Best Practices for Writing Clean Code: A Comprehensive Guide
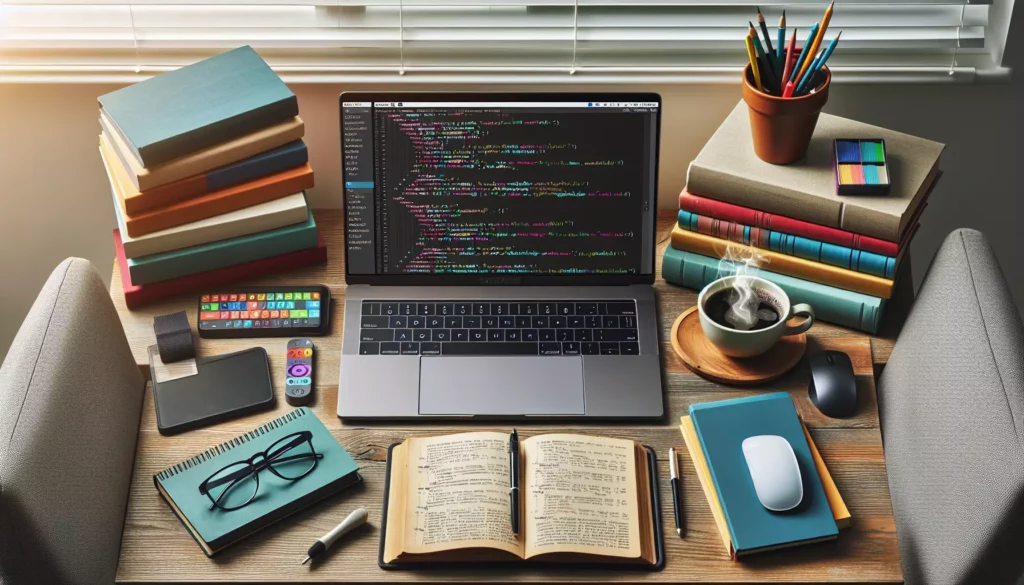
In the world of software development, writing clean code is an essential skill that separates great programmers from the rest. Clean code not only makes your work more readable and maintainable but also enhances collaboration and reduces the likelihood of bugs. In this comprehensive guide, we’ll explore the best practices for writing clean code, helping you elevate your coding skills and become a more efficient developer.
Table of Contents
- Understanding Clean Code
- Naming Conventions
- Code Organization
- Commenting and Documentation
- DRY Principle
- KISS Principle
- SOLID Principles
- Error Handling
- Code Formatting
- Version Control
- Testing
- Code Reviews
- Continuous Improvement
- Conclusion
1. Understanding Clean Code
Clean code is code that is easy to understand, easy to modify, and easy to maintain. It follows a consistent style, is well-organized, and clearly expresses the intent of the programmer. The concept of clean code was popularized by Robert C. Martin in his book “Clean Code: A Handbook of Agile Software Craftsmanship.”
Key characteristics of clean code include:
- Readability: Code should be easily understood by other developers.
- Simplicity: Code should be as simple as possible while still accomplishing its task.
- Maintainability: Code should be easy to modify and extend.
- Testability: Code should be structured in a way that makes it easy to write and run tests.
- Efficiency: Clean code should perform well and use resources efficiently.
2. Naming Conventions
Proper naming is crucial for writing clean code. Good names can make your code self-explanatory, reducing the need for comments and making it easier for others (including your future self) to understand your code.
Variables
- Use descriptive and meaningful names that explain the purpose of the variable.
- Avoid single-letter names (except for loop counters in short blocks).
- Use camelCase for variable names in most programming languages (e.g., JavaScript, Java).
- Use snake_case for variable names in Python.
Example of good variable naming:
// Bad
let d = new Date();
let x = 5;
// Good
let currentDate = new Date();
let numberOfAttempts = 5;
Functions
- Use verbs or verb phrases to name functions (e.g., calculateTotal, fetchUserData).
