Why You’re Solving 100 LeetCode Problems but Still Failing Tech Interviews
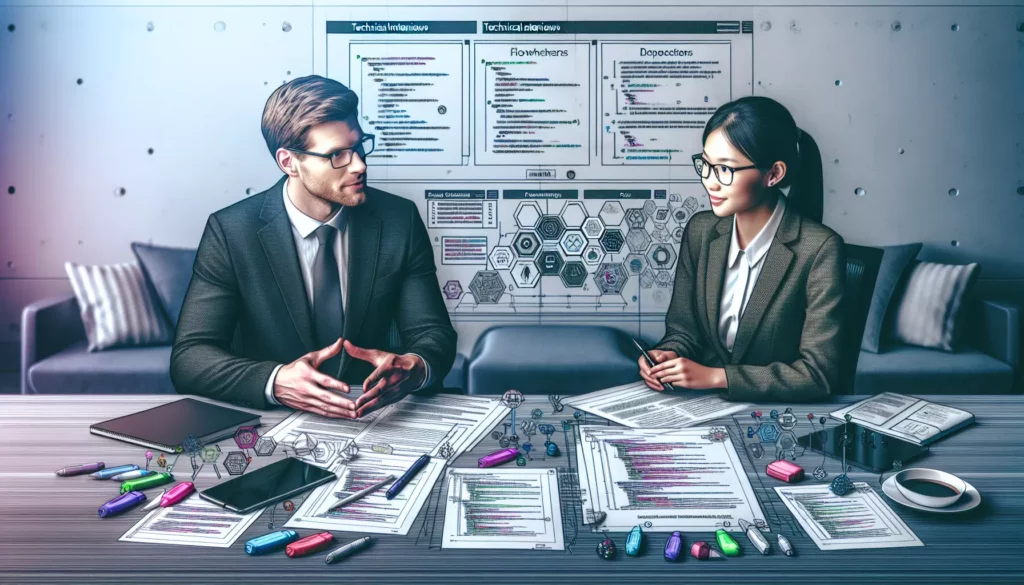
The LeetCode Paradox: Mastering Problems But Failing Interviews
You’ve spent countless hours grinding through LeetCode problems. You’ve solved easy ones, tackled medium challenges, and even conquered some of the hard-rated algorithmic puzzles. Your profile proudly displays 100+ solved problems. Yet somehow, when you sit down for technical interviews, you still struggle. The coding challenges that seemed manageable in practice suddenly become insurmountable obstacles when a hiring manager is watching.
If this sounds familiar, you’re experiencing what I call the “LeetCode Paradox” – the frustrating reality that solving practice problems doesn’t automatically translate to interview success.
This disconnect between practice performance and interview results leaves many developers confused, frustrated, and questioning their abilities. But here’s the truth: technical interviews assess far more than just your ability to solve algorithmic puzzles.
In this comprehensive guide, we’ll explore why solving 100+ LeetCode problems might not be enough to land your dream job, and more importantly, what you can do about it. We’ll uncover the hidden skills that interviewers are actually looking for and provide actionable strategies to bridge the gap between LeetCode mastery and interview success.
The Limitations of LeetCode as Interview Preparation
Before we dive into solutions, let’s understand why LeetCode alone isn’t sufficient preparation for technical interviews.
Problem #1: LeetCode Trains Pattern Recognition, Not Problem-Solving
LeetCode is excellent for teaching you common algorithm patterns. After solving dozens of problems, you start to recognize when to use a two-pointer approach, dynamic programming, or a breadth-first search. This pattern recognition is valuable but fundamentally different from the problem-solving process required in interviews.
In a real interview, you won’t be told “This is a sliding window problem” or “Use a hash map here.” You’ll need to analyze an unfamiliar problem from scratch, recognize which techniques might apply, and explain your reasoning along the way.
Problem #2: The Isolated Environment vs. Interactive Setting
When you solve problems on LeetCode, you’re in a comfortable, low-pressure environment. You can:
- Take as much time as you need
- Search for hints when stuck
- Submit multiple attempts without judgment
- Focus entirely on getting the correct answer
Contrast this with a technical interview, where you must:
- Think aloud while solving the problem
- Engage with the interviewer’s questions and hints
- Manage time pressure and anxiety
- Communicate your thought process clearly
- Write clean, error-free code on the first attempt
These are fundamentally different experiences that require different skill sets.
