Building Resilience: Bouncing Back from Coding Failures
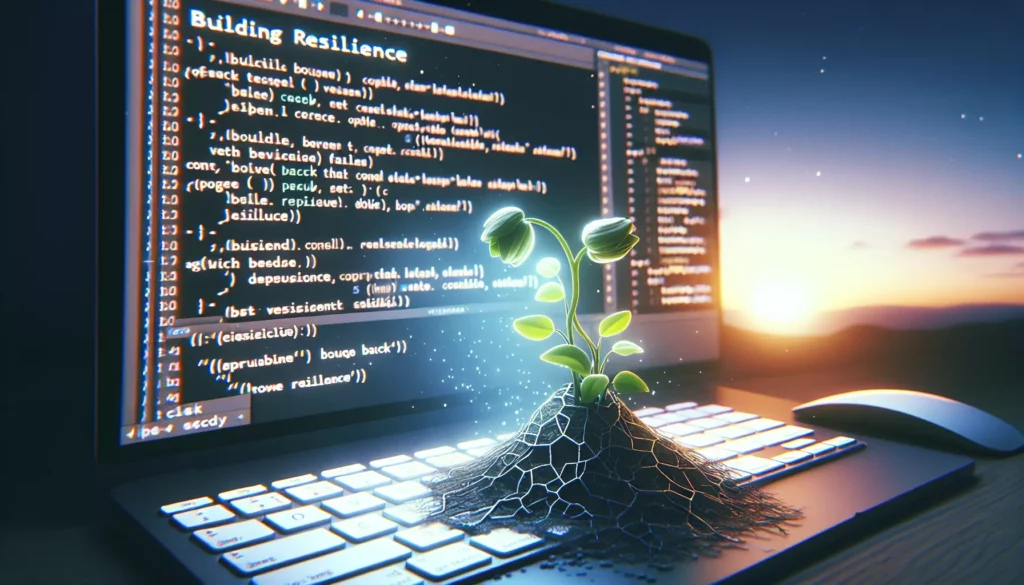
In the world of programming, failure is not just a possibility; it’s an inevitability. Every coder, from novice to expert, encounters bugs, errors, and seemingly insurmountable challenges. The key to success in this field isn’t avoiding failure—it’s learning how to bounce back from it. This article will explore the concept of resilience in coding, why it’s crucial for your growth as a programmer, and how you can develop this essential skill to overcome obstacles and thrive in your coding journey.
Understanding Resilience in Coding
Resilience in coding refers to the ability to persist in the face of challenges, learn from mistakes, and continually improve despite setbacks. It’s about maintaining a positive attitude and a growth mindset when confronted with difficult problems or when your code doesn’t work as expected.
For many beginners, the first encounter with a stubborn bug or a complex algorithm can be disheartening. It’s easy to feel overwhelmed and question your abilities. However, experienced programmers know that these moments are not just normal—they’re opportunities for growth and learning.
The Importance of Resilience in Programming
Developing resilience is crucial for several reasons:
- Problem-solving is at the core of coding: Programming is essentially about solving problems, and not all solutions come easily. Resilience helps you persist when faced with challenging issues.
- Technology is constantly evolving: The tech landscape changes rapidly, and programmers need to adapt continuously. Resilience helps you embrace change and new learning opportunities.
- Debugging is a regular part of the job: A significant portion of a programmer’s time is spent finding and fixing bugs. Resilience helps you maintain patience and a clear mind during these often frustrating tasks.
- Imposter syndrome is common: Many programmers, even experienced ones, sometimes feel like they don’t belong or aren’t skilled enough. Resilience helps combat these negative thoughts and boosts confidence.
Strategies for Building Coding Resilience
1. Embrace the Growth Mindset
Adopt a growth mindset, which is the belief that your abilities can be developed through dedication and hard work. Instead of viewing failures as reflections of your inherent capabilities, see them as opportunities to learn and improve.
Practice saying:
- “I haven’t figured it out yet” instead of “I can’t do this.”
