Burst Balloons: A Deep Dive into Dynamic Programming
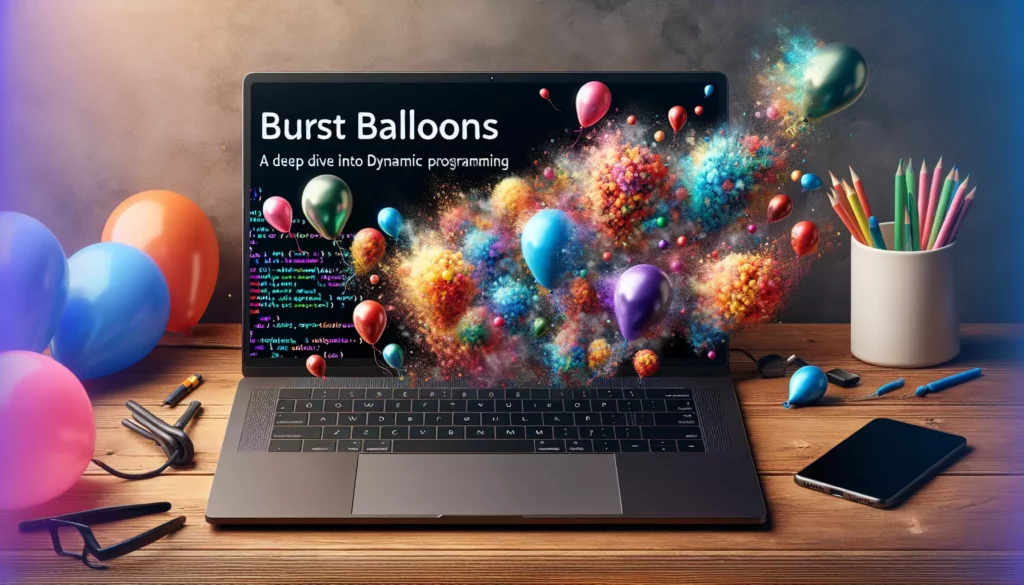
Welcome to another exciting AlgoCademy tutorial! Today, we’re going to tackle a fascinating problem that’s often asked in technical interviews, especially at top tech companies. The problem is called “Burst Balloons,” and it’s an excellent example of how dynamic programming can be used to solve complex optimization problems. By the end of this tutorial, you’ll have a deep understanding of the problem, its solution, and the thought process behind developing an efficient algorithm.
Problem Statement
Let’s start by clearly stating the problem:
You are given n balloons, indexed from 0 to n-1. Each balloon is painted with a number on it represented by an array nums. You are asked to burst all the balloons.
If you burst the ith balloon, you will get nums[i-1] * nums[i] * nums[i+1] coins. If i-1 or i+1 goes out of bounds of the array, then treat it as if there is a balloon with a 1 painted on it.
Return the maximum coins you can collect by bursting the balloons wisely.
Understanding the Problem
Before we dive into the solution, let’s break down the problem and understand its key components:
- We have an array of integers representing the numbers painted on each balloon.
- When we burst a balloon, we get coins based on the product of the current balloon’s number and its adjacent balloons’ numbers.
- After bursting a balloon, the balloons to its left and right become adjacent.
- We need to find the maximum number of coins we can collect by bursting all balloons.
The tricky part of this problem is that the order in which we burst the balloons matters. Each decision affects future possibilities, making this a perfect candidate for dynamic programming.
Naive Approach: Recursion with Backtracking
Before we get to the optimal solution, let’s consider a naive approach using recursion and backtracking. This will help us understand the problem better and see why we need a more efficient solution.
