Balancing Theory and Practice in Learning to Code: A Comprehensive Guide
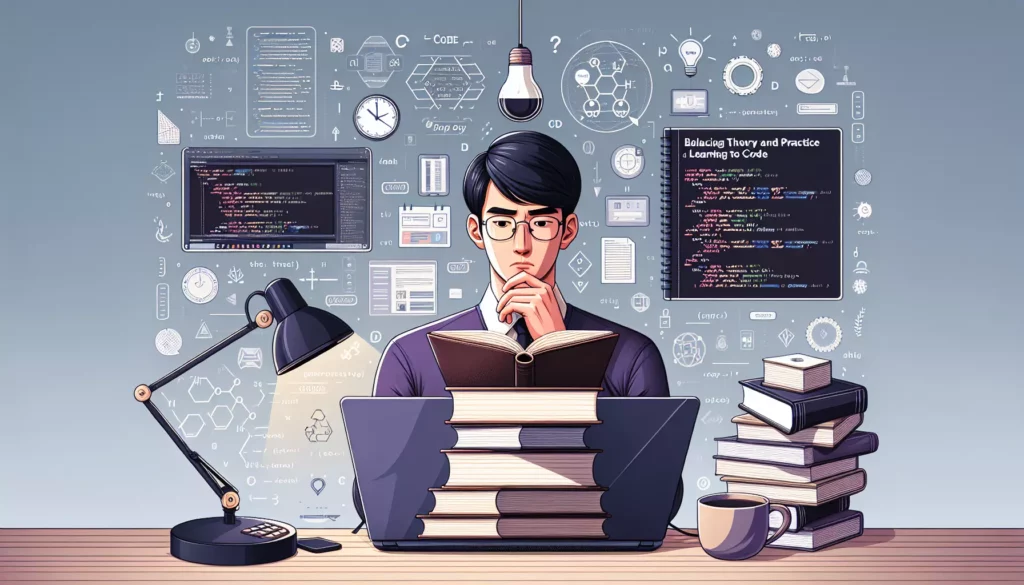
Learning to code is an exciting journey that opens up a world of possibilities in the tech industry. However, one of the most common challenges aspiring programmers face is finding the right balance between theoretical knowledge and practical application. In this comprehensive guide, we’ll explore effective strategies to harmonize theory and practice in your coding education, helping you become a well-rounded and proficient programmer.
The Importance of Balancing Theory and Practice
Before diving into specific strategies, it’s crucial to understand why balancing theory and practice is essential in coding education:
- Comprehensive Understanding: Theory provides the foundation for understanding programming concepts, while practice reinforces and applies that knowledge.
- Problem-Solving Skills: A balanced approach enhances your ability to tackle complex coding challenges from multiple angles.
- Career Readiness: Employers value candidates who possess both theoretical knowledge and hands-on experience.
- Adaptability: Understanding both theory and practice makes it easier to learn new programming languages and technologies.
- Innovation: A solid theoretical background combined with practical skills fosters creativity and innovation in coding solutions.
Strategies for Balancing Theory and Practice
1. Start with Foundational Theory
Begin your coding journey by building a strong theoretical foundation:
- Study basic programming concepts like variables, data types, control structures, and functions.
- Learn about algorithms and data structures to understand efficient problem-solving techniques.
- Explore computer science fundamentals such as binary systems, memory management, and basic networking principles.
Resources for theoretical learning:
- Online courses from platforms like Coursera, edX, or MIT OpenCourseWare
- Textbooks on computer science and programming fundamentals
- Video tutorials and lectures on YouTube or other educational channels
2. Apply Theory Through Coding Exercises
As you learn theoretical concepts, immediately put them into practice:
- Solve coding challenges on platforms like LeetCode, HackerRank, or CodeWars.
- Implement basic algorithms and data structures from scratch.
- Create small projects that utilize the concepts you’ve learned.
Example: After learning about arrays and loops, create a simple program that sorts an array of numbers using different sorting algorithms.
3. Engage in Project-Based Learning
Develop real-world projects to apply your knowledge in practical scenarios:
- Start with small projects and gradually increase complexity as you progress.
- Choose projects that align with your interests to maintain motivation.
- Collaborate with other learners on group projects to simulate real-world development environments.
Project ideas:
- Build a personal website or blog
- Create a simple mobile app
- Develop a basic game (e.g., Tic-Tac-Toe or Snake)
- Implement a command-line tool for file management or data processing
4. Participate in Coding Communities and Forums
Engage with other programmers to gain insights and share knowledge:
- Join online coding communities like Stack Overflow, Reddit’s programming subreddits, or GitHub discussions.
