Best Programming Language for Your Coding Interview
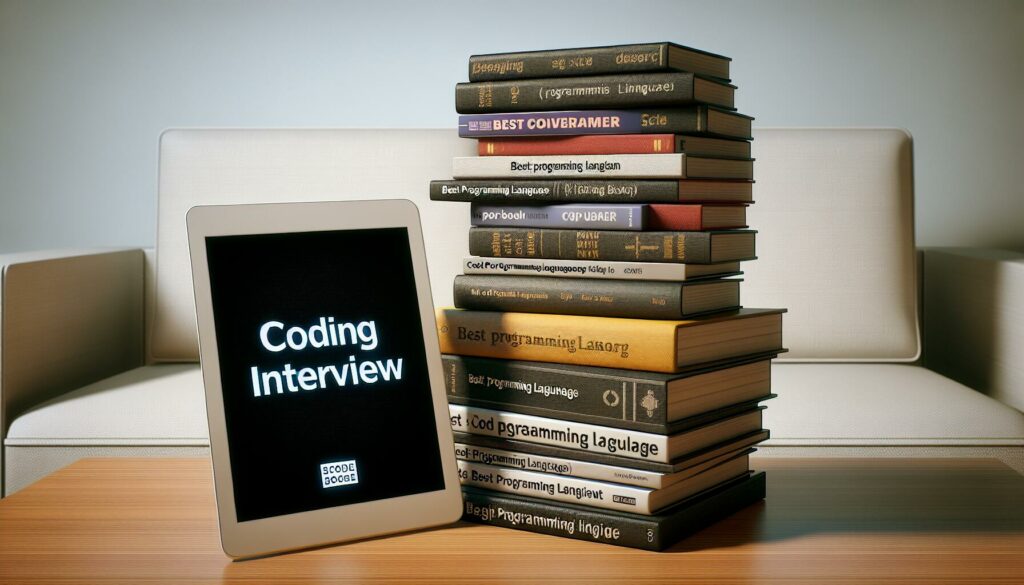
As you prepare for your upcoming coding interview, one crucial decision looms large: which programming language should you choose? This choice can significantly impact your performance and confidence during the interview. In this comprehensive guide, we’ll explore the factors to consider when selecting a programming language for your coding interview, address common concerns, and provide practical advice to help you make the best decision.
The Great Language Debate: Does It Really Matter?
Let’s start by addressing the elephant in the room: does your choice of programming language actually matter in a coding interview? The short answer is both yes and no. Here’s why:
Why It Matters
- Comfort and Fluency: Your chosen language should be one you’re comfortable with. The more fluent you are, the faster and more accurately you can translate your problem-solving thoughts into code.
- Syntax Familiarity: In high-pressure interview situations, you don’t want to be struggling with basic syntax. Choosing a language you know well minimizes the risk of silly mistakes.
- Built-in Functions and Libraries: Different languages have different standard libraries and built-in functions. Knowing these well can save you time during the interview.
- Language-Specific Optimizations: Some languages have unique features or optimizations that can be leveraged to solve problems more efficiently.
Why It Doesn’t Matter (As Much)
- Problem-Solving Skills: Most interviewers are more interested in your problem-solving approach than the specific language you use.
- Algorithmic Knowledge: The core algorithms and data structures are language-agnostic. A good interviewer can evaluate your knowledge regardless of the language.
- Adaptability: Many companies value the ability to learn new languages quickly. Your choice of language is less important than your ability to explain your thought process.
Choosing Your Weapon: Factors to Consider
When selecting a programming language for your coding interview, consider the following factors:
1. Your Proficiency Level
The most crucial factor is your proficiency in the language. Choose the language you’re most comfortable with, as this will allow you to focus on problem-solving rather than syntax.
2. The Job Requirements
If you’re interviewing for a specific role, like an iOS engineer position, the choice might be obvious (Swift, in this case). However, for general software engineering roles, you usually have more flexibility.
3. Language Popularity in the Industry
While not always necessary, choosing a widely-used language can be beneficial. Popular languages like Python, Java, C++, or JavaScript are safe bets for most interviews.
4. Language Features and Expressiveness
Some languages, like Python, offer concise syntax and powerful built-in functions that can help you write solutions more quickly. However, make sure you understand the time complexity of these operations.
5. Interviewers’ Preferences
If you know your interviewers’ backgrounds or the company’s tech stack, you might choose a language they’re familiar with. However, don’t sacrifice your proficiency for this.
The Python Dilemma: Is It Too High-Level?
A common concern among candidates is whether choosing a high-level language like Python might be seen as “taking the easy way out” or result in penalties during the interview. Let’s address this:
Advantages of Using Python
- Readability: Python’s clean, readable syntax can help you communicate your ideas more clearly.
- Rapid Prototyping: Python allows for quick implementation of ideas, which can be beneficial in a time-constrained interview.
- Rich Standard Library: Python’s extensive standard library provides many useful tools out of the box.
