Bit Shift Calculator: Mastering Bitwise Operations for Efficient Coding
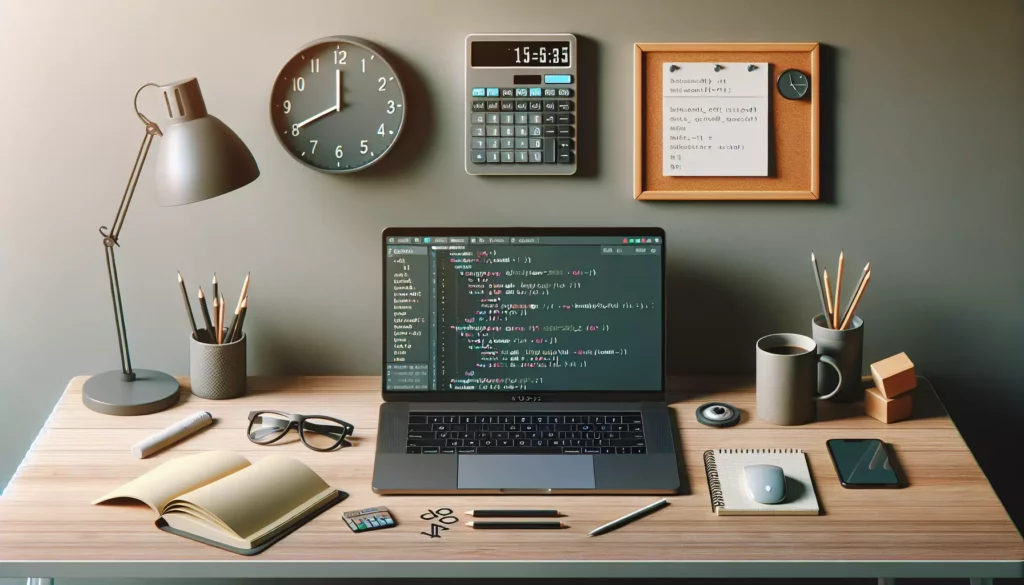
In the world of programming and computer science, understanding bitwise operations is crucial for developing efficient algorithms and optimizing code performance. One of the most fundamental bitwise operations is the bit shift, which can be a powerful tool in a programmer’s arsenal. In this comprehensive guide, we’ll explore the concept of bit shifting, its applications, and how to use a bit shift calculator to enhance your coding skills.
What is Bit Shifting?
Bit shifting is a bitwise operation that involves moving the bits of a binary number to the left or right by a specified number of positions. This operation can be performed on integer values and is often used for various purposes, including multiplication and division by powers of two, efficient data manipulation, and implementing certain algorithms.
There are two types of bit shift operations:
- Left Shift ( Shifts the bits to the left, effectively multiplying the number by 2 for each position shifted.
- Right Shift (>>): Shifts the bits to the right, effectively dividing the number by 2 for each position shifted (for unsigned integers).
How Bit Shifting Works
To understand bit shifting, let’s look at some examples:
Left Shift Example
Consider the binary number 00001010 (decimal 10). If we perform a left shift by 2 positions, we get:
00001010 << 2 = 00101000
The result is 00101000 (decimal 40). As you can see, shifting left by 2 positions is equivalent to multiplying by 4 (2^2).
Right Shift Example
Now, let’s take the binary number 00101000 (decimal 40) and perform a right shift by 2 positions:
00101000 >> 2 = 00001010
The result is 00001010 (decimal 10). Right shifting by 2 positions is equivalent to dividing by 4 (2^2).
Applications of Bit Shifting
Bit shifting has numerous applications in programming and computer science. Here are some common use cases:
1. Efficient Multiplication and Division
Bit shifting can be used to perform quick multiplication and division by powers of two. Left shifting by n positions is equivalent to multiplying by 2^n, while right shifting by n positions is equivalent to dividing by 2^n (for unsigned integers).
2. Bitwise Flags and Masks
Bit shifting is often used to create and manipulate bitwise flags and masks. This is particularly useful for storing multiple boolean values in a single integer, saving memory and improving performance.
3. Data Compression
Bit shifting can be employed in various data compression algorithms to pack and unpack data efficiently.
4. Color Manipulation
In graphics programming, bit shifting is used to manipulate color values, which are often represented as 32-bit integers with separate channels for red, green, blue, and alpha.
5. Cryptography
Many cryptographic algorithms rely on bit shifting operations as part of their encryption and decryption processes.
Using a Bit Shift Calculator
A bit shift calculator is a valuable tool for understanding and visualizing bit shift operations. It allows you to input a number, specify the shift direction and amount, and see the result in both binary and decimal formats. Here’s how you can use a bit shift calculator effectively:
Step 1: Input the Number
Enter the decimal or binary number you want to shift. Most calculators allow both formats.
Step 2: Choose the Shift Direction
Select whether you want to perform a left shift (<<) or a right shift (>>).
Step 3: Specify the Shift Amount
Enter the number of positions you want to shift the bits.
Step 4: Calculate and Analyze the Result
The calculator will display the result in both binary and decimal formats. Analyze how the bits have moved and how the value has changed.