Problem #3: LeetCode Emphasizes Solutions Over Process
On LeetCode, the end result (a working solution) is all that matters. The platform rewards optimal solutions with fast runtimes and minimal memory usage. But in interviews, your problem-solving process is often more important than the final code you produce.
Interviewers want to see:
- How you approach unfamiliar problems
- Your ability to break down complex tasks
- Whether you consider edge cases
- How you test and debug your code
- If you can optimize an initial solution
Even if you arrive at the correct solution, failing to demonstrate a strong process can lead to rejection.
The Hidden Skills Technical Interviewers Actually Evaluate
Technical interviews are designed to assess much more than algorithmic knowledge. Here are the critical skills that LeetCode doesn’t directly teach:
Communication Skills: The Most Underrated Interview Component
The ability to clearly explain your thought process is often the difference between success and failure in technical interviews. Strong communication during coding interviews includes:
- Clarifying requirements: Asking thoughtful questions about the problem statement
- Thinking aloud: Verbalizing your approach before and during coding
- Explaining tradeoffs: Discussing why you chose one approach over alternatives
- Using proper terminology: Demonstrating fluency with computer science concepts
Many candidates who excel at solving LeetCode problems fail interviews because they code in silence or can’t articulate their reasoning effectively.
Problem Decomposition: Breaking Down Complex Tasks
Real-world software engineering requires breaking complex problems into manageable components. Interviewers evaluate your ability to:
- Identify the core challenges within a larger problem
- Create a structured plan before diving into code
- Separate concerns (e.g., data processing, algorithm, output formatting)
- Recognize when to use helper functions or separate classes
This systematic approach to problem-solving is rarely practiced when rushing through LeetCode problems.
Testing and Debugging Mindset
Interviewers want to see that you write reliable code and can identify/fix bugs efficiently. This includes:
- Proactively identifying edge cases
- Creating comprehensive test cases
- Tracing through your code with example inputs
- Efficiently debugging when errors arise
- Verifying correctness after implementation
On LeetCode, the platform handles testing for you and provides feedback on failed test cases. In interviews, you must demonstrate this quality assurance mindset yourself.
Adaptability and Coachability
Interviewers aren’t just evaluating your current skills—they’re assessing how you might perform as a team member. This includes your ability to:
- Respond positively to hints and feedback
- Pivot when your initial approach isn’t working
- Incorporate the interviewer’s suggestions
- Remain composed when facing challenges
These interpersonal qualities don’t develop through solo LeetCode practice but are crucial for interview success.
Common Interview Failure Patterns Despite LeetCode Success
Let’s examine specific ways that LeetCode experts still fail technical interviews and what these patterns reveal about the interview process.
The Silent Coder: Failing to Communicate
The scenario: A candidate immediately starts coding after hearing the problem, working silently for 20+ minutes before presenting a solution. Even if the solution is correct, this approach often leads to rejection.
Why it fails: The interviewer gains no insight into the candidate’s thought process, problem-solving approach, or reasoning abilities. They can’t assess how the candidate might collaborate with team members or approach unfamiliar problems.
What interviewers think: “They might be able to code, but can they work effectively with others? Would they disappear for hours when tackling a problem instead of collaborating?”
The Brute Force Rusher: Skipping the Planning Phase
The scenario: A candidate immediately implements the first solution that comes to mind without considering efficiency, alternatives, or edge cases. They may produce working code but miss opportunities for optimization.
Why it fails: This demonstrates poor judgment and planning skills. In real-world engineering, rushing into implementation without proper planning leads to technical debt and inefficient solutions.
What interviewers think: “Would this person create maintenance nightmares by implementing the first solution that comes to mind without considering alternatives?”
The Algorithm Reciter: Memorization Without Understanding
The scenario: The candidate recognizes the problem category and recites a textbook solution from memory, but struggles when asked to explain why certain decisions were made or how to modify the algorithm for slightly different requirements.
