Code Quality in Coding Interviews: The FizzBuzz Test and Beyond
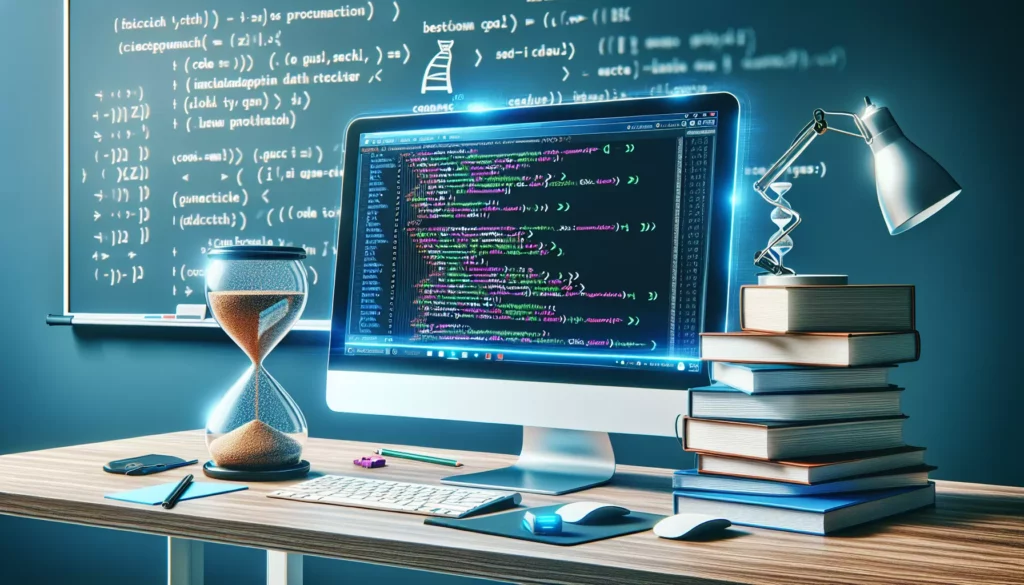
In the ever-evolving landscape of software development, the ability to write clean, efficient, and maintainable code is paramount. This skill becomes especially crucial during coding interviews, where candidates are often asked to demonstrate their programming prowess under pressure. One such test that has gained notoriety over the years is the FizzBuzz challenge. In this comprehensive guide, we’ll explore the FizzBuzz problem, its significance in coding interviews, and how it relates to broader concepts of code quality and best practices in software development.
The Origin of FizzBuzz in Coding Interviews
The story begins about a decade ago when a programmer named Imran shared his observations about hiring practices in the tech industry. He noticed a concerning trend: many candidates with impressive computer science degrees struggled to apply their theoretical knowledge to practical coding tasks. This gap between academic understanding and real-world application prompted Imran to propose a simple yet effective test – the FizzBuzz challenge.
What is FizzBuzz?
FizzBuzz is a children’s game that has been adapted into a programming task. The rules are straightforward:
- Count from 1 to 100
- For multiples of 3, print “Fizz” instead of the number
- For multiples of 5, print “Buzz” instead of the number
- For numbers that are multiples of both 3 and 5, print “FizzBuzz”
While the game itself is simple, translating these rules into code reveals a lot about a programmer’s approach to problem-solving and their coding style.
The Significance of FizzBuzz in Coding Interviews
The FizzBuzz test serves several purposes in a coding interview:
- Basic Coding Ability: It verifies that the candidate can write a functioning program that meets specific requirements.
- Problem-Solving Skills: The task requires breaking down a verbal description into logical steps and implementing them in code.
- Code Organization: How a candidate structures their solution can reveal their approach to writing clean and maintainable code.
- Handling Edge Cases: The requirement to handle multiples of both 3 and 5 tests the candidate’s ability to consider and implement special cases.
- Efficiency: While not the primary focus, more experienced developers might optimize their solution for better performance.
Implementing FizzBuzz: A Step-by-Step Approach
Let’s walk through the process of implementing FizzBuzz, starting with a basic solution and then refining it for better code quality. We’ll use JavaScript for our examples, but the principles apply to any programming language.
Basic Implementation
Here’s a straightforward implementation of FizzBuzz:
for (let i = 1; i <= 100; i++) {
if (i % 3 === 0 && i % 5 === 0) {
console.log("FizzBuzz");
} else if (i % 3 === 0) {
console.log("Fizz");
} else if (i % 5 === 0) {
console.log("Buzz");
} else {
console.log(i);
}
}
This solution works, but it has some drawbacks:
- It repeats the modulo operations, which could be inefficient for larger ranges.
- The logic for “FizzBuzz” is separate from “Fizz” and “Buzz”, making it harder to extend.
- It’s not easily reusable or configurable.