Implementing a Bit Shift Calculator in Code
To deepen your understanding of bit shifting, it’s valuable to implement your own bit shift calculator. Here’s a simple implementation in Python:
def bit_shift_calculator(number, direction, shift_amount):
if direction == "left":
result = number << shift_amount
elif direction == "right":
result = number >> shift_amount
else:
raise ValueError("Invalid direction. Use 'left' or 'right'.")
print(f"Original number: {number} (binary: {bin(number)[2:].zfill(32)})")
print(f"Shifted number: {result} (binary: {bin(result)[2:].zfill(32)})")
return result
# Example usage
number = 10
direction = "left"
shift_amount = 2
bit_shift_calculator(number, direction, shift_amount)
This implementation allows you to input a number, specify the shift direction and amount, and see the result in both decimal and binary formats.
Common Pitfalls and Considerations
While bit shifting is a powerful tool, there are some important considerations and potential pitfalls to keep in mind:
1. Sign Extension in Right Shifts
When performing right shifts on signed integers, the behavior can vary depending on the programming language and hardware. Some systems perform arithmetic right shifts (preserving the sign bit), while others perform logical right shifts (filling with zeros).
2. Overflow and Underflow
Be cautious of potential overflow when left-shifting and underflow when right-shifting, especially when working with fixed-width integer types.
3. Language-Specific Behavior
Different programming languages may have slight variations in how they handle bit shifting, especially for edge cases. Always consult the language documentation for specific details.
4. Shift Amount Limitations
Most programming languages limit the shift amount to the width of the integer type being shifted. Shifting by an amount greater than or equal to the bit width may produce unexpected results or be undefined behavior.
Advanced Bit Shifting Techniques
As you become more comfortable with basic bit shifting, you can explore more advanced techniques and applications:
1. Circular Shifts
Circular shifts (also known as rotations) involve wrapping the bits that are shifted off one end back to the other end. This can be implemented using a combination of shifts and bitwise OR operations.
2. Multi-Precision Arithmetic
Bit shifting can be used to implement arithmetic operations on numbers that are too large to fit in standard integer types, by operating on arrays of integers.
3. Fast Fourier Transform (FFT) Optimization
Some FFT implementations use bit-reversal permutations, which can be efficiently implemented using bit shifting techniques.
4. Gray Code Generation
Gray codes, which are sequences of binary numbers where adjacent numbers differ by only one bit, can be generated efficiently using bit shifting and XOR operations.
Bit Shifting in Competitive Programming
For those interested in competitive programming or preparing for technical interviews, mastering bit shifting can give you a significant advantage. Many algorithmic problems can be solved more efficiently using bitwise operations, including bit shifting. Here are some common problem types where bit shifting can be particularly useful:
1. Power Set Generation
Generating all possible subsets of a set can be done efficiently using bit shifting to represent each subset as a bitmask.
2. Detecting Power of Two
A number is a power of two if and only if it has exactly one bit set in its binary representation. This can be checked efficiently using bit manipulation:
def is_power_of_two(n):
return n > 0 and (n & (n - 1)) == 0
3. Counting Set Bits
The number of set bits (1s) in a binary number can be counted efficiently using techniques like the Brian Kernighan’s algorithm, which uses bit shifting:
def count_set_bits(n):
count = 0
while n:
n &= (n - 1)
count += 1
return count
4. Solving Puzzles and Games
Many puzzle-solving algorithms, particularly for games like chess or Go, use bitboards (a data structure that uses bit shifting for efficient board representation and move generation).
Bit Shifting in Real-World Applications
Understanding bit shifting isn’t just an academic exercise; it has real-world applications in various domains of software development:
1. Network Programming
Bit shifting is often used in network programming for tasks like IP address manipulation, subnet calculations, and packet header parsing.
2. Embedded Systems
In embedded systems and microcontroller programming, bit shifting is crucial for efficient register manipulation and I/O operations.
3. Graphics Programming
As mentioned earlier, graphics programming heavily relies on bit shifting for color manipulation, texture compression, and various rendering optimizations.
4. Database Systems
Some database systems use bit shifting techniques for efficient indexing and query optimization.
5. File Systems
File system implementations often use bit shifting for tasks like permission management and block allocation.
Conclusion
Mastering bit shifting and understanding its applications is an essential skill for any serious programmer. From optimizing algorithms to solving complex problems in competitive programming, the ability to manipulate bits efficiently can significantly enhance your coding prowess.
As you continue your journey in programming and computer science, remember that bit shifting is just one of many powerful tools in the realm of bitwise operations. By combining bit shifting with other bitwise operations like AND, OR, XOR, and NOT, you can unlock even more possibilities for efficient and elegant code.
Practice regularly with a bit shift calculator, implement your own bitwise algorithms, and challenge yourself with problems that require bit manipulation. As you gain experience, you’ll find that thinking at the bit level becomes second nature, opening up new avenues for optimization and problem-solving in your programming career.
Whether you’re preparing for technical interviews, diving into low-level systems programming, or simply looking to write more efficient code, a solid understanding of bit shifting will serve you well. So keep exploring, keep practicing, and watch as the world of bits and bytes unfolds before you, revealing the elegant simplicity that lies at the heart of all computing.