Big-O Notation Practice: Enhancing Your Problem-Solving Skills
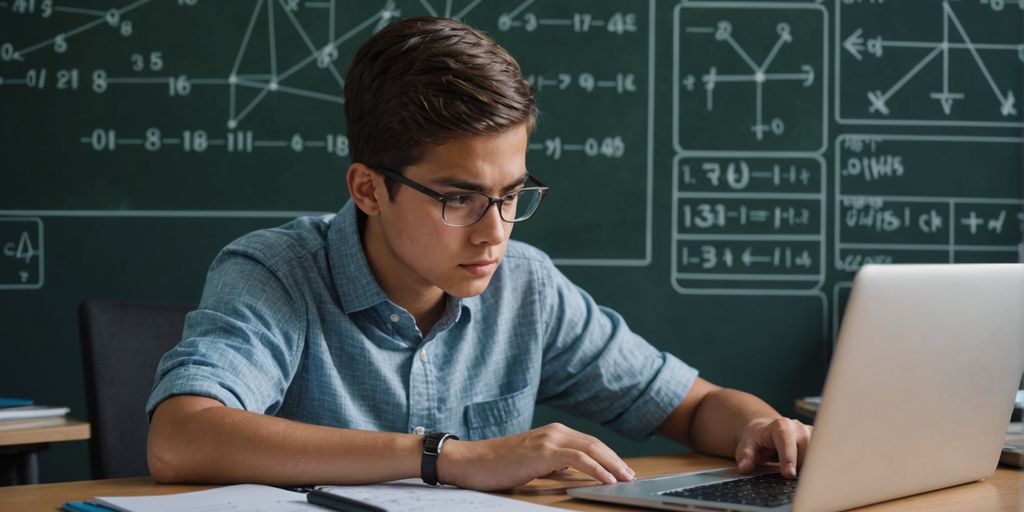
Big-O notation might sound tricky, but it’s a super helpful tool for understanding how fast or slow an algorithm runs. Whether you’re new to coding or have some experience, knowing Big-O can make your code better and faster. Let’s dive into what Big-O is all about and how it can boost your problem-solving skills.
Key Takeaways
- Big-O notation helps you understand the efficiency of algorithms as data size grows.
- Different types of Big-O notations, like O(1) and O(n), describe how an algorithm’s run time changes.
- Big-O is essential for optimizing search and sorting algorithms.
- Knowing Big-O can give you an edge in coding competitions and technical interviews.
- There are many resources, like online platforms and books, to practice and master Big-O notation.
Understanding the Basics of Big-O Notation
Big-O notation is a mathematical representation used to describe the upper bound of an algorithm’s running time, helping developers compare the efficiency of different algorithms. It provides a standardized way to express how an algorithm’s performance scales as the input size grows.
Defining Big-O Notation
Big-O notation is used to describe the performance or complexity of an algorithm. Specifically, it describes the worst-case scenario in terms of time or space complexity. For any function f(n), if there exist constants c > 0 and n0 >= 0 such that f(n) <= cg(n) for all n >= n0, then f(n) is O(g(n)). In simpler terms, f(n) grows no faster than cg(n) for all n >= n0 where c and n0 are constants.
Importance of Big-O in Algorithm Analysis
Big-O notation is important for several reasons:
- Big-O notation helps analyze the efficiency of algorithms.
- It provides a way to describe how the runtime or space requirements of an algorithm grow as the input size increases.
- Allows programmers to compare different algorithms and choose the most efficient one for a specific problem.
- Helps in understanding the scalability of algorithms and predicting how they will perform as the input size grows.
- Enables developers to optimize code and improve overall performance.
Common Misconceptions About Big-O
One common misconception is that Big-O can be used to compare the speed of two algorithms directly. Big-O only indicates how the runtime or space requirements grow with the input size. For example, an algorithm with O(n^2) complexity might be faster than an O(log n) algorithm for small input sizes, but as the input size grows, the O(log n) algorithm will eventually outperform the O(n^2) algorithm.
Understanding Big-O notation is crucial for analyzing and designing efficient algorithms. It helps developers make informed decisions about memory usage, processing power, and other resources.
Common Big-O Notations and Their Implications
Understanding different Big-O notations is crucial for analyzing the efficiency of algorithms. Here, we will explore some common Big-O notations and their implications.
