Behind the Scenes: What Interviewers Are Really Looking For
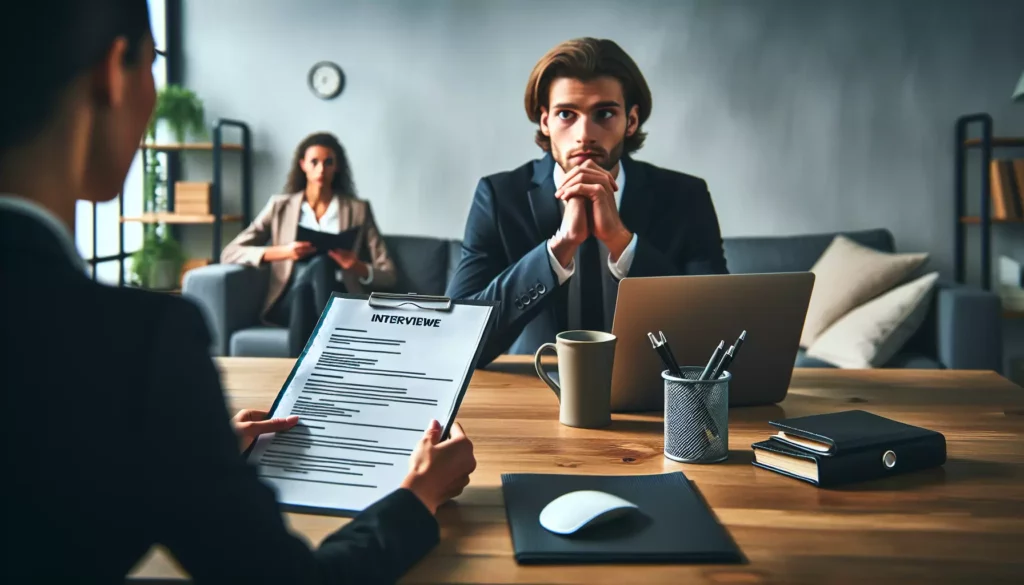
In the competitive world of tech, landing a job at a prestigious company like Google, Amazon, or Facebook (now Meta) is often seen as the ultimate goal for many aspiring programmers. But what exactly are these companies looking for when they interview candidates? As someone who has been on both sides of the interview table, I can tell you that it’s not just about coding skills. In this comprehensive guide, we’ll pull back the curtain and reveal what interviewers are really looking for when they’re assessing candidates for technical positions.
1. Problem-Solving Abilities
First and foremost, interviewers want to see how you approach and solve problems. It’s not just about getting the right answer; it’s about the process you use to get there.
What They’re Looking For:
- Analytical thinking
- Ability to break down complex problems into manageable parts
- Creativity in approaching challenges
- Resilience when faced with obstacles
How to Demonstrate It:
When given a coding problem, think out loud. Explain your thought process, consider multiple approaches, and discuss trade-offs. For example, if asked to reverse a linked list, you might say:
"Okay, let's think about this. We could iterate through the list once to get its length, then swap elements from the outside in. But that would take O(n) space and O(n) time. Alternatively, we could use a three-pointer approach, which would be O(1) space and O(n) time. Let's go with the second approach for better space efficiency."
2. Technical Proficiency
While problem-solving is crucial, technical skills are still a fundamental requirement. Interviewers want to ensure you have a solid grasp of computer science fundamentals and can implement solutions efficiently.
What They’re Looking For:
- Strong understanding of data structures and algorithms
- Proficiency in at least one programming language
- Knowledge of time and space complexity
- Familiarity with system design principles (for more senior roles)
How to Demonstrate It:
Practice implementing common algorithms and data structures from scratch. Be prepared to explain the time and space complexity of your solutions. For instance, when implementing a hash table, you might explain:
"I'm using an array of linked lists for this hash table implementation. The average-case time complexity for insertion, deletion, and lookup is O(1), but in the worst case, where all keys hash to the same bucket, it could degrade to O(n). The space complexity is O(n), where n is the number of key-value pairs stored."
3. Communication Skills
In today’s collaborative work environments, the ability to communicate clearly and effectively is invaluable. Interviewers are looking for candidates who can articulate their ideas and work well with others.
What They’re Looking For:
- Clear and concise explanation of technical concepts
- Active listening and ability to incorporate feedback
- Skill in asking clarifying questions
- Capacity to explain decisions and trade-offs
How to Demonstrate It:
Practice explaining complex topics to non-technical friends or family members. During the interview, ask questions to clarify requirements and constraints. When proposing a solution, explain your reasoning. For example:
"Before we dive into coding, can I clarify a few things? What's the expected scale of this system? Are we optimizing for read or write performance? Given that we're dealing with financial data, I assume data consistency is crucial - is that correct?"
