Balancing Accuracy and Efficiency in Coding Solutions: A Comprehensive Guide
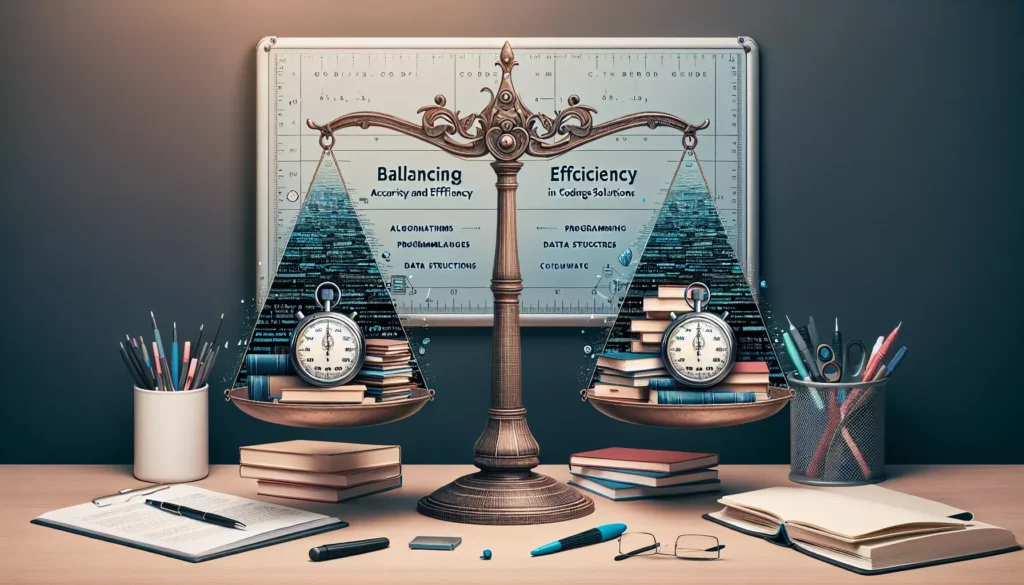
In the world of programming and software development, two critical factors often come into play when creating solutions: accuracy and efficiency. As aspiring developers and coding enthusiasts progress in their journey, they quickly realize that writing code isn’t just about getting the right answer. It’s about finding the optimal balance between producing correct results and doing so in the most efficient manner possible. This balance is particularly crucial when preparing for technical interviews at major tech companies, often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google).
In this comprehensive guide, we’ll explore the importance of balancing accuracy and efficiency in coding solutions, provide strategies to achieve this balance, and offer practical examples to illustrate these concepts. Whether you’re a beginner looking to improve your coding skills or an experienced developer preparing for a technical interview, this article will provide valuable insights to help you create better, more optimized code.
Understanding Accuracy and Efficiency in Coding
Before we dive into the strategies for balancing accuracy and efficiency, let’s first define what these terms mean in the context of coding:
Accuracy
Accuracy in coding refers to the correctness of a solution. An accurate solution produces the correct output for all possible inputs, handles edge cases appropriately, and meets all the requirements specified in the problem statement. Accuracy is non-negotiable; a solution that doesn’t produce correct results is fundamentally flawed, regardless of how efficient it may be.
Efficiency
Efficiency, on the other hand, relates to how well a solution utilizes computational resources. This includes factors such as:
- Time complexity: How the execution time of the algorithm grows with input size
- Space complexity: How much memory the algorithm uses relative to the input size
- CPU usage: How much processing power the algorithm requires
- I/O operations: How many read/write operations the algorithm performs
An efficient solution minimizes resource usage while still producing correct results.
The Importance of Balancing Accuracy and Efficiency
While accuracy is paramount, efficiency can often make the difference between a good solution and a great one. Here’s why balancing these two factors is crucial:
- Real-world applicability: In production environments, especially at large tech companies, code needs to handle massive amounts of data and serve millions of users. Inefficient solutions can lead to slow performance, high costs, and poor user experience.
- Scalability: As datasets grow, inefficient algorithms can become impractical or even unusable. A solution that works well for small inputs might break down completely when faced with large-scale data.
- Resource constraints: In many scenarios, such as embedded systems or mobile applications, resources like memory and processing power are limited. Efficient code is essential in these environments.
- Cost considerations: More efficient code often translates to lower operational costs, especially in cloud computing environments where resources are billed based on usage.
- Technical interviews: Major tech companies often evaluate candidates based on their ability to produce not just correct solutions, but optimal ones. Demonstrating an understanding of efficiency can set you apart in these high-stakes interviews.