Why it fails: It reveals shallow understanding rather than genuine problem-solving ability. Software engineering requires adapting known patterns to novel situations, not just applying memorized solutions.
What interviewers think: “This person might handle standard problems but would likely struggle with the unique challenges our team faces.”
The Edge Case Ignorer: Incomplete Solutions
The scenario: The candidate produces a solution that works for the main test case but fails to consider edge cases like empty inputs, negative numbers, or boundary conditions until prompted by the interviewer.
Why it fails: It suggests a lack of thoroughness and quality consciousness. Production code must be robust across all possible inputs, not just the happy path.
What interviewers think: “Would this candidate’s code cause production incidents because they didn’t thoroughly test their solutions?”
Bridging the Gap: From LeetCode Expert to Interview Success
Now that we understand the limitations of LeetCode preparation, let’s explore practical strategies to develop the full spectrum of skills needed for interview success.
Strategy #1: Simulate Real Interview Conditions
The most effective way to prepare for technical interviews is to practice under conditions that closely mimic the real experience:
Practice Thinking Aloud
Even when solving LeetCode problems alone, verbalize your thought process out loud. Explain:
- How you’re understanding the problem
- What approaches you’re considering
- Why you’re choosing a particular solution
- The time and space complexity of your approach
This may feel awkward at first, but it builds the verbal reasoning muscles needed for interviews.
Use Mock Interviews
Platforms like Pramp, interviewing.io, and mock interviews with peers provide invaluable real-world practice. These experiences force you to:
- Code under time pressure
- Explain your reasoning to another person
- Handle unexpected questions and feedback
- Practice the social dynamics of interviews
Aim for at least 5-10 mock interviews before your actual interviews begin.
Record and Review Your Practice
If possible, record your mock interviews or practice sessions. Watching yourself solve problems reveals:
- Communication patterns you may not be aware of
- Areas where your explanations lack clarity
- Non-verbal cues that might distract interviewers
- Inefficiencies in your problem-solving approach
This self-assessment can be uncomfortable but leads to rapid improvement.
Strategy #2: Focus on Process Over Solutions
Rather than rushing to solve as many LeetCode problems as possible, focus on developing a systematic problem-solving process that you can apply consistently:
The 5-Step Interview Framework
For each practice problem, follow this structured approach:
- Understand and clarify: Restate the problem, ask clarifying questions, and confirm requirements
- Explore approaches: Discuss multiple potential solutions before selecting one
- Plan before coding: Outline your algorithm in pseudocode or with diagrams
- Implement with narration: Write clean code while explaining your implementation choices
- Test and refine: Review your code, test with various inputs, and optimize if needed
This framework ensures you demonstrate the full range of skills interviewers are looking for, not just coding ability.
Here’s how this might look in practice:
// Step 1: Understand and clarify
"So I'm being asked to find the longest substring without repeating characters.
Let me confirm my understanding with an example: for 'abcabcbb', the answer would be 3,
corresponding to the substring 'abc'. I need to return the length, not the substring itself.
Are there any constraints on the input string? Could it be empty?"
// Step 2: Explore approaches
"I see a few ways to approach this. We could use a brute force method and check every
possible substring, but that would be O(n³) time complexity. Alternatively, we could use
a sliding window approach with a hash set to track characters we've seen, which would
give us O(n) time complexity. I think the sliding window approach is more efficient."
// Step 3: Plan before coding
"I'll use two pointers to represent our window: left and right. As I move the right pointer,
I'll add characters to my hash set. If I encounter a character that's already in the set,
I'll move the left pointer forward, removing characters from the set until the duplicate
is gone. Throughout this process, I'll track the maximum window size."
// Step 4: Implement with narration
"Let me code this solution now..."
function lengthOfLongestSubstring(s) {
let charSet = new Set();
let left = 0;
let maxLength = 0;
for (let right = 0; right < s.length; right++) {
// If we find a duplicate, move left pointer until duplicate is removed
while (charSet.has(s[right])) {
charSet.delete(s[left]);
left++;
}
// Add current character to set
charSet.add(s[right]);
// Update max length if current window is larger
maxLength = Math.max(maxLength, right - left + 1);
}
return maxLength;
}
// Step 5: Test and refine
"Let me trace through this with the example 'abcabcbb'..."
