Coding in Iambic Pentameter: Merging Shakespeare with Syntax for Memorable Solutions
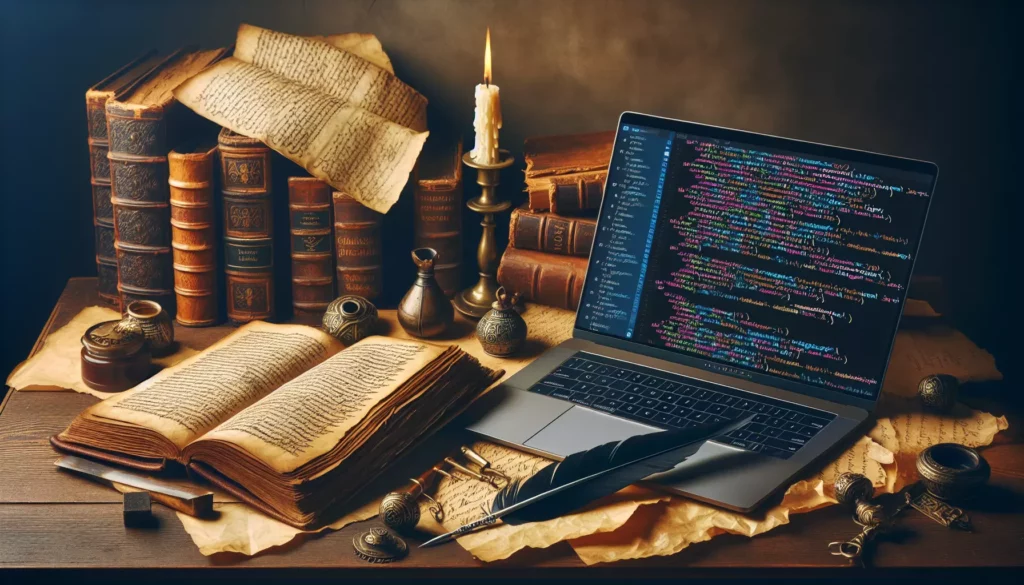
In the realm of coding, where logic reigns supreme and syntax is king, an unexpected muse has emerged to breathe new life into the art of programming. Enter the world of “Coding in Iambic Pentameter,” a creative fusion of Shakespearean verse and modern programming that’s capturing the imagination of developers and literature enthusiasts alike. This unique approach not only adds a touch of poetic flair to the often dry world of code but also serves as a powerful mnemonic device, helping programmers remember complex algorithms and structures with greater ease.
The Bard Meets Binary: An Unlikely Alliance
At first glance, the structured world of programming and the flowing verses of Shakespeare might seem worlds apart. However, upon closer inspection, both share a fundamental characteristic: they rely on patterns and rhythm to convey meaning. Just as iambic pentameter follows a specific meter of unstressed and stressed syllables, programming languages adhere to strict syntactical rules and structures.
The idea of merging these two disciplines isn’t as far-fetched as it might initially appear. In fact, it’s a testament to the creative problem-solving skills that both programmers and poets must possess. By combining the logical precision of code with the lyrical beauty of iambic pentameter, developers are discovering new ways to approach problem-solving and algorithm design.
The Benefits of Poetic Programming
Coding in iambic pentameter offers several unexpected advantages:
- Enhanced Memorization: The rhythmic nature of iambic pentameter makes it easier to remember complex code structures and algorithms.
- Improved Creativity: The constraint of writing code in verse form encourages out-of-the-box thinking and novel solutions.
- Better Documentation: Poetic comments can make code more engaging and memorable for other developers.
- Stress Relief: The playful nature of this approach can help alleviate the stress often associated with coding challenges.
- Interdisciplinary Learning: It bridges the gap between the arts and sciences, potentially attracting a more diverse group of individuals to programming.
Practical Applications: From Sonnets to Sorting Algorithms
Let’s explore how we might implement a simple sorting algorithm, like bubble sort, using iambic pentameter. Here’s an example in Python:
def bubble_sort(arr):
# In code, we sort with grace and might,
# A bubble rises, sets all things right.
n = len(arr)
for i in range(n):
# Through each pass, we gently compare,
# And swap the greater with utmost care.
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
# If left exceeds the right in size,
# We swap them swift before our eyes.
arr[j], arr[j + 1] = arr[j + 1], arr[j]
# Our task complete, the list now true,
# Returns in order, old to new.
return arr
In this example, we’ve maintained the logical structure of the bubble sort algorithm while adding poetic comments that explain each step in iambic pentameter. The rhythm of the verse helps reinforce the flow of the algorithm, making it more memorable for learners and adding a touch of whimsy to an otherwise straightforward piece of code.
Challenges and Considerations
While coding in iambic pentameter can be a fun and educational exercise, it’s important to consider its limitations and potential drawbacks:
- Readability Concerns: Overly poetic code might sacrifice clarity for style, potentially making it harder for other developers to understand and maintain.
