’s why:
1. Deadlines and Project Pressure
In the fast-paced world of software development, you’ll frequently face tight deadlines and high-pressure situations. The ability to remain calm and focused under stress will help you meet these challenges head-on.
2. Debugging and Problem-Solving
When you encounter a critical bug or system failure, staying cool under pressure can mean the difference between a quick resolution and a prolonged crisis.
3. Collaboration and Communication
Stress can negatively impact your ability to communicate effectively with team members and stakeholders. Managing your stress levels helps maintain clear, professional communication.
4. Continuous Learning
The tech industry evolves rapidly, requiring constant learning and adaptation. Stress management skills help you approach new challenges with a growth mindset rather than feeling overwhelmed.
5. Work-Life Balance
Effective stress management techniques learned during interview preparation can help you maintain a healthy work-life balance throughout your career.
Strategies for Managing Stress in Coding Interviews
Now that we understand the importance of stress management, let’s explore some practical strategies you can employ to keep your cool during coding interviews:
1. Practice, Practice, Practice
The more you practice coding problems under simulated interview conditions, the more comfortable you’ll become with the process. Use platforms like AlgoCademy to work through a variety of coding challenges and get used to explaining your thought process out loud.
2. Mindfulness and Meditation
Incorporating mindfulness techniques or meditation into your daily routine can help reduce overall stress levels and improve your ability to focus under pressure. Even a few minutes of deep breathing before an interview can make a significant difference.
3. Reframe Your Perspective
Instead of viewing the interview as a high-stakes test, try to see it as an opportunity to showcase your skills and learn something new. This shift in perspective can help alleviate some of the pressure you feel.
4. Develop a Pre-Interview Routine
Create a calming pre-interview routine that helps you center yourself. This might include reviewing your strengths, doing some light stretching, or listening to music that puts you in a positive mindset.
5. Use the STAR Method
When answering behavioral questions or explaining your problem-solving approach, use the STAR method (Situation, Task, Action, Result) to structure your responses. This can help you stay focused and organized under pressure.
6. Embrace the Rubber Duck Technique
Practice explaining your code and thought process to an inanimate object, like a rubber duck. This technique can help you clarify your thinking and get comfortable with verbalizing your problem-solving approach.
7. Time Management Skills
Develop strong time management skills to help you pace yourself during coding challenges. Practice allocating time for understanding the problem, planning your approach, coding, and testing your solution.
8. Physical Preparation
Don’t underestimate the impact of physical well-being on your mental state. Get enough sleep, eat a balanced meal before the interview, and stay hydrated to help your brain function at its best.
Implementing Stress Management in Your Interview Prep
To truly benefit from stress management techniques, it’s important to incorporate them into your regular interview preparation routine. Here’s how you can do that:
1. Set Up Mock Interviews
Arrange mock interviews with friends, mentors, or through online platforms. Simulate the interview environment as closely as possible, including time constraints and live coding elements. This will help you get accustomed to the pressure and identify areas where stress affects your performance.
2. Utilize AI-Powered Interview Prep Tools
Take advantage of AI-powered interview preparation tools that can provide real-time feedback on your coding solutions and problem-solving approach. These tools can help you build confidence and reduce anxiety by familiarizing you with the interview process.
3. Create a Stress Journal
Keep a journal to track your stress levels during practice sessions. Note what triggers your stress and which coping mechanisms work best for you. This self-awareness will be invaluable when you’re in the actual interview.
4. Gradually Increase Difficulty
Start with easier coding problems and gradually work your way up to more complex challenges. This progressive approach helps build confidence and reduces the likelihood of feeling overwhelmed during the actual interview.
5. Practice Explaining Your Thought Process
Get comfortable with thinking out loud while coding. This not only mimics the interview environment but also helps interviewers understand your problem-solving approach, even if you don’t arrive at the perfect solution.
The Long-Term Benefits of Stress Management Skills
While the immediate goal might be to ace your coding interviews, the stress management skills you develop will continue to pay dividends throughout your career in tech. Here’s how:
1. Improved Decision-Making
When you’re able to manage stress effectively, you’re more likely to make clear, rational decisions. This is crucial in a field where quick thinking and sound judgment are often required.
