Best Strategies for Solving Hard-Level Coding Challenges
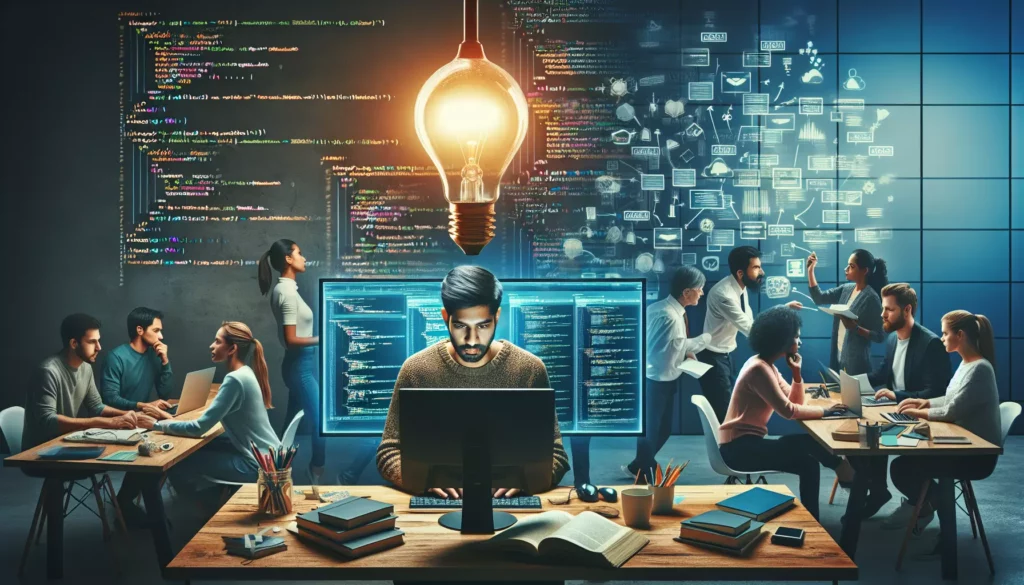
In the world of programming and software development, tackling hard-level coding challenges is an essential skill that separates proficient developers from the rest. Whether you’re preparing for technical interviews at top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google) or simply aiming to sharpen your problem-solving abilities, mastering these challenges is crucial. This comprehensive guide will walk you through the best strategies for approaching and conquering difficult coding problems, helping you elevate your skills to the next level.
1. Understand the Problem Thoroughly
The first and most critical step in solving any hard coding challenge is to fully comprehend the problem at hand. This involves:
- Reading the problem statement multiple times
- Identifying the input and output requirements
- Recognizing any constraints or edge cases
- Asking clarifying questions if anything is unclear
Take your time with this step, as a clear understanding of the problem is the foundation for developing an effective solution. Don’t hesitate to break down the problem into smaller, more manageable parts if it seems overwhelming at first glance.
2. Analyze the Problem and Brainstorm Approaches
Once you have a solid grasp of the problem, start analyzing it and brainstorming potential approaches. Consider the following techniques:
- Look for patterns or similarities to problems you’ve solved before
- Think about relevant data structures that might be useful
- Consider different algorithmic paradigms (e.g., divide and conquer, dynamic programming, greedy algorithms)
- Sketch out possible solutions on paper or a whiteboard
Remember, there’s often more than one way to solve a problem. Explore multiple approaches before settling on one to implement.
3. Start with a Brute Force Solution
When faced with a challenging problem, it’s often helpful to start with a brute force solution. This approach involves solving the problem in the most straightforward way possible, without worrying about efficiency. While brute force solutions are typically not optimal, they offer several advantages:
- They help you better understand the problem and its constraints
- They provide a baseline solution that you can optimize later
- They can be useful for generating test cases
Here’s an example of a brute force solution for finding the maximum subarray sum:
def max_subarray_sum_brute_force(arr):
n = len(arr)
max_sum = float('-inf')
for i in range(n):
for j in range(i, n):
current_sum = sum(arr[i:j+1])
max_sum = max(max_sum, current_sum)
return max_sum
# Example usage
arr = [-2, 1, -3, 4, -1, 2, 1, -5, 4]
print(max_subarray_sum_brute_force(arr)) # Output: 6
This solution has a time complexity of O(n³), which is not efficient for large inputs. However, it serves as a starting point for understanding the problem and developing a more optimized solution.
4. Optimize Your Solution
After implementing a brute force solution, focus on optimizing it. Look for ways to reduce time complexity, space complexity, or both. Common optimization techniques include:
- Using more efficient data structures (e.g., hash tables for faster lookups)
- Applying dynamic programming to avoid redundant calculations
- Utilizing divide and conquer strategies
- Implementing sliding window techniques for array or string problems
Binary search for sorted data
Let’s optimize our previous example using Kadane’s algorithm, which reduces the time complexity to O(n):
def max_subarray_sum_optimized(arr):
max_sum = current_sum = arr[0]
for num in arr[1:]:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
# Example usage
arr = [-2, 1, -3, 4, -1, 2, 1, -5, 4]
print(max_subarray_sum_optimized(arr)) # Output: 6
This optimized solution achieves the same result with significantly improved performance, especially for larger inputs.
