Bucket Sort and Counting Sort: Efficient Sorting Algorithms for Specific Data Distributions
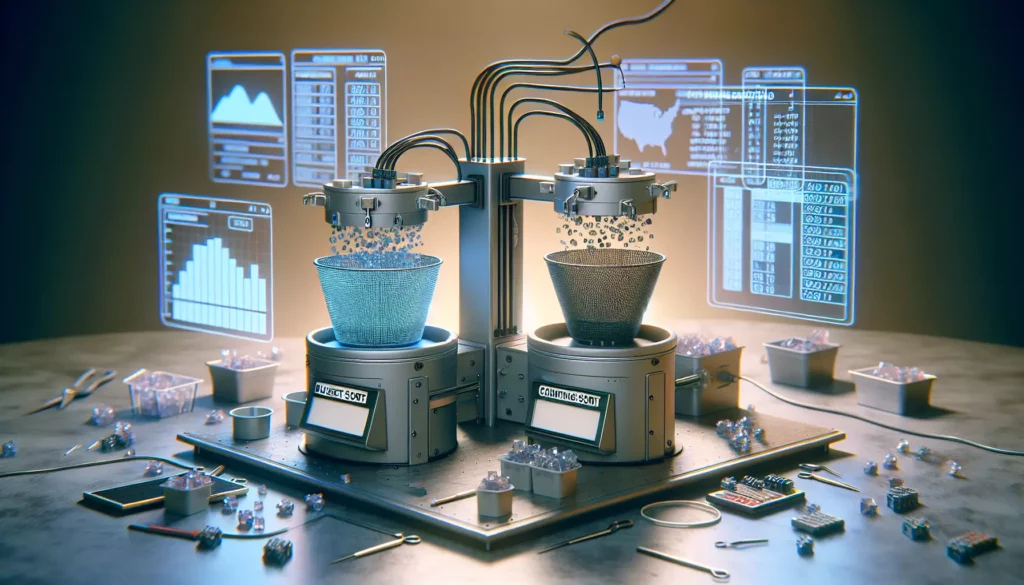
Sorting algorithms are fundamental components of computer science and play a crucial role in organizing and managing data efficiently. While popular algorithms like QuickSort and MergeSort are widely used for general-purpose sorting, there are specialized algorithms designed for specific data distributions that can outperform these general-purpose algorithms in certain scenarios. Two such algorithms are Bucket Sort and Counting Sort. In this comprehensive guide, we’ll explore these sorting techniques, their implementations, time complexities, and use cases.
Understanding Bucket Sort
Bucket Sort is a distribution-based sorting algorithm that works well when the input data is uniformly distributed over a range. The basic idea is to divide the range of input values into a fixed number of buckets, distribute the elements into these buckets, and then sort the elements within each bucket.
How Bucket Sort Works
- Create an array of empty buckets (or lists).
- Iterate through the input array, placing each element in its corresponding bucket based on its value.
- Sort the elements within each bucket using another sorting algorithm (often Insertion Sort for small bucket sizes).
- Concatenate the sorted buckets to get the final sorted array.
Implementing Bucket Sort in Python
Here’s a Python implementation of Bucket Sort:
def bucket_sort(arr):
# Find the maximum and minimum values in the array
max_val = max(arr)
min_val = min(arr)
# Calculate the range and number of buckets
value_range = max_val - min_val
num_buckets = len(arr)
# Create empty buckets
buckets = [[] for _ in range(num_buckets)]
# Distribute elements into buckets
for num in arr:
index = int((num - min_val) * (num_buckets - 1) / value_range)
buckets[index].append(num)
# Sort elements within each bucket (using Insertion Sort)
for bucket in buckets:
insertion_sort(bucket)
# Concatenate sorted buckets
sorted_arr = []
for bucket in buckets:
sorted_arr.extend(bucket)
return sorted_arr
def insertion_sort(arr):
for i in range(1, len(arr)):
key = arr[i]
j = i - 1
while j >= 0 and arr[j] > key:
arr[j + 1] = arr[j]
j -= 1
arr[j + 1] = key
# Example usage
arr = [0.897, 0.565, 0.656, 0.1234, 0.665, 0.3434]
sorted_arr = bucket_sort(arr)
print(sorted_arr)
Time Complexity of Bucket Sort
The time complexity of Bucket Sort depends on the distribution of the input data and the sorting algorithm used for sorting elements within each bucket. In the best and average cases, when the data is uniformly distributed, Bucket Sort can achieve a time complexity of O(n + k), where n is the number of elements and k is the number of buckets.