- Popularity: Many companies use Python, and interviewers are likely familiar with it.
Potential Drawbacks
- Abstraction of Low-Level Details: Python abstracts away some low-level operations, which might make it harder to discuss certain optimization techniques.
- Performance: Python is generally slower than lower-level languages like C++, which might be a concern for some interviewers.
- Lack of Static Typing: This can make it easier to introduce bugs, especially in larger codebases.
The Verdict
In most cases, using Python (or any other high-level language) will not penalize you in an interview. The key is to demonstrate a deep understanding of the language you choose, including its strengths and limitations.
If you choose Python, make sure you can:
- Explain the time and space complexity of your solutions.
- Understand how Python implements key data structures and algorithms behind the scenes.
- Discuss trade-offs between different approaches, including potential performance implications.
The Importance of Language Proficiency
Regardless of which language you choose, it’s crucial to be well-versed in its intricacies. Here are some key areas to focus on:
1. Syntax and Basic Constructs
Ensure you’re comfortable with the language’s syntax for:
- Variable declarations and assignments
- Control structures (if/else, loops, switches)
- Function definitions and calls
- Class and object creation (for OOP languages)
2. Standard Library and Built-in Functions
Familiarize yourself with the language’s standard library, especially:
- Common data structures (lists, dictionaries, sets, etc.)
- String manipulation functions
- File I/O operations
- Basic mathematical operations
3. Time Complexity of Built-in Operations
This is where many candidates falter. It’s essential to understand the time complexity of common operations in your chosen language. For example:
Python Example:
def reverse(input):
result = ""
for char in input:
result = char + result
return result
At first glance, you might think this function has a time complexity of O(n) because there’s only one loop. However, string concatenation in Python creates a new string object each time, resulting in an O(n^2) time complexity.
A more efficient solution would be:
def reverse(input):
return input[::-1] # O(n) time complexity
Understanding these nuances can make a significant difference in your interview performance.
4. Memory Management
Understand how your chosen language handles memory:
- For languages like C or C++, be familiar with manual memory management.
- For garbage-collected languages like Python or Java, understand the basics of how the garbage collector works.
5. Language-Specific Idioms and Best Practices
Each language has its own set of idioms and best practices. Familiarize yourself with these to write more idiomatic and efficient code.
When to Consider an Alternative Language
While sticking to your most proficient language is generally the best approach, there are scenarios where you might consider an alternative:
1. Eccentric or Niche Languages
If your primary language is highly specialized or eccentric (e.g., Brainfuck, APL, or even some domain-specific languages), it might be worth choosing a more mainstream alternative for your interview.
2. Language Mismatch with the Role
If you’re applying for a role that heavily uses a specific language (e.g., Java for Android development), and you’re not proficient in it, consider brushing up on that language if time allows.
3. Performance-Critical Scenarios
If the role involves a lot of low-level or performance-critical work, and your primary language is high-level, you might want to demonstrate proficiency in a lower-level language as well.
Preparing Your Language Skills for the Interview
Once you’ve chosen your language, here are some steps to ensure you’re well-prepared:
1. Practice, Practice, Practice
Solve coding problems in your chosen language regularly. Platforms like LeetCode, HackerRank, or AlgoExpert can be invaluable for this.
2. Review Language Fundamentals
Go through the language documentation and refresh your knowledge of its core features and standard library.
3. Implement Common Data Structures and Algorithms
Try implementing fundamental data structures (linked lists, trees, graphs) and algorithms (sorting, searching) from scratch in your chosen language.
4. Time Complexity Analysis
Practice analyzing the time and space complexity of your solutions. Be prepared to explain these analyses in the interview.
5. Code Without an IDE
Practice writing code without the help of an IDE or autocomplete. Many interviews involve coding on a whiteboard or in a simple text editor.
6. Mock Interviews
Conduct mock interviews with friends or use services that offer practice interviews. This will help you get comfortable explaining your code and thought process.
Conclusion: Confidence is Key
Ultimately, the best programming language for your coding interview is the one that allows you to showcase your problem-solving skills with confidence. While the choice of language can impact your performance, it’s your ability to think critically, communicate clearly, and write efficient code that will truly set you apart.
Remember these key points:
- Choose the language you’re most comfortable with, unless there’s a compelling reason not to.
- Understand the strengths and limitations of your chosen language.
- Be prepared to explain the time and space complexity of your solutions.
- Practice regularly and familiarize yourself with common interview patterns.
- Don’t be afraid to use a higher-level language like Python, but be ready to discuss lower-level concepts if needed.
By following these guidelines and thoroughly preparing in your chosen language, you’ll be well-equipped to tackle your coding interview with confidence. Remember, the goal is not just to write code, but to demonstrate your problem-solving skills and thought process.
Good luck with your interview preparation! And don’t forget to check out the free coding tutorials on AlgoCademy to further hone your skills and explore different programming languages.