Best Websites to Practice JavaScript Questions: A Comprehensive Guide
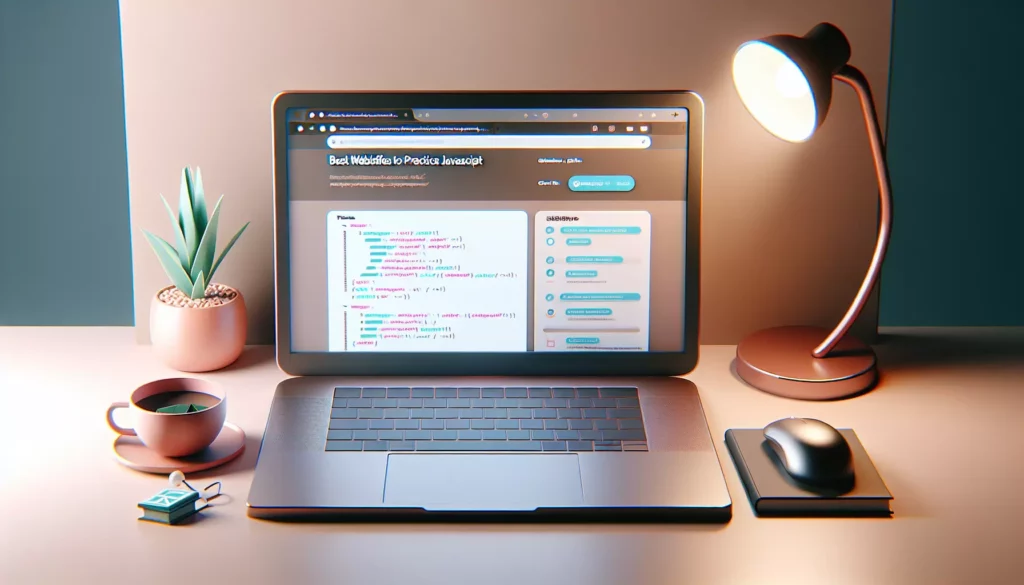
JavaScript has become an essential language for web development, and mastering it requires consistent practice and exposure to various programming challenges. Whether you’re a beginner looking to solidify your fundamentals or an experienced developer preparing for technical interviews, having access to quality JavaScript practice questions is crucial. In this comprehensive guide, we’ll explore some of the best websites to hone your JavaScript skills, with a special focus on AlgoCademy, a platform that stands out for its unique features and comprehensive approach to coding education.
1. AlgoCademy: Your One-Stop Solution for JavaScript Practice
When it comes to practicing JavaScript questions and preparing for technical interviews, AlgoCademy emerges as a top contender. This platform offers a unique blend of features that cater to learners at various skill levels, from beginners to those aiming for positions at major tech companies.
Key Features of AlgoCademy:
- Interactive Coding Tutorials: AlgoCademy provides hands-on coding experiences that allow you to write and execute JavaScript code directly in your browser.
- AI-Powered Assistance: Get personalized help and hints as you work through challenging problems, mimicking a one-on-one tutoring experience.
- Comprehensive Problem Sets: From basic JavaScript concepts to advanced algorithmic challenges, AlgoCademy covers a wide range of topics.
- FAANG-Focused Preparation: Tailored content to help you prepare for interviews at top tech companies like Facebook, Amazon, Apple, Netflix, and Google.
- Progress Tracking: Monitor your improvement over time with detailed analytics and performance metrics.
- Community Support: Engage with other learners, share solutions, and participate in discussions to enhance your learning experience.
What sets AlgoCademy apart is its focus on developing not just coding skills, but also problem-solving abilities and algorithmic thinking. This holistic approach ensures that you’re not just memorizing solutions, but truly understanding the underlying concepts and logic.
How to Get Started with AlgoCademy:
- Sign up for an account on the AlgoCademy website.
- Take the initial assessment to determine your current skill level.
- Follow the personalized learning path created based on your assessment results.
- Practice daily with a mix of tutorials, coding challenges, and mock interview questions.
- Utilize the AI assistant when you’re stuck or need additional explanations.
- Participate in the community forums to discuss problems and share insights.
By consistently using AlgoCademy, you’ll not only improve your JavaScript skills but also develop a strong foundation in computer science principles and problem-solving techniques essential for succeeding in technical interviews.
2. LeetCode: A Classic Choice for Algorithm Practice
LeetCode is a well-known platform among developers preparing for technical interviews. While it covers multiple programming languages, it offers an extensive collection of JavaScript problems.
Highlights of LeetCode:
- Large problem database with varying difficulty levels
- Company-specific question sets
- Contests and competitions to test your skills
- Discussion forums for each problem
- Performance statistics to track your progress
LeetCode is particularly useful for those focusing on algorithmic problem-solving and data structures. Its problems are often similar to those asked in technical interviews at large tech companies.
3. HackerRank: Diverse Challenges and Skill Certifications
HackerRank offers a wide range of programming challenges, including a dedicated JavaScript track. It’s popular among both job seekers and employers for its skill assessment capabilities.
Key Features of HackerRank:
- Skill-based challenges and certifications
- Coding contests and hackathons
- Interview preparation kits
- Integrated development environment (IDE) for coding
- Leaderboards to compare your performance with others
HackerRank is an excellent platform for those looking to validate their JavaScript skills through certifications and participate in coding competitions.
4. Codewars: Learn Through Community-Driven Challenges
Codewars takes a unique approach to coding practice by offering “kata” – coding challenges created and solved by its community members.
