Breaking Down Code: How to Understand Any Syntax You Encounter
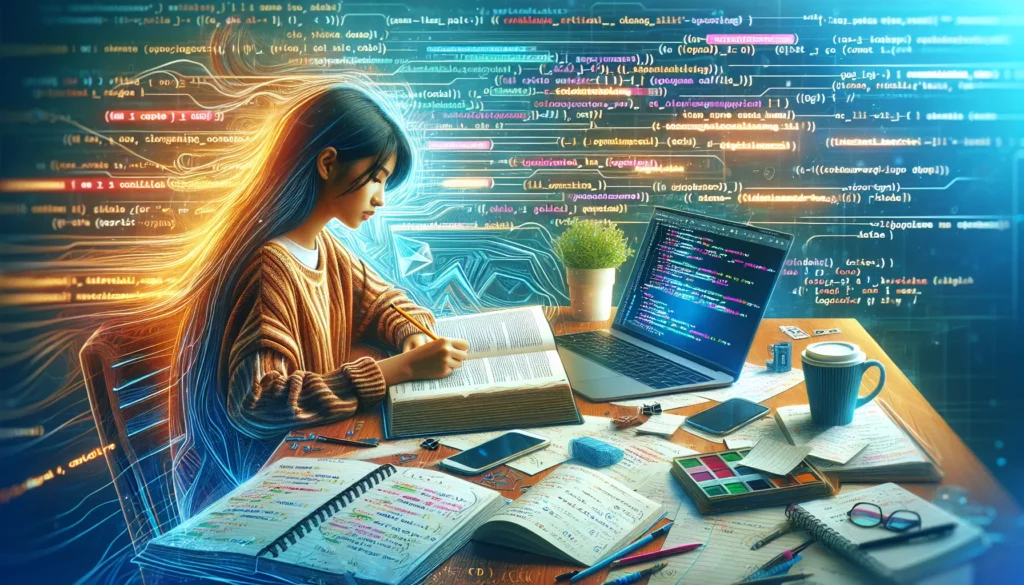
In the vast world of programming, encountering unfamiliar code syntax is a common experience, even for seasoned developers. Whether you’re a beginner just starting your coding journey or an experienced programmer exploring a new language, the ability to decipher and understand various syntaxes is a crucial skill. This comprehensive guide will walk you through the process of breaking down code and understanding any syntax you come across, empowering you to become a more versatile and confident programmer.
1. The Importance of Understanding Syntax
Before we dive into the techniques for breaking down code, let’s first understand why syntax comprehension is so vital in programming:
- Efficient Learning: Grasping syntax quickly allows you to learn new programming languages more efficiently.
- Debugging Skills: Understanding syntax helps in identifying and fixing errors in your code.
- Code Reading: It enables you to read and understand others’ code, which is crucial for collaboration and maintaining existing projects.
- Language Flexibility: Being syntax-savvy makes it easier to switch between different programming languages as needed.
- Problem-Solving: A solid grasp of syntax allows you to focus more on problem-solving and algorithmic thinking rather than getting stuck on language specifics.
2. Basic Elements of Programming Syntax
To effectively break down code, it’s essential to familiarize yourself with the basic elements that make up programming syntax. These elements are often common across many languages:
2.1. Variables and Data Types
Variables are used to store data, and each programming language has its own set of data types. Common data types include:
- Integers (whole numbers)
- Floating-point numbers (decimals)
- Strings (text)
- Booleans (true/false)
- Arrays or Lists
- Objects or Dictionaries
2.2. Operators
Operators are symbols used to perform operations on variables and values. They include:
- Arithmetic operators (+, -, *, /, %)
- Comparison operators (==, !=, <, >, <=, >=)
- Logical operators (AND, OR, NOT)
- Assignment operators (=, +=, -=, *=, /=)
2.3. Control Structures
Control structures determine the flow of program execution. Common control structures include:
- If-else statements
- Loops (for, while, do-while)
- Switch statements
2.4. Functions or Methods
Functions (also called methods in some languages) are reusable blocks of code that perform specific tasks. They typically have a name, parameters, and a return value.
2.5. Classes and Objects
In object-oriented programming languages, classes are blueprints for creating objects, which are instances of those classes.
3. Strategies for Breaking Down Unfamiliar Code
Now that we’ve covered the basic elements, let’s explore strategies for understanding unfamiliar code syntax:
3.1. Identify the Programming Language
The first step in breaking down code is to identify the programming language. This can often be done by looking at the file extension or by recognizing certain language-specific keywords or structures. Once you know the language, you can look up its documentation for reference.
3.2. Look for Familiar Patterns
Even in unfamiliar languages, you’ll often find patterns similar to languages you already know. Look for structures that resemble if-statements, loops, or function definitions. This can give you a general idea of what the code is doing.
3.3. Break the Code into Smaller Chunks
Don’t try to understand everything at once. Break the code into smaller, manageable chunks. Focus on understanding one section or function at a time.
3.4. Identify Variables and Their Types
Look for variable declarations and try to determine their types. This will give you insight into what kind of data the program is working with.
3.5. Trace the Program Flow
Follow the program’s execution flow. Start from the main entry point (often a main() function) and trace how the program progresses through different functions and control structures.
3.6. Use Online Resources
Don’t hesitate to use online resources like language documentation, Stack Overflow, or programming forums to look up unfamiliar syntax or functions.
3.7. Experiment with the Code
If possible, try running the code and experimenting with it. Modify parts of it and see how the output changes. This hands-on approach can greatly enhance your understanding.
