Bridging the Gap: Applying Programming Concepts to Real-World Problems
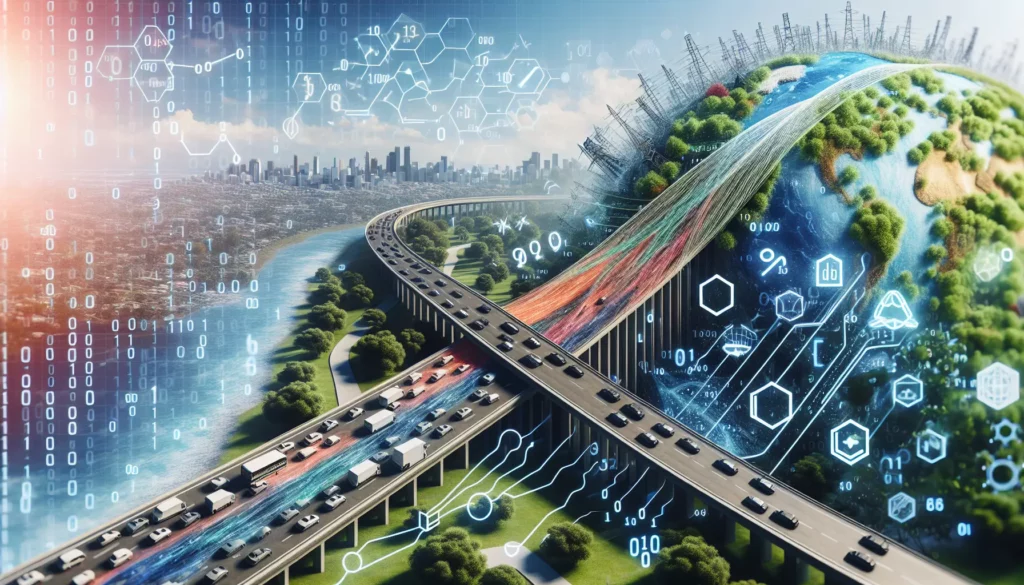
In the rapidly evolving landscape of technology, the ability to apply programming concepts to real-world problems has become an invaluable skill. For new programmers, this transition from theoretical knowledge to practical application can be both exciting and challenging. It demands not only a solid understanding of programming fundamentals but also the development of critical thinking and problem-solving abilities. In this comprehensive guide, we’ll explore strategies to help budding programmers bridge this gap, with a focus on the resources and methodologies offered by platforms like AlgoCademy.
Understanding the Challenge
Before diving into solutions, it’s crucial to recognize the hurdles that new programmers face when attempting to apply their skills to real-world scenarios:
- Complexity of Real-World Problems: Unlike textbook examples, real-world problems are often messy, with multiple variables and constraints.
- Lack of Clear Instructions: In educational settings, problems are usually well-defined. Real-world issues often require defining the problem itself.
- Scale and Performance Considerations: Solutions need to be efficient and scalable, not just functional.
- Integration with Existing Systems: Real-world programming often involves working with legacy code or integrating with other systems.
- Time and Resource Constraints: Unlike academic environments, real projects have deadlines and budget limitations.
The Role of Platforms like AlgoCademy
Coding education platforms like AlgoCademy play a pivotal role in bridging the gap between theoretical knowledge and practical application. Here’s how they contribute:
1. Interactive Coding Tutorials
Interactive tutorials provide a hands-on approach to learning. They allow programmers to:
- Experiment with code in real-time
- Receive immediate feedback on their solutions
- Progress at their own pace
For example, a tutorial on sorting algorithms might present a real-world scenario where efficient sorting is crucial, such as organizing a large database of customer information.
2. AI-Powered Assistance
AI assistants can provide personalized guidance, helping learners to:
- Identify areas for improvement
- Suggest alternative approaches to problem-solving
- Explain complex concepts in simpler terms
This feature is particularly useful when tackling complex problems that mimic real-world scenarios, as it can provide hints and explanations tailored to the individual’s progress and learning style.
