Breaking Down Complex Problems into Manageable Parts: A Programmer’s Guide
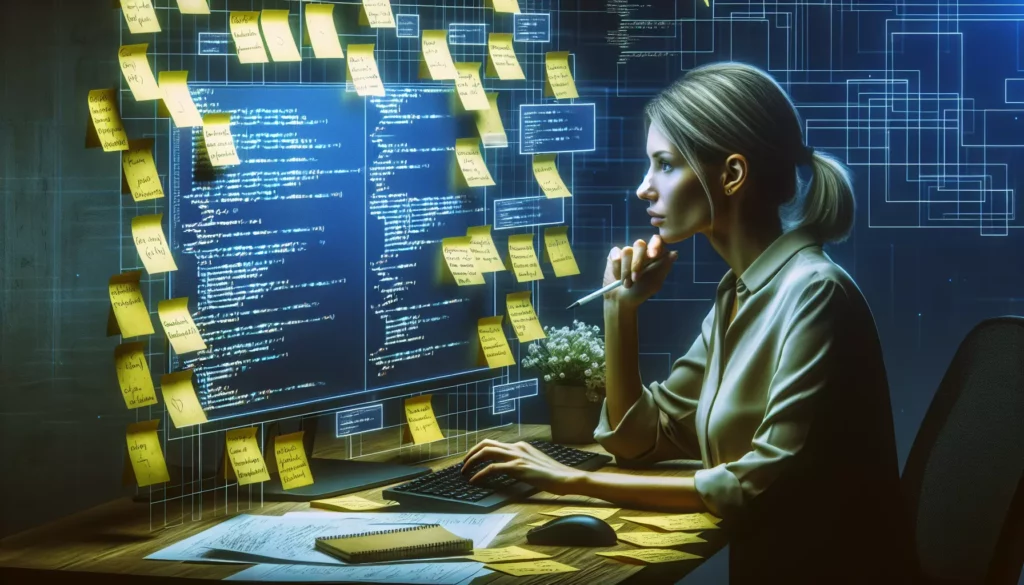
In the world of programming, tackling complex problems is an everyday challenge. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at major tech companies, the ability to break down intricate problems into smaller, manageable parts is an essential skill. This approach, often referred to as “problem decomposition,” is not only crucial for solving coding challenges but also for developing robust, scalable software solutions. In this comprehensive guide, we’ll explore the art of breaking down complex problems and how it can significantly enhance your programming skills and problem-solving abilities.
Why Breaking Down Problems Matters
Before we dive into the techniques of problem decomposition, it’s important to understand why this skill is so valuable in programming:
- Simplifies Complexity: Large, complex problems can be overwhelming. Breaking them down makes them more approachable and less daunting.
- Improves Understanding: Decomposing a problem forces you to analyze its different aspects, leading to a deeper understanding of the challenge at hand.
- Facilitates Collaboration: When working in teams, breaking down problems allows for better task distribution and parallel development.
- Enhances Problem-Solving Skills: Regular practice in decomposition sharpens your analytical and critical thinking abilities.
- Aids in Debugging: Smaller components are easier to test and debug, leading to more reliable code.
- Prepares for Technical Interviews: Many technical interviews, especially at FAANG companies, assess candidates’ ability to approach complex problems methodically.
Techniques for Breaking Down Complex Problems
Now that we understand the importance of problem decomposition, let’s explore some effective techniques you can use to break down complex problems:
1. Identify the Main Goal
Start by clearly defining the primary objective of the problem. What is the end result you’re trying to achieve? Having a clear goal in mind helps guide your decomposition process.
Example:
If the problem is to create a social media application, the main goal might be: “Develop a platform where users can create profiles, connect with friends, and share content.”
2. List the Major Components
Once you have the main goal, identify the major components or subsystems that make up the solution. These are the high-level building blocks of your program.
Example:
For the social media application, major components might include:
- User Authentication System
- Profile Management
- Friend Connection System
- Content Sharing Mechanism
- News Feed Generator
3. Break Down Each Component
Take each major component and break it down further into smaller, more manageable tasks or functions. This step often involves identifying the specific actions or processes within each component.