- “This is challenging, but it’s helping me grow” instead of “This is too hard for me.”
2. Set Realistic Expectations
Understand that learning to code takes time and that everyone progresses at their own pace. Set realistic goals for yourself and celebrate small victories along the way.
For example:
- Aim to understand and implement one new concept each week.
- Celebrate when you successfully debug a piece of code, no matter how small.
3. Practice Regularly
Consistent practice is key to building both your coding skills and your resilience. Make coding a regular part of your routine, even if it’s just for short periods each day.
Try these approaches:
- Commit to solving one coding problem daily on platforms like LeetCode or HackerRank.
- Work on a personal project that interests you, dedicating a set amount of time each week.
4. Learn from Failures
When you encounter a bug or your code doesn’t work as expected, treat it as a learning opportunity. Analyze what went wrong, understand why, and think about how you can prevent similar issues in the future.
Steps to take when facing a coding failure:
- Take a step back and breathe.
- Analyze the error messages or unexpected behavior.
- Break down the problem into smaller parts.
- Research similar issues and solutions.
- Document what you learn for future reference.
5. Seek Support and Collaboration
Don’t isolate yourself when facing challenges. Reach out to peers, mentors, or online communities for support and advice. Collaborating with others can provide new perspectives and solutions you might not have considered.
Ways to connect with other coders:
- Join coding forums or Discord servers.
- Participate in local coding meetups or hackathons.
- Contribute to open-source projects on GitHub.
- Form or join a study group with fellow learners.
6. Take Breaks and Practice Self-Care
Burnout can severely impact your resilience. Remember to take regular breaks, maintain a healthy work-life balance, and practice self-care to keep your mind fresh and motivated.
Self-care tips for coders:
- Use the Pomodoro Technique: Work for 25 minutes, then take a 5-minute break.
- Practice eye exercises to reduce strain from screen time.
- Engage in physical activity to boost mood and cognitive function.
- Ensure you’re getting enough sleep to support learning and problem-solving.
Common Coding Challenges and How to Overcome Them
Let’s look at some common challenges programmers face and strategies to overcome them resilient
Challenge 1: Debugging a Complex Issue
Debugging can be one of the most frustrating aspects of coding, especially when dealing with complex systems or unfamiliar codebases.
Resilient Approach:
- Break the problem down into smaller, manageable parts.
- Use debugging tools and print statements to trace the flow of your program.
- Implement a systematic approach, such as the scientific method, to identify and isolate the issue.
- Take breaks when feeling stuck to return with a fresh perspective.
- Document your debugging process for future reference.
Example: Let’s say you’re working on a web application and encounter a bug where user data isn’t being saved correctly. A resilient approach might look like this:
// Step 1: Add logging to track the flow of data
console.log('User data received:', userData);
// Step 2: Check if data is being sent correctly
fetch('/api/save-user', {
method: 'POST',
body: JSON.stringify(userData),
headers: {
'Content-Type': 'application/json'
}
})
.then(response => response.json())
.then(data => {
console.log('Server response:', data);
// Step 3: Verify server-side processing
})
.catch(error => {
console.error('Error:', error);
// Step 4: Implement error handling
});
// Step 5: Review and optimize the entire save process
Challenge 2: Learning a New Programming Language or Framework
The tech industry evolves rapidly, and programmers often need to learn new languages or frameworks to stay relevant.
Resilient Approach:
- Start with the basics and build a strong foundation.
- Set small, achievable goals to maintain motivation.
- Practice regularly with hands-on projects.
- Utilize various learning resources (documentation, tutorials, courses).
- Join communities specific to the language or framework for support.
Example: If you’re a JavaScript developer learning Python, you might approach it like this:
# Goal: Create a simple Python script that mimics a JavaScript function
# JavaScript version
# function greet(name) {
# return `Hello, ${name}!`;
# }
# Python equivalent
def greet(name):
return f"Hello, {name}!"