3. Focus on Algorithmic Thinking
Platforms that emphasize algorithmic thinking help programmers to:
- Break down complex problems into manageable steps
- Develop efficient and scalable solutions
- Understand the underlying principles of problem-solving in programming
This focus is crucial for tackling real-world problems, which often require creative thinking and the ability to optimize solutions for performance.
4. Preparation for Technical Interviews
By offering resources tailored to technical interviews, especially for major tech companies, these platforms help programmers:
- Understand the types of problems they might face in real-world scenarios
- Practice solving problems under time constraints
- Learn to communicate their thought process effectively
This preparation is invaluable, as it simulates the problem-solving environment of actual tech jobs.
Strategies for Applying Programming Concepts to Real-World Problems
With the foundation provided by platforms like AlgoCademy, here are strategies that new programmers can employ to effectively apply their skills to real-world problems:
1. Start with Problem Analysis
Before writing any code, thoroughly analyze the problem at hand:
- Identify the core issue and desired outcome
- Break down the problem into smaller, manageable components
- Consider potential constraints and edge cases
Example: If tasked with creating a system to manage a library’s book inventory, start by listing all the functionalities needed (adding books, checking out, returns, etc.) and any constraints (e.g., maximum loan period, late fees).
2. Plan Your Approach
Develop a clear plan of action:
- Sketch out a high-level design of your solution
- Choose appropriate data structures and algorithms
- Consider scalability and performance from the outset
For the library system, you might decide to use a database to store book information, implement a search algorithm for finding books, and create a user authentication system for borrowers.
3. Start Small and Iterate
Begin with a minimal viable solution and build upon it:
- Implement core functionality first
- Test each component thoroughly before moving on
- Gradually add features and optimizations
In our library example, you might start with a simple command-line interface for adding and listing books, then progressively add features like checkout functionality and a graphical user interface.
4. Leverage Existing Resources
Don’t reinvent the wheel:
- Use libraries and frameworks where appropriate
- Study and adapt existing solutions to similar problems
- Consult documentation and community resources
For instance, you might use an existing database library for managing book records rather than creating a custom storage solution from scratch.
5. Practice Debugging and Testing
Develop robust debugging and testing skills:
- Write unit tests for individual components
- Implement integration tests for the system as a whole
- Use debugging tools to identify and fix issues efficiently
In the library system, you might write tests to ensure that book checkouts correctly update the inventory and that late fees are calculated accurately.
6. Seek Feedback and Collaborate
Engage with others to improve your solution:
- Share your code with peers or mentors for review
- Participate in coding forums and communities
- Collaborate on open-source projects to gain real-world experience
You could share your library system code on a platform like GitHub and ask for feedback from the developer community.
Practical Examples: Applying Concepts to Real-World Scenarios
Let’s explore some concrete examples of how programming concepts can be applied to solve real-world problems:
Example 1: Optimizing Delivery Routes
Problem: A local delivery company wants to optimize its routes to minimize fuel consumption and delivery time.
Concepts Applied:
- Graph theory
- Shortest path algorithms (e.g., Dijkstra’s algorithm)
- Optimization techniques
Solution Approach:
- Model the city as a graph, with intersections as nodes and roads as edges.
- Assign weights to edges based on distance, traffic patterns, and other relevant factors.
- Implement a shortest path algorithm to find optimal routes between delivery points.
- Use a scheduling algorithm to optimize the order of deliveries.
