Best Practices for Writing Clean Code: A Comprehensive Guide
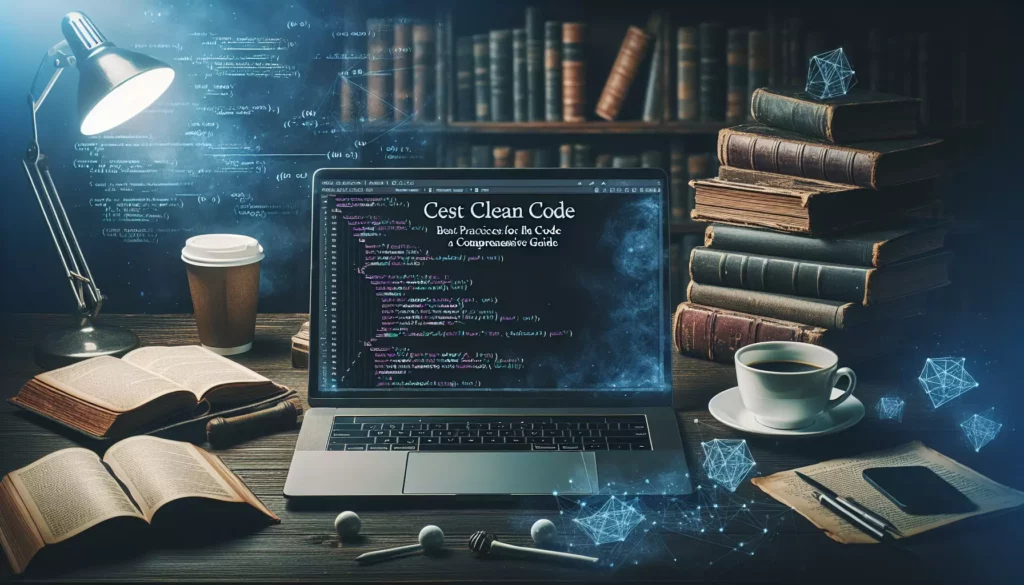
In the world of software development, writing clean code is an essential skill that separates great programmers from good ones. Clean code not only makes your work more readable and maintainable but also enhances collaboration and reduces the likelihood of bugs. Whether you’re a beginner just starting your coding journey or an experienced developer looking to refine your skills, understanding and implementing clean code practices is crucial for your success in the tech industry.
In this comprehensive guide, we’ll explore the best practices for writing clean code, providing you with practical tips and examples to elevate your coding skills. By the end of this article, you’ll have a solid foundation in clean coding techniques that will serve you well in your programming career, whether you’re aiming for a position at a major tech company or working on personal projects.
Table of Contents
- Why Clean Code Matters
- Naming Conventions
- Code Structure and Organization
- Comments and Documentation
- DRY Principle
- SOLID Principles
- Error Handling and Exception Management
- Writing Clean Tests
- The Importance of Code Reviews
- Continuous Refactoring
- Tools and Resources for Clean Coding
- Conclusion
1. Why Clean Code Matters
Before diving into specific practices, it’s crucial to understand why clean code is so important:
- Readability: Clean code is easy to read and understand, not just for you but for other developers who may work on the project in the future.
- Maintainability: Well-structured code is easier to maintain and update, reducing the time and effort required for future modifications.
- Reduced Bugs: Clean code is less prone to bugs and errors, as its clarity makes it easier to spot and fix issues.
- Efficiency: Writing clean code from the start saves time in the long run by reducing the need for extensive refactoring and debugging.
- Collaboration: Clean code facilitates better collaboration among team members, as it’s easier for others to understand and contribute to your work.
2. Naming Conventions
One of the fundamental aspects of clean code is using clear and descriptive names for variables, functions, classes, and other elements. Good naming conventions make your code self-explanatory and reduce the need for excessive comments.
Tips for Effective Naming:
- Use descriptive and meaningful names that convey the purpose or functionality of the element.
- Follow consistent capitalization conventions (e.g., camelCase for variables and functions, PascalCase for classes).
- Avoid abbreviations unless they are widely understood in the context.
- Use verb phrases for function names (e.g.,
calculateTotal()
,getUserData()
). - Choose names that are pronounceable and searchable.
Examples:
// Poor naming
function f(x, y) {
return x + y;
}
// Good naming
function calculateSum(firstNumber, secondNumber) {
return firstNumber + secondNumber;
}
// Poor naming
let d = new Date();
// Good naming
let currentDate = new Date();
By following these naming conventions, you make your code more intuitive and easier to understand at a glance.
3. Code Structure and Organization
The way you structure and organize your code plays a significant role in its cleanliness and readability. A well-structured codebase is easier to navigate and maintain.
Best Practices for Code Structure:
- Keep functions small and focused on a single task.
- Organize related code into modules or classes.
- Use consistent indentation and formatting.