4. Example: Breaking Down Python Code
Let’s apply these strategies to a Python code snippet:
def factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial(n-1)
numbers = [5, 3, 8, 2]
factorials = list(map(factorial, numbers))
print(factorials)
Let’s break it down:
- We can identify this as Python code based on the syntax and indentation.
- We see a function definition
def factorial(n):
. This defines a function named “factorial” that takes one parameter “n”. - Inside the function, we see an if-else statement, which is a control structure.
- The function appears to be recursive, as it calls itself within its own definition.
- Outside the function, we see a list being defined:
numbers = [5, 3, 8, 2]
. - The
map()
function is used along withlist()
to apply the factorial function to each number in the list. - Finally, the result is printed.
By breaking it down this way, we can understand that this code calculates the factorial of each number in the list and prints the results.
5. Common Syntax Patterns Across Languages
While programming languages can differ significantly, many share common syntax patterns. Recognizing these can help you quickly understand code in unfamiliar languages:
5.1. Variable Declaration and Assignment
Most languages have a way to declare variables and assign values to them. For example:
// JavaScript
let x = 5;
# Python
x = 5
// Java
int x = 5;
// C++
int x = 5;
5.2. Function Definitions
Functions are typically defined with a name, parameters, and a body. For instance:
// JavaScript
function greet(name) {
console.log("Hello, " + name);
}
# Python
def greet(name):
print("Hello, " + name)
// Java
public void greet(String name) {
System.out.println("Hello, " + name);
}
// C++
void greet(string name) {
cout << "Hello, " << name << endl;
}
5.3. Conditional Statements
If-else statements are common across many languages:
// JavaScript
if (x > 0) {
console.log("Positive");
} else {
console.log("Non-positive");
}
# Python
if x > 0:
print("Positive")
else:
print("Non-positive")
// Java
if (x > 0) {
System.out.println("Positive");
} else {
System.out.println("Non-positive");
}
// C++
if (x > 0) {
cout << "Positive" << endl;
} else {
cout << "Non-positive" << endl;
}
5.4. Loops
For and while loops are common iteration structures:
// JavaScript
for (let i = 0; i < 5; i++) {
console.log(i);
}
# Python
for i in range(5):
print(i)
// Java
for (int i = 0; i < 5; i++) {
System.out.println(i);
}
// C++
for (int i = 0; i < 5; i++) {
cout << i << endl;
}
6. Advanced Syntax Concepts
As you become more comfortable with basic syntax, you’ll encounter more advanced concepts. Here are a few to be aware of:
6.1. Lambda Functions (Anonymous Functions)
Many modern languages support lambda or anonymous functions, which are functions without a name:
// JavaScript
const square = x => x * x;
# Python
square = lambda x: x * x
// Java (Java 8+)
Function<Integer, Integer> square = x -> x * x;
// C++ (C++11+)
auto square = [](int x) { return x * x; };
6.2. List Comprehensions
Some languages, like Python, offer list comprehensions for creating lists based on existing lists:
# Python
squares = [x**2 for x in range(10)]
6.3. Decorators
Decorators are used in languages like Python to modify or enhance functions:
# Python
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
6.4. Generics
Generics allow you to write flexible, reusable code that can work with different types:
// Java
public class Box<T> {
private T t;
public void set(T t) { this.t = t; }
public T get() { return t; }
}
7. Tools and Techniques for Code Analysis
To aid in understanding complex or unfamiliar code, consider using these tools and techniques:
7.1. Integrated Development Environments (IDEs)
IDEs like Visual Studio Code, PyCharm, or IntelliJ IDEA offer features like syntax highlighting, code navigation, and intelligent code completion, which can help you understand code structure and syntax.
7.2. Debuggers
Debuggers allow you to step through code line by line, inspect variable values, and understand the flow of execution. This is invaluable for understanding complex logic.
7.3. Code Visualization Tools
Tools like PythonTutor allow you to visualize code execution step by step, which can be particularly helpful for understanding algorithms and data structures.
7.4. Static Code Analysis Tools
Tools like SonarQube or ESLint can analyze code for potential issues and provide insights into code quality and structure.
7.5. Version Control Systems
Using version control systems like Git allows you to track changes over time, which can be helpful in understanding how code evolves and why certain decisions were made.
8. Practice and Continuous Learning
Understanding diverse code syntaxes is a skill that improves with practice. Here are some ways to enhance your skills:
8.1. Code Reading Exercises
Regularly read code in languages you’re less familiar with. Websites like GitHub are great sources of open-source projects you can explore.
8.2. Coding Challenges
Participate in coding challenges on platforms like LeetCode, HackerRank, or CodeWars. Try solving problems in different languages to expose yourself to various syntaxes.
8.3. Contribute to Open Source
Contributing to open-source projects exposes you to different coding styles and syntaxes while also allowing you to learn from experienced developers.
8.4. Learn Multiple Programming Paradigms
Familiarize yourself with different programming paradigms like object-oriented, functional, and procedural programming. This broadens your understanding of how code can be structured.
Conclusion
Breaking down and understanding unfamiliar code syntax is a valuable skill that every programmer should cultivate. By familiarizing yourself with common programming elements, employing effective strategies for code analysis, and consistently practicing with diverse code samples, you can enhance your ability to quickly comprehend and work with any syntax you encounter.
Remember, the key to mastering this skill is patience and persistence. Don’t get discouraged if you don’t understand everything immediately. With time and practice, you’ll develop an intuition for breaking down code, making you a more versatile and effective programmer.
As you continue your journey in programming, embrace the challenge of unfamiliar syntaxes. Each new language or framework you encounter is an opportunity to expand your skills and deepen your understanding of programming concepts. Happy coding!