Sample Code Snippet (Python):
import networkx as nx
def optimize_route(city_graph, start, destinations):
# Create a graph
G = nx.Graph(city_graph)
# Find the shortest path between all points
shortest_paths = nx.all_pairs_dijkstra_path(G, weight='distance')
# Implement a simple greedy algorithm for route optimization
current_location = start
optimized_route = [start]
remaining_destinations = set(destinations)
while remaining_destinations:
next_destination = min(remaining_destinations,
key=lambda x: len(shortest_paths[current_location][x]))
optimized_route.extend(shortest_paths[current_location][next_destination][1:])
current_location = next_destination
remaining_destinations.remove(next_destination)
return optimized_route
# Example usage
city_graph = {
'A': {'B': 5, 'C': 2},
'B': {'A': 5, 'C': 1, 'D': 3},
'C': {'A': 2, 'B': 1, 'D': 6},
'D': {'B': 3, 'C': 6}
}
start_point = 'A'
delivery_points = ['B', 'C', 'D']
optimal_route = optimize_route(city_graph, start_point, delivery_points)
print("Optimal delivery route:", ' -> '.join(optimal_route))
This example demonstrates how graph theory and pathfinding algorithms can be applied to a real-world logistics problem. The solution can be further optimized by considering real-time traffic data, delivery time windows, and vehicle capacities.
Example 2: Sentiment Analysis for Customer Feedback
Problem: An e-commerce company wants to automatically analyze customer reviews to gauge product satisfaction and identify areas for improvement.
Concepts Applied:
- Natural Language Processing (NLP)
- Machine Learning
- Data preprocessing
Solution Approach:
- Collect and preprocess customer reviews (tokenization, removing stop words, etc.).
- Use a pre-trained sentiment analysis model or train a custom model on the dataset.
- Apply the model to classify reviews as positive, negative, or neutral.
- Aggregate results to provide insights on overall customer satisfaction and trending topics.
Sample Code Snippet (Python):
from textblob import TextBlob
import pandas as pd
def analyze_sentiment(text):
analysis = TextBlob(text)
if analysis.sentiment.polarity > 0:
return 'Positive'
elif analysis.sentiment.polarity == 0:
return 'Neutral'
else:
return 'Negative'
# Load customer reviews
df = pd.read_csv('customer_reviews.csv')
# Apply sentiment analysis
df['sentiment'] = df['review_text'].apply(analyze_sentiment)
# Aggregate results
sentiment_counts = df['sentiment'].value_counts()
print("Sentiment Analysis Results:")
print(sentiment_counts)
# Identify common words in positive and negative reviews
positive_words = ' '.join(df[df['sentiment'] == 'Positive']['review_text']).split()
negative_words = ' '.join(df[df['sentiment'] == 'Negative']['review_text']).split()
from collections import Counter
print("\nTop 5 words in positive reviews:")
print(Counter(positive_words).most_common(5))
print("\nTop 5 words in negative reviews:")
print(Counter(negative_words).most_common(5))
This example showcases how NLP and basic statistical analysis can be applied to gain insights from textual data. In a real-world scenario, this could be expanded to include more sophisticated NLP techniques, topic modeling, and integration with a dashboard for real-time analysis.
Example 3: Predictive Maintenance for Industrial Equipment
Problem: A manufacturing company wants to predict when their equipment is likely to fail to schedule maintenance proactively and minimize downtime.
Concepts Applied:
- Time series analysis
- Machine learning (classification and regression)
- Data visualization
Solution Approach:
- Collect historical data on equipment performance, maintenance records, and failure incidents.
- Preprocess and engineer features from the time series data.
- Train a machine learning model to predict the probability of failure within a given time frame.
- Implement a system to continuously monitor equipment and trigger alerts when the failure probability exceeds a threshold.
