Coding in Character: Approaching Problems as Famous Programmers Throughout History
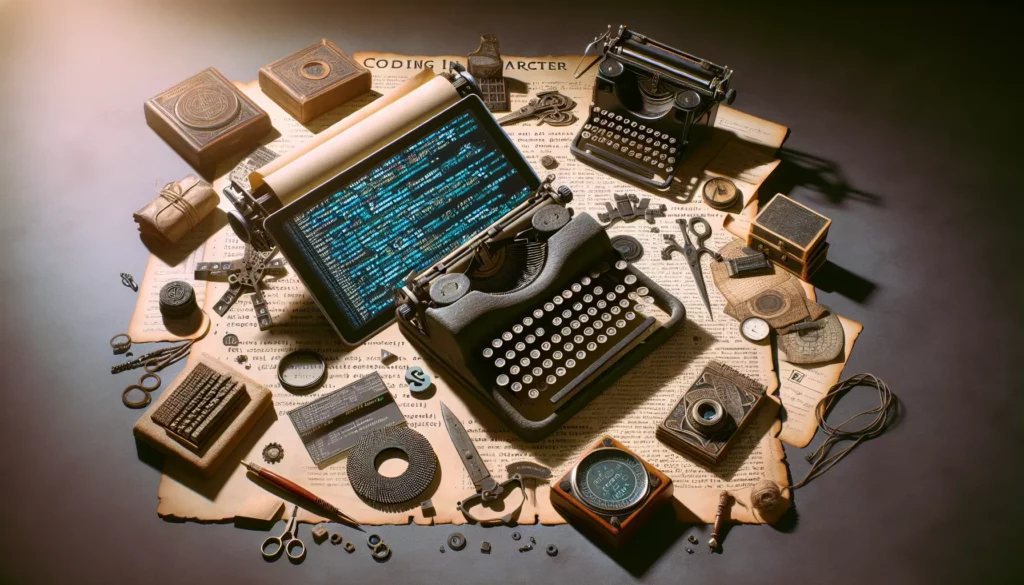
In the vast landscape of programming and computer science, we often find ourselves facing complex problems that require innovative solutions. While modern tools and techniques are invaluable, there’s much to be learned from the approaches of legendary programmers throughout history. In this article, we’ll explore how adopting the mindset of famous programmers can enhance our problem-solving skills and provide fresh perspectives on coding challenges.
The Value of Historical Perspectives in Coding
Before we dive into specific personalities, it’s worth considering why looking back at historical figures in programming can be beneficial:
- Foundational Thinking: Early programmers often worked with severe hardware limitations, forcing them to think creatively and efficiently.
- Diverse Approaches: Each programmer brought unique philosophies and methodologies to their work, offering a range of problem-solving strategies.
- Inspiration: Understanding the challenges these pioneers overcame can motivate us in our own coding journeys.
- Historical Context: Seeing how programming has evolved helps us appreciate current tools and practices while identifying timeless principles.
Now, let’s explore some iconic programmers and how we might approach problems through their lenses.
1. Ada Lovelace: The Analytical Approach
Ada Lovelace, often regarded as the world’s first computer programmer, was known for her analytical and forward-thinking approach to computation.
Lovelace’s Approach:
- Meticulous Planning: Lovelace was known for her detailed notes and thorough analysis before implementation.
- Interdisciplinary Thinking: She saw connections between mathematics, science, and even poetry, applying broad knowledge to problem-solving.
- Visionary Concepts: Lovelace imagined computers could be used for more than just calculations, foreseeing their potential for creating music and art.
Applying Lovelace’s Method:
When facing a complex coding problem, channel Lovelace by:
- Thoroughly analyzing the problem before writing any code.
- Creating detailed flowcharts or pseudocode to map out your solution.
- Considering how your solution might be applied beyond its immediate use case.
Here’s an example of how we might approach a sorting problem with Lovelace’s analytical mindset:
def lovelace_sort(arr):
# Step 1: Analyze the input
print(f"Input array: {arr}")
print(f"Array length: {len(arr)}")
# Step 2: Plan the sorting process
print("Sorting plan:")
print("1. Iterate through the array")
print("2. Compare each element with the next")
print("3. Swap if out of order")
print("4. Repeat until no swaps are needed")
# Step 3: Implement the sorting algorithm (Bubble Sort)
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
# Step 4: Analyze the output
print(f"Sorted array: {arr}")
print("Potential applications: Organizing data, Preparing for binary search, etc.")
return arr
# Example usage
unsorted_array = [64, 34, 25, 12, 22, 11, 90]
sorted_array = lovelace_sort(unsorted_array)
This approach, while more verbose, encourages a deeper understanding of the problem and solution, true to Lovelace’s analytical style.
2. Alan Turing: Breaking Down Complex Problems
Alan Turing, famous for his work in theoretical computer science and artificial intelligence, was adept at tackling seemingly insurmountable problems by breaking them down into manageable parts.