- Group related variables and functions together.
- Separate concerns by dividing your code into logical sections or files.
Example of Well-Structured Code:
class UserManager {
constructor(database) {
this.database = database;
}
async createUser(userData) {
// Validate user data
if (!this.isValidUserData(userData)) {
throw new Error('Invalid user data');
}
// Create user in database
const userId = await this.database.insertUser(userData);
// Send welcome email
await this.sendWelcomeEmail(userData.email);
return userId;
}
isValidUserData(userData) {
// Implementation of data validation
}
async sendWelcomeEmail(email) {
// Implementation of email sending
}
}
In this example, the UserManager
class encapsulates user-related functionality, with small, focused methods that handle specific tasks.
4. Comments and Documentation
While clean code should be largely self-explanatory, comments and documentation still play an important role in providing context and explaining complex logic.
Guidelines for Effective Comments:
- Use comments to explain why something is done, not what is being done (the code itself should show what’s being done).
- Keep comments concise and to the point.
- Update comments when you change the code to ensure they remain accurate.
- Use documentation comments (e.g., JSDoc in JavaScript) for public APIs and functions.
- Avoid commenting out code – use version control instead.
Example of Good Comments:
/**
* Calculates the total price including tax.
* @param {number} basePrice - The base price of the item.
* @param {number} taxRate - The tax rate as a decimal (e.g., 0.1 for 10%).
* @returns {number} The total price including tax.
*/
function calculateTotalPrice(basePrice, taxRate) {
// Use Math.round to avoid floating-point precision issues
return Math.round((basePrice * (1 + taxRate)) * 100) / 100;
}
In this example, the comment explains the purpose of the function and provides details about the parameters and return value. The inline comment explains the reasoning behind using Math.round
.
5. DRY Principle
DRY stands for “Don’t Repeat Yourself.” This principle encourages you to avoid duplicating code by abstracting common functionality into reusable functions or classes.
Benefits of DRY Code:
- Reduces code duplication
- Makes maintenance easier
- Improves code consistency
- Reduces the chances of bugs
Example of Applying DRY:
// Before applying DRY
function calculateCircleArea(radius) {
return Math.PI * radius * radius;
}
function calculateCircleCircumference(radius) {
return 2 * Math.PI * radius;
}
// After applying DRY
class Circle {
constructor(radius) {
this.radius = radius;
}
area() {
return Math.PI * this.radius ** 2;
}
circumference() {
return 2 * Math.PI * this.radius;
}
}
const myCircle = new Circle(5);
console.log(myCircle.area());
console.log(myCircle.circumference());
In the DRY version, we’ve encapsulated the circle-related calculations into a single Circle
class, reducing duplication and improving organization.
6. SOLID Principles
SOLID is an acronym for five principles of object-oriented programming and design that contribute to creating clean, maintainable code:
- Single Responsibility Principle (SRP)
- Open/Closed Principle (OCP)
- Liskov Substitution Principle (LSP)
- Interface Segregation Principle (ISP)
- Dependency Inversion Principle (DIP)
Let’s focus on the Single Responsibility Principle as an example:
Single Responsibility Principle (SRP)
This principle states that a class or module should have only one reason to change. In other words, it should have only one job or responsibility.
Example of SRP:
// Before applying SRP
class User {
constructor(name, email) {
this.name = name;
this.email = email;
}
saveToDatabase() {
// Code to save user to database
}
sendEmail(message) {
// Code to send email
}
}
// After applying SRP
class User {
constructor(name, email) {
this.name = name;
this.email = email;
}
}
class UserRepository {
saveUser(user) {
// Code to save user to database
}
}
class EmailService {
sendEmail(recipient, message) {
// Code to send email
}
}
In the SRP version, we’ve separated the responsibilities of user data management, database operations, and email sending into distinct classes, making the code more modular and easier to maintain.
7. Error Handling and Exception Management
Proper error handling is crucial for writing robust and reliable code. Clean code should handle errors gracefully and provide meaningful feedback.
Best Practices for Error Handling:
- Use try-catch blocks to handle exceptions.
- Create custom error classes for specific error types.
- Provide meaningful error messages.
- Log errors for debugging purposes.
- Avoid using exceptions for flow control.
Example of Good Error Handling:
class InvalidInputError extends Error {
constructor(message) {
super(message);
this.name = 'InvalidInputError';
}
}
function divideNumbers(a, b) {
if (typeof a !== 'number' || typeof b !== 'number') {
throw new InvalidInputError('Both arguments must be numbers');
}
if (b === 0) {
throw new Error('Cannot divide by zero');
}
return a / b;
}
try {
const result = divideNumbers(10, 'a');
console.log(result);
} catch (error) {
if (error instanceof InvalidInputError) {
console.error('Invalid input:', error.message);
} else {
console.error('An error occurred:', error.message);
}
}
This example demonstrates proper error handling with custom error classes, input validation, and specific error messages.