The idea is to try bursting each remaining balloon, recursively solve the subproblem for the remaining balloons, and choose the maximum result. Here’s how we could implement this:
class Solution {
public:
int maxCoins(vector<int>& nums) {
return burstBalloons(nums, 0, nums.size() - 1);
}
private:
int burstBalloons(vector<int>& nums, int left, int right) {
if (left > right) return 0;
int maxCoins = 0;
for (int i = left; i <= right; i++) {
int coins = getCoins(nums, left - 1, i, right + 1);
int leftCoins = burstBalloons(nums, left, i - 1);
int rightCoins = burstBalloons(nums, i + 1, right);
maxCoins = max(maxCoins, coins + leftCoins + rightCoins);
}
return maxCoins;
}
int getCoins(vector<int>& nums, int left, int i, int right) {
int leftValue = (left < 0) ? 1 : nums[left];
int rightValue = (right >= nums.size()) ? 1 : nums[right];
return leftValue * nums[i] * rightValue;
}
};
While this solution works, it has a time complexity of O(n!), which is extremely inefficient for large inputs. The problem with this approach is that we’re repeatedly solving the same subproblems, leading to redundant computations.
Optimal Approach: Dynamic Programming
To solve this problem efficiently, we need to use dynamic programming. The key insight is to approach the problem in reverse: instead of considering which balloon to burst first, we consider which balloon to burst last.
Here’s the step-by-step thought process:
- Add two dummy balloons with value 1 at the beginning and end of the array. This simplifies our calculations for edge cases.
- Define dp[i][j] as the maximum coins we can get by bursting all balloons between i and j, exclusive.
- For each possible last balloon k between i and j, calculate the coins we get from bursting it last, plus the maximum coins from the left and right subarrays.
- The final answer will be in dp[0][n+1], where n is the number of balloons.
Let’s implement this solution:
class Solution {
public:
int maxCoins(vector<int>& nums) {
int n = nums.size();
nums.insert(nums.begin(), 1);
nums.push_back(1);
vector<vector<int>> dp(n + 2, vector<int>(n + 2, 0));
for (int len = 1; len <= n; len++) {
for (int i = 1; i <= n - len + 1; i++) {
int j = i + len - 1;
for (int k = i; k <= j; k++) {
dp[i][j] = max(dp[i][j],
dp[i][k-1] + nums[i-1] * nums[k] * nums[j+1] + dp[k+1][j]);
}
}
}
return dp[1][n];
}
};
Explanation of the Dynamic Programming Solution
Let’s break down the solution to understand it better:
- Initialization: We add dummy balloons with value 1 at the start and end of the array. This simplifies our calculations by eliminating the need for special edge case handling.
- DP Table: We create a 2D DP table where dp[i][j] represents the maximum coins we can get by bursting all balloons between i and j (exclusive).
- Iteration: We iterate over all possible subarray lengths (len) and starting positions (i). For each subarray, we consider all possible last balloons to burst (k).
- DP Transition: For each k, we calculate:
- Coins from bursting k last: nums[i-1] * nums[k] * nums[j+1]
- Maximum coins from left subarray: dp[i][k-1]
- Maximum coins from right subarray: dp[k+1][j]
We take the maximum of all these calculations to fill dp[i][j].
- Final Answer: The maximum coins for the entire array is stored in dp[1][n], where n is the original number of balloons.
Time and Space Complexity Analysis
Time Complexity: O(n^3), where n is the number of balloons. We have three nested loops: two for iterating over all subarrays, and one for considering all possible last balloons to burst.
Space Complexity: O(n^2) for the DP table.
While O(n^3) might seem high, this is a significant improvement over the naive O(n!) solution, making it feasible for the constraints typically given in coding interviews (usually n ≤ 500).
