Binary Indexed Tree (Fenwick Tree): A Comprehensive Guide
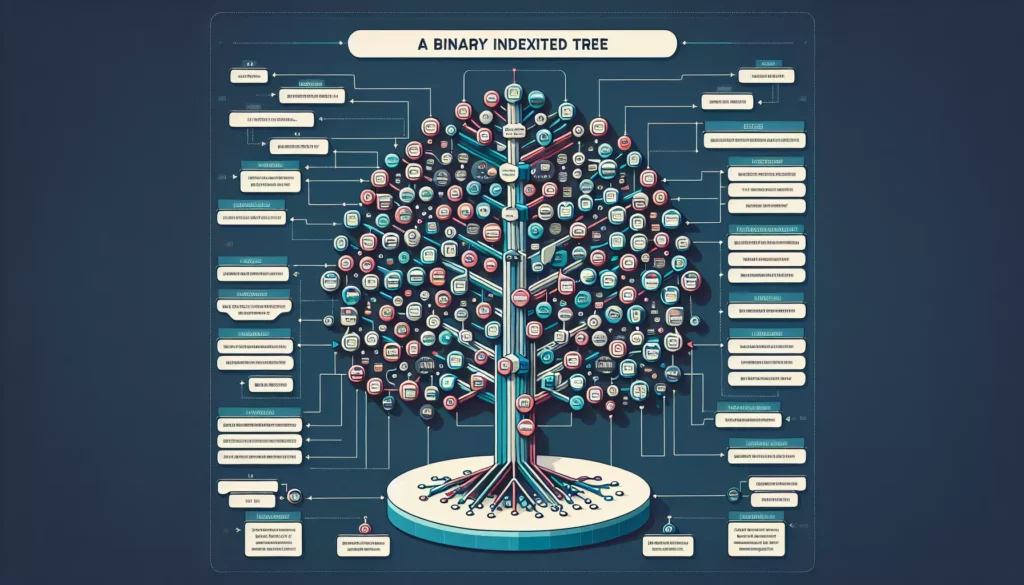
In the world of competitive programming and advanced data structures, the Binary Indexed Tree (BIT), also known as a Fenwick Tree, stands out as a powerful and efficient tool. This data structure, invented by Peter M. Fenwick in 1994, offers a clever way to perform range queries and updates on an array in logarithmic time. In this comprehensive guide, we’ll dive deep into the Binary Indexed Tree, exploring its concepts, implementation, and applications.
Table of Contents
- Introduction to Binary Indexed Trees
- The Concept Behind Binary Indexed Trees
- Basic Operations
- Implementation in Python
- Time Complexity Analysis
- Applications and Use Cases
- Comparison with Other Data Structures
- Advanced Techniques and Variations
- Practice Problems
- Conclusion
1. Introduction to Binary Indexed Trees
A Binary Indexed Tree, or Fenwick Tree, is a data structure that provides efficient methods for calculating and manipulating cumulative frequency tables. It was designed to improve the performance of prefix sum calculations and range sum queries in arrays.
The main advantages of Binary Indexed Trees include:
- Efficient updates and queries in O(log n) time complexity
- Simple implementation compared to other advanced data structures
- Space-efficient, requiring only O(n) space
- Versatility in solving various problems involving range queries
2. The Concept Behind Binary Indexed Trees
The key idea behind a Binary Indexed Tree is to represent an array of numbers in a way that allows quick cumulative frequency computations. It achieves this by storing partial sum information in an intelligent manner.
To understand how a BIT works, let’s break down its core concepts:
2.1 Binary Representation
The structure of a BIT is closely tied to the binary representation of indices. Each index in the BIT represents a range of elements in the original array. The size of this range is determined by the least significant bit (LSB) of the index.
2.2 Parent-Child Relationship
In a BIT, each element is responsible for a certain range of the original array. The parent of an element is responsible for a larger range that includes the range of its child. This hierarchical structure allows for efficient querying and updating.
2.3 Prefix Sums
The BIT stores partial sums in such a way that the sum of any prefix of the array can be computed by combining a small number of these partial sums. This is the key to its efficiency in range sum queries.
3. Basic Operations
The two fundamental operations in a Binary Indexed Tree are:
3.1 Update Operation
The update operation is used to modify a single element in the array. When an element is updated, all the partial sums that include this element need to be updated as well. This is done efficiently by updating only the necessary nodes in the tree.
3.2 Query Operation
The query operation is used to compute the sum of elements in a given range. It does this by combining the partial sums stored in the BIT nodes.
4. Implementation in Python
Let’s implement a Binary Indexed Tree in Python. We’ll create a class that supports both update and query operations.
class BinaryIndexedTree:
def __init__(self, size):
self.size = size
self.tree = [0] * (size + 1)
def update(self, index, value):
while index <= self.size:
self.tree[index] += value
index += index & (-index)
def query(self, index):
total = 0
while index > 0:
total += self.tree[index]
index -= index & (-index)
return total
def range_query(self, left, right):
return self.query(right) - self.query(left - 1)
Let’s break down the implementation:
- The
__init__
method initializes the BIT with a given size. Note that we allocate an extra element (size + 1) because BITs are typically 1-indexed. - The
update
method adds a value to an element and updates all necessary partial sums. - The
query
method computes the sum of elements from index 1 to the given index. - The
range_query
method computes the sum of elements in a given range by using the difference of two prefix sums.