- Attend local meetups or coding events to network with fellow programmers.
- Participate in online hackathons or coding competitions to challenge yourself and learn from others.
5. Utilize Interactive Learning Platforms
Take advantage of platforms that combine theory and practice:
- Use interactive coding tutorials that provide immediate feedback on your code.
- Explore platforms like AlgoCademy that offer a blend of theoretical concepts and practical coding exercises.
- Try gamified learning experiences that make coding education more engaging and hands-on.
6. Read and Analyze Open-Source Code
Studying existing code can bridge the gap between theory and practice:
- Explore open-source projects on GitHub related to your areas of interest.
- Analyze how experienced developers implement theoretical concepts in real-world applications.
- Contribute to open-source projects to gain practical experience and receive feedback from the community.
7. Implement Coding Challenges with Time Constraints
Practice solving problems under time pressure to improve your problem-solving skills:
- Participate in timed coding challenges on platforms like LeetCode or HackerRank.
- Set personal time limits for solving problems to simulate technical interview scenarios.
- Analyze your performance and identify areas for improvement in both theoretical knowledge and practical skills.
8. Teach Others What You’ve Learned
Teaching reinforces your understanding of both theory and practice:
- Start a coding blog or YouTube channel to explain concepts you’ve mastered.
- Mentor beginners in coding communities or local coding bootcamps.
- Create tutorials or documentation for your personal projects.
9. Regularly Review and Reflect on Your Learning
Maintain a balance by periodically assessing your progress:
- Keep a learning journal to track concepts you’ve studied and projects you’ve completed.
- Identify gaps in your knowledge and create a plan to address them.
- Reflect on how theoretical concepts have helped you in practical applications and vice versa.
10. Explore Advanced Topics and Specializations
As you progress, delve deeper into specific areas of interest:
- Study advanced algorithms and data structures for technical interviews.
- Explore specialized fields like machine learning, web development, or mobile app development.
- Learn about software architecture and design patterns to understand large-scale application development.
Practical Examples: Balancing Theory and Practice
Let’s explore some concrete examples of how to balance theory and practice in different areas of programming:
Web Development
Theory: Learn HTML, CSS, and JavaScript fundamentals, as well as web architecture and HTTP protocols.
Practice: Build a responsive website from scratch, implement interactive features using JavaScript, and deploy it to a web hosting service.
Balanced Approach:
- Study HTML and CSS basics, then immediately create a simple static webpage.
- Learn JavaScript fundamentals, followed by adding interactive elements to your webpage.
- Explore web architecture concepts, then set up a basic server using Node.js or another backend technology.
- Study database theory, then integrate a database into your web application.
Data Structures and Algorithms
Theory: Study common data structures (arrays, linked lists, trees, graphs) and algorithmic paradigms (divide and conquer, dynamic programming, greedy algorithms).
Practice: Implement data structures from scratch, solve algorithm problems on coding platforms, and analyze time and space complexity.
Balanced Approach:
- Learn about a specific data structure (e.g., binary search tree), then implement it in your preferred programming language.
- Study an algorithmic technique (e.g., dynamic programming), then solve related problems on LeetCode or HackerRank.
- Analyze the time and space complexity of your implementations and solutions.
- Create a project that utilizes multiple data structures and algorithms, such as a pathfinding visualization tool.
Machine Learning
Theory: Study linear algebra, calculus, probability, and statistics, as well as machine learning concepts and algorithms.
Practice: Implement machine learning algorithms from scratch, use popular libraries like TensorFlow or PyTorch, and work on real-world datasets.
Balanced Approach:
- Learn the mathematical foundations, then implement simple linear regression from scratch.
- Study classification algorithms, then use scikit-learn to solve a basic classification problem.
- Explore neural network theory, followed by building a small neural network using TensorFlow or PyTorch.
- Work on a capstone project that involves data preprocessing, model selection, training, and evaluation on a real-world dataset.
Common Pitfalls to Avoid
As you strive to balance theory and practice in your coding education, be aware of these common pitfalls:
1. Overemphasizing Theory
Pitfall: Spending too much time studying concepts without applying them practically.
Solution: Set a goal to implement or use each new concept you learn within 24-48 hours of studying it. This could be through a small coding exercise, a project feature, or a coding challenge.