- Be specific about what the function does.
- Use camelCase for function names in most programming languages.
Example of good function naming:
// Bad
function do_stuff(data) {
// ...
}
// Good
function processUserInput(userInput) {
// ...
}
Classes
- Use nouns or noun phrases for class names.
- Use PascalCase for class names in most object-oriented languages.
Example of good class naming:
// Bad
class data_processor {
// ...
}
// Good
class DataProcessor {
// ...
}
3. Code Organization
Properly organizing your code is essential for maintainability and readability. Here are some best practices for code organization:
File Structure
- Group related files together in directories.
- Use a consistent and logical file naming convention.
- Separate concerns by creating different modules or classes for distinct functionalities.
Function Length
- Keep functions short and focused on a single task.
- Aim for functions that are no longer than 20-30 lines.
- If a function grows too large, consider breaking it into smaller, more manageable functions.
Class Structure
- Follow the Single Responsibility Principle (SRP) – a class should have only one reason to change.
- Group related methods and properties together.
- Use access modifiers (public, private, protected) to control the visibility of class members.
Code Grouping
- Group related code blocks together.
- Use whitespace to separate logical sections of code.
- Maintain a consistent order for methods and properties within a class.
4. Commenting and Documentation
While clean code should be self-explanatory to a large extent, comments and documentation still play a crucial role in maintaining and understanding code.
Inline Comments
- Use inline comments sparingly and only when necessary to explain complex logic or non-obvious decisions.
- Avoid commenting out code – use version control instead.
- Keep comments up-to-date as the code changes.
Example of good inline commenting:
// Calculate the discount based on the customer's loyalty status
let discount = calculateDiscount(customer.loyaltyYears);
// Apply additional holiday discount if applicable
if (isHolidaySeason()) {
discount += HOLIDAY_DISCOUNT_PERCENTAGE;
}
Function and Class Documentation
- Use documentation comments (e.g., JSDoc, Javadoc) to describe the purpose, parameters, and return values of functions and classes.
- Include examples of usage when appropriate.
- Document any exceptions or errors that may be thrown.
Example of good function documentation:
/**
* Calculates the total price of items in the shopping cart.
*
* @param {Array} items - An array of objects representing cart items.
* @param {number} taxRate - The tax rate as a decimal (e.g., 0.08 for 8%).
* @returns {number} The total price including tax.
* @throws {Error} If the items array is empty or the tax rate is negative.
*/
function calculateTotalPrice(items, taxRate) {
// Function implementation...
}
README Files
- Include a README file in your project root directory.
- Provide an overview of the project, installation instructions, and basic usage examples.
- Keep the README up-to-date as the project evolves.
5. DRY Principle
DRY stands for “Don’t Repeat Yourself.” This principle emphasizes the importance of avoiding code duplication. Adhering to the DRY principle leads to more maintainable and less error-prone code.
Benefits of DRY Code
- Easier maintenance: Changes only need to be made in one place.
- Reduced chance of inconsistencies.
- Improved readability and organization.
Implementing DRY
- Extract common code into functions or methods.
- Use inheritance and composition to share behavior between classes.
- Utilize design patterns to solve recurring problems in a standardized way.
Example of applying the DRY principle:
// Before (Not DRY)
function calculateCircleArea(radius) {
return 3.14159 * radius * radius;
}
function calculateCircleCircumference(radius) {
return 2 * 3.14159 * radius;
}
// After (DRY)
const PI = 3.14159;
function calculateCircleArea(radius) {
return PI * radius * radius;
}
function calculateCircleCircumference(radius) {
return 2 * PI * radius;
}
6. KISS Principle
KISS stands for “Keep It Simple, Stupid” (or “Keep It Short and Simple”). This principle emphasizes the importance of simplicity in design and implementation.
Benefits of KISS
- Easier to understand and maintain code.
- Reduced likelihood of bugs and errors.
- Faster development and debugging processes.
Implementing KISS
- Avoid over-engineering solutions.
