Cloud-Native Development Practices: Building Applications Optimized for Cloud Environments
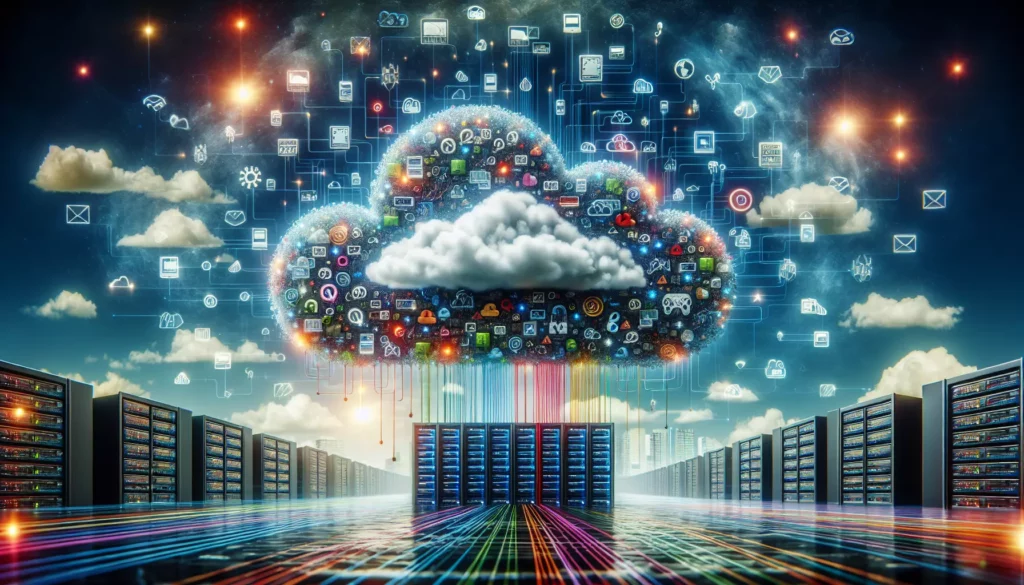
In today’s rapidly evolving technological landscape, cloud computing has become an integral part of modern software development. As organizations increasingly migrate their applications and infrastructure to the cloud, it’s crucial for developers to understand and adopt cloud-native development practices. These practices enable the creation of applications that are specifically designed to leverage the full potential of cloud environments, resulting in improved scalability, reliability, and efficiency. In this comprehensive guide, we’ll explore the world of cloud-native development, its key principles, and how you can implement these practices to build optimized applications for the cloud.
What is Cloud-Native Development?
Cloud-native development refers to the approach of building and running applications that fully exploit the advantages of the cloud computing model. These applications are designed from the ground up to run in cloud environments, taking advantage of features such as elasticity, scalability, and distributed computing. Cloud-native applications are typically built using microservices architecture, containerization, and DevOps practices, allowing for faster development cycles, easier maintenance, and improved resilience.
Key Principles of Cloud-Native Development
To effectively build cloud-native applications, it’s essential to understand and adhere to the following key principles:
1. Microservices Architecture
Microservices architecture is a fundamental principle of cloud-native development. It involves breaking down applications into smaller, loosely coupled services that can be developed, deployed, and scaled independently. Each microservice focuses on a specific business capability and communicates with other services through well-defined APIs.
Benefits of microservices architecture include:
- Improved scalability and flexibility
- Easier maintenance and updates
- Enhanced fault isolation
- Support for polyglot development (using different programming languages and technologies for different services)
2. Containerization
Containerization is another crucial aspect of cloud-native development. Containers provide a lightweight, portable, and consistent environment for running applications across different cloud platforms and environments. Docker is the most popular containerization platform, but alternatives like containerd and CRI-O are also widely used.
Key advantages of containerization include:
- Consistency across development, testing, and production environments
- Improved resource utilization
- Faster deployment and scaling
- Simplified dependency management
3. DevOps and Continuous Integration/Continuous Deployment (CI/CD)
Cloud-native development embraces DevOps practices and CI/CD pipelines to streamline the development, testing, and deployment processes. This approach enables faster iterations, automated testing, and seamless deployments, resulting in shorter time-to-market and improved software quality.
Key aspects of DevOps and CI/CD in cloud-native development include:
- Automated build and test processes
- Infrastructure as Code (IaC)
- Continuous monitoring and logging
- Automated deployment and rollback strategies
4. Scalability and Elasticity
Cloud-native applications are designed to scale horizontally, allowing them to handle varying workloads efficiently. This scalability is achieved through auto-scaling capabilities provided by cloud platforms, enabling applications to automatically adjust resources based on demand.
