Attention to Detail: The Key to Acing Your Coding Interview
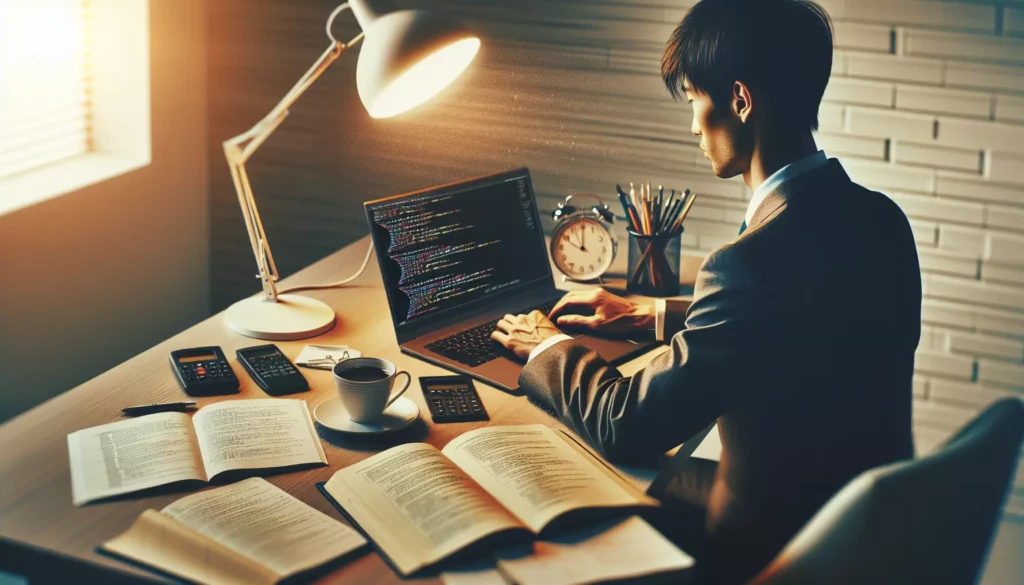
In the competitive world of software development, landing your dream job often hinges on your performance during coding interviews. While having a solid grasp of algorithms and data structures is crucial, there’s another skill that can make or break your interview success: attention to detail. In this comprehensive guide, we’ll explore why paying close attention to the nuances of interview questions is vital and how it can significantly improve your chances of impressing potential employers.
The Importance of Details in Coding Interviews
When faced with a coding challenge during an interview, it’s easy to get caught up in the excitement of problem-solving and overlook critical information provided in the question. However, these details are often intentionally included to guide you towards the optimal solution or to test your ability to consider all aspects of a problem.
As noted by experienced interviewers, candidates frequently miss important clues or constraints mentioned in the problem statement. This oversight can lead to suboptimal solutions or, worse, completely incorrect approaches. Let’s delve into some common scenarios where attention to detail can make a significant difference.
Scenario 1: Sorted Arrays and Time Complexity
Consider this situation: An interviewer presents you with a problem involving a sorted array. They might say something like, “Given a sorted array of integers, find the index of a specific element.” Many candidates, in their haste to solve the problem, might immediately jump to a linear search algorithm, resulting in an O(n) time complexity.
However, the key detail here is that the array is sorted. This information should immediately trigger thoughts of using a binary search algorithm, which would yield a much more efficient O(log n) time complexity. By missing this crucial detail, you’ve not only provided a less optimal solution but also demonstrated a lack of attention to the problem’s specifics.
Example: Binary Search Implementation
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
# Usage
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15]
target = 7
result = binary_search(sorted_array, target)
print(f"Index of {target}: {result}")
This binary search implementation takes advantage of the sorted nature of the array, demonstrating your ability to recognize and utilize important problem details.
Scenario 2: Array Permutations
Another common scenario involves questions about array permutations. For instance, an interviewer might ask, “Given two arrays that are permutations of each other, find the minimum number of swaps required to transform one array into the other.”
In this case, the critical detail is that the arrays are permutations of each other. This information guarantees that both arrays contain the same elements, just in a different order. Many candidates might overlook this fact and provide a solution that works for any two arrays, potentially complicating the problem unnecessarily.
By recognizing that the arrays are permutations, you can devise a more efficient solution that takes advantage of this property. For example, you could use a hash map to track the indices of elements in one array and then compare it with the other array to determine the minimum number of swaps.
Example: Minimum Swaps for Permutations
def min_swaps(arr1, arr2):
if len(arr1) != len(arr2):
return -1 # Not permutations
index_map = {num: i for i, num in enumerate(arr1)}
swaps = 0
for i in range(len(arr2)):
if arr1[i] != arr2[i]:
swap_index = index_map[arr2[i]]
arr1[i], arr1[swap_index] = arr1[swap_index], arr1[i]
index_map[arr1[swap_index]] = swap_index
swaps += 1
return swaps
# Usage
array1 = [2, 3, 4, 1, 5]
array2 = [1, 2, 3, 4, 5]
result = min_swaps(array1, array2)
print(f"Minimum swaps required: {result}")
This solution efficiently calculates the minimum number of swaps by leveraging the permutation property of the arrays.
The Consequences of Overlooking Details
Failing to pay attention to details during a coding interview can have several negative consequences:
- Suboptimal Solutions: As demonstrated in the examples above, missing key information can lead to less efficient algorithms or unnecessarily complex code.
