Bartending Algorithms: Mixing the Perfect Coding Cocktail for Interview Success
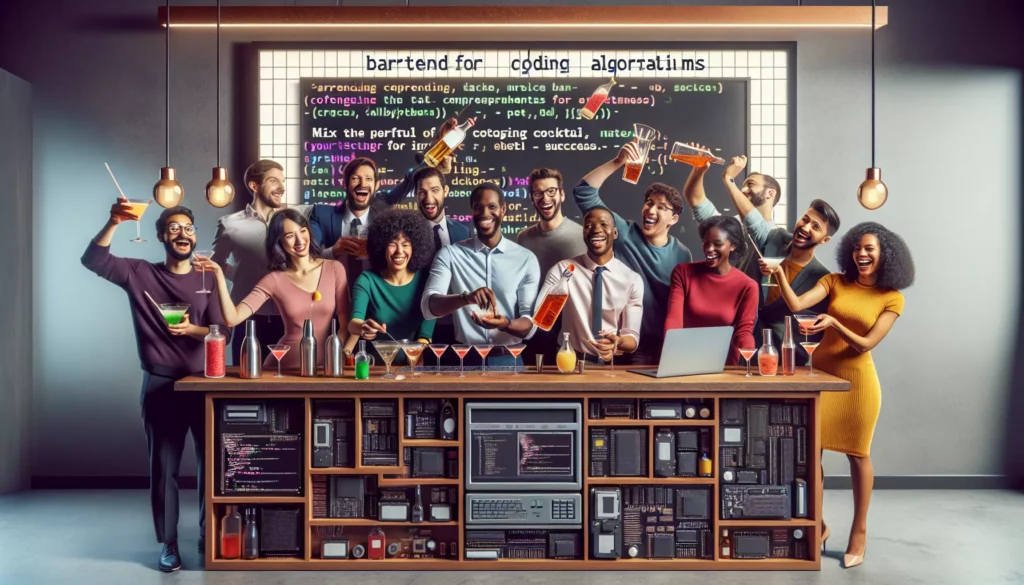
Welcome to the AlgoCademy mixology class, where we’re about to shake up your coding skills and stir your algorithmic thinking! Just as a master bartender crafts the perfect cocktail, a skilled programmer blends various algorithms and data structures to concoct elegant solutions to complex problems. In this comprehensive guide, we’ll explore how the art of bartending can teach us valuable lessons about coding and help you prepare for technical interviews at top tech companies.
The Ingredients: Essential Algorithms and Data Structures
Before we start mixing our coding cocktails, let’s stock our bar with the essential ingredients. In the world of programming, these are the fundamental algorithms and data structures that form the basis of many complex solutions:
1. Arrays and Strings: The Basic Spirits
Arrays and strings are the vodka and rum of our coding bar – versatile, widely used, and the foundation for many algorithms. Understanding how to manipulate these data structures efficiently is crucial for any aspiring programmer.
Key operations to master:
- Traversing
- Searching
- Sorting
- Insertion and deletion
2. Linked Lists: The Mixers
Like the sodas and juices that add complexity to a cocktail, linked lists bring dynamic flavor to our code. They offer flexibility in insertion and deletion operations, making them ideal for certain types of problems.
Essential linked list concepts:
- Singly and doubly linked lists
- Insertion and deletion at various positions
- Reversing a linked list
- Detecting cycles
3. Stacks and Queues: The Shakers and Stirrers
Just as shakers and stirrers are essential tools for a bartender, stacks and queues are fundamental data structures for managing data flow in algorithms. They’re particularly useful in parsing and graph traversal problems.
Key operations:
- Push and pop for stacks
- Enqueue and dequeue for queues
- Implementing stacks and queues using arrays and linked lists
4. Trees and Graphs: The Complex Liqueurs
Trees and graphs add depth and sophistication to our coding cocktails, much like fine liqueurs in mixology. These structures are essential for representing hierarchical and networked data.
Important concepts:
- Binary trees and binary search trees
- Tree traversals (in-order, pre-order, post-order)
- Graph representations (adjacency list, adjacency matrix)
- Graph traversals (BFS, DFS)
5. Hash Tables: The Flavor Enhancers
Hash tables are like bitters or syrups in cocktails – they can dramatically improve the efficiency of our algorithms by providing fast lookup and insertion operations.
Key aspects to understand:
- Hash functions
- Collision resolution techniques
- Time complexity analysis
The Techniques: Algorithmic Problem-Solving Approaches
Now that we have our ingredients, let’s explore some bartending techniques that parallel important problem-solving approaches in coding:
1. The Classic Cocktail: Brute Force
Sometimes, the straightforward approach is the best. Like mixing a classic martini, brute force algorithms solve problems by exploring all possible solutions. While not always efficient, understanding this technique is crucial as it often forms the basis for more sophisticated approaches.