# Test the function
print(greet("Alice")) # Output: Hello, Alice!
# Next goal: Implement a more complex function or explore Python-specific features
Challenge 3: Optimizing Code Performance
As projects grow in scale and complexity, optimizing code for better performance becomes crucial but can be challenging.
Resilient Approach:
- Profile your code to identify bottlenecks.
- Research and apply optimization techniques specific to your language and use case.
- Implement changes incrementally and measure their impact.
- Seek code reviews from more experienced developers.
- Balance optimization with code readability and maintainability.
Example: Optimizing a function that finds the nth Fibonacci number:
// Original, inefficient recursive implementation
function fibonacci(n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
// Optimized version using memoization
function fibonacciOptimized(n, memo = {}) {
if (n in memo) return memo[n];
if (n <= 1) return n;
memo[n] = fibonacciOptimized(n - 1, memo) + fibonacciOptimized(n - 2, memo);
return memo[n];
}
// Test and compare performance
console.time('Original');
console.log(fibonacci(30));
console.timeEnd('Original');
console.time('Optimized');
console.log(fibonacciOptimized(30));
console.timeEnd('Optimized');
The Role of Failure in Learning and Growth
It’s essential to understand that failure is not just an unavoidable part of coding—it’s a crucial component of the learning process. Each error you encounter, each bug you fix, and each optimization you implement contributes to your growth as a programmer.
Reframing Failure
Instead of viewing failures as setbacks, try to reframe them as:
- Learning opportunities: Each failure teaches you something new about coding, problem-solving, or the specific technology you’re working with.
- Stepping stones: Failures are necessary steps on the path to success and mastery.
- Character builders: Overcoming challenges builds resilience, patience, and perseverance—qualities that are invaluable in any career.
The Iteration Process
Programming is inherently iterative. Rarely does a perfect solution emerge on the first try. Embracing this iterative process is key to building resilience:
- Write: Create an initial solution.
- Test: Run your code and identify issues.
- Analyze: Understand why certain parts aren’t working as expected.
- Refine: Make improvements based on your analysis.
- Repeat: Continue this cycle until you achieve the desired outcome.
This process not only improves your code but also enhances your problem-solving skills and deepens your understanding of the programming concepts at play.
Building a Growth-Oriented Coding Practice
To truly build resilience and thrive as a programmer, it’s important to cultivate a growth-oriented coding practice. Here are some strategies to incorporate into your routine:
1. Maintain a Learning Journal
Keep a journal or log of your coding experiences, challenges, and successes. This practice helps you track your progress, identify patterns in your learning, and reflect on your growth over time.
Example journal entry:
Date: 2023-06-15
Topic: Asynchronous JavaScript
What I learned today:
- The concept of Promises and how they handle asynchronous operations
- The syntax for async/await and how it simplifies working with Promises
Challenges faced:
- Initially confused about the difference between .then() chains and async/await
- Struggled with error handling in async functions
How I overcame challenges:
- Created small code samples to compare Promise syntax with async/await
- Researched and practiced different error handling techniques
Next steps:
- Build a small project utilizing async/await to fetch and process data from an API
- Review and refactor some of my older asynchronous code using these new concepts
2. Set SMART Coding Goals
Use the SMART framework (Specific, Measurable, Achievable, Relevant, Time-bound) to set coding goals that challenge you while remaining attainable.
Example SMART goal:
- Specific: Complete 30 LeetCode problems in the “Medium” difficulty category
- Measurable: Track progress through LeetCode’s built-in problem counter
- Achievable: Aim for 1-2 problems per day
- Relevant: Focus on problems related to data structures and algorithms
- Time-bound: Complete within the next 30 days
3. Engage in Code Reviews
Regularly participate in code reviews, both as a reviewer and by having your code reviewed. This practice exposes you to different coding styles, problem-solving approaches, and provides valuable feedback for improvement.
Tips for effective code reviews:
- Be open to constructive criticism and view it as an opportunity to learn.
