Atlassian Technical Interview Prep: A Comprehensive Guide
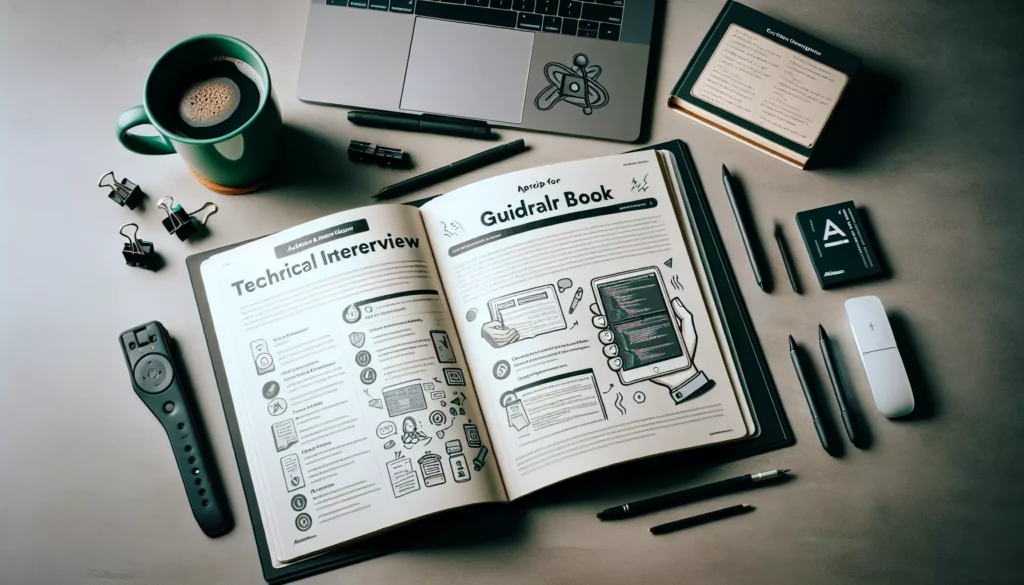
Are you gearing up for a technical interview at Atlassian? Congratulations! You’re on the path to potentially joining one of the most innovative software companies in the world. Atlassian, known for products like Jira, Confluence, and Trello, is always on the lookout for talented developers to join their ranks. In this comprehensive guide, we’ll walk you through everything you need to know to ace your Atlassian technical interview.
Table of Contents
- Understanding Atlassian’s Interview Process
- Core Concepts to Master
- Coding Skills and Languages
- Data Structures and Algorithms
- System Design and Architecture
- Familiarity with Atlassian Products
- Soft Skills and Cultural Fit
- Practice Resources and Mock Interviews
- What to Expect on Interview Day
- Post-Interview Follow-up
1. Understanding Atlassian’s Interview Process
Atlassian’s interview process typically consists of several stages:
- Initial Screening: This usually involves a phone call or video chat with a recruiter to discuss your background and interest in Atlassian.
- Technical Phone Screen: A short technical interview, often involving coding questions or discussions about your past projects.
- Take-home Assignment: Some positions may require you to complete a coding assignment at home.
- On-site Interviews: A series of interviews, including in-depth technical discussions, coding exercises, and system design questions.
- Cultural Fit Interview: A conversation to assess how well you align with Atlassian’s values and culture.
Understanding this process will help you prepare effectively for each stage. Remember, Atlassian values not just technical skills, but also teamwork, innovation, and a customer-first mindset.
2. Core Concepts to Master
To excel in your Atlassian technical interview, make sure you have a solid grasp of these core concepts:
- Object-Oriented Programming (OOP): Understand principles like encapsulation, inheritance, and polymorphism.
- Design Patterns: Familiarize yourself with common patterns like Singleton, Factory, and Observer.
- Version Control: Be proficient with Git and understand branching strategies.
- Testing: Know about unit testing, integration testing, and test-driven development (TDD).
- Agile Methodologies: Understand Scrum and Kanban, as Atlassian is a big proponent of Agile.
- RESTful APIs: Know how to design and consume RESTful services.
- Databases: Understand both relational (SQL) and NoSQL databases.
Let’s dive deeper into one of these concepts with a code example. Here’s a simple implementation of the Singleton pattern in Java:
public class Singleton {
private static Singleton instance;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
public void showMessage() {
System.out.println("Hello, I am a singleton!");
}
}
// Usage
Singleton object = Singleton.getInstance();
object.showMessage();
This pattern ensures that a class has only one instance and provides a global point of access to it. It’s commonly used in logging, driver objects, caching, and thread pool implementations.