2. Neglecting Theoretical Foundations
Pitfall: Jumping into coding without understanding the underlying principles.
Solution: For each new programming concept or technology you encounter, allocate time to understand its theoretical background. Use resources like textbooks, academic papers, or in-depth tutorials to build a solid foundation.
3. Tutorial Hell
Pitfall: Continuously following tutorials without developing independent problem-solving skills.
Solution: After completing a tutorial, challenge yourself to build a similar project with added features or solve related problems without guidance. This helps transition from guided learning to independent application of knowledge.
4. Ignoring Computer Science Fundamentals
Pitfall: Focusing solely on programming languages and frameworks without understanding core CS concepts.
Solution: Dedicate time to study essential computer science topics like data structures, algorithms, operating systems, and databases. Platforms like AlgoCademy can help you learn these fundamentals in an interactive way.
5. Not Seeking Code Review
Pitfall: Working in isolation without receiving feedback on your code.
Solution: Regularly share your code with peers, mentors, or online communities. Participate in code reviews to gain insights into best practices and areas for improvement.
6. Perfectionism Paralysis
Pitfall: Striving for perfect understanding before moving on to new topics or projects.
Solution: Embrace the iterative nature of learning. It’s okay to move forward with partial understanding and revisit concepts later. Practice progressive learning by building on your knowledge over time.
Tools and Resources for Balanced Learning
To help you maintain a balance between theory and practice, consider using these tools and resources:
1. Interactive Learning Platforms
- AlgoCademy: Offers a blend of theoretical concepts and practical coding exercises, with a focus on algorithmic thinking and problem-solving.
- freeCodeCamp: Provides a comprehensive curriculum covering various aspects of web development, with a mix of theory and practical projects.
- Codecademy: Offers interactive coding lessons in various programming languages and technologies.
2. Coding Challenge Platforms
- LeetCode: Provides a vast collection of coding problems, ranging from easy to hard, with a focus on technical interview preparation.
- HackerRank: Offers coding challenges in various domains, including algorithms, data structures, and specific programming languages.
- Project Euler: Presents a series of challenging mathematical/computer programming problems that require both theoretical knowledge and coding skills to solve.
3. Version Control and Collaboration Tools
- GitHub: Use Git for version control and GitHub for hosting your projects, collaborating with others, and exploring open-source code.
- GitLab: An alternative to GitHub that offers similar features for version control and collaboration.
4. Integrated Development Environments (IDEs)
- Visual Studio Code: A versatile, open-source code editor with extensive plugin support for various programming languages and tools.
- PyCharm: An IDE specifically designed for Python development, offering advanced features for coding, debugging, and testing.
- IntelliJ IDEA: A powerful IDE for Java development that also supports other programming languages.
5. Documentation and Learning Resources
- MDN Web Docs: Comprehensive documentation for web technologies, including HTML, CSS, and JavaScript.
- Python Documentation: Official documentation for the Python programming language, including tutorials and references.
- Stack Overflow: A community-driven Q&A platform for programmers to ask questions and share knowledge.
6. Coding Visualization Tools
- Pythontutor: Visualizes code execution step-by-step, helping you understand how programs work under the hood.
- Algorithm Visualizer: Provides interactive visualizations of various algorithms and data structures.
Conclusion: Embracing a Lifelong Learning Mindset
Balancing theory and practice in coding education is not a one-time achievement but an ongoing process throughout your programming career. As you progress in your journey, remember these key takeaways:
- Continuous Integration: Regularly integrate theoretical knowledge with practical application to reinforce your learning.
- Adaptability: Be prepared to adjust your learning approach as you encounter new technologies and concepts.
- Curiosity: Cultivate a sense of curiosity about both the theoretical foundations and practical applications of programming.
- Community Engagement: Stay connected with the programming community to share knowledge, gain insights, and stay updated on industry trends.
- Reflection and Iteration: Periodically reflect on your learning progress and iterate on your approach to maintain an effective balance.
By consistently striving to balance theory and practice, you’ll develop a well-rounded skill set that will serve you well in your programming career. Whether you’re preparing for technical interviews at top tech companies or building innovative applications, this balanced approach will help you tackle complex challenges with confidence and creativity.
Remember that platforms like AlgoCademy are designed to support this balanced learning approach, offering a combination of theoretical concepts, practical coding exercises, and real-world problem-solving scenarios. Leverage these resources to accelerate your growth as a programmer and prepare for success in the ever-evolving world of technology.