4. Cultural Fit and Soft Skills
Tech giants aren’t just looking for coding machines; they want well-rounded individuals who can contribute to the company culture and work effectively in teams.
What They’re Looking For:
- Adaptability and willingness to learn
- Teamwork and collaboration skills
- Leadership potential (especially for senior roles)
- Alignment with company values
How to Demonstrate It:
Research the company’s values and culture before the interview. Prepare examples from your past experiences that demonstrate these qualities. For instance:
"In my last project, we faced a major setback when a key API was deprecated. I took the initiative to research alternatives and presented three options to the team. We collaboratively decided on the best approach, and I led the implementation, resulting in minimal delay to our project timeline."
5. Passion for Technology
Interviewers love to see candidates who are genuinely excited about technology and stay up-to-date with the latest developments in the field.
What They’re Looking For:
- Enthusiasm for learning new technologies
- Side projects or contributions to open-source
- Awareness of current trends in tech
- Curiosity and desire to understand how things work
How to Demonstrate It:
Talk about personal projects, tech blogs you follow, or recent technologies you’ve been exploring. For example:
"I've been fascinated by the potential of WebAssembly lately. I've been experimenting with compiling Rust to WASM for high-performance web applications. It's amazing how it can bring near-native speed to the browser while still maintaining the security sandbox."
6. Code Quality and Best Practices
Writing code that works is one thing, but writing code that’s clean, maintainable, and follows best practices is what separates good programmers from great ones.
What They’re Looking For:
- Clean, readable, and well-organized code
- Proper naming conventions and commenting
- Adherence to principles like DRY (Don’t Repeat Yourself) and SOLID
- Consideration for edge cases and error handling
How to Demonstrate It:
When writing code during the interview, think aloud about your coding choices. Explain why you’re structuring your code in a certain way. For instance:
"I'm going to create a separate function for this validation logic because it's likely to be reused. This adheres to the DRY principle and makes the code more maintainable. I'll also add a comment explaining the validation rules for future reference."
7. System Design and Scalability
Especially for more senior positions, interviewers want to see that you can think beyond individual components and understand how to design and scale entire systems.
What They’re Looking For:
- Understanding of distributed systems
- Knowledge of caching, load balancing, and database sharding
- Ability to make appropriate trade-offs in system design
- Awareness of real-world constraints and limitations
How to Demonstrate It:
When faced with a system design question, start by clarifying requirements and constraints. Then, walk through your design process step by step. For example:
"For this URL shortening service, we'll need a few key components. First, a load balancer to distribute incoming requests. Then, a set of application servers to handle the logic. For data storage, we'll use a NoSQL database like Cassandra for its high write throughput and horizontal scalability. To improve read performance, we'll implement a caching layer using Redis."
8. Debugging and Troubleshooting Skills
The ability to efficiently identify and fix issues is crucial in any development role. Interviewers often look for candidates who can systematically approach debugging.
What They’re Looking For:
- Systematic approach to problem identification
- Ability to use debugging tools effectively
- Skill in reading and understanding error messages
- Patience and persistence in solving complex issues
How to Demonstrate It:
If you encounter a bug during a coding interview, don’t panic. Instead, narrate your debugging process:
"It looks like we're getting an 'IndexOutOfBoundsException'. Let's add some print statements to see the value of our index at each iteration. Ah, I see the issue - we're not updating our loop counter correctly. Let's fix that and run it again."
9. Adaptability and Learning Agility
In the fast-paced tech world, the ability to quickly learn and adapt to new technologies and situations is invaluable.
What They’re Looking For:
- Openness to feedback and willingness to improve
- Ability to quickly grasp new concepts
- Flexibility in approach when initial solutions don’t work
- Comfort with ambiguity and changing requirements
How to Demonstrate It:
During the interview, if you’re not familiar with a concept, be honest and show your process for learning:
"I haven't worked with that specific technology before, but it sounds similar to X, which I have experience with. In a situation like this, I would typically start by reading the official documentation, then look for tutorials or courses to get hands-on practice. Can you tell me more about how you use this technology in your projects?"
10. Time Management and Prioritization
Interviewers want to see that you can manage your time effectively and prioritize tasks, especially when dealing with complex problems under time constraints.
