Blindfolded Coding: Enhancing Your Mental Model Through Sightless Programming
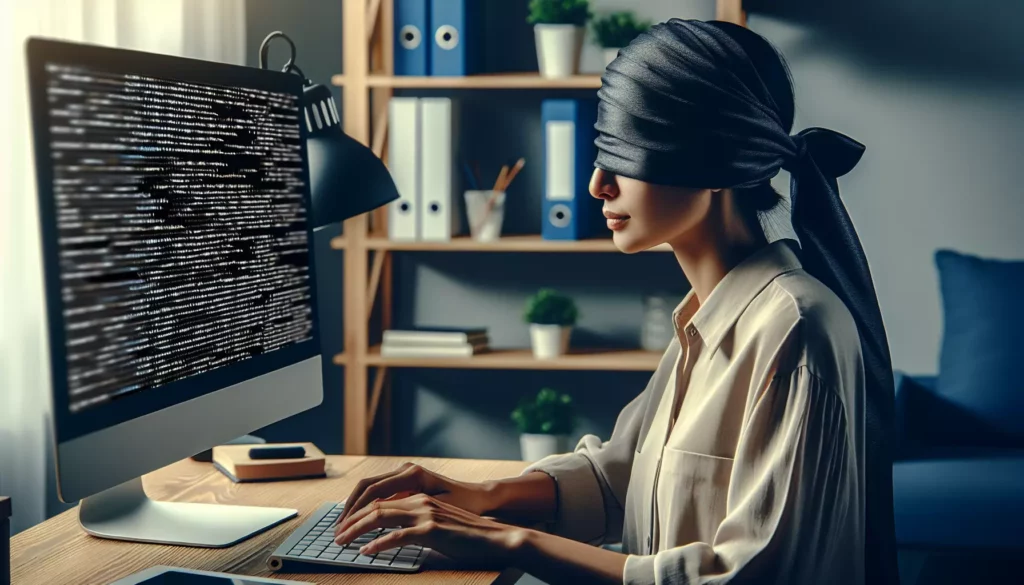
In the world of coding and software development, we often rely heavily on our visual senses. We stare at screens for hours, scrutinizing lines of code, debugging errors, and crafting elegant solutions to complex problems. But what if we were to remove our sense of sight from the equation? Enter the intriguing concept of blindfolded coding – a practice that challenges developers to write code without looking at their screens. This unconventional approach not only sharpens your coding skills but also enhances your mental model of programming, leading to improved problem-solving abilities and a deeper understanding of code structure.
What is Blindfolded Coding?
Blindfolded coding, as the name suggests, involves writing code without visual feedback. Developers attempt to write entire functions or solve coding problems relying solely on their mental representation of the code and their typing skills. This practice forces programmers to visualize the code structure in their minds, reinforcing their understanding of syntax, logic, and algorithm design.
While it may seem counterintuitive at first, blindfolded coding offers numerous benefits that can significantly enhance a programmer’s skills and cognitive abilities. Let’s explore these advantages and how you can incorporate this technique into your coding practice.
The Benefits of Blindfolded Coding
1. Strengthens Your Mental Model
One of the primary benefits of blindfolded coding is the strengthening of your mental model. When you can’t rely on visual cues, you’re forced to construct and maintain a clear mental image of your code structure. This process enhances your ability to think abstractly about code, improving your overall understanding of programming concepts and patterns.
2. Enhances Memory and Recall
Coding without visual aids requires you to remember syntax, function names, and coding patterns more effectively. This exercise in active recall strengthens your memory, making it easier to write code fluently in the future, even when you have access to visual feedback.
3. Improves Touch Typing Skills
Blindfolded coding naturally improves your touch typing skills. As you practice writing code without looking at the keyboard, you’ll become more proficient at typing, reducing the cognitive load associated with input and allowing you to focus more on problem-solving.
4. Boosts Problem-Solving Abilities
When you code blindfolded, you’re forced to think through problems more thoroughly before implementation. This approach encourages better planning and problem decomposition, leading to more efficient and well-structured solutions.
5. Increases Confidence
Successfully writing code without visual feedback can be a significant confidence booster. As you improve at blindfolded coding, you’ll gain more trust in your abilities, which can translate to increased confidence in your overall coding skills.