8. Writing Clean Tests
Clean code extends beyond just the production code – it’s equally important to write clean, maintainable tests. Good tests serve as documentation and help ensure the reliability of your code.
Principles for Clean Tests:
- Follow the AAA pattern: Arrange, Act, Assert
- Keep tests simple and focused on a single behavior
- Use descriptive test names
- Avoid logic in tests
- Make tests independent and idempotent
Example of a Clean Test:
describe('calculateTotalPrice', () => {
it('should calculate the correct total price with tax', () => {
// Arrange
const basePrice = 100;
const taxRate = 0.1;
// Act
const totalPrice = calculateTotalPrice(basePrice, taxRate);
// Assert
expect(totalPrice).toBe(110);
});
it('should throw an error for negative base price', () => {
// Arrange
const basePrice = -50;
const taxRate = 0.1;
// Act & Assert
expect(() => calculateTotalPrice(basePrice, taxRate)).toThrow('Base price must be positive');
});
});
These tests are clear, focused, and follow the AAA pattern, making them easy to understand and maintain.
9. The Importance of Code Reviews
Code reviews are a crucial part of maintaining clean code and improving overall code quality. They provide an opportunity for peers to offer feedback, catch potential issues, and share knowledge.
Benefits of Code Reviews:
- Identify bugs and potential issues early
- Ensure adherence to coding standards and best practices
- Share knowledge and techniques among team members
- Improve overall code quality and consistency
- Provide learning opportunities for both reviewers and authors
Tips for Effective Code Reviews:
- Be constructive and respectful in your feedback
- Focus on the code, not the person
- Use a checklist to ensure consistent review criteria
- Provide specific examples and suggestions for improvement
- Be open to discussion and alternative approaches
Implementing a robust code review process can significantly contribute to maintaining clean code across your entire codebase.
10. Continuous Refactoring
Refactoring is the process of restructuring existing code without changing its external behavior. It’s an ongoing practice that helps maintain clean code over time.
Benefits of Continuous Refactoring:
- Improves code readability and maintainability
- Reduces technical debt
- Makes it easier to add new features
- Helps identify and fix potential bugs
Refactoring Techniques:
- Extract Method: Move a code fragment into a separate method
- Rename Variable/Method: Choose more descriptive names
- Extract Class: Split a large class into smaller, more focused classes
- Replace Conditional with Polymorphism: Use object-oriented principles to simplify complex conditionals
Example of Refactoring:
// Before refactoring
function calculateTotal(items) {
let total = 0;
for (let i = 0; i < items.length; i++) {
total += items[i].price * items[i].quantity;
if (items[i].type === 'food') {
total *= 0.9; // 10% discount on food items
}
}
return total;
}
// After refactoring
function calculateTotal(items) {
return items.reduce((total, item) => total + calculateItemTotal(item), 0);
}
function calculateItemTotal(item) {
const baseTotal = item.price * item.quantity;
return applyDiscount(baseTotal, item.type);
}
function applyDiscount(total, itemType) {
return itemType === 'food' ? total * 0.9 : total;
}
This refactored version separates concerns, improves readability, and makes the code more modular and easier to maintain.
11. Tools and Resources for Clean Coding
There are numerous tools and resources available to help you write and maintain clean code:
Linters and Code Formatters:
- ESLint (JavaScript)
- Pylint (Python)
- RuboCop (Ruby)
- Prettier (Various languages)
Code Analysis Tools:
- SonarQube
- CodeClimate
- Codacy
Version Control Systems:
- Git
- GitHub/GitLab/Bitbucket
Continuous Integration/Continuous Deployment (CI/CD) Tools:
- Jenkins
- Travis CI
- CircleCI
Books on Clean Code:
- “Clean Code” by Robert C. Martin
- “Refactoring” by Martin Fowler
- “The Pragmatic Programmer” by Andrew Hunt and David Thomas
Incorporating these tools and resources into your development workflow can help you consistently write and maintain clean code.
12. Conclusion
Writing clean code is an essential skill for any programmer, regardless of their experience level or the specific technologies they work with. By following the best practices outlined in this guide – from using descriptive names and organizing your code structure to applying SOLID principles and conducting regular code reviews – you can significantly improve the quality, readability, and maintainability of your code.
Remember that writing clean code is an ongoing process that requires continuous learning and practice. As you work on projects, whether personal or professional, always strive to apply these principles and refine your coding skills. Not only will this make you a more effective developer, but it will also make you a valuable asset to any development team, especially when aiming for positions at top tech companies.
Clean code is more than just a set of rules; it’s a mindset and a commitment to craftsmanship in software development. By embracing these practices, you’ll be well on your way to becoming a skilled programmer capable of creating robust, efficient, and maintainable software solutions.
Keep practicing, stay curious, and always look for ways to improve your code. Happy coding!