- Wasted Time: If you start solving the problem with an incorrect assumption, you may spend valuable interview time heading down the wrong path.
- Negative Impression: Interviewers often use these details to assess your problem-solving approach and attention to detail. Missing them can create a poor impression, even if you eventually solve the problem.
- Missed Opportunities: Some details might hint at potential follow-up questions or optimizations. Missing these can prevent you from showcasing the full extent of your skills.
Strategies for Improving Attention to Detail
Developing a keen eye for details is a skill that can be cultivated with practice. Here are some strategies to help you improve:
1. Active Reading and Listening
When presented with a problem, whether in written or verbal form, practice active reading or listening. This means engaging with the information critically, rather than passively absorbing it.
- Read the problem statement multiple times.
- Underline or highlight key phrases and constraints.
- If the problem is presented verbally, take notes and repeat the key points back to the interviewer to ensure understanding.
2. Ask Clarifying Questions
Don’t hesitate to ask questions about the problem. This not only helps you gather more information but also demonstrates your thoroughness to the interviewer.
- Confirm any assumptions you’re making about the input or expected output.
- Ask about edge cases or specific scenarios that might affect your approach.
- Inquire about any constraints on time or space complexity if they’re not explicitly stated.
3. Verbalize Your Thought Process
As you begin to solve the problem, explain your thinking out loud. This practice can help you catch details you might have missed and allows the interviewer to provide guidance if needed.
- Describe the key aspects of the problem as you understand them.
- Explain why you’re choosing a particular approach based on the given information.
- Discuss any trade-offs or alternative solutions you’re considering.
4. Practice with Intentionality
When preparing for coding interviews, make a conscious effort to identify and utilize all relevant information in practice problems.
- After solving a problem, review the problem statement again to ensure you didn’t miss any details.
- Challenge yourself to find the most optimal solution based on all given information.
- Review solutions that make clever use of problem constraints and try to understand their approach.
Common Details to Watch For
While every coding problem is unique, there are some common types of details that frequently appear in interview questions. Being aware of these can help you spot them more easily:
1. Input Characteristics
- Is the input sorted, partially sorted, or unsorted?
- Are there any constraints on the input size or values?
- Is the input a special type of data structure (e.g., binary tree, graph)?
2. Time and Space Complexity Requirements
- Are there specific time complexity expectations (e.g., O(n), O(log n))?
- Is there a constraint on space usage (e.g., in-place algorithm required)?
3. Output Format
- What exactly should be returned (e.g., a boolean, an integer, a modified data structure)?
- How should edge cases or error conditions be handled?
4. Problem-Specific Properties
- Are there any mathematical properties or patterns mentioned?
- Is there information about the frequency or distribution of elements?
Real-World Application of Attention to Detail
The skill of paying attention to details extends far beyond coding interviews. In real-world software development, this ability is crucial for:
1. Requirements Gathering
Understanding and implementing all aspects of a project’s requirements is essential for delivering successful software. Missing key details can lead to incomplete features or misaligned functionality.
2. Bug Fixing
Identifying and resolving bugs often requires a meticulous examination of code and system behavior. Attention to detail helps in spotting subtle issues that might be causing problems.
3. Code Review
When reviewing colleagues’ code, a keen eye for detail allows you to catch potential issues, suggest optimizations, and ensure adherence to coding standards and best practices.
4. Performance Optimization
Improving the efficiency of existing systems often involves analyzing fine-grained details of algorithms and data structures to identify opportunities for optimization.
Conclusion: Mastering the Art of Detail-Oriented Problem Solving
In the world of coding interviews and professional software development, the devil is truly in the details. By honing your ability to recognize and utilize all relevant information in a problem statement, you not only increase your chances of acing technical interviews but also become a more effective and valuable developer.
Remember, interviewers are not just testing your ability to write code; they’re assessing your problem-solving approach, your attention to detail, and your ability to think critically about the challenges presented to you. By making a conscious effort to improve in this area, you’ll set yourself apart from other candidates and demonstrate the kind of thorough, thoughtful approach that top tech companies are looking for.
As you continue to prepare for coding interviews, make attention to detail a core part of your practice routine. Analyze problems carefully, question your assumptions, and always be on the lookout for those crucial hints and constraints that can lead you to optimal solutions.
With dedication and practice, you’ll find that this skill not only helps you in interviews but also makes you a more effective problem solver and developer in your day-to-day work. So, the next time you’re faced with a coding challenge, take a deep breath, read carefully, and let your attention to detail guide you to success.
Additional Resources
To further enhance your coding interview skills and attention to detail, consider exploring the following resources:
- AlgoCademy: Offers free coding tutorials and interactive exercises to help you prepare for technical interviews.
- LeetCode and HackerRank: Platforms with a wide variety of coding challenges to practice your problem-solving skills.
- “Cracking the Coding Interview” by Gayle Laakmann McDowell: A comprehensive guide to preparing for coding interviews, including tips on problem-solving approaches.
- Online courses on algorithms and data structures: Platforms like Coursera and edX offer in-depth courses to strengthen your foundational knowledge.
Remember, becoming proficient in coding interviews is a journey that requires consistent practice and a willingness to learn from each experience. By focusing on developing your attention to detail alongside your technical skills, you’ll be well-equipped to tackle any coding challenge that comes your way. Good luck with your interview preparation!