Example:
Let’s break down the “User Authentication System”:
- User Registration
- Collect user information
- Validate input
- Store user data securely
- Login Process
- Accept username/email and password
- Verify credentials
- Generate and manage session tokens
- Password Recovery
- Implement forgot password functionality
- Send reset instructions via email
- Allow secure password reset
4. Identify Dependencies
Determine how different components or tasks relate to each other. Are there dependencies between certain parts? Understanding these relationships helps in organizing your development process and identifying potential bottlenecks.
Example:
In our social media app:
- The Friend Connection System depends on the User Authentication System being in place.
- The News Feed Generator relies on both the Friend Connection System and the Content Sharing Mechanism.
5. Prioritize and Order Tasks
Based on the dependencies and the overall project goals, prioritize the tasks. Determine which components or features are essential for a minimum viable product (MVP) and which can be developed later.
Example:
A possible order of development:
- User Authentication System
- Profile Management
- Friend Connection System
- Content Sharing Mechanism
- News Feed Generator
6. Use Pseudocode or Flowcharts
For complex algorithms or processes within your components, use pseudocode or flowcharts to outline the logic before diving into actual coding. This step helps in visualizing the flow of your program and can reveal potential issues early on.
Example:
Pseudocode for the login process:
function login(username, password):
if username exists in database:
if password matches stored password for username:
generate session token
return success and session token
else:
return error: incorrect password
else:
return error: user not found
7. Implement Incrementally
Start implementing your solution one component at a time. This approach allows you to test and validate each part individually before integrating it into the larger system.
Example:
Begin by implementing the User Authentication System. Once it’s working correctly, move on to Profile Management, and so on.
Applying Problem Decomposition in Different Scenarios
The techniques for breaking down problems can be applied in various programming scenarios. Let’s look at how this approach can be used in different contexts:
Algorithmic Challenges
When faced with complex algorithmic problems, such as those often encountered in technical interviews, breaking down the problem is crucial. Here’s an example of how you might approach a classic algorithmic challenge:
Problem: Implement a function to find the longest palindromic substring in a given string.
Breaking it down:
- Understand what a palindrome is (reads the same backwards as forwards).
- Decide on an approach (e.g., expand around center method).
- Implement a helper function to expand around a center.
- Iterate through the string, considering each character as a potential center.
- Keep track of the longest palindrome found.
- Return the longest palindromic substring.
Here’s a simplified implementation in Python:
def longestPalindrome(s):
def expandAroundCenter(left, right):
while left >= 0 and right < len(s) and s[left] == s[right]:
left -= 1
right += 1
return s[left+1:right]
result = ""
for i in range(len(s)):
# Odd length palindromes
palindrome1 = expandAroundCenter(i, i)
# Even length palindromes
palindrome2 = expandAroundCenter(i, i+1)
# Update result if a longer palindrome is found
result = max(result, palindrome1, palindrome2, key=len)
return result
System Design
In system design problems, breaking down the system into components is essential. Let’s consider a high-level example:
Problem: Design a basic e-commerce platform.
Breaking it down:
- User Management System
- Registration
- Authentication
- Profile management
- Product Catalog
- Product listing
- Search functionality
- Categorization
- Shopping Cart
- Add/remove items
- Update quantities
- Calculate totals
- Order Processing
- Checkout process
- Payment integration
- Order confirmation
- Inventory Management
- Stock tracking
- Reorder notifications
Full-Stack Development
When working on a full-stack project, breaking down the problem helps in organizing both frontend and backend development. Here’s an example:
Project: Develop a task management application
Breaking it down:
- Backend Development
- Database design (Users, Tasks, Projects)
- API development
- User authentication endpoints
- CRUD operations for tasks
- Project management endpoints
- Server setup and configuration
- Frontend Development
- User Interface Design
- Dashboard layout
- Task list component
- Task creation/editing forms
- State Management
- User authentication state
- Task and project data management
- API Integration
- Implement API calls to backend
- Handle data fetching and updates
- User Interface Design
- Testing
- Unit tests for backend functions
- Integration tests for API endpoints
- Frontend component testing
- End-to-end testing
- Deployment
- Set up production environment
- Configure CI/CD pipeline
- Handle database migrations
Common Pitfalls and How to Avoid Them
While breaking down problems is a powerful technique, there are some common pitfalls to be aware of:
1. Over-complicating the Solution
Pitfall: Breaking down the problem into too many small parts, making the solution unnecessarily complex.