How to Practice Blindfolded Coding
Now that we’ve explored the benefits, let’s discuss how you can incorporate blindfolded coding into your practice routine:
1. Start Small
Begin with simple functions or algorithms that you’re already familiar with. Try writing a function to calculate the factorial of a number or implement a basic sorting algorithm. Here’s an example of a simple function you might attempt:
def factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial(n - 1)
2. Use a Text-to-Speech Tool
To check your work without breaking the blindfolded experience, consider using a text-to-speech tool. This allows you to hear your code read back to you, helping you catch syntax errors or logical mistakes without relying on visual feedback.
3. Practice Regularly
Like any skill, blindfolded coding improves with practice. Set aside time regularly – perhaps 15-30 minutes a day – to practice coding without looking at your screen.
4. Gradually Increase Complexity
As you become more comfortable with blindfolded coding, challenge yourself with more complex problems. Try implementing data structures, solving algorithmic challenges, or even working on small projects.
5. Use a Coding Partner
Practice blindfolded coding with a partner who can provide verbal feedback. This mimics pair programming and can help you catch errors more quickly while still maintaining the benefits of coding without visual input.
Blindfolded Coding Challenges
To help you get started with blindfolded coding, here are a few challenges you can try:
Challenge 1: Reverse a String
Try implementing a function to reverse a string without looking at your screen. Here’s what the function might look like:
def reverse_string(s):
return s[::-1]
Challenge 2: FizzBuzz
Attempt the classic FizzBuzz problem blindfolded. Remember, print “Fizz” for multiples of 3, “Buzz” for multiples of 5, and “FizzBuzz” for multiples of both:
def fizzbuzz(n):
for i in range(1, n+1):
if i % 3 == 0 and i % 5 == 0:
print("FizzBuzz")
elif i % 3 == 0:
print("Fizz")
elif i % 5 == 0:
print("Buzz")
else:
print(i)
Challenge 3: Binary Search
For a more advanced challenge, try implementing a binary search algorithm without visual feedback:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
Integrating Blindfolded Coding with AlgoCademy
As an AlgoCademy user focused on improving your coding skills and preparing for technical interviews, incorporating blindfolded coding into your practice routine can be incredibly beneficial. Here’s how you can integrate this technique with your AlgoCademy learning experience:
1. Review and Memorize
After completing an AlgoCademy tutorial or solving a problem with the platform’s guidance, try recreating the solution blindfolded. This will reinforce your understanding and help you internalize the concepts and patterns you’ve learned.
2. Pre-Interview Practice
As you prepare for technical interviews, especially for FAANG companies, use blindfolded coding to simulate the pressure of coding on a whiteboard. This can help you become more comfortable with explaining your thought process while coding, a crucial skill in many technical interviews.
3. Algorithm Mastery
AlgoCademy emphasizes algorithmic thinking. After studying a particular algorithm, challenge yourself to implement it blindfolded. This will deepen your understanding of the algorithm’s structure and logic.
4. Problem-Solving Skills
Use blindfolded coding to enhance your problem-solving skills. Before attempting to solve a new problem on AlgoCademy, try to plan out your approach mentally or verbally without looking at any code. This can improve your ability to break down problems and design solutions effectively.
5. Code Review Practice
After writing code blindfolded, use AlgoCademy’s AI-powered assistance to review your code. This can help you identify areas where your mental model might be lacking and provide opportunities for improvement.
