Behavioral-Driven Development (BDD): Enhancing Collaboration in Software Development
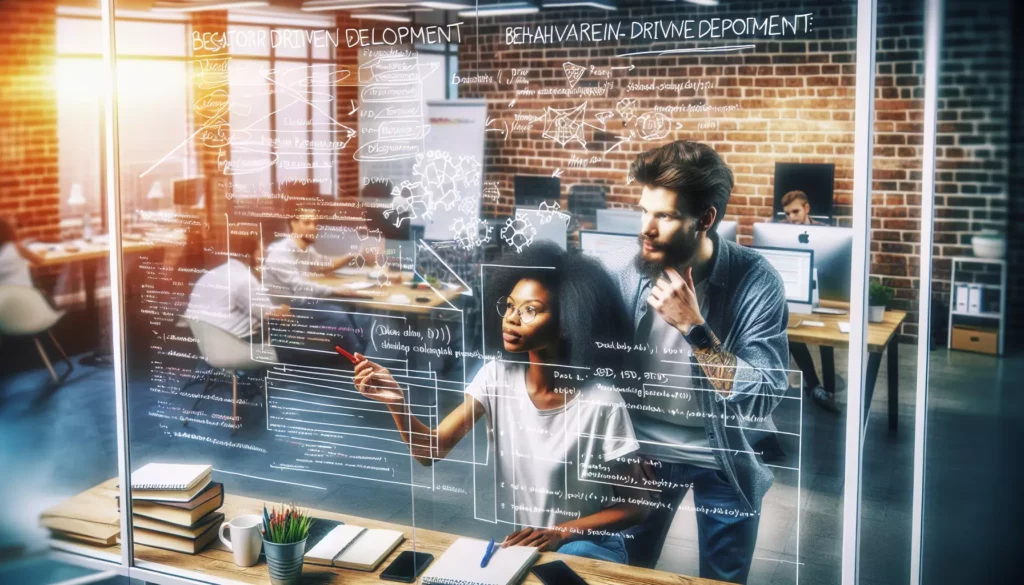
In the ever-evolving landscape of software development methodologies, Behavioral-Driven Development (BDD) has emerged as a powerful approach to enhance collaboration between technical and non-technical team members. This article delves deep into the world of BDD, exploring its principles, benefits, and practical implementation in the context of modern software development practices.
What is Behavioral-Driven Development?
Behavioral-Driven Development is an agile software development process that encourages collaboration between developers, QA testers, and non-technical or business participants in a software project. It extends the Test-Driven Development (TDD) approach by focusing on the behavior of the system from the end user’s perspective.
BDD aims to create a shared understanding of how an application should behave by describing its functionality in a natural language that all stakeholders can understand. This approach bridges the gap between technical and non-technical team members, fostering better communication and alignment on project goals.
The Core Principles of BDD
To fully grasp the concept of Behavioral-Driven Development, it’s essential to understand its core principles:
- Collaboration: BDD emphasizes the importance of involving all stakeholders in the development process, including developers, testers, and business representatives.
- Focus on Value: By describing behavior from the user’s perspective, BDD ensures that development efforts are aligned with business value.
- Ubiquitous Language: BDD promotes the use of a common language that all team members can understand, reducing misunderstandings and improving communication.
- Iterative Development: BDD encourages an iterative approach to development, allowing for continuous feedback and improvement.
- Automation: While not mandatory, BDD often involves automating behavior specifications to create living documentation and executable tests.
The BDD Process
The BDD process typically follows these steps:
- Discovery: Stakeholders collaborate to explore, discuss, and agree on the desired behavior of the system.
- Formulation: The agreed-upon behaviors are documented in a structured, natural language format (often using Gherkin syntax).
- Automation: Developers create automated tests based on the behavior specifications.
- Implementation: Code is written to satisfy the behavior specifications and pass the automated tests.
- Verification: The implemented features are verified against the original behavior specifications.
BDD vs. TDD: Understanding the Difference
While BDD and TDD share some similarities, they have distinct differences:
Aspect | BDD | TDD |
---|---|---|
Focus | Behavior of the system | Individual units of code |
Language | Natural language (e.g., Gherkin) | Programming language |
Stakeholders | Developers, testers, business representatives | Primarily developers |
Scope | System-level behavior | Unit-level functionality |
Tools and Frameworks for BDD
Several tools and frameworks support the implementation of BDD in software development projects:
- Cucumber: A popular BDD tool that supports multiple programming languages and uses Gherkin syntax for behavior specifications.