What They’re Looking For:
- Ability to estimate task complexity and duration
- Skill in breaking down large problems into manageable tasks
- Capacity to focus on critical aspects first
- Awareness of when to ask for help or clarification
How to Demonstrate It:
When given a complex problem, outline your approach before diving into coding:
"Let's break this down into steps. First, we'll need to parse the input, which should take about 5 minutes. Then, we'll implement the core algorithm, which is the most critical part - let's allocate 20 minutes for that. Finally, we'll handle edge cases and optimize if time allows. Does this approach sound reasonable?"
11. Testing and Quality Assurance Mindset
Writing code is only part of the development process. Interviewers also want to see that you understand the importance of testing and can write reliable, bug-free code.
What They’re Looking For:
- Understanding of different types of testing (unit, integration, end-to-end)
- Ability to write testable code
- Skill in identifying edge cases and potential bugs
- Proactive approach to quality assurance
How to Demonstrate It:
After implementing a solution, discuss how you would test it:
"Now that we have the basic implementation, let's think about testing. We should start with unit tests for each function, ensuring they handle normal cases, edge cases, and potential error conditions. For example, we'd want to test our sorting function with an empty array, an array with one element, and arrays of various sizes. We should also consider integration tests to ensure the components work together correctly."
12. Version Control and Collaboration Tools
Modern software development is a collaborative effort, and familiarity with version control systems and collaboration tools is often expected.
What They’re Looking For:
- Proficiency with Git or other version control systems
- Understanding of branching strategies and merge conflict resolution
- Familiarity with code review processes
- Experience with project management and collaboration tools
How to Demonstrate It:
When discussing your experience, mention your workflow and collaboration practices:
"In my previous role, we used Git with a feature branch workflow. I'm comfortable with creating branches, submitting pull requests, and addressing code review feedback. We also used JIRA for project management and Slack for team communication. I find that clear communication and well-organized code repositories significantly improve team productivity."
13. Security Awareness
With the increasing importance of cybersecurity, interviewers often look for candidates who understand basic security principles and can write secure code.
What They’re Looking For:
- Understanding of common security vulnerabilities (e.g., SQL injection, XSS)
- Knowledge of secure coding practices
- Awareness of data privacy concerns
- Familiarity with security tools and practices (e.g., encryption, authentication)
How to Demonstrate It:
When discussing your solutions, mention security considerations:
"For this user input field, we'll need to implement input validation to prevent XSS attacks. We should also use parameterized queries when interacting with the database to prevent SQL injection. Additionally, we'll want to ensure that all sensitive data is encrypted both in transit and at rest."
14. Performance Optimization Skills
As applications grow in complexity and scale, the ability to optimize performance becomes increasingly important.
What They’re Looking For:
- Understanding of performance bottlenecks and how to identify them
- Knowledge of optimization techniques for both time and space complexity
- Familiarity with profiling tools
- Ability to make informed trade-offs between performance and other factors
How to Demonstrate It:
When discussing your solution, consider potential optimizations:
"This solution works, but we can optimize it further. By using a hash map instead of a nested loop, we can reduce the time complexity from O(n^2) to O(n). This trades some space complexity for significant time savings, which is worthwhile for large inputs. In a real-world scenario, we'd want to profile the application to identify the most impactful optimizations."
15. Continuous Learning and Growth Mindset
Finally, interviewers want to see candidates who are committed to continuous learning and professional growth.
What They’re Looking For:
- Proactive approach to learning new technologies and skills
- Ability to reflect on past experiences and learn from them
- Openness to feedback and willingness to improve
- Interest in mentoring and knowledge sharing
How to Demonstrate It:
Share your approach to professional development:
"I'm always looking to expand my skills. Recently, I've been focusing on machine learning, taking online courses and working on personal projects to apply what I've learned. I also enjoy attending tech meetups and conferences to stay current with industry trends. In my last role, I initiated a weekly 'tech talk' series where team members could share knowledge on various topics."
Conclusion
Landing a job at a top tech company is about much more than just coding skills. Interviewers are looking for well-rounded candidates who can solve problems, communicate effectively, work well in teams, and continually adapt and learn. By understanding and demonstrating these qualities, you’ll be well-positioned to impress interviewers and land your dream job.
Remember, the interview process is not just about the company evaluating you – it’s also an opportunity for you to assess whether the company and role are a good fit for your goals and values. Approach interviews with confidence, be authentic, and let your passion for technology shine through. With preparation and the right mindset, you can navigate the interview process successfully and take the next big step in your tech career.
Good luck with your interviews, and may your coding journey be filled with growth, challenges, and exciting opportunities!