5. Test Your Solution Thoroughly
Once you have an optimized solution, it’s crucial to test it thoroughly. This involves:
- Creating a diverse set of test cases, including edge cases
- Testing with small inputs to verify correctness
- Testing with large inputs to ensure performance
- Considering corner cases and boundary conditions
Here’s an example of how you might test the max subarray sum function:
def test_max_subarray_sum():
assert max_subarray_sum_optimized([-2, 1, -3, 4, -1, 2, 1, -5, 4]) == 6
assert max_subarray_sum_optimized([1]) == 1
assert max_subarray_sum_optimized([-1]) == -1
assert max_subarray_sum_optimized([]) == 0
assert max_subarray_sum_optimized([-1, -2, -3, -4]) == -1
assert max_subarray_sum_optimized([1, 2, 3, 4]) == 10
print("All tests passed!")
test_max_subarray_sum()
Thorough testing helps you identify and fix any bugs or edge cases you might have missed during implementation.
6. Analyze Time and Space Complexity
Understanding the time and space complexity of your solution is crucial, especially when dealing with hard-level coding challenges. This analysis helps you:
- Identify potential bottlenecks in your algorithm
- Compare different approaches objectively
- Determine if your solution meets the problem’s constraints
For our optimized max subarray sum solution, the time complexity is O(n) because we iterate through the array once. The space complexity is O(1) as we only use a constant amount of extra space.
When analyzing complexity, consider both the average case and worst-case scenarios. Be prepared to explain your analysis during technical interviews.
7. Practice Regularly with Various Problem Types
Consistency is key when it comes to improving your ability to solve hard coding challenges. Make it a habit to practice regularly, focusing on a variety of problem types. Some important categories to cover include:
- Array and String Manipulation
- Tree and Graph Algorithms
- Dynamic Programming
- Backtracking
- Greedy Algorithms
- Bit Manipulation
- System Design (for more advanced interviews)
Platforms like AlgoCademy, LeetCode, HackerRank, and CodeSignal offer a wide range of problems to practice with. Start with easier problems and gradually work your way up to harder ones as you build confidence and skills.
8. Learn to Recognize Common Patterns
As you practice more, you’ll start to recognize common patterns in coding challenges. Familiarizing yourself with these patterns can significantly speed up your problem-solving process. Some frequently encountered patterns include:
- Two Pointers
- Sliding Window
- Fast and Slow Pointers
- Merge Intervals
- Cyclic Sort
- In-place Reversal of a LinkedList
- Tree Breadth-First Search
- Tree Depth-First Search
- Two Heaps
- Subsets
- Modified Binary Search
- Top K Elements
- K-way Merge
- Topological Sort
Let’s look at an example of the Two Pointers pattern for solving the “Container With Most Water” problem:
def max_area(height):
left, right = 0, len(height) - 1
max_water = 0
while left < right:
width = right - left
container_height = min(height[left], height[right])
water = width * container_height
max_water = max(max_water, water)
if height[left] < height[right]:
left += 1
else:
right -= 1
return max_water
# Example usage
heights = [1, 8, 6, 2, 5, 4, 8, 3, 7]
print(max_area(heights)) # Output: 49
This solution uses two pointers (left and right) to efficiently find the maximum area of water that can be contained between two vertical lines.
9. Implement Time Management Strategies
When solving hard coding challenges, especially during timed interviews or coding competitions, effective time management is crucial. Here are some strategies to help you manage your time efficiently:
- Allocate time for understanding the problem (5-10 minutes)
- Set a time limit for brainstorming and planning (10-15 minutes)
- Implement your solution within a reasonable timeframe (20-30 minutes)
- Leave time for testing and debugging (10-15 minutes)
If you’re stuck on a problem for too long, consider moving on to the next one and coming back later if time permits. It’s better to solve multiple easier problems than to get bogged down on a single difficult one.