Example: Finding the maximum subarray sum
def max_subarray_sum_brute_force(arr):
n = len(arr)
max_sum = float('-inf')
for i in range(n):
current_sum = 0
for j in range(i, n):
current_sum += arr[j]
max_sum = max(max_sum, current_sum)
return max_sum
# Usage
arr = [-2, 1, -3, 4, -1, 2, 1, -5, 4]
print(max_subarray_sum_brute_force(arr)) # Output: 6
2. The Layered Cocktail: Divide and Conquer
Like creating a layered cocktail, divide and conquer algorithms break down complex problems into smaller, manageable subproblems. This technique is often used in efficient sorting and searching algorithms.
Example: Merge Sort
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
# Usage
arr = [38, 27, 43, 3, 9, 82, 10]
sorted_arr = merge_sort(arr)
print(sorted_arr) # Output: [3, 9, 10, 27, 38, 43, 82]
3. The Infusion Technique: Dynamic Programming
Dynamic programming is like infusing spirits with flavors over time. It involves breaking down a problem into smaller subproblems and storing their solutions to avoid redundant computations. This technique is powerful for optimization problems.
Example: Fibonacci sequence with memoization
def fibonacci(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci(n-1, memo) + fibonacci(n-2, memo)
return memo[n]
# Usage
print(fibonacci(10)) # Output: 55
4. The Reduction Method: Greedy Algorithms
Greedy algorithms, like reducing a cocktail to intensify flavors, make locally optimal choices at each step with the hope of finding a global optimum. While not always guaranteed to find the best solution, they’re often efficient and work well for many problems.
Example: Coin change problem (assuming coins of values 1, 5, 10, 25)
def coin_change_greedy(amount):
coins = [25, 10, 5, 1]
result = []
for coin in coins:
while amount >= coin:
result.append(coin)
amount -= coin
return result
# Usage
print(coin_change_greedy(67)) # Output: [25, 25, 10, 5, 1, 1]
The Presentation: Clean Code and Optimization
Just as the presentation of a cocktail is crucial, the way we write and optimize our code is essential for interview success. Let’s explore some key aspects:
1. Code Clarity: The Clear Ice Cube
Clear, well-structured code is like a perfectly clear ice cube in a premium cocktail. It enhances the overall quality and makes your solution more appealing to interviewers.
Tips for writing clear code:
- Use meaningful variable and function names
- Keep functions small and focused
- Use comments judiciously to explain complex logic
- Follow consistent formatting and style guidelines
2. Time Complexity: The Smooth Pour
Optimizing the time complexity of your algorithms is like perfecting the smooth pour of a cocktail. It demonstrates your skill and understanding of efficiency.
Key points to remember:
- Understand Big O notation and be able to analyze your code’s time complexity
- Look for opportunities to reduce nested loops or use more efficient data structures
- Be prepared to discuss trade-offs between time and space complexity
3. Space Complexity: The Right Glass
Managing space complexity is like choosing the right glass for a cocktail. It’s about using memory efficiently and appropriately for the problem at hand.
Considerations for space optimization:
- Use in-place algorithms when possible
- Be mindful of creating unnecessary copies of data structures
- Understand when it’s appropriate to trade space for time (and vice versa)
The Tasting Menu: Common Interview Questions
Now that we’ve covered the ingredients and techniques, let’s sample a tasting menu of common coding interview questions. These problems will help you apply the concepts we’ve discussed and prepare for the real interview experience.
1. The Aperitif: Two Sum
Problem: Given an array of integers and a target sum, return indices of two numbers such that they add up to the target.
def two_sum(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
# Usage
nums = [2, 7, 11, 15]
target = 9
print(two_sum(nums, target)) # Output: [0, 1]
2. The First Course: Reverse a Linked List
Problem: Reverse a singly linked list.