O(1) – Constant Time Complexity
An algorithm with O(1) complexity runs in constant time, regardless of the input size. This means that the execution time remains the same, no matter how large the input is. This is the most efficient time complexity because it doesn’t grow with the input size.
O(n) – Linear Time Complexity
O(n) complexity means that the execution time of the algorithm grows linearly with the input size. If the input size doubles, the execution time also doubles. This is common in algorithms that involve a single loop through the input data.
O(n^2) – Quadratic Time Complexity
Algorithms with O(n^2) complexity have their execution time grow quadratically as the input size increases. This is typical in algorithms with nested loops, where each element in the input is compared with every other element. As a result, these algorithms can become very slow with large inputs.
O(log n) – Logarithmic Time Complexity
O(log n) complexity indicates that the execution time grows logarithmically as the input size increases. This is often seen in algorithms that repeatedly divide the input in half, such as binary search. These algorithms are efficient for large datasets because the time complexity increases very slowly compared to the input size.
Big-O notation represents the upper bound of the running time of an algorithm. Therefore, it gives the worst-case complexity of an algorithm.
Practical Applications of Big-O Notation
Optimizing Search Algorithms
When developing software, engineers often have to choose between multiple algorithms to solve a particular problem. Big-O notation helps them select the most efficient algorithm by comparing their time and space complexities. For example, in search algorithms, understanding whether an algorithm operates in O(1), O(n), or O(log n) time can significantly impact performance, especially with large datasets.
Improving Sorting Techniques
Sorting is a fundamental operation in computer science, and different algorithms have varying efficiencies. Big-O notation allows developers to compare these algorithms and choose the best one for their needs. For instance, while Bubble Sort has a time complexity of O(n^2), Quick Sort operates in O(n log n) on average, making it more suitable for larger datasets.
Real-World Examples of Big-O in Action
Big-O notation is not just theoretical; it has practical applications in real-world scenarios. In software development, it helps in algorithm selection and performance optimization. In database systems, it aids in query optimization and indexing strategies. Additionally, in system design, it assists in scalability analysis and resource allocation. Understanding the real-world applications of Big-O notation can lead to more efficient and effective solutions across various domains.
Big-O notation is a crucial tool for software engineers, helping them make informed decisions about algorithm efficiency and system performance.
Techniques for Analyzing Algorithm Complexity
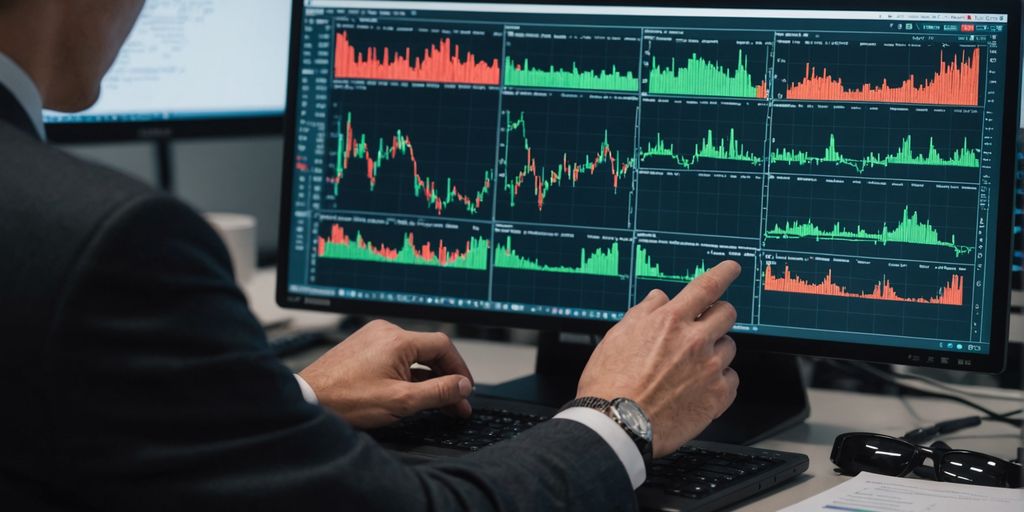
Asymptotic Analysis
Asymptotic analysis helps you understand how an algorithm behaves as the input size grows towards infinity. It’s like watching your code from a helicopter view to see how it performs in the long run. This method helps predict the time or space needed for large data sizes. For example, sorting two items is quick, but what about sorting two million items? Asymptotic analysis gives you a high-level understanding of the scaling.