10. Utilize Pseudocode and Comments
Before diving into writing actual code, it’s often helpful to outline your approach using pseudocode or comments. This practice offers several benefits:
- It helps organize your thoughts and break down the problem into smaller steps
- It allows you to focus on the algorithm without getting distracted by syntax details
- It makes it easier to explain your approach to interviewers or collaborators
- It serves as a roadmap when implementing the actual code
Here’s an example of using pseudocode for a binary search algorithm:
# Binary Search Pseudocode
# Input: sorted array, target value
# Output: index of target value or -1 if not found
# Set left pointer to 0 and right pointer to length of array - 1
# While left pointer is less than or equal to right pointer:
# Calculate mid index as (left + right) // 2
# If element at mid index equals target:
# Return mid index
# Else if element at mid index is less than target:
# Set left pointer to mid + 1
# Else:
# Set right pointer to mid - 1
# If target not found, return -1
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
# Example usage
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15]
target = 7
result = binary_search(sorted_array, target)
print(f"Target {target} found at index: {result}") # Output: Target 7 found at index: 3
Using pseudocode or comments helps you think through the logic of your solution before committing to a specific implementation, potentially saving time and reducing errors.
11. Learn from Others and Seek Feedback
Solving hard coding challenges isn’t just about individual practice; it’s also about learning from others and continuously improving. Here are some ways to enhance your skills through collaboration and feedback:
- Participate in coding forums and discussion groups
- Attend coding meetups or hackathons
- Review solutions from other developers after solving a problem
- Pair program with a friend or colleague
- Seek code reviews from more experienced developers
Platforms like AlgoCademy often provide community features or discussion boards where you can interact with other learners and share insights. Take advantage of these resources to broaden your perspective and learn new approaches to problem-solving.
12. Develop a Problem-Solving Framework
As you gain more experience with hard coding challenges, it’s helpful to develop a personal problem-solving framework. This framework should guide you through the process of tackling any new problem efficiently. Here’s an example of what such a framework might look like:
- Understand the problem
- Read the problem statement carefully
- Identify input/output requirements
- Clarify any ambiguities
- Analyze and plan
- Break down the problem into smaller subproblems
- Consider relevant data structures and algorithms
- Sketch out a high-level approach
- Implement a solution
- Start with a brute force approach if necessary
- Optimize the solution iteratively
- Use clear variable names and add comments
- Test and debug
- Create test cases, including edge cases
- Run the code with sample inputs
- Debug any issues that arise
- Analyze complexity
- Determine time and space complexity
- Consider ways to further optimize if needed
- Reflect and learn
- Review your approach and identify areas for improvement
- Compare your solution with other approaches
- Note any new concepts or techniques learned
Having a structured approach like this can help you tackle even the most challenging coding problems with confidence and efficiency.
13. Stay Updated with New Algorithms and Data Structures
The field of computer science is constantly evolving, with new algorithms and data structures being developed or refined. Staying up-to-date with these advancements can give you an edge when solving hard coding challenges. Here are some ways to keep your knowledge current:
- Follow computer science research papers and publications
- Attend tech conferences or watch recorded sessions online
- Take advanced algorithm courses on platforms like Coursera or edX
- Experiment with implementing new algorithms you learn about
For example, familiarizing yourself with advanced data structures like Segment Trees, Fenwick Trees, or Disjoint Set Union can open up new possibilities for solving complex problems efficiently.
14. Practice Explaining Your Thought Process
In many technical interviews, the interviewer is just as interested in your problem-solving process as they are in your final solution. Practicing how to articulate your thoughts clearly can significantly improve your performance in these situations. Try the following techniques:
- Solve problems out loud, explaining each step as you go
- Record yourself solving problems and review the recordings
- Practice with a friend or mentor who can provide feedback
- Write blog posts or create video tutorials explaining complex algorithms
Being able to communicate your approach effectively not only helps in interviews but also improves your overall understanding of the problem-solving process.
15. Develop a Growth Mindset
Finally, one of the most important strategies for tackling hard coding challenges is to cultivate a growth mindset. This means viewing challenges as opportunities to learn and grow, rather than as threats or insurmountable obstacles. Here are some ways to foster a growth mindset:
- Embrace difficulties as chances to improve your skills
- View mistakes as learning experiences rather than failures
- Focus on the process of problem-solving, not just the outcome
- Celebrate small victories and progress along the way
- Set challenging but achievable goals for yourself
Remember that even the most experienced developers face challenges and continue to learn. By maintaining a positive attitude and a willingness to learn, you’ll be better equipped to handle any coding challenge that comes your way.
Conclusion
Mastering hard-level coding challenges is a journey that requires dedication, practice, and a strategic approach. By implementing the strategies outlined in this guide—from thorough problem analysis and optimized solution development to regular practice and continuous learning—you’ll be well-equipped to tackle even the most complex coding problems.
Remember that improvement comes with time and experience. Don’t get discouraged if you struggle with difficult problems at first. Keep practicing, stay curious, and always be open to learning new techniques and approaches. With persistence and the right strategies, you’ll find yourself solving challenging coding problems with increasing confidence and skill.
Whether you’re preparing for technical interviews at top tech companies or simply aiming to become a more proficient programmer, the skills you develop through solving hard coding challenges will serve you well throughout your career in software development. Happy coding!