Improved Implementation
Let’s refine our solution to address these issues:
function fizzBuzz(n) {
for (let i = 1; i <= n; i++) {
let output = "";
if (i % 3 === 0) output += "Fizz";
if (i % 5 === 0) output += "Buzz";
console.log(output || i);
}
}
fizzBuzz(100);
This improved version:
- Uses a function for better reusability and testability
- Builds the output string incrementally, making it easier to extend
- Reduces repetition of modulo operations
- Uses a parameter for the range, making it more flexible
Beyond FizzBuzz: Code Quality in the Real World
While FizzBuzz is a useful starting point, real-world coding challenges require a deeper understanding of code quality principles. Let’s explore some key aspects of writing high-quality code that interviewers often look for:
1. Readability and Maintainability
Clear, self-documenting code is crucial in professional settings. This includes:
- Meaningful variable and function names
- Consistent formatting and indentation
- Appropriate comments for complex logic
- Breaking down complex functions into smaller, focused ones
2. Scalability and Extensibility
Code should be designed to accommodate future changes and growth:
- Use of design patterns where appropriate
- Modular architecture that separates concerns
- Configurable parameters instead of hard-coded values
3. Error Handling and Edge Cases
Robust code anticipates and handles potential issues:
- Input validation and sanitization
- Proper error handling and logging
- Consideration of boundary conditions and edge cases
4. Performance and Optimization
Efficient code is crucial, especially at scale:
- Appropriate data structures and algorithms
- Minimizing unnecessary computations or memory usage
- Understanding and optimizing for time and space complexity
5. Testing and Debugging
Quality code includes provisions for verification:
- Unit tests for individual components
- Integration tests for system interactions
- Debugging tools and techniques
Advanced FizzBuzz: Demonstrating Higher-Level Skills
To showcase more advanced programming concepts, let’s create a more sophisticated version of FizzBuzz that incorporates some of the principles we’ve discussed:
class FizzBuzzGame {
constructor(rules = [
{ divisor: 3, word: "Fizz" },
{ divisor: 5, word: "Buzz" }
]) {
this.rules = rules;
}
generate(start, end) {
return Array.from({ length: end - start + 1 }, (_, i) => this.processNumber(i + start));
}
processNumber(num) {
const result = this.rules
.filter(rule => num % rule.divisor === 0)
.map(rule => rule.word)
.join("");
return result || num.toString();
}
}
// Usage
const game = new FizzBuzzGame();
console.log(game.generate(1, 100));
// Extended usage with custom rules
const customGame = new FizzBuzzGame([
{ divisor: 3, word: "Fizz" },
{ divisor: 5, word: "Buzz" },
{ divisor: 7, word: "Bazz" }
]);
console.log(customGame.generate(1, 105));
This advanced implementation demonstrates several important concepts:
- Object-Oriented Design: The FizzBuzzGame class encapsulates the game logic.
- Flexibility: The constructor allows for custom rules, making the game easily extensible.
- Functional Programming: The use of map and filter showcases a more declarative style of programming.
- Separation of Concerns: The generate and processNumber methods have distinct responsibilities.
- Reusability: The class can be instantiated with different rules for various use cases.
Preparing for Coding Interviews: Beyond FizzBuzz
While FizzBuzz is a good starting point, preparing for coding interviews requires a much broader skill set. Here are some key areas to focus on:
1. Data Structures and Algorithms
Understanding fundamental data structures (arrays, linked lists, trees, graphs) and algorithms (sorting, searching, dynamic programming) is crucial. Platforms like AlgoCademy offer structured learning paths and practice problems to master these concepts.
2. Problem-Solving Techniques
Develop strategies for breaking down complex problems, identifying patterns, and approaching solutions systematically. Practice explaining your thought process out loud, as this is a key component of many technical interviews.
3. System Design
For more senior positions, understanding how to design scalable systems is important. This includes knowledge of distributed systems, database design, and architectural patterns.
4. Language Proficiency
While many concepts are language-agnostic, having deep knowledge of at least one programming language is crucial. Be familiar with language-specific features, standard libraries, and best practices.
5. Soft Skills
Communication, collaboration, and problem-solving under pressure are all important skills that interviewers assess. Practice explaining your code and decisions clearly and concisely.
Leveraging Tools and Resources
To enhance your coding skills and prepare for interviews, consider using the following resources:
- Online Platforms: Websites like AlgoCademy, LeetCode, and HackerRank offer a wide range of coding challenges and interview preparation materials.
- Interactive Tutorials: Many platforms provide step-by-step guidance for solving algorithmic problems, often with AI-powered assistance to help you learn at your own pace.
- Code Review Tools: Familiarize yourself with tools like GitHub, GitLab, or Bitbucket for version control and code review processes.
- IDEs and Debuggers: Proficiency with development environments and debugging tools can significantly improve your coding efficiency and problem-solving abilities.
Conclusion: The Path to Coding Excellence
The journey from tackling FizzBuzz to excelling in complex coding interviews is one of continuous learning and practice. While mastering the fundamentals is crucial, true excellence in software development comes from a combination of technical skills, problem-solving abilities, and a commitment to writing clean, efficient, and maintainable code.
Remember that coding interviews, much like the FizzBuzz test, are designed not just to assess your ability to solve a specific problem, but to evaluate your overall approach to software development. By focusing on code quality, continuously expanding your knowledge, and practicing regularly, you’ll be well-prepared to tackle any coding challenge that comes your way.
As you progress in your coding journey, platforms like AlgoCademy can provide structured learning paths, interactive coding exercises, and AI-powered assistance to help you build the skills needed to excel in technical interviews and beyond. Whether you’re a beginner looking to master the basics or an experienced developer preparing for interviews at top tech companies, remember that the key to success lies in consistent practice, a willingness to learn, and a commitment to writing high-quality code.