Optimizations and Variations
While the solution we’ve discussed is the standard approach to this problem, there are a few optimizations and variations worth mentioning:
1. Bottom-Up vs Top-Down DP
The solution we’ve implemented is a bottom-up DP approach. It’s also possible to solve this problem using a top-down DP approach with memoization. Here’s how that might look:
class Solution {
public:
vector<vector<int>> memo;
vector<int> nums;
int maxCoins(vector<int>& nums) {
int n = nums.size();
this->nums = vector<int>(n+2, 1);
for(int i=0; i<n; i++) {
this->nums[i+1] = nums[i];
}
memo = vector<vector<int>>(n+2, vector<int>(n+2, -1));
return dp(0, n+1);
}
int dp(int left, int right) {
if(left + 1 == right) return 0;
if(memo[left][right] != -1) return memo[left][right];
int ans = 0;
for(int i = left+1; i < right; i++) {
ans = max(ans, nums[left] * nums[i] * nums[right] + dp(left, i) + dp(i, right));
}
memo[left][right] = ans;
return ans;
}
};
This approach can be more intuitive for some people and can potentially be faster in practice due to its lazy evaluation nature, especially if not all subproblems need to be solved.
2. Space Optimization
While we can’t reduce the time complexity below O(n^3) for this problem, we can optimize the space usage. Notice that when we’re filling dp[i][j], we only need the values from the current row and the rows below it. This means we can reduce our space complexity to O(n) by only keeping two rows of the DP table at a time.
3. Divide and Conquer with Memoization
Another interesting approach to this problem is to use a divide and conquer strategy with memoization. The idea is to choose the last balloon to burst, divide the problem into left and right subarrays, and recursively solve these subarrays. This approach can be more intuitive and easier to implement for some people.
Related Problems and Extensions
The “Burst Balloons” problem is a great introduction to a class of dynamic programming problems that involve optimal substructure with non-obvious subproblems. Here are some related problems that you might find interesting:
- Matrix Chain Multiplication: This classic DP problem involves finding the most efficient way to multiply a chain of matrices. It has a similar structure to Burst Balloons, where you’re choosing the order of operations to optimize a result.
- Optimal Binary Search Tree: This problem involves constructing a binary search tree with the minimum expected search time, given the frequencies of searches for each key. It uses a similar DP approach where you consider different roots for subtrees.
- Boolean Parenthesization: Given a boolean expression with AND, OR, and XOR operators, find the number of ways to parenthesize it so that it evaluates to true. This problem also involves considering different ways to divide the expression and combine results.
Practical Applications
While bursting balloons might not seem like a practical problem at first glance, the underlying concepts and problem-solving techniques have several real-world applications:
- Resource Allocation in Cloud Computing: Optimizing the allocation and deallocation of virtual machines or containers in a cloud environment to maximize resource utilization while minimizing cost.
- Financial Modeling: Determining the optimal order to execute a series of financial transactions to maximize profit, considering that each transaction can affect the market conditions for subsequent transactions.
- Game Theory and Strategy: In turn-based strategy games, determining the optimal sequence of moves to maximize score or minimize opponent’s options.
- Operations Research: Optimizing production schedules in manufacturing, where the order of operations can significantly affect overall efficiency and output.
Conclusion
The “Burst Balloons” problem is a fantastic example of how dynamic programming can be used to solve complex optimization problems. It teaches us several important lessons:
- The importance of carefully defining our subproblems in DP. Sometimes, the most intuitive subproblem definition (like “which balloon to burst first”) doesn’t lead to an efficient solution.
- The power of thinking in reverse. By considering which balloon to burst last instead of first, we were able to develop an efficient DP solution.
- The value of adding “dummy” elements to simplify edge cases. This is a common technique in DP and can often make our code cleaner and easier to reason about.
- The process of improving from a naive recursive solution to an optimized DP solution. This progression is common in algorithm development and is an important skill to cultivate.
As you continue your journey in algorithmic problem solving, remember that problems like “Burst Balloons” are not just academic exercises. They represent a class of optimization problems that have real-world applications in various fields. The skills you develop in solving these problems – breaking down complex scenarios, identifying optimal substructures, and efficiently combining solutions to subproblems – are invaluable in many areas of computer science and beyond.
Keep practicing, stay curious, and happy coding!