Turing’s Approach:
- Abstraction: Turing excelled at creating abstract models of complex systems.
- Systematic Problem-Solving: He approached problems step-by-step, often using his concept of the Turing machine.
- Questioning Assumptions: Turing wasn’t afraid to challenge existing paradigms and think outside the box.
Applying Turing’s Method:
To approach a problem like Turing:
- Break the problem down into its smallest possible components.
- Create abstract models or simulations of each component.
- Systematically solve each part before combining them into a whole solution.
Let’s apply Turing’s approach to solving a classic computer science problem: determining if a string is a palindrome.
def turing_palindrome_checker(s):
# Step 1: Abstract the problem
print("Problem: Determine if a string is a palindrome")
print("Abstraction: Compare characters from both ends, moving inward")
# Step 2: Break down the problem
print("Steps:")
print("1. Preprocess the string (remove spaces, convert to lowercase)")
print("2. Set up two pointers (start and end of string)")
print("3. Compare characters at pointers")
print("4. Move pointers inward")
print("5. Repeat until pointers meet or mismatch found")
# Step 3: Implement the solution
def preprocess(string):
return ''.join(char.lower() for char in string if char.isalnum())
s = preprocess(s)
left, right = 0, len(s) - 1
while left < right:
if s[left] != s[right]:
return False
left += 1
right -= 1
return True
# Example usage
test_string = "A man, a plan, a canal: Panama"
result = turing_palindrome_checker(test_string)
print(f"Is '{test_string}' a palindrome? {result}")
This implementation showcases Turing’s systematic approach, breaking down the problem into clear, logical steps before implementation.
3. Grace Hopper: Innovating and Simplifying
Grace Hopper, a pioneer in computer programming, is known for her work on early compilers and for popularizing machine-independent programming languages.
Hopper’s Approach:
- Simplification: Hopper believed in making programming more accessible and understandable.
- Innovation: She wasn’t afraid to challenge the status quo and develop new solutions.
- Practical Problem-Solving: Hopper focused on real-world applications and user-friendly designs.
Applying Hopper’s Method:
To code like Grace Hopper:
- Look for ways to simplify complex processes.
- Consider the end-user experience in your design.
- Don’t be afraid to innovate or create new tools if existing ones are inadequate.
Let’s apply Hopper’s approach to creating a simple, user-friendly calculator:
def hopper_calculator():
print("Welcome to the Hopper Simple Calculator!")
print("This calculator uses simple English commands.")
while True:
command = input("Enter your calculation (e.g., 'add 5 and 3') or 'quit' to exit: ").lower()
if command == 'quit':
print("Thank you for using the Hopper Calculator. Goodbye!")
break
parts = command.split()
if len(parts) != 4 or parts[2] != 'and':
print("I'm sorry, I didn't understand that. Please use the format: operation number and number")
continue
operation, num1, _, num2 = parts
try:
num1, num2 = float(num1), float(num2)
except ValueError:
print("Please enter valid numbers.")
continue
if operation == 'add':
result = num1 + num2
elif operation == 'subtract':
result = num1 - num2
elif operation == 'multiply':
result = num1 * num2
elif operation == 'divide':
if num2 == 0:
print("Cannot divide by zero!")
continue
result = num1 / num2
else:
print(f"I'm sorry, I don't know how to {operation}.")
continue
print(f"The result is: {result}")
# Run the calculator
hopper_calculator()
This calculator embodies Hopper’s principles by using simple English commands, providing clear instructions, and focusing on user-friendly interaction.
4. Linus Torvalds: Open Collaboration and Modularity
Linus Torvalds, creator of Linux and Git, is known for his approach to open-source development and creating modular, scalable systems.
Torvalds’ Approach:
- Modularity: Designing systems with independent, interchangeable components.
- Open Collaboration: Leveraging community contributions and feedback.
- Pragmatism: Focusing on practical solutions rather than theoretical perfection.
Applying Torvalds’ Method:
To code like Linus Torvalds:
- Design your code with modularity in mind, allowing for easy updates and extensions.
- Embrace open-source principles, documenting your code well and considering how others might contribute.
- Focus on creating working solutions quickly, then refine based on real-world usage and feedback.
Let’s create a simple, modular text processing system inspired by Torvalds’ approach:
import re
class TextProcessor:
def __init__(self):
self.processors = {}
def register_processor(self, name, func):
self.processors[name] = func
def process(self, text, operations):
for op in operations:
if op in self.processors:
text = self.processors[op](text)
else:
print(f"Warning: Unknown operation '{op}'. Skipping.")
return text
# Define modular text processing functions
def to_lowercase(text):
return text.lower()
def remove_punctuation(text):
return re.sub(r'[^\w\s]', '', text)
def remove_numbers(text):
return re.sub(r'\d+', '', text)
# Create and set up the text processor
processor = TextProcessor()
processor.register_processor('lowercase', to_lowercase)
processor.register_processor('remove_punctuation', remove_punctuation)
processor.register_processor('remove_numbers', remove_numbers)
# Example usage
text = "Hello, World! 123 This is a TEST."
operations = ['lowercase', 'remove_punctuation', 'remove_numbers']
result = processor.process(text, operations)
print(f"Original: {text}")
print(f"Processed: {result}")
# Community contribution (simulating open-source collaboration)
def reverse_words(text):
return ' '.join(word[::-1] for word in text.split())
processor.register_processor('reverse_words', reverse_words)
result_with_reverse = processor.process(result, ['reverse_words'])
print(f"With community contribution: {result_with_reverse}")
This example demonstrates modularity, allowing easy addition of new text processing functions, and simulates the open collaboration model that Torvalds champions.
