Building Your First Simple Program: From Concept to Code
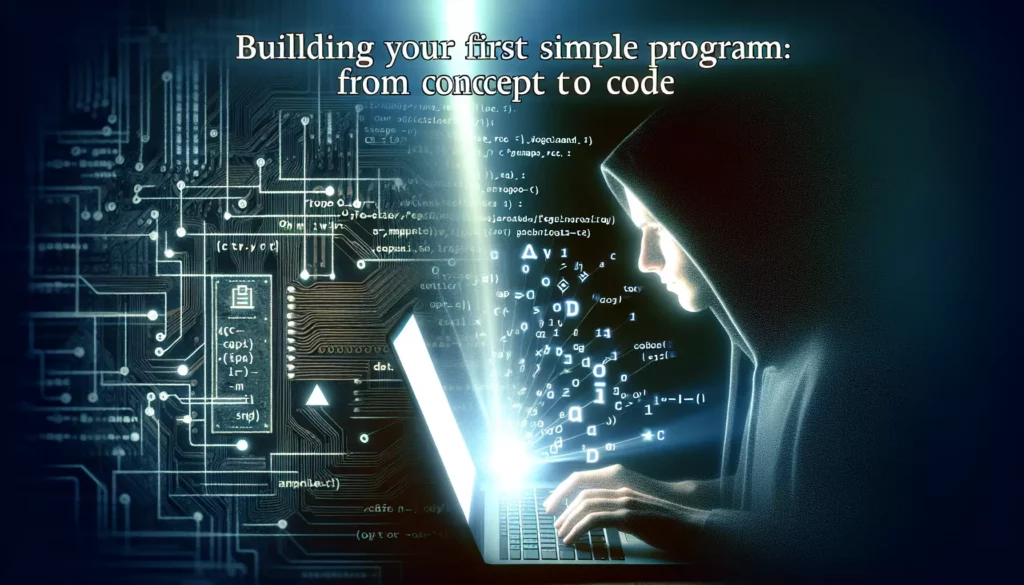
Welcome to the exciting world of programming! If you’re just starting your journey into coding, you’re in for an incredible adventure. In this comprehensive guide, we’ll walk you through the process of building your first simple program, from the initial concept to the final code. By the end of this article, you’ll have a solid understanding of the basic principles of programming and be ready to take on more complex challenges.
Understanding the Basics
Before we dive into creating our first program, let’s cover some fundamental concepts that will serve as the foundation for your coding journey.
What is Programming?
Programming is the process of creating a set of instructions that tell a computer how to perform a specific task. These instructions, written in a programming language, are called code. The code is then translated into a form that the computer can understand and execute.
Choosing a Programming Language
There are numerous programming languages to choose from, each with its own strengths and use cases. For beginners, popular choices include:
- Python: Known for its simplicity and readability
- JavaScript: Widely used for web development
- Java: Versatile and used in many enterprise applications
- C++: Powerful and commonly used in game development and system programming
For this tutorial, we’ll use Python due to its beginner-friendly syntax and versatility.
Setting Up Your Development Environment
Before we start coding, we need to set up our development environment. Here’s how to get started with Python:
- Visit the official Python website (python.org) and download the latest version for your operating system.
- Run the installer and follow the installation prompts. Make sure to check the box that says “Add Python to PATH” during installation.
- Once installed, you can open a terminal or command prompt and type “python” to enter the Python interactive shell.
- For a more feature-rich coding experience, consider installing an Integrated Development Environment (IDE) like PyCharm or Visual Studio Code.
Conceptualizing Your First Program
Now that we have our environment set up, let’s think about what our first program will do. A classic starting point for many programmers is the “Hello, World!” program. This simple program outputs the text “Hello, World!” to the screen.
While this is a great introduction, let’s take it a step further and create a program that interacts with the user. Our program will:
- Ask the user for their name
- Greet the user by name
- Ask the user for their age
- Calculate and display the year when the user will turn 100 years old
Writing the Code
Let’s break down our program into steps and write the code for each part:
Step 1: Asking for the User’s Name
We’ll use the input()
function to get information from the user:
name = input("What is your name? ")
Step 2: Greeting the User
We’ll use the print()
function to display output:
print("Hello, " + name + "! Nice to meet you.")
Step 3: Asking for the User’s Age
We’ll use input()
again, but this time we need to convert the input to an integer:
age = int(input("How old are you? "))
Step 4: Calculating and Displaying the Year
We’ll use some basic arithmetic and the current year to calculate when the user will turn 100:
import datetime
current_year = datetime.datetime.now().year
years_to_100 = 100 - age
turn_100_year = current_year + years_to_100
print("You will turn 100 years old in the year " + str(turn_100_year) + ".")
Putting It All Together
Now, let’s combine all these steps into our complete program:
import datetime
# Ask for the user's name
name = input("What is your name? ")
# Greet the user
print("Hello, " + name + "! Nice to meet you.")
# Ask for the user's age
age = int(input("How old are you? "))
# Calculate the year when the user will turn 100
current_year = datetime.datetime.now().year
years_to_100 = 100 - age
turn_100_year = current_year + years_to_100
# Display the result
print("You will turn 100 years old in the year " + str(turn_100_year) + ".")
Running Your Program
To run your program:
- Save the code in a file with a .py extension (e.g., my_first_program.py).
- Open a terminal or command prompt.
- Navigate to the directory where you saved the file.
- Type
python my_first_program.py
and press Enter.
You should see your program run, asking for input and providing output based on the user’s responses.
Understanding the Code
Let’s break down some key elements of our program:
Importing Modules
The line import datetime
allows us to use Python’s datetime module, which provides functions for working with dates and times.