Strategies for Balancing Accuracy and Efficiency
Now that we understand the importance of balancing accuracy and efficiency, let’s explore some strategies to achieve this balance:
1. Start with a Correct Solution
Always begin by ensuring your solution is accurate. It’s easier to optimize a correct solution than to fix an efficient but incorrect one. Follow these steps:
- Thoroughly understand the problem requirements
- Consider edge cases and potential input variations
- Implement a solution that correctly handles all cases
- Test your solution with various inputs, including edge cases
2. Analyze Time and Space Complexity
Once you have a correct solution, analyze its time and space complexity. This will help you identify areas for improvement. Use Big O notation to express the complexity in terms of the input size. For example:
def linear_search(arr, target):
for i in range(len(arr)):
if arr[i] == target:
return i
return -1
# Time complexity: O(n)
# Space complexity: O(1)
3. Identify Bottlenecks
Look for parts of your code that contribute most to inefficiency. Common bottlenecks include:
- Nested loops
- Redundant calculations
- Inefficient data structures
- Unnecessary I/O operations
4. Apply Optimization Techniques
Once you’ve identified bottlenecks, apply appropriate optimization techniques. Some common techniques include:
- Memoization and dynamic programming
- Using more efficient data structures (e.g., hash tables for fast lookups)
- Preprocessing data to speed up subsequent operations
- Reducing the number of iterations or recursive calls
5. Use Built-in Functions and Libraries
Many programming languages and libraries offer optimized implementations of common algorithms and data structures. Utilize these when appropriate, as they’re often more efficient than custom implementations. For example, in Python:
import bisect
def binary_search(arr, target):
index = bisect.bisect_left(arr, target)
if index < len(arr) and arr[index] == target:
return index
return -1
# This uses Python's built-in bisect module, which implements an efficient binary search
6. Measure and Profile Your Code
Don’t rely solely on theoretical analysis. Use profiling tools to measure the actual performance of your code. This can help you identify unexpected bottlenecks and verify that your optimizations are effective. Many programming languages have built-in profiling tools, such as Python’s cProfile:
import cProfile
def function_to_profile():
# Your code here
pass
cProfile.run('function_to_profile()')
7. Consider Trade-offs
Sometimes, improving efficiency might come at the cost of code readability or increased complexity. Consider whether the performance gain is worth the trade-off. In some cases, a slightly less efficient but more maintainable solution might be preferable.
Practical Examples: Balancing Accuracy and Efficiency
Let’s look at some practical examples to illustrate how we can balance accuracy and efficiency in real coding scenarios.
Example 1: Finding the Maximum Subarray Sum
Problem: Given an array of integers, find the contiguous subarray with the largest sum.
Naive Solution (Accurate but Inefficient):
def max_subarray_sum_naive(arr):
n = len(arr)
max_sum = float('-inf')
for i in range(n):
for j in range(i, n):
current_sum = sum(arr[i:j+1])
max_sum = max(max_sum, current_sum)
return max_sum
# Time complexity: O(n^3)
# Space complexity: O(1)
This solution is accurate but highly inefficient, with a time complexity of O(n^3).
Optimized Solution (Accurate and Efficient):
def max_subarray_sum_optimized(arr):
max_sum = current_sum = arr[0]
for num in arr[1:]:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
# Time complexity: O(n)
# Space complexity: O(1)
This solution, known as Kadane’s algorithm, maintains accuracy while dramatically improving efficiency to O(n) time complexity.
Example 2: Finding the nth Fibonacci Number
Problem: Calculate the nth Fibonacci number.
Recursive Solution (Accurate but Inefficient):
def fibonacci_recursive(n):
if n <= 1:
return n
return fibonacci_recursive(n-1) + fibonacci_recursive(n-2)
# Time complexity: O(2^n)
# Space complexity: O(n) due to the call stack
This solution is accurate but extremely inefficient for large n, with exponential time complexity.
Dynamic Programming Solution (Accurate and Efficient):
def fibonacci_dp(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
# Time complexity: O(n)
# Space complexity: O(n)
This solution maintains accuracy while improving efficiency to O(n) time complexity.
Further Optimized Solution (Accurate and Most Efficient):
def fibonacci_optimized(n):
if n <= 1:
return n
a, b = 0, 1
for _ in range(2, n + 1):
a, b = b, a + b
return b
# Time complexity: O(n)
# Space complexity: O(1)
This solution achieves the same time complexity as the dynamic programming approach but reduces the space complexity to O(1).
Common Pitfalls in Balancing Accuracy and Efficiency
While striving for the perfect balance between accuracy and efficiency, developers often encounter several common pitfalls. Being aware of these can help you avoid them in your own coding practice:
1. Premature Optimization
One of the most famous quotes in computer science, attributed to Donald Knuth, states: “Premature optimization is the root of all evil.” This means that trying to optimize code before it’s necessary can lead to overly complex solutions, harder-to-maintain code, and wasted time. Always ensure your code is correct first, then optimize only if needed and where it matters most.