However, in the worst case, when all elements are placed in a single bucket, the time complexity can degrade to O(n^2) if using a quadratic sorting algorithm like Insertion Sort for the buckets.
Use Cases for Bucket Sort
- Sorting floating-point numbers uniformly distributed over a range
- Sorting strings with a known distribution of first characters
- External sorting, where data doesn’t fit in memory and is distributed across multiple files
Understanding Counting Sort
Counting Sort is another distribution-based sorting algorithm that works efficiently when the range of input values is known and limited. Unlike comparison-based sorting algorithms, Counting Sort uses the actual values of the elements to sort them.
How Counting Sort Works
- Find the range of input values (minimum and maximum).
- Create a count array to store the count of each unique object.
- Modify the count array to store the actual position of each object in the output array.
- Build the output array using the modified count array.
Implementing Counting Sort in Python
Here’s a Python implementation of Counting Sort:
def counting_sort(arr):
# Find the range of input values
max_val = max(arr)
min_val = min(arr)
range_val = max_val - min_val + 1
# Create and initialize the count array
count = [0] * range_val
# Count the occurrences of each element
for num in arr:
count[num - min_val] += 1
# Modify count array to store actual positions
for i in range(1, len(count)):
count[i] += count[i - 1]
# Build the output array
output = [0] * len(arr)
for num in reversed(arr):
index = count[num - min_val] - 1
output[index] = num
count[num - min_val] -= 1
return output
# Example usage
arr = [4, 2, 2, 8, 3, 3, 1]
sorted_arr = counting_sort(arr)
print(sorted_arr)
Time Complexity of Counting Sort
Counting Sort has a time complexity of O(n + k), where n is the number of elements in the input array and k is the range of input values. This makes it highly efficient when k is not significantly larger than n. The space complexity is also O(n + k) due to the auxiliary arrays used.
Use Cases for Counting Sort
- Sorting integers with a small range
- Sorting characters in a string
- As a subroutine in Radix Sort for sorting integers with a larger range
Comparing Bucket Sort and Counting Sort
While both Bucket Sort and Counting Sort are distribution-based sorting algorithms, they have some key differences:
Aspect | Bucket Sort | Counting Sort |
---|---|---|
Data Distribution | Works best with uniform distribution | Works with any distribution within a known range |
Time Complexity | O(n + k) average case, O(n^2) worst case | O(n + k) in all cases |
Space Complexity | O(n + k) | O(n + k) |
Stability | Can be stable depending on the inner sorting algorithm | Stable |
In-place | No | No |
Advanced Techniques and Optimizations
Optimizing Bucket Sort
1. Dynamic Bucket Sizing: Instead of using a fixed number of buckets, you can dynamically adjust the number of buckets based on the input size and distribution. This can help balance the trade-off between the number of buckets and the number of elements per bucket.
2. Adaptive Sorting: Use different sorting algorithms for different bucket sizes. For example, use Insertion Sort for small buckets and QuickSort for larger buckets.
3. Parallel Processing: Since buckets are independent, you can sort them in parallel to improve performance on multi-core systems.
Optimizing Counting Sort
1. In-place Counting Sort: Modify the algorithm to sort the array in-place, reducing the space complexity to O(k).
2. Handling Negative Numbers: Extend the algorithm to handle negative numbers by shifting the range.
3. Radix Sort Integration: Use Counting Sort as a subroutine in Radix Sort to handle larger ranges of integers efficiently.
Real-world Applications
Bucket Sort Applications
- Network Bandwidth Management: Sorting packets based on their size for efficient bandwidth allocation.
- Image Processing: Sorting pixels by color intensity for various image manipulation tasks.
- Geospatial Data: Organizing geographical coordinates for efficient spatial querying.
Counting Sort Applications
- Sorting Student Grades: When grades are within a fixed range (e.g., 0-100).
- Lexicographical Sorting: Sorting strings based on their ASCII values.
- Age Distribution Analysis: Sorting and analyzing age data in demographic studies.