Sample Code Snippet (Python):
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import classification_report
import matplotlib.pyplot as plt
# Load and preprocess data
df = pd.read_csv('equipment_data.csv')
df['timestamp'] = pd.to_datetime(df['timestamp'])
df.set_index('timestamp', inplace=True)
# Feature engineering
df['rolling_mean_temp'] = df['temperature'].rolling(window=24).mean()
df['rolling_std_vibration'] = df['vibration'].rolling(window=24).std()
# Prepare features and target
X = df[['temperature', 'pressure', 'vibration', 'rolling_mean_temp', 'rolling_std_vibration']]
y = df['failure_within_24h']
# Split data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train model
model = RandomForestClassifier(n_estimators=100, random_state=42)
model.fit(X_train, y_train)
# Evaluate model
y_pred = model.predict(X_test)
print(classification_report(y_test, y_pred))
# Visualize feature importance
feature_importance = pd.DataFrame({'feature': X.columns, 'importance': model.feature_importances_})
feature_importance = feature_importance.sort_values('importance', ascending=False)
plt.figure(figsize=(10, 6))
plt.bar(feature_importance['feature'], feature_importance['importance'])
plt.title('Feature Importance for Predicting Equipment Failure')
plt.xlabel('Features')
plt.ylabel('Importance')
plt.xticks(rotation=45)
plt.tight_layout()
plt.show()
# Function to predict failure probability
def predict_failure_probability(new_data):
return model.predict_proba(new_data)[:, 1]
# Example usage
new_equipment_data = pd.DataFrame({
'temperature': [85],
'pressure': [110],
'vibration': [0.7],
'rolling_mean_temp': [82],
'rolling_std_vibration': [0.1]
})
failure_prob = predict_failure_probability(new_equipment_data)
print(f"Probability of failure within 24 hours: {failure_prob[0]:.2%}")
This example illustrates how machine learning can be applied to solve a critical problem in industrial settings. The solution combines time series analysis, feature engineering, and predictive modeling to create a system that can potentially save significant costs by preventing unexpected equipment failures.
Overcoming Common Challenges
As new programmers work on applying their skills to real-world problems, they may encounter several challenges. Here are some common issues and strategies to overcome them:
1. Dealing with Ambiguity
Challenge: Real-world problems often lack clear specifications or have evolving requirements.
Strategy:
- Practice asking clarifying questions to define the problem scope clearly.
- Develop prototypes or minimum viable products (MVPs) to gather feedback early.
- Use agile methodologies to adapt to changing requirements.
2. Handling Large-Scale Data
Challenge: Real-world applications often involve processing and analyzing large volumes of data.
Strategy:
- Learn and apply efficient data structures and algorithms.
- Utilize database management systems for data storage and retrieval.
- Explore big data technologies like Hadoop or Spark for distributed computing.
3. Ensuring Code Quality and Maintainability
Challenge: Writing code that is not only functional but also clean, maintainable, and scalable.
Strategy:
- Study and apply design patterns and principles (e.g., SOLID principles).
- Practice code refactoring to improve existing codebases.
- Use version control systems like Git to manage code changes effectively.
4. Integrating with Existing Systems
Challenge: Many real-world projects require integrating new code with legacy systems or third-party APIs.
Strategy:
- Develop a strong understanding of API design and integration patterns.
- Practice working with different data formats (JSON, XML, etc.) and protocols (REST, SOAP, etc.).
- Learn to read and understand documentation for various systems and APIs.
5. Optimizing Performance
Challenge: Ensuring that solutions are not just correct but also efficient and scalable.
Strategy:
- Study and apply performance optimization techniques specific to your programming language and domain.
- Learn to use profiling tools to identify performance bottlenecks.
- Practice optimizing algorithms and data structures for time and space complexity.
Conclusion: Bridging Theory and Practice
Applying programming concepts to real-world problems is a crucial skill that distinguishes proficient developers. While the journey from theory to practice can be challenging, it is also incredibly rewarding. Platforms like AlgoCademy play a vital role in this transition by providing structured learning paths, interactive tutorials, and practical problem-solving experiences.
To successfully bridge the gap between programming concepts and real-world applications:
- Continuously practice problem-solving with real-world scenarios.
- Engage in projects that challenge you to apply your skills in new contexts.
- Stay updated with industry trends and emerging technologies.
- Collaborate with others and seek mentorship from experienced developers.
- Reflect on your problem-solving process and learn from both successes and failures.
Remember that becoming proficient in applying programming concepts to real-world problems is an ongoing journey. Each challenge you tackle not only solves a specific problem but also enhances your overall capability as a programmer. By combining theoretical knowledge with practical application, leveraging resources like AlgoCademy, and persistently working on diverse projects, you’ll develop the skills and confidence to tackle even the most complex real-world programming challenges.