2. Overlooking Readability
In the pursuit of efficiency, developers sometimes create code that’s difficult to read and understand. Remember that code is read far more often than it’s written. A slightly less efficient but more readable solution is often preferable, especially in collaborative environments.
3. Ignoring the Context
The importance of efficiency can vary greatly depending on the context of your application. A highly optimized algorithm might be crucial for a real-time system processing millions of transactions, but unnecessary for a small-scale, infrequently used internal tool. Always consider the specific requirements and constraints of your project.
4. Overcomplicating Solutions
Sometimes, in an attempt to create the most efficient solution possible, developers might overcomplicate their code. This can lead to bugs, maintenance issues, and difficulty in understanding the code later. Strive for simplicity unless complexity is absolutely necessary for performance reasons.
5. Neglecting Edge Cases
When optimizing code, it’s easy to focus on the common cases and forget about edge cases. Always ensure that your optimized solution still handles all possible inputs correctly, including edge cases and unexpected scenarios.
6. Relying Too Heavily on Theoretical Analysis
While Big O notation and theoretical analysis are crucial, they don’t tell the whole story. Factors like constant factors, cache behavior, and real-world data distributions can significantly impact actual performance. Always combine theoretical analysis with real-world profiling and testing.
7. Not Considering Space-Time Tradeoffs
Sometimes, improving time efficiency comes at the cost of increased space usage, or vice versa. It’s important to consider both aspects and make informed decisions based on your specific constraints and requirements.
Advanced Techniques for Balancing Accuracy and Efficiency
As you progress in your coding journey, you’ll encounter more complex problems that require advanced techniques to balance accuracy and efficiency. Here are some advanced strategies to consider:
1. Approximation Algorithms
For some problems, finding an exact solution efficiently might be infeasible. In such cases, approximation algorithms can provide a near-optimal solution with guaranteed bounds on the error. These algorithms trade some accuracy for significant improvements in efficiency.
Example: The Traveling Salesman Problem (TSP) is NP-hard, meaning there’s no known polynomial-time algorithm for solving it exactly. However, there are efficient approximation algorithms that can find a tour within a factor of the optimal solution.
2. Randomized Algorithms
Randomized algorithms use random numbers to guide their execution. They can often achieve better average-case performance than deterministic algorithms while maintaining probabilistic guarantees of correctness.
Example: Quicksort with random pivot selection is a randomized algorithm that achieves O(n log n) expected time complexity, avoiding the O(n^2) worst-case of deterministic Quicksort.
3. Amortized Analysis
Amortized analysis considers the average performance of a sequence of operations, rather than focusing on the worst-case scenario for each individual operation. This can provide a more realistic assessment of an algorithm’s efficiency in practice.
Example: The dynamic array (like ArrayList in Java or list in Python) has O(1) amortized time complexity for append operations, even though occasional resizing takes O(n) time.
4. Lazy Evaluation
Lazy evaluation is a technique where computation is deferred until the result is actually needed. This can improve efficiency by avoiding unnecessary calculations.
Example: In Python, generators use lazy evaluation to produce values on-demand, which can be more memory-efficient than creating a full list upfront.
def infinite_sequence():
num = 0
while True:
yield num
num += 1
# This generator can represent an infinite sequence without using infinite memory
5. Parallel and Distributed Algorithms
For large-scale problems, leveraging parallel processing or distributed computing can significantly improve efficiency while maintaining accuracy.
Example: MapReduce is a programming model for processing and generating large datasets with a parallel, distributed algorithm on a cluster.
6. Caching and Memoization
Storing and reusing the results of expensive function calls can dramatically improve efficiency in scenarios with repeated computations.
Example: Memoization in dynamic programming:
def fibonacci_memoized(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci_memoized(n-1, memo) + fibonacci_memoized(n-2, memo)
return memo[n]
# This solution combines recursion with memoization for efficiency
Conclusion
Balancing accuracy and efficiency in coding solutions is a crucial skill for any developer, especially those preparing for technical interviews at major tech companies. By understanding the importance of this balance, applying appropriate strategies, and being aware of common pitfalls, you can create solutions that are not only correct but also optimized for performance.
Remember that achieving this balance is often an iterative process. Start with a correct solution, analyze its efficiency, and then apply optimization techniques as needed. Always consider the specific context and requirements of your problem, and don’t forget to measure and profile your code to ensure your optimizations are effective.
As you continue to practice and refine your skills, you’ll develop an intuition for identifying potential optimizations and selecting the most appropriate techniques for each situation. This balance of accuracy and efficiency will not only help you excel in technical interviews but also make you a more effective and valuable developer in real-world scenarios.
Keep coding, keep optimizing, and always strive for that perfect balance between getting it right and getting it fast!