2. Enhanced Creativity
Stress can stifle creativity, which is an essential component of innovative problem-solving in tech. By managing your stress levels, you open up mental space for more creative and elegant solutions to coding challenges.
3. Better Team Dynamics
Your ability to remain calm under pressure can have a positive impact on your entire team. It can help create a more relaxed and productive work environment, leading to better collaboration and outcomes.
4. Career Resilience
The tech industry is known for its ups and downs. Whether it’s navigating layoffs, startup volatility, or rapid technological changes, your stress management skills will help you maintain perspective and adapt to new situations more easily.
5. Leadership Potential
As you progress in your career, the ability to stay cool under pressure becomes increasingly valuable. It’s a key trait that managers and executives look for when identifying future leaders in the organization.
Coding Examples: Stress-Testing Your Skills
To help you practice managing stress while coding, let’s look at a couple of examples that might come up in an interview situation. Remember, the goal is not just to solve the problem, but to remain calm and articulate your thought process as you work through it.
Example 1: Reversing a Linked List
Here’s a classic problem that often appears in coding interviews. Try to solve it while imagining you’re in an interview setting:
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def reverseLinkedList(head):
prev = None
current = head
while current is not None:
next_temp = current.next
current.next = prev
prev = current
current = next_temp
return prev
# Test the function
# Create a linked list: 1 -> 2 -> 3 -> 4 -> 5
head = ListNode(1)
head.next = ListNode(2)
head.next.next = ListNode(3)
head.next.next.next = ListNode(4)
head.next.next.next.next = ListNode(5)
# Reverse the linked list
new_head = reverseLinkedList(head)
# Print the reversed list
while new_head:
print(new_head.val, end=" -> ")
new_head = new_head.next
print("None")
As you work through this problem, practice explaining each step out loud. Focus on maintaining a steady breathing pattern and a calm demeanor, even if you encounter difficulties.
Example 2: Implementing a Queue using Two Stacks
This problem tests your understanding of data structures and your ability to think creatively under pressure:
class MyQueue:
def __init__(self):
self.stack1 = []
self.stack2 = []
def push(self, x: int) -> None:
self.stack1.append(x)
def pop(self) -> int:
self._transfer()
return self.stack2.pop()
def peek(self) -> int:
self._transfer()
return self.stack2[-1]
def empty(self) -> bool:
return len(self.stack1) == 0 and len(self.stack2) == 0
def _transfer(self):
if not self.stack2:
while self.stack1:
self.stack2.append(self.stack1.pop())
# Test the MyQueue class
queue = MyQueue()
queue.push(1)
queue.push(2)
print(queue.peek()) # Output: 1
print(queue.pop()) # Output: 1
print(queue.empty()) # Output: False
As you implement this solution, practice staying calm if you make a mistake or if your initial approach doesn’t work. Remember that the interview process is as much about seeing how you handle challenges as it is about getting the perfect answer.
Conclusion: Embracing the Challenge
As you prepare for coding interviews, remember that learning to manage stress is not just an added bonus—it’s an essential skill that will serve you well throughout your career in tech. By incorporating stress management techniques into your interview preparation, you’re not only increasing your chances of success in landing your dream job but also building resilience that will help you thrive in the fast-paced, ever-changing world of software development.
Platforms like AlgoCademy offer valuable resources for honing both your technical skills and your ability to perform under pressure. Take advantage of these tools, but also remember to step back and focus on your overall well-being. A balanced approach that addresses both your coding abilities and your mental resilience will set you up for long-term success in the tech industry.
Embrace the challenge of coding interviews as an opportunity for growth. With each practice session and each real interview experience, you’re not just becoming a better coder—you’re becoming a more composed, confident, and capable professional. The skills you develop in managing stress during this process will be your secret weapon, not just in acing interviews, but in navigating the exciting and demanding career that lies ahead of you in the world of technology.