Worst-Case vs. Average-Case Analysis
When measuring the complexity of an algorithm, it’s important to consider different scenarios:
- Worst-case analysis: This is mostly used and considers the maximum time or space an algorithm will take.
- Best-case analysis: This is very rarely used and looks at the minimum time or space required.
- Average-case analysis: This is rarely used and calculates the expected time or space for a typical input.
Space Complexity Considerations
Space complexity refers to the amount of memory an algorithm uses as a function of the input size. It helps understand how much memory is needed to store data and execute operations. Similar to time complexity, space complexity is also expressed using Big O notation to describe the upper bound of the algorithm’s memory usage.
- O(1): Constant space complexity, meaning the algorithm uses a fixed amount of memory regardless of the input size.
- O(n): Linear space complexity, where memory usage grows linearly with the input size.
- O(n^2): Quadratic space complexity, where memory usage grows quadratically with the input size.
Analyzing space complexity is essential for understanding an algorithm’s memory requirements, optimizing memory usage, and ensuring efficient resource utilization, especially in memory-constrained environments.
Big-O Notation in Competitive Programming
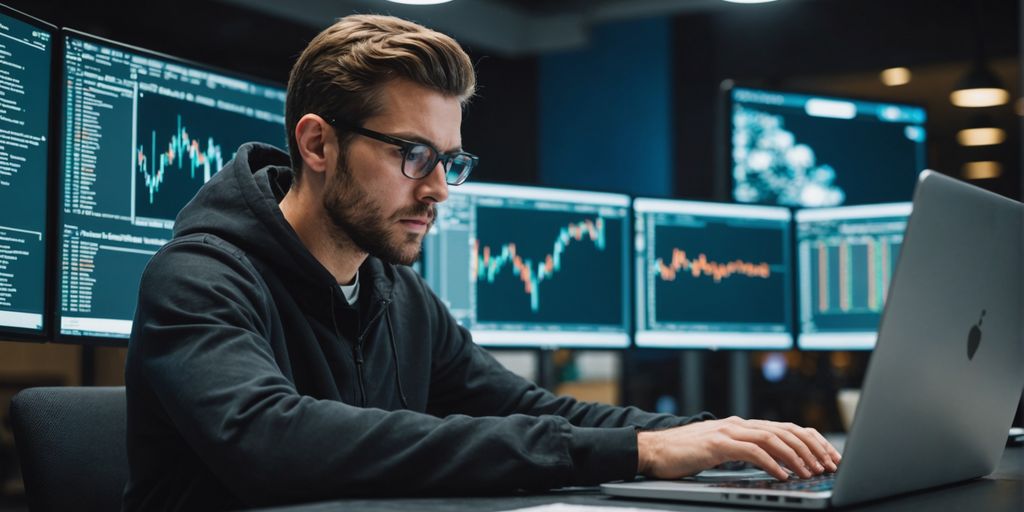
Importance in Coding Competitions
In coding competitions, understanding Big-O notation is crucial. It helps you quickly evaluate the efficiency of your solutions. Knowing the time complexity of your code can be the difference between passing all test cases and failing due to time limits.
Common Problems and Their Big-O Solutions
Here are some common problems and their typical Big-O solutions:
- Sorting Algorithms: QuickSort (O(n log n)), MergeSort (O(n log n)), BubbleSort (O(n^2))
- Search Algorithms: Binary Search (O(log n)), Linear Search (O(n))
- Graph Algorithms: Dijkstra’s Algorithm (O(V^2)), Depth-First Search (O(V + E))
Tips for Efficient Problem Solving
- Analyze the Problem: Understand the problem constraints and requirements.
- Choose the Right Data Structures: Use data structures that offer the best time complexity for the operations you need.
- Optimize Your Code: Look for ways to reduce the time complexity, such as avoiding nested loops or using efficient algorithms.
- Practice: Regular practice helps you recognize patterns and improve your problem-solving speed.