Variables
We use variables like name
, age
, and turn_100_year
to store and manipulate data in our program.
Input and Output
The input()
function gets data from the user, while print()
displays information to the user.
Type Conversion
We use int()
to convert the age input from a string to an integer, allowing us to perform calculations with it.
String Concatenation
We combine strings using the +
operator to create meaningful messages for the user.
Expanding Your Program
Now that you have a working program, consider ways to expand and improve it:
- Add error handling to deal with invalid inputs (e.g., non-numeric age).
- Implement a loop to allow multiple users to use the program without restarting it.
- Add more calculations, such as determining the user’s zodiac sign based on their birth month and day.
Best Practices for Beginners
As you continue your programming journey, keep these best practices in mind:
- Comment your code: Add explanations to help others (and your future self) understand your code.
- Use meaningful variable names: Choose names that clearly describe what the variable represents.
- Keep your code organized: Use proper indentation and group related code together.
- Test your code frequently: Run your program often as you add new features to catch errors early.
- Learn to debug: Understanding how to find and fix errors is a crucial skill for any programmer.
Next Steps in Your Coding Journey
Congratulations on building your first program! This is just the beginning of your coding adventure. Here are some suggestions for what to explore next:
1. Learn More Python Basics
Dive deeper into Python by learning about:
- Data types (strings, integers, floats, lists, dictionaries)
- Control structures (if statements, for and while loops)
- Functions and modules
- File handling
2. Explore Object-Oriented Programming (OOP)
OOP is a fundamental concept in many programming languages. Learn about classes, objects, inheritance, and polymorphism.
3. Try Some Coding Challenges
Websites like HackerRank, LeetCode, and Project Euler offer coding challenges that can help you improve your problem-solving skills.
4. Build More Complex Projects
As you gain confidence, try building more complex projects like:
- A simple calculator
- A to-do list application
- A basic web scraper
- A simple game (like Tic-Tac-Toe or Hangman)
5. Learn Version Control
Git is an essential tool for programmers. Learn how to use Git and GitHub to manage your code and collaborate with others.
6. Explore Web Development
If you’re interested in creating websites, start learning HTML, CSS, and JavaScript. Then, explore web frameworks like Django (for Python) or React (for JavaScript).
7. Join Coding Communities
Engage with other learners and experienced programmers through:
- Online forums like Stack Overflow
- Local coding meetups
- Coding bootcamps or classes
- Open source projects on GitHub
Common Pitfalls for Beginners (and How to Avoid Them)
As you start your coding journey, be aware of these common mistakes and learn how to avoid them:
1. Trying to Learn Everything at Once
Pitfall: Feeling overwhelmed by the vast amount of programming concepts and languages.
Solution: Focus on mastering one language and its fundamental concepts before moving on. Build a strong foundation before expanding your knowledge.
2. Not Practicing Enough
Pitfall: Only reading about programming without hands-on practice.
Solution: Dedicate time to coding regularly. Try to implement what you learn in small projects or coding exercises.
3. Copy-Pasting Code Without Understanding
Pitfall: Using code snippets from the internet without understanding how they work.
Solution: Always try to understand the code you’re using. If you use a snippet, break it down and learn how each part functions.
4. Neglecting to Plan Before Coding
Pitfall: Jumping into coding without thinking through the problem and outlining a solution.
Solution: Spend time planning your approach. Write pseudocode or create a flowchart before starting to code.
5. Not Asking for Help
Pitfall: Struggling alone with a problem for too long without seeking assistance.
Solution: Don’t hesitate to ask for help from online communities, mentors, or peers. Learning to ask good questions is a valuable skill in programming.
Resources for Continued Learning
To support your ongoing learning, here are some valuable resources:
Online Courses and Tutorials
- Codecademy: Interactive coding courses for various languages
- Coursera: Offers university-level programming courses
- freeCodeCamp: Free, comprehensive web development curriculum
- Udacity: In-depth courses on programming and computer science
Books
- “Python Crash Course” by Eric Matthes
- “Clean Code: A Handbook of Agile Software Craftsmanship” by Robert C. Martin
- “Introduction to Algorithms” by Thomas H. Cormen et al.
YouTube Channels
- Corey Schafer: In-depth Python tutorials
- Traversy Media: Web development tutorials
- CS Dojo: Algorithm and data structure explanations
Coding Practice Platforms
- LeetCode: Programming challenges often used in technical interviews
- HackerRank: Coding challenges and competitions
- CodeWars: Improve your skills by training with others
Conclusion: Your Coding Journey Begins
Building your first program is an exciting milestone in your coding journey. Remember, every expert programmer started exactly where you are now. The key to success in programming is persistence, curiosity, and continuous learning.
As you progress, you’ll encounter challenges and moments of frustration, but don’t let these discourage you. Each problem you solve and each concept you master brings you one step closer to becoming a proficient programmer. Embrace the learning process, celebrate your successes (no matter how small), and don’t be afraid to make mistakes – they’re often the best teachers.
Keep coding, keep learning, and most importantly, enjoy the journey. The world of programming is vast and ever-evolving, offering endless opportunities for creativity, problem-solving, and innovation. Your first simple program is just the beginning of what could be an incredibly rewarding and impactful career or hobby.
Remember, the programming community is generally welcoming and supportive. Don’t hesitate to reach out for help, share your accomplishments, and even mentor others as you grow. Your unique perspective and experiences can contribute valuable insights to the field.
So, what’s next? Take the concepts you’ve learned here, expand on them, and start building more complex programs. Challenge yourself to solve real-world problems with code. Explore different areas of programming to find what truly excites you. And always keep that sense of wonder and excitement that you felt when you ran your first program.
Welcome to the world of coding – your adventure starts now!