Behaviour-Driven Development: A Comprehensive Guide for Modern Software Engineering
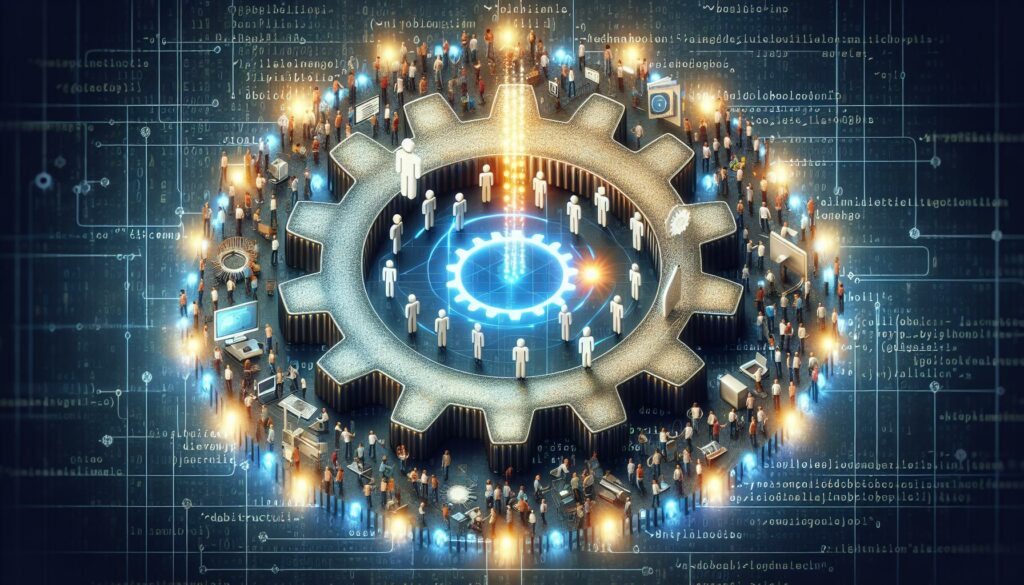
In the ever-evolving landscape of software development, methodologies and practices continually emerge to enhance the efficiency, quality, and collaboration in building software products. One such approach that has gained significant traction in recent years is Behaviour-Driven Development (BDD). This comprehensive guide will delve into the intricacies of BDD, exploring its principles, benefits, and practical implementation in modern software engineering projects.
What is Behaviour-Driven Development?
Behaviour-Driven Development is an agile software development process that encourages collaboration between developers, quality assurance testers, and non-technical or business participants in a software project. It extends the Test-Driven Development (TDD) approach by focusing on the behavior of the system from the end user’s perspective.
BDD aims to create a shared understanding of how an application should behave by using a common language that can be understood by all stakeholders. This approach helps bridge the gap between technical and non-technical team members, ensuring that everyone is on the same page regarding the project’s goals and requirements.
The Core Principles of BDD
To fully grasp the concept of Behaviour-Driven Development, it’s essential to understand its core principles:
- Focus on Business Value: BDD emphasizes defining features that deliver tangible business value.
- Ubiquitous Language: It promotes the use of a common language that all stakeholders can understand and use to describe the system’s behavior.
- Collaboration: BDD encourages close collaboration between developers, testers, and business stakeholders throughout the development process.
- Examples: It uses concrete examples to illustrate the desired behavior of the system.
- Automation: BDD supports the automation of behavioral specifications to ensure continuous validation of the system’s behavior.
The BDD Process
The BDD process typically follows these steps:
- Discovery: Stakeholders collaborate to explore, discover, and agree on the behaviors that the system should exhibit.
- Formulation: The agreed-upon behaviors are formulated into concrete examples and scenarios.
- Automation: The scenarios are automated into executable specifications.
- Implementation: The actual code is written to implement the behavior described in the scenarios.
- Verification: The automated scenarios are run to verify that the implemented behavior matches the expected behavior.
BDD vs. TDD: Understanding the Differences
While BDD is often seen as an extension of Test-Driven Development (TDD), there are some key differences between the two approaches:
Aspect | BDD | TDD |
---|---|---|
Focus | Behavior of the system | Individual units of code |
Language | Natural language (Gherkin) | Programming language |
Stakeholders | Developers, testers, business analysts | Primarily developers |
Scope | Entire feature or user story | Individual functions or methods |
Primary Goal | Ensure software meets business requirements | Ensure code correctness and design |
The Benefits of Implementing BDD
Adopting Behaviour-Driven Development in your software development process can yield numerous benefits:
- Improved Communication: BDD fosters better communication between technical and non-technical team members by using a common language.
- Reduced Misunderstandings: The use of concrete examples helps clarify requirements and reduce misinterpretations.
- Faster Feedback: Automated scenarios provide quick feedback on whether the implemented behavior matches the expected behavior.
- Higher Quality Software: By focusing on behavior and user scenarios, BDD helps create software that better meets user needs.
- Better Documentation: BDD scenarios serve as living documentation that is always up-to-date with the current system behavior.
- Increased Collaboration: The BDD process encourages collaboration between different roles in the development team.