How to Avoid: Start with broader components and only break them down further if necessary. Aim for a balance between simplicity and thoroughness.
2. Losing Sight of the Big Picture
Pitfall: Focusing too much on individual components and forgetting how they fit into the overall solution.
How to Avoid: Regularly step back and review how your components interact and contribute to the main goal. Use diagrams or high-level documentation to maintain perspective.
3. Ignoring Edge Cases
Pitfall: Failing to consider unusual or extreme scenarios when breaking down the problem.
How to Avoid: For each component, brainstorm potential edge cases and how they might affect your solution. Include these considerations in your breakdown.
4. Premature Optimization
Pitfall: Spending too much time optimizing individual components before understanding how they fit together.
How to Avoid: Focus on getting a working solution first. Optimize only after you have a complete picture of how the components interact and where the bottlenecks are.
5. Neglecting Scalability
Pitfall: Breaking down the problem for the current requirements without considering future growth or changes.
How to Avoid: When decomposing the problem, think about how each component might need to scale or adapt in the future. Design with flexibility in mind.
Tools and Techniques to Aid Problem Decomposition
Several tools and techniques can assist in the process of breaking down complex problems:
1. Mind Mapping
Use mind mapping software or even pen and paper to visually represent the problem and its components. This technique helps in brainstorming and seeing connections between different parts of the problem.
2. UML Diagrams
Unified Modeling Language (UML) diagrams, such as class diagrams or sequence diagrams, can be invaluable for visualizing the structure and behavior of complex systems.
3. Task Management Tools
Tools like Trello, Jira, or Asana can help in organizing and tracking the various components and tasks identified during the breakdown process.
4. Pseudocode Editors
Specialized pseudocode editors or even simple text editors can be used to sketch out the logic of complex algorithms before actual coding begins.
5. Whiteboarding
Whether physical or digital, whiteboarding is an excellent way to collaboratively break down problems, especially in team settings or during technical interviews.
6. Version Control Systems
Tools like Git allow you to manage different components of your project separately, making it easier to work on decomposed parts in isolation.
Practicing Problem Decomposition
Like any skill, breaking down complex problems improves with practice. Here are some ways to hone this skill:
1. Solve Coding Challenges
Platforms like LeetCode, HackerRank, or AlgoCademy offer a variety of algorithmic problems. Practice breaking these down before implementing the solution.
2. Analyze Open Source Projects
Study how large, complex open-source projects are structured. Try to understand and document their component breakdown.
3. Participate in System Design Discussions
Engage in or read about system design discussions. These often involve breaking down large-scale systems into manageable components.
4. Reverse Engineer Applications
Take an application you use regularly and try to break it down into its components. Think about how you would structure it if you were building it from scratch.
5. Teach Others
Explaining complex concepts to others often requires breaking them down into simpler parts. This process can significantly improve your decomposition skills.
Conclusion
Breaking down complex problems into manageable parts is a fundamental skill in programming and software development. It’s an approach that not only makes difficult challenges more approachable but also leads to more organized, maintainable, and scalable solutions. Whether you’re tackling algorithmic puzzles, designing large-scale systems, or developing full-stack applications, the ability to decompose problems effectively will serve you well throughout your programming career.
Remember, the goal is not just to solve the problem at hand, but to do so in a way that is clear, efficient, and adaptable to future changes. By regularly practicing and refining your problem decomposition skills, you’ll find yourself better equipped to handle even the most complex programming challenges, whether in your daily work or during technical interviews at top tech companies.
As you continue your journey in programming and computer science, make problem decomposition a cornerstone of your approach. It’s a skill that will not only make you a better programmer but also a more effective problem solver in all areas of life. Happy coding!