Key considerations for scalability and elasticity include:
- Stateless services
- Distributed caching
- Load balancing
- Asynchronous communication patterns
5. Resilience and Fault Tolerance
Cloud-native applications are built to be resilient and fault-tolerant, capable of handling failures gracefully. This involves implementing strategies such as circuit breakers, retries, and fallback mechanisms to ensure that the application remains available and functional even in the face of network issues or service failures.
6. Observability
Observability is crucial for understanding the behavior and performance of cloud-native applications. This involves implementing comprehensive logging, monitoring, and tracing solutions to gain insights into application health, performance metrics, and potential issues.
Implementing Cloud-Native Development Practices
Now that we’ve covered the key principles, let’s explore how to implement cloud-native development practices in your projects:
1. Choose the Right Cloud Platform
Selecting the appropriate cloud platform is crucial for successful cloud-native development. Popular options include:
- Amazon Web Services (AWS)
- Microsoft Azure
- Google Cloud Platform (GCP)
- IBM Cloud
- DigitalOcean
Each platform offers a range of services and tools to support cloud-native development. Consider factors such as pricing, available services, geographical coverage, and your team’s expertise when making a decision.
2. Adopt Microservices Architecture
To implement microservices architecture:
- Identify bounded contexts within your application
- Design services around business capabilities
- Define clear APIs for inter-service communication
- Implement service discovery and load balancing
- Use event-driven architectures for asynchronous communication
Here’s a simple example of a microservice using Node.js and Express:
<!-- User Service -->
const express = require('express');
const app = express();
const port = 3000;
app.get('/users/:id', (req, res) => {
const userId = req.params.id;
// Fetch user data from database
const user = { id: userId, name: 'John Doe', email: 'john@example.com' };
res.json(user);
});
app.listen(port, () => {
console.log(`User service listening at http://localhost:${port}`);
});
3. Containerize Your Applications
To containerize your applications:
- Install Docker on your development machine
- Create a Dockerfile for each service
- Build and test your Docker images locally
- Use Docker Compose for multi-container applications
- Push your images to a container registry (e.g., Docker Hub, AWS ECR, or Google Container Registry)
Here’s an example Dockerfile for a Node.js application:
# Use an official Node.js runtime as the base image
FROM node:14
# Set the working directory in the container
WORKDIR /usr/src/app
# Copy package.json and package-lock.json to the working directory
COPY package*.json ./
# Install application dependencies
RUN npm install
# Copy the application code to the working directory
COPY . .
# Expose the port the app runs on
EXPOSE 3000
# Define the command to run the application
CMD ["node", "app.js"]
4. Implement CI/CD Pipelines
To set up CI/CD pipelines:
- Choose a CI/CD tool (e.g., Jenkins, GitLab CI, CircleCI, or AWS CodePipeline)
- Define your build, test, and deployment stages
- Automate unit tests, integration tests, and security scans
- Implement infrastructure as code using tools like Terraform or AWS CloudFormation
- Set up automated deployments to your target environments
Here’s an example GitLab CI/CD configuration file:
stages:
- build
- test
- deploy
build:
stage: build
image: docker:latest
services:
- docker:dind
script:
- docker build -t myapp:$CI_COMMIT_SHA .
- docker push myregistry.com/myapp:$CI_COMMIT_SHA
test:
stage: test
image: node:14
script:
- npm install
- npm test
deploy:
stage: deploy
image: alpine
script:
- apk add --no-cache curl
- curl -LO "https://storage.googleapis.com/kubernetes-release/release/$(curl -s https://storage.googleapis.com/kubernetes-release/release/stable.txt)/bin/linux/amd64/kubectl"
- chmod +x ./kubectl
- ./kubectl set image deployment/myapp myapp=myregistry.com/myapp:$CI_COMMIT_SHA
only:
- master
5. Design for Scalability and Elasticity
To ensure your applications are scalable and elastic:
- Use stateless services whenever possible
- Implement caching strategies (e.g., Redis or Memcached)
- Utilize message queues for asynchronous processing (e.g., RabbitMQ or Apache Kafka)
- Implement auto-scaling policies based on metrics like CPU usage or request rate
- Use load balancers to distribute traffic across multiple instances
Here’s an example of setting up auto-scaling using AWS CloudFormation:
Resources:
AutoScalingGroup:
Type: AWS::AutoScaling::AutoScalingGroup
Properties:
LaunchConfigurationName: !Ref LaunchConfig
MinSize: '1'
MaxSize: '5'
DesiredCapacity: '2'
VPCZoneIdentifier:
- !Ref Subnet1
- !Ref Subnet2
TargetGroupARNs:
- !Ref ALBTargetGroup
ScalingPolicy:
Type: AWS::AutoScaling::ScalingPolicy
Properties:
AutoScalingGroupName: !Ref AutoScalingGroup
PolicyType: TargetTrackingScaling
TargetTrackingConfiguration:
PredefinedMetricSpecification:
PredefinedMetricType: ASGAverageCPUUtilization
TargetValue: 70.0
6. Implement Resilience Patterns
To improve the resilience of your cloud-native applications:
- Implement circuit breakers to prevent cascading failures
- Use retry mechanisms with exponential backoff for transient failures
- Implement fallback mechanisms for graceful degradation
- Use health checks and liveness probes
- Implement proper error handling and logging
Here’s an example of implementing a circuit breaker using the Hystrix library in Java:
import com.netflix.hystrix.HystrixCommand;
import com.netflix.hystrix.HystrixCommandGroupKey;
public class ExampleCommand extends HystrixCommand<String> {
private final String name;
public ExampleCommand(String name) {
super(HystrixCommandGroupKey.Factory.asKey("ExampleGroup"));
this.name = name;
}
@Override
protected String run() {
// This is where you would make an external service call
return "Hello " + name + "!";
}
@Override
protected String getFallback() {
return "Hello Guest!";
}
}
// Usage
String result = new ExampleCommand("World").execute();
7. Implement Observability
To implement observability in your cloud-native applications:
- Use structured logging with tools like ELK stack (Elasticsearch, Logstash, Kibana) or Splunk
- Implement distributed tracing using solutions like Jaeger or Zipkin
- Set up metrics collection and visualization using Prometheus and Grafana
- Use cloud-native monitoring solutions provided by your cloud platform (e.g., AWS CloudWatch or Google Cloud Monitoring)
- Implement alerting and notification systems
Here’s an example of setting up Prometheus metrics in a Node.js application:
const express = require('express');
const promClient = require('prom-client');
const app = express();
const collectDefaultMetrics = promClient.collectDefaultMetrics;
collectDefaultMetrics({ timeout: 5000 });
const httpRequestDurationMicroseconds = new promClient.Histogram({
name: 'http_request_duration_seconds',
help: 'Duration of HTTP requests in microseconds',
labelNames: ['method', 'route', 'status_code'],
buckets: [0.1, 0.5, 1, 5]
});
app.use((req, res, next) => {
const start = process.hrtime();
res.on('finish', () => {
const duration = process.hrtime(start);
const durationInSeconds = duration[0] + duration[1] / 1e9;
httpRequestDurationMicroseconds
.labels(req.method, req.route.path, res.statusCode)
.observe(durationInSeconds);
});
next();
});
app.get('/metrics', async (req, res) => {
res.set('Content-Type', promClient.register.contentType);
res.end(await promClient.register.metrics());
});
app.listen(3000, () => {
console.log('Server listening on port 3000');
});
Best Practices for Cloud-Native Development
To ensure success in your cloud-native development journey, consider the following best practices:
- Embrace Infrastructure as Code (IaC): Use tools like Terraform, AWS CloudFormation, or Pulumi to manage your infrastructure declaratively.
- Implement security at every layer: Follow the principle of least privilege, use encryption for data at rest and in transit, and implement proper authentication and authorization mechanisms.
- Design for failure: Assume that components will fail and design your applications to be resilient and self-healing.
- Use cloud-native storage solutions: Leverage cloud-native storage services like Amazon S3, Azure Blob Storage, or Google Cloud Storage for scalable and durable data storage.
- Implement proper logging and monitoring: Ensure that your applications generate meaningful logs and metrics to facilitate troubleshooting and performance optimization.
- Embrace serverless computing: Where appropriate, use serverless platforms like AWS Lambda, Azure Functions, or Google Cloud Functions to reduce operational overhead and improve scalability.
- Optimize for cost: Take advantage of cloud pricing models, use auto-scaling to match resources with demand, and implement proper resource tagging for cost allocation.
- Continuously learn and adapt: Stay up-to-date with the latest cloud-native technologies and best practices, and be willing to refactor and improve your applications over time.
Conclusion
Cloud-native development practices offer numerous benefits for building modern, scalable, and resilient applications. By embracing microservices architecture, containerization, DevOps practices, and other cloud-native principles, developers can create applications that fully leverage the power of cloud computing.
As you embark on your cloud-native development journey, remember that it’s an iterative process. Start small, learn from your experiences, and gradually adopt more advanced practices as your team and applications mature. With the right approach and mindset, you’ll be well-equipped to build applications that thrive in cloud environments and deliver value to your users efficiently and effectively.
By mastering cloud-native development practices, you’ll not only enhance your skills as a developer but also position yourself at the forefront of modern software engineering. As cloud computing continues to evolve and shape the technology landscape, the ability to build cloud-native applications will become an increasingly valuable skill in the job market.
So, dive in, experiment with these practices, and start building the next generation of cloud-optimized applications. The cloud is your canvas, and with cloud-native development, you have the tools to create truly remarkable software solutions.