In competitive programming, worst-case analysis is often used to ensure your solution will run efficiently under all conditions.
Advanced Topics in Big-O Notation
Amortized Analysis
Amortized analysis is a method used to average the time complexity of an algorithm over a sequence of operations. Instead of looking at the worst-case time for a single operation, it considers the average time per operation in the worst-case scenario. This is particularly useful for algorithms where occasional operations are expensive, but most are cheap. An example is the dynamic array, where resizing happens infrequently but can be costly.
Probabilistic Analysis
Probabilistic analysis involves using probability to analyze the performance of an algorithm. This type of analysis is useful when the input is random or when the algorithm itself uses randomization. It helps in understanding the expected time complexity rather than the worst-case scenario. For instance, quicksort has an average-case time complexity of O(n log n) when the pivot is chosen randomly.
Big-Omega and Big-Theta Notations
Big-Omega (Ω) notation provides a lower bound on the time complexity of an algorithm, indicating the best-case scenario. On the other hand, Big-Theta (Θ) notation gives a tight bound, meaning it describes both the upper and lower bounds. These notations are essential for a comprehensive understanding of an algorithm’s performance. They help in comparing different algorithms more effectively by providing a complete picture of their efficiency.
Understanding these advanced topics in Big-O notation can significantly enhance your problem-solving skills and help you design more efficient algorithms.
Tools and Resources for Practicing Big-O Notation
Online Platforms for Coding Practice
To get better at Big-O notation, you can use various online platforms. Websites like HackerRank, LeetCode, and CodeSignal offer coding challenges that help you understand and apply Big-O concepts. These platforms often provide instant feedback on your solutions, which is crucial for learning.
Recommended Books and Courses
Books and courses are excellent resources for deepening your understanding of Big-O notation. Some highly recommended books include "Introduction to Algorithms" by Cormen, Leiserson, Rivest, and Stein, and "Grokking Algorithms" by Aditya Bhargava. Online courses from platforms like Coursera and Udacity also offer structured learning paths.
Community and Forum Discussions
Engaging with a community can significantly enhance your learning experience. Websites like Stack Overflow, Reddit, and specialized forums offer a space to ask questions, share insights, and discuss problems related to Big-O notation. Participating in these discussions can provide practical insights and different perspectives on complex topics.
Big-O notation is more than just a theoretical concept; it is a practical tool for anyone working with data and algorithms. It helps you predict performance and optimize your code effectively.
By leveraging these tools and resources, you can improve your problem-solving skills and gain a deeper understanding of Big-O notation.
Conclusion
Big-O notation is more than just a theoretical concept; it’s a practical tool that can significantly enhance your problem-solving skills. By understanding and applying Big-O notation, you can write more efficient code, optimize algorithms, and make informed decisions about which solutions are best suited for different problems. Whether you’re preparing for coding interviews, working on complex projects, or just aiming to improve your coding skills, mastering Big-O notation is essential. Keep practicing, stay curious, and you’ll find that your ability to tackle challenging problems will improve dramatically.
Frequently Asked Questions
What is Big-O notation?
Big-O notation is a way to describe how the time or space requirements of an algorithm grow as the input size increases. It’s like a measuring stick for understanding how an algorithm performs as the data gets bigger.
Why is Big-O notation important?
Big-O notation helps us understand the efficiency of algorithms. It lets us compare different algorithms and pick the best one for a problem, ensuring that our code runs faster and uses less memory.
Can you give a real-life example of using Big-O notation?
Sure! Imagine you’re searching for a name in a phone book. If you start from the beginning and go one by one, that’s O(n) time complexity. But if you use a more efficient method, like binary search, you can do it in O(log n) time.
What does O(1) mean?
O(1) means constant time complexity. This means that the time it takes to run the algorithm doesn’t change with the size of the input. It’s like flipping a light switch; it takes the same amount of time no matter how many lights you have.
How is Big-O notation used in coding competitions?
In coding competitions, Big-O notation helps participants write efficient code that runs within time limits. Understanding it can give you an edge in solving problems faster and more effectively.
What’s the difference between worst-case and average-case analysis?
Worst-case analysis looks at the maximum time an algorithm could take, while average-case analysis considers the typical time for random inputs. Both are important for understanding an algorithm’s performance.