5. Margaret Hamilton: Rigorous Systems Thinking
Margaret Hamilton, who coined the term “software engineering,” is known for her work on the Apollo space program and her emphasis on rigorous system design and error prevention.
Hamilton’s Approach:
- Systems Thinking: Considering how individual components interact within a larger system.
- Error Prevention: Designing systems to anticipate and handle potential errors.
- Thorough Testing: Emphasizing comprehensive testing to ensure reliability.
Applying Hamilton’s Method:
To code like Margaret Hamilton:
- Think about your code as part of a larger system, considering all possible interactions and edge cases.
- Implement robust error handling and recovery mechanisms.
- Develop comprehensive test suites to validate your code under various conditions.
Let’s apply Hamilton’s approach to creating a robust file processing system:
import os
import logging
class RobustFileProcessor:
def __init__(self, input_dir, output_dir):
self.input_dir = input_dir
self.output_dir = output_dir
self.setup_logging()
def setup_logging(self):
logging.basicConfig(filename='file_processor.log', level=logging.INFO,
format='%(asctime)s - %(levelname)s - %(message)s')
def process_files(self):
try:
self.check_directories()
files = self.get_input_files()
for file in files:
try:
self.process_file(file)
except Exception as e:
logging.error(f"Error processing file {file}: {str(e)}")
logging.info("File processing completed successfully.")
except Exception as e:
logging.critical(f"Critical error in file processing: {str(e)}")
def check_directories(self):
if not os.path.exists(self.input_dir):
raise FileNotFoundError(f"Input directory {self.input_dir} does not exist.")
if not os.path.exists(self.output_dir):
os.makedirs(self.output_dir)
logging.info(f"Created output directory {self.output_dir}")
def get_input_files(self):
files = [f for f in os.listdir(self.input_dir) if f.endswith('.txt')]
if not files:
logging.warning("No .txt files found in input directory.")
return files
def process_file(self, filename):
input_path = os.path.join(self.input_dir, filename)
output_path = os.path.join(self.output_dir, f"processed_{filename}")
with open(input_path, 'r') as infile, open(output_path, 'w') as outfile:
for line in infile:
processed_line = line.upper() # Simple processing: convert to uppercase
outfile.write(processed_line)
logging.info(f"Processed file: {filename}")
# Example usage
processor = RobustFileProcessor("input_files", "output_files")
processor.process_files()
This example embodies Hamilton’s principles by implementing thorough error handling, logging, and considering the system as a whole, including input/output operations and file management.
Conclusion: Synthesizing Historical Approaches in Modern Coding
As we’ve explored the approaches of these legendary programmers, it becomes clear that each brings unique strengths to the problem-solving process:
- Ada Lovelace’s analytical and visionary thinking
- Alan Turing’s ability to break down complex problems
- Grace Hopper’s focus on simplification and user-friendly design
- Linus Torvalds’ emphasis on modularity and open collaboration
- Margaret Hamilton’s rigorous systems thinking and error prevention
In our modern coding practices, we can benefit greatly by synthesizing these approaches. Here’s how we might combine these historical perspectives in tackling a coding challenge:
- Start with Lovelace’s analytical approach, thoroughly understanding the problem and its broader implications.
- Use Turing’s method to break the problem down into manageable components.
- Apply Hopper’s principles to simplify the solution and make it user-friendly.
- Implement Torvalds’ modularity to create flexible, extensible code.
- Incorporate Hamilton’s rigorous error handling and system-wide thinking to ensure reliability.
By channeling these diverse perspectives, we can approach coding challenges with a rich toolkit of problem-solving strategies. This not only helps in creating more robust and innovative solutions but also connects us to the rich history of computer science, reminding us that we stand on the shoulders of giants.
As you face your next coding challenge, consider: “How would Ada analyze this? How might Turing break it down? What would Grace simplify? How could Linus make it modular? And how would Margaret ensure its reliability?” By doing so, you’ll not only honor the legacy of these programming pioneers but also elevate your own problem-solving skills to new heights.
Remember, the goal isn’t to perfectly mimic these historical figures, but to draw inspiration from their unique approaches and adapt them to modern challenges. In doing so, we continue the tradition of innovation and problem-solving that has defined the field of computer science since its inception.