Overcoming Challenges in Blindfolded Coding
While blindfolded coding offers numerous benefits, it’s not without its challenges. Here are some common obstacles you might face and strategies to overcome them:
1. Syntax Errors
Without visual feedback, it’s easy to make syntax errors. To combat this:
- Focus on mastering the syntax of your chosen programming language
- Practice writing common constructs (like loops and conditionals) repeatedly until they become second nature
- Use a linter or code formatter after your blindfolded session to catch and learn from any syntax errors
2. Losing Track of Structure
It can be challenging to keep track of nested structures or long functions when coding blindfolded. To address this:
- Start with shorter functions and gradually increase complexity
- Use clear, consistent indentation (even though you can’t see it)
- Verbalize your code structure as you write, like “opening if statement” or “closing while loop”
3. Difficulty Debugging
Debugging without visual input can be particularly challenging. To make it easier:
- Focus on writing clean, error-free code from the start
- Use print statements or logging to track the flow of your program
- Practice rubber duck debugging by explaining your code out loud
4. Frustration and Discouragement
Blindfolded coding can be frustrating, especially when you’re just starting. To stay motivated:
- Set realistic goals and celebrate small victories
- Remember that the process is about improving your skills, not achieving perfection
- Take breaks when needed and return to the practice with a fresh mind
Advanced Blindfolded Coding Techniques
As you become more comfortable with basic blindfolded coding, you can explore more advanced techniques to further enhance your skills:
1. Blindfolded Refactoring
Challenge yourself to refactor existing code without looking at it. This exercise helps you think critically about code structure and design patterns. For example, try converting a series of if-else statements into a more elegant switch statement or dictionary lookup.
2. Mental Debugging
Practice debugging code in your head. Given a description of a bug, try to mentally trace through the code to identify the issue. This enhances your ability to reason about code execution and spot logical errors.
3. Blindfolded Code Reviews
Have a colleague read out code to you and provide a code review without seeing the code. This sharpens your ability to understand and critique code aurally, a valuable skill for pair programming and code reviews.
4. Time-Constrained Challenges
Add a time constraint to your blindfolded coding sessions. This simulates the pressure of coding interviews and helps you think and code more efficiently.
5. Multi-Language Practice
If you’re proficient in multiple programming languages, practice blindfolded coding in different languages. This helps reinforce your understanding of language-specific syntax and idioms.
The Science Behind Blindfolded Coding
The effectiveness of blindfolded coding isn’t just anecdotal; it’s backed by cognitive science and learning theories:
1. Active Recall
Blindfolded coding is a form of active recall, a learning principle that involves actively stimulating memory during the learning process. Research has shown that active recall leads to stronger memory formation compared to passive review.
2. Elaborative Rehearsal
When coding blindfolded, you’re forced to think more deeply about the code you’re writing, engaging in elaborative rehearsal. This process helps transfer information from short-term to long-term memory more effectively.
3. Cognitive Load Theory
By removing visual input, blindfolded coding reduces extraneous cognitive load, allowing you to focus more cognitive resources on the intrinsic aspects of coding and problem-solving.
4. Neuroplasticity
The challenge of coding without visual feedback promotes neuroplasticity – the brain’s ability to form new neural connections. This can lead to improved cognitive function and problem-solving skills over time.
Blindfolded Coding in Professional Development
While blindfolded coding is an excellent personal development tool, it also has applications in professional settings:
1. Team Building Exercises
Blindfolded coding can be used as a fun and challenging team-building exercise, promoting communication and collaboration among team members.
2. Interview Techniques
Some companies have incorporated elements of blindfolded coding into their interview processes to assess candidates’ deep understanding of programming concepts and their ability to communicate their thought processes.
3. Code Quality Improvement
Teams that practice blindfolded coding often report improvements in overall code quality, as the practice encourages more thoughtful and structured coding approaches.
4. Accessibility Awareness
Blindfolded coding can increase awareness of accessibility issues in software development, encouraging developers to create more inclusive and accessible applications.
Conclusion
Blindfolded coding is more than just a novel exercise; it’s a powerful tool for enhancing your programming skills, deepening your understanding of code, and improving your problem-solving abilities. By removing visual input, this practice forces you to strengthen your mental model of programming, leading to more robust and efficient coding skills.
As you progress in your coding journey with AlgoCademy, consider incorporating blindfolded coding into your regular practice routine. Start small, be patient with yourself, and gradually increase the complexity of your blindfolded coding challenges. Remember, the goal is not to become an expert at coding without sight, but to use this technique as a means to enhance your overall coding abilities.
Whether you’re a beginner looking to solidify your understanding of basic concepts or an experienced developer preparing for technical interviews at top tech companies, blindfolded coding can be an invaluable addition to your skill-building toolkit. Embrace the challenge, push your limits, and watch as your coding abilities reach new heights. Happy coding!