- Break complex problems into smaller, manageable parts.
- Use clear and straightforward logic.
- Avoid unnecessary abstractions or premature optimizations.
Example of applying the KISS principle:
// Over-complicated (Not KISS)
function isEven(num) {
return num % 2 == 0 ? true : false;
}
// Simple (KISS)
function isEven(num) {
return num % 2 === 0;
}
7. SOLID Principles
SOLID is an acronym for five principles of object-oriented programming and design. These principles, when applied together, make it easier to create more maintainable, understandable, and flexible software.
S – Single Responsibility Principle (SRP)
A class should have only one reason to change. In other words, a class should have only one job or responsibility.
O – Open-Closed Principle (OCP)
Software entities (classes, modules, functions, etc.) should be open for extension but closed for modification.
L – Liskov Substitution Principle (LSP)
Objects of a superclass should be replaceable with objects of its subclasses without affecting the correctness of the program.
I – Interface Segregation Principle (ISP)
Many client-specific interfaces are better than one general-purpose interface.
D – Dependency Inversion Principle (DIP)
High-level modules should not depend on low-level modules. Both should depend on abstractions. Abstractions should not depend on details. Details should depend on abstractions.
Example of applying the Single Responsibility Principle:
// Not following SRP
class User {
constructor(name) {
this.name = name;
}
saveUser() {
// Save user to database
}
sendEmail() {
// Send email to user
}
}
// Following SRP
class User {
constructor(name) {
this.name = name;
}
}
class UserRepository {
saveUser(user) {
// Save user to database
}
}
class EmailService {
sendEmail(user) {
// Send email to user
}
}
8. Error Handling
Proper error handling is crucial for writing robust and reliable code. It helps in identifying and resolving issues quickly, and provides a better user experience.
Best Practices for Error Handling
- Use try-catch blocks to handle exceptions.
- Create custom error classes for specific types of errors.
- Provide meaningful error messages.
- Log errors for debugging purposes.
- Fail fast: detect and report errors as soon as possible.
Example of good error handling:
class InsufficientFundsError extends Error {
constructor(message) {
super(message);
this.name = 'InsufficientFundsError';
}
}
function withdrawMoney(account, amount) {
try {
if (amount <= 0) {
throw new Error('Invalid withdrawal amount');
}
if (account.balance < amount) {
throw new InsufficientFundsError('Insufficient funds for withdrawal');
}
account.balance -= amount;
return amount;
} catch (error) {
console.error(`Error: ${error.message}`);
// Log the error or perform other error handling tasks
throw error; // Re-throw the error for the caller to handle
}
}
9. Code Formatting
Consistent code formatting improves readability and makes it easier for team members to collaborate. Many programming languages have established style guides that you can follow.
Formatting Best Practices
- Use consistent indentation (spaces or tabs).
- Limit line length (typically 80-120 characters).
- Use whitespace to separate logical blocks of code.
- Place opening braces on the same line as control statements in most languages.
- Use meaningful blank lines to separate functions and logical sections.
Tools for Formatting
- Use an Integrated Development Environment (IDE) with built-in formatting capabilities.
- Implement linters and code formatters in your development workflow (e.g., ESLint for JavaScript, Black for Python).
- Consider using tools like Prettier for automatic code formatting.
Example of well-formatted code:
function calculateAverage(numbers) {
if (numbers.length === 0) {
return 0;
}
let sum = 0;
for (let i = 0; i < numbers.length; i++) {
sum += numbers[i];
}
return sum / numbers.length;
}
const scores = [85, 90, 78, 92, 88];
const averageScore = calculateAverage(scores);
console.log(`The average score is: ${averageScore}`);
10. Version Control
Using version control systems like Git is essential for managing code changes, collaborating with others, and maintaining a history of your project.
Version Control Best Practices
- Commit frequently with meaningful commit messages.
- Use branches for developing new features or fixing bugs.
- Pull changes from the main branch regularly to stay up-to-date.