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def reverse_linked_list(head):
prev = None
current = head
while current:
next_node = current.next
current.next = prev
prev = current
current = next_node
return prev
# Usage
# Create a linked list: 1 -> 2 -> 3 -> 4 -> 5
head = ListNode(1)
head.next = ListNode(2)
head.next.next = ListNode(3)
head.next.next.next = ListNode(4)
head.next.next.next.next = ListNode(5)
# Reverse the linked list
new_head = reverse_linked_list(head)
# Print the reversed list
while new_head:
print(new_head.val, end=" ")
new_head = new_head.next
# Output: 5 4 3 2 1
3. The Main Course: Longest Substring Without Repeating Characters
Problem: Find the length of the longest substring without repeating characters.
def length_of_longest_substring(s):
char_index = {}
max_length = 0
start = 0
for end, char in enumerate(s):
if char in char_index and char_index[char] >= start:
start = char_index[char] + 1
else:
max_length = max(max_length, end - start + 1)
char_index[char] = end
return max_length
# Usage
s = "abcabcbb"
print(length_of_longest_substring(s)) # Output: 3
4. The Dessert: Merge K Sorted Lists
Problem: Merge k sorted linked lists into one sorted linked list.
import heapq
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def merge_k_lists(lists):
heap = []
dummy = ListNode(0)
current = dummy
# Add the head of each list to the heap
for i, lst in enumerate(lists):
if lst:
heapq.heappush(heap, (lst.val, i, lst))
while heap:
val, i, node = heapq.heappop(heap)
current.next = node
current = current.next
if node.next:
heapq.heappush(heap, (node.next.val, i, node.next))
return dummy.next
# Usage
# Create 3 sorted linked lists
list1 = ListNode(1)
list1.next = ListNode(4)
list1.next.next = ListNode(5)
list2 = ListNode(1)
list2.next = ListNode(3)
list2.next.next = ListNode(4)
list3 = ListNode(2)
list3.next = ListNode(6)
# Merge the lists
merged = merge_k_lists([list1, list2, list3])
# Print the merged list
while merged:
print(merged.val, end=" ")
merged = merged.next
# Output: 1 1 2 3 4 4 5 6
The Garnish: Soft Skills and Interview Etiquette
Just as a garnish adds the finishing touch to a cocktail, your soft skills and interview etiquette can make a lasting impression on your interviewers. Here are some tips to polish your performance:
1. Communication: The Art of Description
Clearly explaining your thought process is as important as solving the problem itself. Practice talking through your solutions out loud, as if you were describing a complex cocktail recipe to a customer.
Tips for effective communication:
- Start by restating the problem to ensure understanding
- Explain your approach before diving into coding
- Discuss trade-offs and alternative solutions
- Ask clarifying questions when needed
2. Problem-Solving Attitude: The Mixologist’s Creativity
Approach each problem with the creativity and adaptability of a skilled mixologist. Be open to feedback and willing to adjust your approach if needed.
Demonstrating problem-solving skills:
- Break down complex problems into smaller, manageable parts
- Consider edge cases and potential issues
- Be prepared to optimize your initial solution
- Show enthusiasm for tackling challenging problems
3. Time Management: Pacing Your Performance
Managing your time during an interview is like pacing yourself during a busy night at the bar. Stay calm and focused, and allocate your time wisely across different aspects of the problem.
Time management strategies:
- Spend a few minutes planning before coding
- Set mental checkpoints to assess your progress
- If stuck, consider moving on to another part of the problem and returning later
- Leave time for testing and explaining your solution
The After-Party: Continuous Learning and Practice
Just as a bartender continually refines their craft, a successful programmer must commit to ongoing learning and practice. Here are some strategies to keep your skills sharp:
1. Regular Coding Exercises: Daily Cocktail Hour
Set aside time each day for coding practice. Platforms like AlgoCademy, LeetCode, and HackerRank offer a wide variety of problems to solve.
2. Mock Interviews: Dry Runs
Participate in mock interviews with peers or use online platforms that simulate interview conditions. This helps you get comfortable with the pressure and time constraints of real interviews.
3. Stay Updated: Following Mixology Trends
Keep up with the latest developments in programming languages, frameworks, and industry trends. This knowledge can give you an edge in interviews and demonstrate your passion for the field.
4. Build Projects: Creating Your Signature Cocktails
Work on personal coding projects to apply your skills in real-world scenarios. This not only reinforces your learning but also provides great talking points during interviews.
Conclusion: Mixing Your Way to Success
As we’ve seen, the art of coding for interviews shares many parallels with the craft of bartending. Both require a solid foundation of knowledge, creative problem-solving skills, attention to detail, and the ability to perform under pressure. By mastering the essential algorithms and data structures, honing your problem-solving techniques, and polishing your soft skills, you’ll be well-equipped to mix up the perfect coding cocktail that will impress even the most discerning interviewer.
Remember, like becoming a master mixologist, becoming a skilled coder takes time, practice, and patience. Embrace the learning process, stay curious, and don’t be afraid to experiment with different approaches. With dedication and the right mindset, you’ll soon be crafting elegant solutions that will make you stand out in the competitive world of tech interviews.
So, raise a glass (of code) to your future success, and happy coding! May your algorithms be efficient, your solutions elegant, and your career in tech long and prosperous. Cheers!