// (walks through the algorithm step by step)
"The function returns 3, which matches our expected output. Let me check some edge cases:
- Empty string: returns 0 ✓
- Single character: returns 1 ✓
- All same characters ('aaa'): returns 1 ✓
- No repeating characters ('abcdef'): returns 6 ✓
The time complexity is O(n) where n is the string length, as we traverse the string once.
Space complexity is O(min(m,n)) where m is the size of the character set."
Quality Over Quantity
Instead of racing through 100+ problems, deeply analyze 30-40 problems covering different algorithm patterns. For each problem:
- Solve it using the 5-step framework
- Implement multiple approaches (brute force, optimized)
- Analyze the tradeoffs between different solutions
- Create your own test cases, including edge cases
- Explain your solution as if teaching someone else
This deeper engagement builds stronger problem-solving skills than superficial exposure to many problems.
Strategy #3: Develop Your Communication Skills
Technical communication is a skill that can be deliberately practiced and improved:
Learn to Explain Complex Concepts Simply
After solving a problem, practice explaining your solution to someone without a technical background. This forces you to:
- Distill complex ideas to their essence
- Use clear, jargon-free language
- Create helpful analogies and visualizations
- Focus on the "why" behind your approach
This skill directly translates to interview success, as interviewers value clear communication highly.
Practice Written Communication
For some LeetCode problems, write detailed explanations of your solutions, including:
- The problem-solving approach
- Why you chose specific data structures
- Time and space complexity analysis
- Potential optimizations and tradeoffs
This writing practice helps organize your thoughts and develop precise technical language.
Master Whiteboarding Techniques
Even in virtual interviews, the ability to visually communicate your ideas is valuable:
- Practice drawing clear diagrams to illustrate data structures
- Use visual aids to explain algorithm steps
- Develop a consistent notation system for your explanations
- Maintain organized, readable code with proper indentation and spacing
These visual communication skills complement verbal explanations and demonstrate professionalism.
Strategy #4: Develop a Testing and Debugging Mindset
Strong engineers don't just write code—they ensure it works correctly under all conditions:
Create Comprehensive Test Cases
For each LeetCode problem, before submitting your solution, create your own test cases that cover:
- Normal/expected inputs
- Edge cases (empty arrays, single elements, etc.)
- Boundary conditions (minimum/maximum values)
- Invalid inputs (if applicable)
- Performance tests with large inputs
This practice develops the testing mindset that interviewers look for.
Narrate Your Debugging Process
When your code doesn't work as expected, practice verbalizing your debugging approach:
- Identify specific test cases that fail
- Form hypotheses about potential issues
- Use print statements or step through code mentally
- Systematically isolate and fix bugs
- Verify fixes with additional testing
Debugging skills demonstrate resilience and problem-solving abilities that extend beyond coding.
Beyond LeetCode: Holistic Interview Preparation
While algorithmic problem-solving is important, technical interviews often include other components that require specific preparation:
System Design Interviews: The Senior Engineer Test
As you advance in your career, system design questions become increasingly important. These open-ended discussions evaluate your ability to:
- Design scalable, resilient systems
- Make appropriate technology choices
- Consider performance, reliability, and security
- Communicate architectural decisions clearly
Resources like "System Design Interview" by Alex Xu, Grokking the System Design Interview, and the System Design Primer on GitHub can help you prepare for these discussions.
Behavioral Questions: Your Professional Story
Technical skills alone won't secure job offers. Prepare for behavioral questions that assess your:
- Past experiences and accomplishments
- Collaboration and conflict resolution skills
- Problem-solving approach in real-world scenarios
- Alignment with company values and culture
Use the STAR method (Situation, Task, Action, Result) to structure compelling responses to behavioral questions.
Company-Specific Knowledge: Demonstrating Interest
Research each company you interview with to understand:
- Their products, services, and business model
- Technical challenges specific to their industry
- Recent news, updates, or product launches
- Their engineering culture and values
This knowledge allows you to ask insightful questions and tailor your responses to the company's context.