- Use pull requests for code reviews before merging changes.
- Tag important releases or versions of your code.
Writing Good Commit Messages
- Use the imperative mood in the subject line (e.g., “Add feature” not “Added feature”).
- Keep the subject line short (50 characters or less).
- Provide more detailed explanations in the commit body if necessary.
- Reference issue numbers or pull requests when applicable.
Example of a good commit message:
Add user authentication feature
- Implement login and registration forms
- Set up JWT token-based authentication
- Create protected routes for authenticated users
Closes #123
11. Testing
Writing tests for your code is crucial for ensuring its correctness, catching bugs early, and making it easier to refactor and maintain your codebase.
Types of Tests
- Unit Tests: Test individual components or functions in isolation.
- Integration Tests: Test how different parts of the system work together.
- End-to-End Tests: Test the entire application from the user’s perspective.
Testing Best Practices
- Write tests before or alongside your code (Test-Driven Development).
- Aim for high test coverage, but focus on critical and complex parts of your code.
- Keep tests simple, fast, and independent of each other.
- Use descriptive test names that explain what is being tested.
- Regularly run your test suite, especially before committing changes.
Example of a unit test using Jest (JavaScript testing framework):
// Function to be tested
function add(a, b) {
return a + b;
}
// Test suite
describe('add function', () => {
test('adds two positive numbers correctly', () => {
expect(add(2, 3)).toBe(5);
});
test('adds a positive and a negative number correctly', () => {
expect(add(5, -3)).toBe(2);
});
test('adds two negative numbers correctly', () => {
expect(add(-2, -4)).toBe(-6);
});
});
12. Code Reviews
Code reviews are an essential part of the development process, helping to improve code quality, share knowledge, and catch potential issues before they make it into production.
Benefits of Code Reviews
- Identify bugs and potential issues early.
- Ensure adherence to coding standards and best practices.
- Share knowledge and foster team learning.
- Improve overall code quality and maintainability.
Code Review Best Practices
- Review code in small, manageable chunks.
- Use a checklist to ensure consistent review quality.
- Provide constructive feedback and suggestions for improvement.
- Focus on the code, not the person.
- Be timely in your reviews to avoid blocking team progress.
What to Look for in Code Reviews
- Adherence to coding standards and best practices.
- Potential bugs or logic errors.
- Code duplication or opportunities for refactoring.
- Security vulnerabilities.
- Performance issues.
- Proper error handling and edge case consideration.
- Adequate test coverage.
13. Continuous Improvement
Writing clean code is an ongoing process that requires continuous learning and improvement. Here are some strategies to help you continually enhance your coding skills:
Stay Updated
- Follow industry blogs, podcasts, and newsletters.
- Attend conferences and webinars.
- Participate in online coding communities (e.g., Stack Overflow, GitHub).
Practice Regularly
- Work on personal projects or contribute to open-source initiatives.
- Solve coding challenges on platforms like LeetCode, HackerRank, or CodeWars.
- Participate in coding competitions or hackathons.
Seek Feedback
- Ask for code reviews from more experienced developers.
- Pair program with colleagues to learn different approaches.
- Be open to constructive criticism and use it to improve your skills.
Refactor and Optimize
- Regularly review and refactor your old code.
- Look for opportunities to optimize and improve existing solutions.
- Experiment with different design patterns and architectural approaches.
14. Conclusion
Writing clean code is a skill that takes time and practice to master. By following the best practices outlined in this guide – from proper naming conventions and code organization to adhering to principles like DRY and SOLID – you can significantly improve the quality of your code.
Remember that clean code is not just about following rules; it’s about creating software that is easy to understand, maintain, and extend. It’s about writing code that you and your team members will thank you for in the future.
As you continue your journey as a developer, make clean code a priority in your work. Regularly revisit these practices, stay updated with evolving standards, and always strive for improvement. With dedication and practice, writing clean code will become second nature, making you a more effective and valuable programmer.
Happy coding!