- SpecFlow: A .NET-based BDD framework that integrates with Visual Studio and supports Gherkin syntax.
- JBehave: A Java-based BDD framework that allows for behavior-driven development in Java projects.
- Behat: A PHP-based BDD framework that supports behavior-driven development for PHP applications.
- Jasmine: A behavior-driven development framework for testing JavaScript code.
Writing Effective BDD Scenarios
One of the key aspects of BDD is writing clear and effective behavior scenarios. These scenarios typically follow the Given-When-Then format, which helps structure the behavior in a way that’s easy to understand and implement. Here’s an example of a BDD scenario using Gherkin syntax:
Feature: User Login
Scenario: Successful login
Given the user is on the login page
When the user enters valid credentials
And clicks the login button
Then the user should be redirected to the dashboard
And see a welcome message
When writing BDD scenarios, keep these tips in mind:
- Use clear and concise language that all stakeholders can understand.
- Focus on describing the behavior, not the implementation details.
- Keep scenarios independent and atomic.
- Use realistic and meaningful examples.
- Avoid technical jargon unless necessary.
Benefits of Implementing BDD
Adopting Behavioral-Driven Development in your software development process can bring numerous benefits:
- Improved Collaboration: BDD fosters better communication and understanding between technical and non-technical team members.
- Clearer Requirements: By focusing on behavior, BDD helps clarify and refine project requirements.
- Reduced Misunderstandings: The use of a ubiquitous language minimizes misinterpretations of project goals and features.
- Better Alignment with Business Goals: BDD ensures that development efforts are directly tied to business value and user needs.
- Living Documentation: BDD scenarios serve as up-to-date documentation of the system’s behavior.
- Faster Feedback: The iterative nature of BDD allows for quicker feedback and course correction.
- Improved Quality: By focusing on behavior and automating tests, BDD can lead to higher quality software.
Challenges in Implementing BDD
While BDD offers many advantages, it’s important to be aware of potential challenges:
- Learning Curve: Team members may need time to adapt to the BDD approach and learn new tools.
- Time Investment: Initially, BDD may require more time for planning and writing scenarios.
- Maintaining Scenarios: As the project evolves, keeping BDD scenarios up-to-date can be challenging.
- Overcomplicating Scenarios: There’s a risk of creating overly complex or numerous scenarios, which can be counterproductive.
- Resistance to Change: Some team members may resist adopting a new development methodology.
Best Practices for Successful BDD Implementation
To maximize the benefits of BDD and overcome potential challenges, consider these best practices:
- Start Small: Begin by implementing BDD on a small project or feature to gain experience and build confidence.
- Provide Training: Ensure all team members understand BDD principles and practices through proper training and resources.
- Focus on Collaboration: Encourage active participation from all stakeholders in the BDD process.
- Keep Scenarios Simple: Write clear, concise scenarios that focus on essential behaviors.
- Automate Wisely: While automation is beneficial, be selective about which scenarios to automate to maintain efficiency.
- Regularly Review and Refine: Continuously evaluate and improve your BDD process and scenarios.
- Integrate with Existing Practices: Combine BDD with other agile practices for a comprehensive development approach.
BDD in the Context of AlgoCademy
As we explore Behavioral-Driven Development, it’s worth considering how this approach aligns with the goals and focus of AlgoCademy, a platform dedicated to coding education and programming skills development.
AlgoCademy emphasizes interactive coding tutorials, resources for learners, and tools to help individuals progress from beginner-level coding to preparing for technical interviews. The platform’s focus on algorithmic thinking, problem-solving, and practical coding skills can benefit from the principles of BDD in several ways:
- Clear Learning Objectives: BDD’s emphasis on behavior specification can help create clear, user-focused learning objectives for coding tutorials and exercises.
- Interactive Scenarios: The Given-When-Then format of BDD scenarios can be adapted to create interactive coding challenges that simulate real-world problems.
- Collaborative Learning: BDD’s collaborative nature can be leveraged to create peer programming exercises or group projects that mimic real-world development scenarios.
- Practical Skill Development: By incorporating BDD principles into coding exercises, learners can gain experience with industry-standard practices used in major tech companies.