Now, let’s see how to use this implementation:
# Create a BIT for an array of size 10
bit = BinaryIndexedTree(10)
# Update some values
bit.update(1, 3)
bit.update(2, 2)
bit.update(3, 5)
bit.update(4, 1)
bit.update(5, 4)
# Query prefix sums
print(bit.query(3)) # Sum of elements from 1 to 3: 3 + 2 + 5 = 10
print(bit.query(5)) # Sum of elements from 1 to 5: 3 + 2 + 5 + 1 + 4 = 15
# Query range sum
print(bit.range_query(2, 4)) # Sum of elements from 2 to 4: 2 + 5 + 1 = 8
5. Time Complexity Analysis
The efficiency of Binary Indexed Trees comes from their clever use of binary representation. Let’s analyze the time complexity of the main operations:
5.1 Update Operation
The update operation has a time complexity of O(log n), where n is the size of the array. This is because in each step, we move to a parent node by adding the least significant bit, which effectively divides the remaining distance by 2.
5.2 Query Operation
The query operation also has a time complexity of O(log n). Similar to the update operation, we move up the tree by removing the least significant bit in each step, which again divides the remaining distance by 2.
5.3 Range Query
The range query operation involves two query operations, so its time complexity is also O(log n).
5.4 Space Complexity
The space complexity of a Binary Indexed Tree is O(n), as it requires an array of size n+1 to store the partial sums.
6. Applications and Use Cases
Binary Indexed Trees are versatile data structures with numerous applications in competitive programming and real-world scenarios. Some common use cases include:
6.1 Range Sum Queries
BITs excel at answering range sum queries efficiently, making them ideal for problems involving cumulative frequencies or sums over ranges.
6.2 Data Analysis
In data analysis and statistics, BITs can be used to efficiently compute moving averages or maintain cumulative statistics over dynamic datasets.
6.3 Computational Geometry
BITs can be applied to solve various computational geometry problems, such as counting inversions or finding the number of smaller elements to the right.
6.4 Game Development
In game development, BITs can be used for efficient collision detection or managing game state that requires frequent updates and range queries.
7. Comparison with Other Data Structures
To better understand the strengths of Binary Indexed Trees, let’s compare them with other data structures used for similar purposes:
7.1 BIT vs. Segment Tree
Both BITs and Segment Trees support range queries and updates in O(log n) time. However:
- BITs are simpler to implement and have a smaller constant factor in their time complexity.
- Segment Trees are more versatile and can handle a wider range of operations (like finding the minimum in a range).
- BITs use less memory (O(n) vs O(n log n) for Segment Trees).
7.2 BIT vs. Prefix Sum Array
Prefix Sum Arrays offer O(1) range sum queries but O(n) updates. BITs provide a balance with O(log n) for both operations, making them more suitable for scenarios with frequent updates.
7.3 BIT vs. Square Root Decomposition
Square Root Decomposition offers O(√n) complexity for both updates and queries. BITs are generally faster for larger datasets but may be overkill for smaller arrays where Square Root Decomposition could be simpler and effective.
8. Advanced Techniques and Variations
While the basic Binary Indexed Tree is powerful, there are several advanced techniques and variations that extend its capabilities:
8.1 2D Binary Indexed Tree
A 2D BIT can handle range sum queries and updates on a 2D grid. It’s particularly useful in problems involving matrices or 2D coordinate systems.
class BinaryIndexedTree2D:
def __init__(self, rows, cols):
self.rows = rows
self.cols = cols
self.tree = [[0] * (cols + 1) for _ in range(rows + 1)]
def update(self, row, col, value):
i = row
while i <= self.rows:
j = col
while j <= self.cols:
self.tree[i][j] += value
j += j & (-j)
i += i & (-i)
def query(self, row, col):
total = 0
i = row
while i > 0:
j = col
while j > 0:
total += self.tree[i][j]
j -= j & (-j)
i -= i & (-i)
return total
def range_query(self, row1, col1, row2, col2):
return (self.query(row2, col2) - self.query(row1 - 1, col2) -
self.query(row2, col1 - 1) + self.query(row1 - 1, col1 - 1))
8.2 Range Update, Point Query
While the standard BIT supports point updates and range queries, it’s possible to modify it to support range updates and point queries. This is achieved by storing differences between consecutive elements instead of the actual values.
8.3 Order Statistic Tree
By using a BIT to count the number of elements, we can implement an Order Statistic Tree, which can find the k-th smallest element in a dynamic set efficiently.
8.4 Persistent Binary Indexed Tree
A persistent version of BIT allows querying previous versions of the data structure after each update, which can be useful in certain algorithmic problems.
9. Practice Problems
To solidify your understanding of Binary Indexed Trees, here are some practice problems from various online judges:
- SPOJ – Inversion Count: A classic problem that can be efficiently solved using a BIT.
- Codeforces – Multiset: This problem requires a creative use of BIT for both insertion and deletion operations.
- LeetCode – Range Sum Query – Mutable: A straightforward application of BIT for range sum queries with updates.
- HackerEarth – Help Ashu: This problem combines BIT with parity checking.
- CodeChef – Spread the Word: A challenging problem that requires a 2D BIT implementation.
Working through these problems will help you gain practical experience in implementing and applying Binary Indexed Trees to solve various algorithmic challenges.
10. Conclusion
Binary Indexed Trees are a powerful and elegant data structure that provide an efficient solution for range query problems. Their simplicity in implementation, coupled with their logarithmic time complexity for both updates and queries, makes them an invaluable tool in a programmer’s arsenal.
As you continue your journey in algorithmic problem-solving and competitive programming, mastering the Binary Indexed Tree will undoubtedly enhance your ability to tackle a wide range of problems efficiently. Remember that practice is key to fully grasping the nuances of this data structure and its applications.
Whether you’re preparing for technical interviews, participating in programming contests, or working on real-world applications that require efficient range queries, the knowledge of Binary Indexed Trees will serve you well. Keep exploring, practicing, and pushing the boundaries of what you can achieve with this remarkable data structure!