- Time Constraints: Writing code in verse form undoubtedly takes more time and effort, which may not be practical in professional settings with tight deadlines.
- Learning Curve: Not all programmers are versed in poetic meters, which could create a barrier to entry for some.
- Maintaining Consistency: It can be challenging to maintain the iambic pentameter structure throughout larger codebases or more complex algorithms.
Integrating Poetic Programming into Education
Despite these challenges, the concept of coding in iambic pentameter holds significant potential as an educational tool. By incorporating this approach into coding curricula, educators can:
- Engage Different Learning Styles: Visual and auditory learners may find the rhythmic nature of iambic pentameter helpful in grasping coding concepts.
- Encourage Creativity: Students are challenged to think about code in a new way, fostering creativity and problem-solving skills.
- Bridge Disciplines: This approach can help attract students from humanities backgrounds to explore computer science.
- Improve Code Documentation: Teaching students to write clear, engaging comments in verse form can lead to better documentation habits.
Examples in Various Programming Languages
Let’s explore how coding in iambic pentameter might look in different programming languages:
JavaScript
function fibonacci(n) {
// In Fibonacci's sequence grand,
// Each number joins its neighbor's hand.
if (n <= 1) return n;
// For greater sums, we must recurse,
// And add the last two in our verse.
return fibonacci(n - 1) + fibonacci(n - 2);
}
Java
public class PrimeChecker {
public static boolean isPrime(int num) {
// A prime, alone it stands so tall,
// Divisible by one and all.
if (num <= 1) return false;
// We check each number in its range,
// To see if any can arrange.
for (int i = 2; i <= Math.sqrt(num); i++) {
if (num % i == 0) return false;
}
// If none divide, then prime it be,
// A number special, wild and free.
return true;
}
}
C++
#include <iostream>
#include <vector>
std::vector<int> merge_sort(std::vector<int> arr) {
// If but one element doth reside,
// No need to sort, let it abide.
if (arr.size() <= 1) return arr;
// Divide the array in twain with care,
// Then conquer each half with flair.
int mid = arr.size() / 2;
std::vector<int> left(arr.begin(), arr.begin() + mid);
std::vector<int> right(arr.begin() + mid, arr.end());
left = merge_sort(left);
right = merge_sort(right);
// Now merge the halves with grace and might,
// Till order reigns and all is right.
std::vector<int> result;
int i = 0, j = 0;
while (i < left.size() && j < right.size()) {
if (left[i] < right[j]) {
result.push_back(left[i++]);
} else {
result.push_back(right[j++]);
}
}
while (i < left.size()) result.push_back(left[i++]);
while (j < right.size()) result.push_back(right[j++]);
// Our task complete, the list now true,
// Returns in order, old to new.
return result;
}
The Future of Poetic Programming
As the field of computer science continues to evolve, interdisciplinary approaches like coding in iambic pentameter may play an increasingly important role in education and professional development. Here are some potential future developments:
- AI-Assisted Poetic Coding: Machine learning algorithms could be developed to assist in translating standard code into iambic pentameter or other poetic forms, making it easier for developers to adopt this style.
- Coding Poetry Slams: Competitive events where programmers showcase their ability to solve complex problems using code written in verse could become popular in tech communities and educational institutions.
- Language-Specific Poetic Styles: Different programming languages might develop their own unique poetic styles that complement their syntax and structure.
- Poetic Code Analyzers: Tools could be created to analyze code for both syntactical correctness and adherence to poetic meters, helping developers refine their poetic programming skills.
- Integration with Natural Language Processing: As NLP technologies advance, we might see the development of systems that can understand and generate code in various poetic forms, bridging the gap between human language and programming languages.
Conclusion: A New Verse in Coding Education
Coding in iambic pentameter represents more than just a quirky experiment; it’s a testament to the creative potential that exists at the intersection of art and technology. By merging the structure of programming with the beauty of poetry, we open up new avenues for learning, problem-solving, and self-expression in the world of computer science.
While it may not replace traditional coding practices, this approach offers a valuable supplement to existing educational methods. It challenges us to think about code in new ways, potentially leading to innovative solutions and a deeper appreciation for the artistry inherent in programming.
As we continue to explore the boundaries between different disciplines, approaches like coding in iambic pentameter remind us that creativity knows no bounds. In the grand tapestry of technology and art, this unique blend of Shakespeare and syntax weaves a new pattern, inviting both seasoned developers and aspiring coders to see their craft through a different lens.
So, whether you’re a programmer looking to add some flair to your code or an educator seeking new ways to engage your students, consider the rhythmic potential of iambic pentameter. In the words of the Bard himself, “To code, or not to code in verse? That is the question.” And perhaps, in exploring this question, we’ll discover new depths to the art of programming, one poetic line at a time.