- Reduced Development Costs: By catching misunderstandings and issues early in the development process, BDD can help reduce overall development costs.
Implementing BDD: Tools and Frameworks
To implement Behaviour-Driven Development effectively, various tools and frameworks are available. Some popular ones include:
- Cucumber: A widely used tool that supports BDD in multiple programming languages.
- SpecFlow: A BDD framework for .NET developers.
- JBehave: A framework for behavior-driven development in Java.
- Behat: A BDD framework for PHP.
- Jasmine: A behavior-driven development framework for testing JavaScript code.
Let’s take a closer look at how to use Cucumber, one of the most popular BDD tools, to implement a simple scenario.
Using Cucumber for BDD
Cucumber uses a language called Gherkin to define test cases in a human-readable format. Here’s an example of a Gherkin scenario:
Feature: User Login
Scenario: Successful login with valid credentials
Given the user is on the login page
When the user enters valid username and password
And clicks the login button
Then the user should be redirected to the dashboard
To implement this scenario using Cucumber with Ruby, you would create step definitions that map to each step in the scenario:
Given(/^the user is on the login page$/) do
visit login_path
end
When(/^the user enters valid username and password$/) do
fill_in 'Username', with: 'validuser'
fill_in 'Password', with: 'validpassword'
end
And(/^clicks the login button$/) do
click_button 'Login'
end
Then(/^the user should be redirected to the dashboard$/) do
expect(current_path).to eq(dashboard_path)
end
These step definitions would then be executed when running the Cucumber tests, automating the verification of the described behavior.
Best Practices for Implementing BDD
To get the most out of Behaviour-Driven Development, consider the following best practices:
- Start with the Big Picture: Begin by defining high-level features and user stories before diving into specific scenarios.
- Use Ubiquitous Language: Ensure that all stakeholders use the same terminology to describe the system’s behavior.
- Focus on Business Value: Prioritize scenarios that deliver the most business value.
- Keep Scenarios Simple: Write clear, concise scenarios that focus on one specific behavior.
- Use Real-world Examples: Base your scenarios on real-world use cases to make them more relatable and understandable.
- Involve All Stakeholders: Ensure that developers, testers, and business analysts all participate in the BDD process.
- Automate Wisely: While automation is important, not all scenarios need to be automated. Focus on automating the most critical and frequently changing behaviors.
- Maintain Living Documentation: Keep your BDD scenarios up-to-date as the system evolves to serve as accurate documentation.
Challenges in Adopting BDD
While Behaviour-Driven Development offers numerous benefits, it’s not without its challenges. Some common obstacles in adopting BDD include:
- Resistance to Change: Team members may be resistant to changing their existing development processes.
- Learning Curve: There’s a learning curve associated with BDD concepts and tools.
- Time Investment: Initially, BDD may seem to slow down the development process as teams adjust to the new approach.
- Maintaining Scenarios: As the system evolves, keeping all scenarios up-to-date can be challenging.
- Over-specification: There’s a risk of creating too many detailed scenarios, which can become burdensome to maintain.
To overcome these challenges, it’s crucial to provide proper training, start with small pilot projects, and gradually scale up BDD adoption across the organization.
BDD in the Context of Agile and DevOps
Behaviour-Driven Development aligns well with Agile methodologies and DevOps practices. In an Agile context, BDD supports the iterative and incremental development approach by providing a way to continuously validate that the software meets business requirements.
In a DevOps environment, BDD can be integrated into the continuous integration and continuous delivery (CI/CD) pipeline. Automated BDD scenarios can be run as part of the CI process, providing quick feedback on whether new code changes have broken existing behavior. This helps catch issues early and ensures that only code that passes all behavior specifications is deployed to production.
The Future of BDD
As software development continues to evolve, so does Behaviour-Driven Development. Some trends and potential future directions for BDD include:
- AI-assisted Scenario Generation: AI could help generate more comprehensive and edge-case scenarios based on existing specifications.
- Natural Language Processing: Improved NLP could enhance the ability to translate natural language scenarios into executable tests.
- Visual BDD: Tools that allow for visual representation and manipulation of BDD scenarios could make the process more intuitive.
- BDD for Microservices: As microservices architecture becomes more prevalent, BDD practices may evolve to better support this paradigm.
- BDD in Low-Code/No-Code Platforms: BDD principles could be integrated into low-code and no-code development platforms to ensure behavior-driven approach even in these environments.
Conclusion
Behaviour-Driven Development represents a powerful approach to software development that bridges the gap between technical and non-technical stakeholders. By focusing on the behavior of the system from the user’s perspective, BDD helps create software that truly meets business needs.
While implementing BDD comes with its challenges, the benefits in terms of improved communication, higher quality software, and better alignment with business goals make it a valuable methodology to consider. As with any development approach, the key to success with BDD lies in understanding its principles, adapting it to your specific context, and continuously refining your practice.
As we look to the future of software development, BDD is likely to play an increasingly important role in creating user-centric, high-quality software solutions. Whether you’re a developer, tester, or business analyst, understanding and applying BDD principles can significantly enhance your ability to contribute to successful software projects.