What Makes Codewars Special:
- Community-created challenges ensure diverse and interesting problems
- Ranking system to track your progress
- Ability to compare your solutions with others after solving a problem
- Translations of problems in multiple programming languages
- Gamified learning experience with achievements and honors
Codewars is great for those who enjoy a more collaborative and community-driven learning environment.
5. freeCodeCamp: Comprehensive Curriculum with Real-World Projects
freeCodeCamp offers a comprehensive, project-based approach to learning JavaScript and web development. While it’s not exclusively focused on practice questions, it provides a solid foundation and practical experience.
freeCodeCamp’s Offerings:
- Full curriculum covering JavaScript fundamentals to advanced topics
- Interactive coding challenges integrated into lessons
- Real-world projects to build a portfolio
- Certifications upon completion of curriculum tracks
- Active community forum for support and discussions
freeCodeCamp is ideal for beginners who want a structured learning path and hands-on experience building projects.
6. Edabit: Bite-Sized Coding Challenges
Edabit focuses on providing short, engaging coding challenges that are perfect for daily practice.
Why Choose Edabit:
- Quick, bite-sized challenges that can be completed in minutes
- Gamified learning with XP and achievements
- Challenges range from very easy to expert level
- Explanations and resources provided for each challenge
- Ability to create and share your own challenges
Edabit is great for those who want to practice regularly but may not have large blocks of time to dedicate to coding.
7. JavaScript30: 30 Day Vanilla JS Coding Challenge
JavaScript30 is a unique, project-based course created by Wes Bos. While not a traditional practice question platform, it offers hands-on experience with pure JavaScript.
What JavaScript30 Offers:
- 30 different projects built with vanilla JavaScript
- Video tutorials explaining each project
- Focus on practical, real-world applications
- No frameworks, compilers, libraries, or boilerplate
- Free and accessible to all skill levels
JavaScript30 is excellent for intermediate learners looking to strengthen their core JavaScript skills through project-based learning.
Maximizing Your JavaScript Practice
While these platforms offer excellent resources for practicing JavaScript, it’s essential to approach your learning strategically. Here are some tips to make the most of your practice:
- Consistency is Key: Set aside regular time for practice, even if it’s just 30 minutes a day.
- Vary Your Practice: Don’t stick to one type of problem. Mix easy and challenging questions to keep your skills sharp across different areas.
- Understand, Don’t Memorize: Focus on understanding the underlying concepts rather than memorizing solutions.
- Review and Reflect: After solving a problem, take time to review other solutions and reflect on what you’ve learned.
- Apply Your Knowledge: Try to apply what you’ve learned in personal projects or open-source contributions.
- Engage with the Community: Participate in forums, discuss problems with peers, and learn from others’ experiences.
- Track Your Progress: Use the analytics tools provided by platforms like AlgoCademy to monitor your improvement over time.
Integrating AlgoCademy into Your Learning Routine
While all the platforms mentioned offer valuable resources, AlgoCademy stands out for its comprehensive approach to coding education and interview preparation. Here’s how you can effectively integrate AlgoCademy into your JavaScript learning routine:
- Start with the Basics: Use AlgoCademy’s interactive tutorials to solidify your understanding of JavaScript fundamentals.
- Progress to Algorithmic Challenges: As you become more comfortable, tackle AlgoCademy’s algorithmic problems to improve your problem-solving skills.
- Utilize AI Assistance: When you’re stuck, make use of the AI-powered hints and explanations to guide your learning.
- Practice Interview-Style Questions: Take advantage of AlgoCademy’s FAANG-focused content to prepare for technical interviews.
- Engage in Peer Learning: Participate in AlgoCademy’s community forums to discuss problems and learn from others.
- Track and Analyze: Regularly review your progress using AlgoCademy’s analytics tools to identify areas for improvement.
Sample JavaScript Problem and Solution
To give you a taste of what you might encounter on these platforms, here’s a sample JavaScript problem along with its solution:
Problem: Reverse a String
Write a function that takes a string as input and returns the string reversed.
Solution:
function reverseString(str) {
return str.split("").reverse().join("");
}
// Test the function
console.log(reverseString("hello")); // Output: "olleh"
console.log(reverseString("JavaScript")); // Output: "tpircSavaJ"
This solution uses the following steps:
str.split("")
converts the string into an array of characters..reverse()
reverses the order of elements in the array..join("")
joins the array elements back into a string.
While this is a simple example, it demonstrates the kind of thinking and JavaScript methods you’ll be working with as you practice.
Conclusion
Improving your JavaScript skills requires dedication, consistent practice, and exposure to a variety of programming challenges. While platforms like LeetCode, HackerRank, Codewars, freeCodeCamp, Edabit, and JavaScript30 all offer valuable resources, AlgoCademy stands out as a comprehensive solution that combines interactive learning, AI-assisted problem-solving, and targeted interview preparation.
By leveraging AlgoCademy’s unique features and integrating it with other practice resources, you can create a well-rounded learning experience that not only enhances your JavaScript skills but also prepares you for real-world programming challenges and technical interviews at top tech companies.
Remember, the key to mastering JavaScript lies not just in solving problems, but in understanding the underlying concepts, developing strong problem-solving skills, and applying your knowledge to practical scenarios. With dedication and the right resources, you’ll be well on your way to becoming a proficient JavaScript developer ready to tackle any coding challenge that comes your way.