- When reviewing others’ code, provide specific, actionable feedback.
- Ask questions to understand the reasoning behind certain implementations.
- Suggest alternative approaches or optimizations when appropriate.
4. Contribute to Open Source Projects
Contributing to open source projects is an excellent way to build resilience, as it exposes you to real-world codebases, collaborative development practices, and the challenges of working on established projects.
Steps to get started with open source contributions:
- Find a project that aligns with your interests and skill level.
- Read the project’s contribution guidelines and code of conduct.
- Start with small contributions, such as fixing typos or improving documentation.
- Gradually work your way up to more complex issues or feature implementations.
- Engage with the project’s community through issues, pull requests, and discussions.
Overcoming Imposter Syndrome
Imposter syndrome—the feeling that you’re not as competent as others perceive you to be—is common among programmers at all levels. It can significantly impact your resilience and confidence. Here are strategies to combat imposter syndrome:
1. Acknowledge Your Achievements
Keep a record of your accomplishments, no matter how small. This could include:
- Problems you’ve solved
- Projects you’ve completed
- Positive feedback you’ve received
- New skills or concepts you’ve mastered
Regularly reviewing these achievements can help counter feelings of inadequacy.
2. Embrace the “Yet” Mindset
When faced with a challenge, instead of thinking “I can’t do this,” add the word “yet” to the end of the sentence. This simple change shifts your perspective from a fixed mindset to a growth mindset.
For example:
- “I don’t understand recursive algorithms yet.”
- “I’m not proficient in React yet.”
3. Normalize Not Knowing Everything
Remember that even the most experienced programmers don’t know everything. The field of technology is vast and constantly evolving. It’s okay—and normal—to not have all the answers.
Practice saying:
- “I’m not familiar with that, but I’d love to learn more about it.”
- “I’m not sure, but let me research and get back to you.”
4. Seek Mentorship and Be a Mentor
Having a mentor can provide perspective, guidance, and reassurance when you’re doubting your abilities. Similarly, mentoring others can reinforce your own knowledge and highlight how much you’ve learned.
The Long-Term Benefits of Coding Resilience
Developing resilience in coding doesn’t just help you overcome immediate challenges—it has long-lasting benefits for your career and personal growth:
1. Adaptability in a Rapidly Changing Field
The tech industry is known for its rapid evolution. Languages, frameworks, and best practices can change quickly. Resilience equips you with the mindset and skills to adapt to these changes, ensuring your long-term relevance in the field.
2. Improved Problem-Solving Skills
The resilience you build through coding challenges enhances your overall problem-solving abilities. These skills are transferable to other areas of your life and career, making you a more effective professional in any role.
3. Increased Confidence and Self-Efficacy
As you overcome coding challenges and build resilience, your confidence in your abilities grows. This increased self-efficacy can lead to taking on more ambitious projects, pursuing leadership roles, or even starting your own tech ventures.
4. Better Stress Management
The strategies you develop for handling coding setbacks can be applied to managing stress in other areas of your life. This can lead to better overall mental health and work-life balance.
5. Continuous Learning and Growth
A resilient mindset fosters a love for continuous learning. This not only keeps your skills sharp but also opens up new opportunities for career advancement and personal development.
Conclusion
Building resilience in coding is not just about becoming a better programmer—it’s about developing a mindset that will serve you well throughout your career and life. By embracing challenges, learning from failures, and persistently working towards your goals, you’ll not only improve your coding skills but also cultivate qualities that are valuable in any profession.
Remember that every expert was once a beginner, and every successful programmer has faced numerous setbacks along the way. What sets them apart is their resilience—their ability to bounce back, learn, and keep moving forward.
As you continue your coding journey, whether you’re just starting out or are an experienced developer, keep nurturing your resilience. Embrace the challenges, celebrate the victories (big and small), and always keep learning. With a resilient mindset, there’s no limit to what you can achieve in the world of programming and beyond.
Happy coding, and may your resilience grow with every line of code you write!