Learning from Interview Failures: The Growth Mindset
Even with excellent preparation, you may face rejections. How you respond to these setbacks often determines your long-term success:
Request and Analyze Feedback
Whenever possible, ask for specific feedback after interviews:
- What areas could you improve in?
- Were there specific questions or problems you struggled with?
- Did your communication style or approach raise concerns?
This feedback, though sometimes difficult to hear, provides invaluable guidance for improvement.
Identify Patterns in Interview Performance
After several interviews, look for consistent themes in your performance:
- Do you consistently struggle with certain algorithm types?
- Do you run out of time on coding challenges?
- Is your communication clear during pressure situations?
- Do you handle unexpected questions or hints effectively?
These patterns reveal specific areas for focused improvement.
Reframe Rejections as Learning Opportunities
Rather than viewing rejections as failures, see them as valuable data points:
- Each interview provides practice in high-pressure situations
- Exposure to different problem types expands your knowledge
- Feedback highlights blind spots you might not have noticed
- The interview process itself becomes less intimidating with experience
This growth mindset keeps you resilient and focused on continuous improvement.
Case Studies: From LeetCode Expert to Interview Success
Let's examine two hypothetical but realistic scenarios that illustrate the journey from LeetCode mastery to interview success:
Case Study #1: Sarah's Transformation
Background: Sarah had solved 150+ LeetCode problems and could recognize most common patterns, but repeatedly failed technical interviews despite providing correct solutions.
The Problem: Through mock interviews, Sarah discovered she was diving into code too quickly without explaining her thought process. Her silent coding style made interviewers unsure of her reasoning abilities.
The Solution: Sarah focused on the following changes:
- Started each problem by restating it and asking clarifying questions
- Verbalized multiple approaches before choosing one
- Narrated her code implementation line by line
- Proactively discussed time and space complexity
- Practiced with 15 mock interviews to build these habits
The Result: After two months of focused practice on communication, Sarah received offers from two tech companies, with interviewers specifically praising her clear thinking and collaborative approach.
Case Study #2: Michael's Edge Case Epiphany
Background: Michael had completed 120+ LeetCode problems with optimal solutions but received feedback that his code was "brittle" and "lacked thoroughness."
The Problem: While Michael could solve the core algorithmic challenges, he rarely considered edge cases until prompted. His solutions often failed on empty arrays, boundary values, or unusual inputs.
The Solution: Michael developed a systematic testing process:
- Created a checklist of common edge cases to verify for each problem
- Implemented a "test-first" approach, writing test cases before coding
- Practiced talking through his testing strategy during mock interviews
- Developed the habit of manually tracing code execution with diverse inputs
The Result: Michael's interview performance improved dramatically. In his successful interview with a financial technology company, the interviewer noted his "exceptional attention to detail" and "rigorous testing approach."
Final Thoughts: Beyond the LeetCode Grind
LeetCode and similar platforms are valuable tools for learning algorithms and data structures, but they're just one component of interview preparation. Technical interviews assess your potential as a colleague and problem solver, not just your ability to solve puzzles.
Remember these key principles as you prepare:
- Process matters more than solutions. How you approach problems is often more important than whether you solve them perfectly.
- Communication transforms good coders into great candidates. Your ability to explain your thinking clearly can compensate for technical imperfections.
- Interview skills are distinct from coding skills. The social, communicative aspects of interviews require deliberate practice.
- Quality beats quantity. Deep understanding of fundamental patterns trumps exposure to hundreds of problems.
- Growth comes from feedback and reflection. Each interview, successful or not, provides data for improvement.
By expanding your preparation beyond algorithm puzzles to include communication, testing, and holistic problem-solving, you'll transform from a LeetCode expert into an interview success story. The skills you develop through this process won't just help you pass interviews—they'll make you a more effective software engineer throughout your career.
Remember that interviewing is itself a skill that improves with practice. Stay persistent, keep refining your approach, and trust that your dedication will eventually lead to the right opportunity. Your next technical interview might just be the one where everything clicks into place.