Choosing the right programming language for your interview
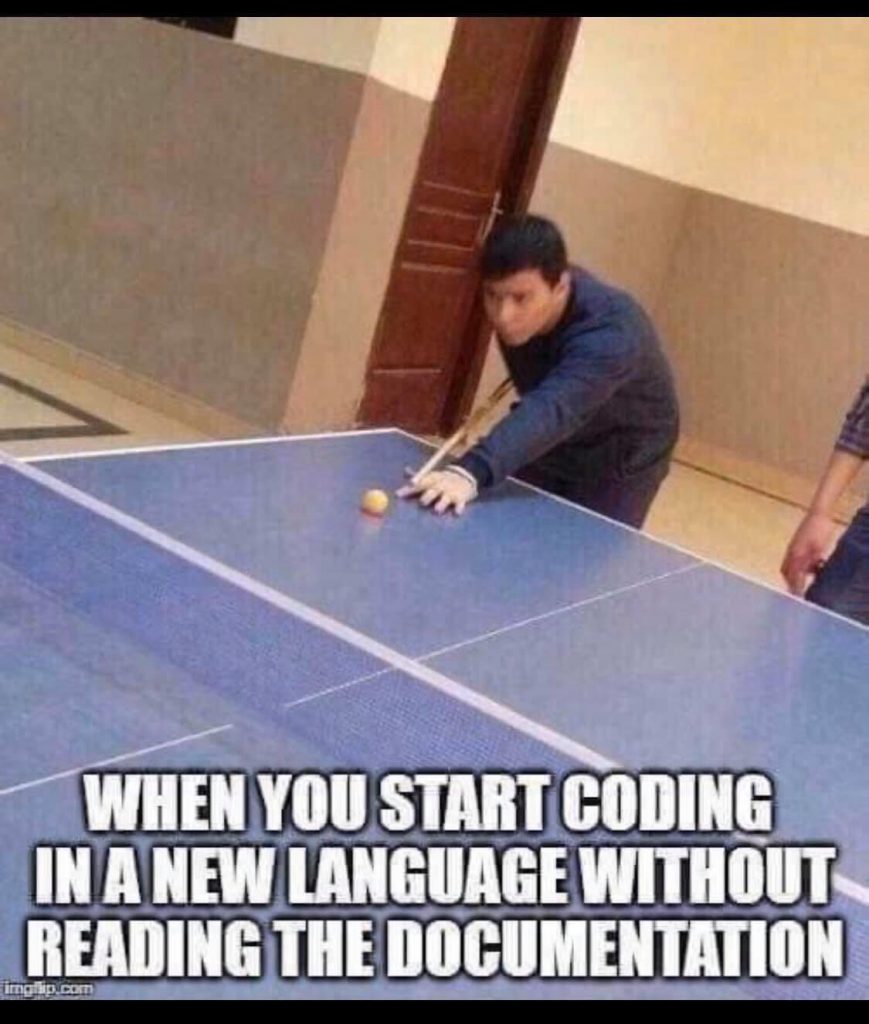
You recently started coding in Python or JavaScript and you realize it’s a way better choice for your upcoming coding interview. But is it really? Is it the best choice for you?
You can easily fall into the trap of thinking you know how to code in a new language, but you might not realize some fine details that ends up being a disaster for your coding interview.
Let’s take the following problem: You are given a string and you need to return the reverse string without using builtin functions. You quickly jump in Python and write the following:
def reverse(input):
result = ''
for char in input:
result = char + result
return result
Simple and efficient, isn’t it? Or is it? Your interviewer asks what is the time complexity of this? And you quickly answer that it’s linear time complexity because you iterate through each character once.
You see, this is where you failed because you didn’t know that strings are immutable in Python. What does this mean? Well, it means that \(char + result\) does not run in constant time, but rather in \(O(n)\) because it needs to copy the two strings into another one. The final time complexity ending up being \(O(n^2)\).
Let’s take another example. You are given a problem where you need to sort an array of integers as an initial step. You quickly jump and write the following JavaScript code:
nums.sort()
This surely looks right, no? Well, let’s say \(nums \) is \([1, 2, 11]\). After sorting you will notice that you end up with \([1, 11, 2]\). Not exactly what you would expect, is it?
Well, by default JavaScript considers your objects as strings and sorts them in alphabetical order, so now it’s obvious that 11 is less than 2 in alphabetical order! To make it work you need to use a comparator function that specifies how to compare two values:
nums.sort((a, b) => a - b)
One last example, let’s say you have an array and you want to print pairs containing the index and the value, but the index needs to start at 1. So you start writing the following JavaScript code:
for (let i in nums) {
let index = i + 1
console.log(index, ': ', nums[i])
}
That should do the trick, right? Well, let’s see for \(nums=[1, 2, 3]\) we get
01: 1
11: 2
21: 3
What happened? Well, the \(in\) operator is not meant to be used for arrays, but for objects. While it will give you the indices 0, 1 and 2, they will be strings, so when you add 1, you will actually concatenate the two strings.
What I’m trying to say is that you should stick with the programming language you have deep knowledge about and feel comfortable writing code without the use of an IDE / autocomplete / syntax highlighting, possibly even on a whiteboard.