- Interview Preparation: Understanding BDD can give learners an edge in technical interviews, as many companies value knowledge of agile development practices.
By integrating BDD concepts into its curriculum, AlgoCademy can provide a more holistic learning experience that goes beyond just coding skills, preparing learners for the collaborative and behavior-focused nature of modern software development.
Case Study: BDD in Action
To illustrate the practical application of BDD, let’s consider a hypothetical case study of a team developing a new feature for an e-commerce platform:
Project: Adding a “Wishlist” Feature
The development team is tasked with adding a wishlist feature to an existing e-commerce platform. They decide to use BDD to ensure clear communication and alignment with business goals.
Step 1: Discovery
The team holds a discovery workshop involving developers, testers, and business stakeholders. They discuss the desired behavior of the wishlist feature, considering user needs and business objectives.
Step 2: Formulation
Based on the discovery session, the team formulates BDD scenarios. Here’s an example:
Feature: Wishlist
Scenario: Adding a product to the wishlist
Given a logged-in user is viewing a product
When the user clicks the "Add to Wishlist" button
Then the product should be added to the user's wishlist
And the user should see a confirmation message
Scenario: Viewing the wishlist
Given a logged-in user with items in their wishlist
When the user navigates to the wishlist page
Then they should see a list of all items in their wishlist
And each item should display its name, price, and an option to remove it
Step 3: Automation
The development team uses Cucumber to automate these scenarios, creating step definitions that link the natural language scenarios to executable code.
Step 4: Implementation
Developers implement the wishlist feature, guided by the BDD scenarios. They write code to satisfy each step of the scenarios.
Step 5: Verification
The team runs the automated tests to verify that the implemented feature meets the specified behavior. They also conduct manual testing to ensure the feature works as expected from a user’s perspective.
Results
By using BDD, the team experiences several benefits:
- Clear understanding of requirements among all team members
- Reduced miscommunication between developers and business stakeholders
- Early detection of potential issues or misalignments
- Automated tests that serve as living documentation of the feature’s behavior
- Increased confidence in the quality of the implemented feature
This case study demonstrates how BDD can streamline the development process, improve communication, and ensure that the final product meets both user needs and business objectives.
The Future of BDD
As software development continues to evolve, Behavioral-Driven Development is likely to play an increasingly important role. Some trends and potential future developments in BDD include:
- Integration with AI and Machine Learning: AI could be used to generate or suggest BDD scenarios based on project requirements or user stories.
- Enhanced Visualization Tools: New tools may emerge to better visualize and manage BDD scenarios, making them more accessible to non-technical stakeholders.
- Closer Integration with DevOps: BDD practices may become more tightly integrated with DevOps pipelines, further streamlining the development and deployment process.
- Expansion into New Domains: While BDD is primarily used in software development, its principles could be adapted for other fields such as hardware development or business process design.
- Improved Natural Language Processing: Advancements in NLP could lead to more sophisticated tools for writing and interpreting BDD scenarios.
Conclusion
Behavioral-Driven Development represents a significant shift in how we approach software development, emphasizing collaboration, clear communication, and a focus on user-centric behavior. By bridging the gap between technical and non-technical stakeholders, BDD helps ensure that development efforts are aligned with business goals and user needs.
While implementing BDD may present some challenges, the benefits it offers in terms of improved collaboration, clearer requirements, and higher quality software make it a valuable methodology for many development teams. As the software industry continues to evolve, BDD is likely to play an increasingly important role in creating user-focused, high-quality applications.
For those looking to enhance their software development skills, understanding and implementing BDD can be a valuable addition to their toolkit. Whether you’re a seasoned developer or just starting your journey in coding, embracing BDD principles can help you create more effective, user-centered software solutions.
As we’ve seen in the context of platforms like AlgoCademy, the principles of BDD can also be valuable in educational settings, helping to bridge the gap between theoretical knowledge and practical, industry-relevant skills. By incorporating BDD concepts into learning experiences, aspiring developers can gain a more holistic understanding of modern software development practices.
In conclusion, Behavioral-Driven Development offers a powerful approach to software development that aligns technical implementation with business value and user needs. As you continue your journey in software development, consider how BDD principles can enhance your projects and contribute to creating more effective, user-centered software solutions.