Common Pitfalls and How to Avoid Them
Bucket Sort Pitfalls
- Uneven Distribution: When data is not uniformly distributed, some buckets may become significantly larger than others, leading to poor performance. Solution: Implement adaptive bucket sizing or use a different algorithm for highly skewed data.
- Memory Overhead: Creating too many buckets can lead to excessive memory usage. Solution: Balance the number of buckets with the expected input size and available memory.
Counting Sort Pitfalls
- Large Range of Values: When the range of input values is much larger than the number of elements, Counting Sort becomes inefficient. Solution: Consider using Radix Sort or a comparison-based algorithm for such cases.
- Floating-point Numbers: Counting Sort is designed for integers and doesn’t work directly with floating-point numbers. Solution: Use Bucket Sort for floating-point numbers or discretize the values if possible.
Integration with Other Algorithms
Both Bucket Sort and Counting Sort can be integrated with other algorithms to create more powerful sorting solutions:
Hybrid Sorting Algorithms
1. BucketQuick Sort: Use QuickSort to sort individual buckets in Bucket Sort for improved performance on non-uniform distributions.
2. Radix Sort with Counting Sort: Implement Radix Sort using Counting Sort as the stable sorting algorithm for each digit or character position.
Example: Radix Sort Implementation Using Counting Sort
def counting_sort_for_radix(arr, exp):
n = len(arr)
output = [0] * n
count = [0] * 10
for i in range(n):
index = arr[i] // exp
count[index % 10] += 1
for i in range(1, 10):
count[i] += count[i - 1]
i = n - 1
while i >= 0:
index = arr[i] // exp
output[count[index % 10] - 1] = arr[i]
count[index % 10] -= 1
i -= 1
for i in range(n):
arr[i] = output[i]
def radix_sort(arr):
max_val = max(arr)
exp = 1
while max_val // exp > 0:
counting_sort_for_radix(arr, exp)
exp *= 10
# Example usage
arr = [170, 45, 75, 90, 802, 24, 2, 66]
radix_sort(arr)
print(arr)
Performance Analysis and Benchmarking
To truly understand the efficiency of Bucket Sort and Counting Sort compared to other sorting algorithms, it’s essential to perform benchmarks on different data distributions and sizes. Here’s a simple benchmark comparing Bucket Sort, Counting Sort, and Python’s built-in sorted() function (which uses Timsort, a hybrid sorting algorithm):
import time
import random
def benchmark_sorting_algorithms(arr):
# Bucket Sort
start_time = time.time()
bucket_sort(arr.copy())
bucket_time = time.time() - start_time
# Counting Sort
start_time = time.time()
counting_sort(arr.copy())
counting_time = time.time() - start_time
# Python's built-in sort
start_time = time.time()
sorted(arr)
python_time = time.time() - start_time
print(f"Bucket Sort: {bucket_time:.6f} seconds")
print(f"Counting Sort: {counting_time:.6f} seconds")
print(f"Python's sorted(): {python_time:.6f} seconds")
# Generate random arrays for benchmarking
uniform_dist = [random.uniform(0, 1) for _ in range(10000)]
integer_dist = [random.randint(0, 1000) for _ in range(10000)]
print("Benchmarking uniform distribution:")
benchmark_sorting_algorithms(uniform_dist)
print("\nBenchmarking integer distribution:")
benchmark_sorting_algorithms(integer_dist)
This benchmark will give you an idea of how these algorithms perform on different data distributions compared to a general-purpose sorting algorithm.
Conclusion
Bucket Sort and Counting Sort are powerful sorting algorithms that can outperform comparison-based sorting algorithms in specific scenarios. Bucket Sort excels when dealing with uniformly distributed data, while Counting Sort is highly efficient for sorting integers within a limited range.
Understanding these algorithms and their appropriate use cases is crucial for any programmer or computer scientist. By leveraging the strengths of these distribution-based sorting techniques, you can significantly optimize your code’s performance when working with suitable data distributions.
As you continue to develop your algorithmic skills, remember that choosing the right algorithm for a given problem is often as important as implementing it correctly. Bucket Sort and Counting Sort are valuable tools to have in your algorithmic toolkit, alongside more general-purpose sorting algorithms.
Practice implementing and using these algorithms in various scenarios to gain a deeper understanding of their behavior and performance characteristics. This knowledge will serve you well in technical interviews and real-world programming challenges, particularly when optimizing data processing pipelines or working with large datasets.