3. Coding Skills and Languages
Atlassian uses a variety of programming languages, but some are more prevalent than others. Focus on mastering:
- Java: The primary language for many Atlassian products.
- JavaScript: Essential for front-end development and increasingly for back-end with Node.js.
- Python: Used in various tools and scripts.
- TypeScript: Gaining popularity for its type safety in large-scale JavaScript projects.
Beyond language proficiency, Atlassian values clean, efficient, and well-documented code. Practice writing code that is not just functional, but also maintainable and scalable. Here’s an example of a well-structured and documented Python function:
def calculate_discount(price: float, discount_percentage: float) -> float:
"""
Calculate the discounted price of an item.
Args:
price (float): The original price of the item.
discount_percentage (float): The discount percentage (0-100).
Returns:
float: The price after applying the discount.
Raises:
ValueError: If the discount percentage is not between 0 and 100.
"""
if not 0 <= discount_percentage <= 100:
raise ValueError("Discount percentage must be between 0 and 100")
discount_amount = price * (discount_percentage / 100)
discounted_price = price - discount_amount
return round(discounted_price, 2)
# Usage
try:
original_price = 100
discount = 20
final_price = calculate_discount(original_price, discount)
print(f"The discounted price is ${final_price}")
except ValueError as e:
print(f"Error: {e}")
This function demonstrates clear naming conventions, type hints, comprehensive docstrings, error handling, and rounding for financial calculations.
4. Data Structures and Algorithms
A strong foundation in data structures and algorithms is crucial for any technical interview, including at Atlassian. Make sure you’re comfortable with:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
For algorithms, focus on:
- Sorting and Searching
- Depth-First Search (DFS) and Breadth-First Search (BFS)
- Dynamic Programming
- Greedy Algorithms
- Recursion
Here’s an example of a common algorithm question you might encounter, implementing a binary search in Java:
public class BinarySearch {
public static int binarySearch(int[] arr, int target) {
int left = 0;
int right = arr.length - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1; // Target not found
}
public static void main(String[] args) {
int[] arr = {2, 3, 4, 10, 40};
int target = 10;
int result = binarySearch(arr, target);
if (result == -1) {
System.out.println("Element not present in array");
} else {
System.out.println("Element found at index " + result);
}
}
}
This implementation demonstrates efficient searching in a sorted array with a time complexity of O(log n).
5. System Design and Architecture
For more senior positions, system design questions are a crucial part of the interview process. You should be prepared to discuss:
- Scalability: How to design systems that can handle growth.
- Load Balancing: Techniques for distributing network traffic.
- Caching: Implementing and managing cache to improve performance.
- Database Sharding: Strategies for horizontal partitioning of data.
- Microservices Architecture: Understanding the pros and cons of microservices.
- API Design: Best practices for creating robust and scalable APIs.
A common system design question might be: “Design a URL shortening service like bit.ly.” Here’s a high-level approach:
- Requirements Clarification:
- Functional Requirements: Shorten URL, redirect to original URL
- Non-Functional Requirements: High availability, low latency, scalability
- Capacity Estimation:
- Assume 100 million URLs shortened per month
- 100:1 read to write ratio
- System API:
createShortURL(api_dev_key, original_url, custom_alias=None, user_name=None, expire_date=None) getOriginalURL(api_dev_key, short_url)
- Database Design:
- Use a NoSQL database like Cassandra for its scalability
- Two tables: URL mapping and User data
- Basic System Design:
- Application servers to handle requests
- Load balancers for distributing traffic
- Cache servers to store frequently accessed URLs
- Data Partitioning and Replication:
- Range based partitioning of URLs
- Replicate data across multiple servers
- Cache:
- Use Memcached or Redis to cache hot URLs
- Load Balancer:
- Use Round Robin initially, then switch to more intelligent balancing
- Purging or DB Cleanup:
- Set up a cleanup service to remove expired links
Remember, in system design questions, there’s rarely a single correct answer. The goal is to demonstrate your thought process and ability to consider various aspects of large-scale system design.
6. Familiarity with Atlassian Products
While deep expertise in Atlassian products isn’t always necessary, having a working knowledge of their major offerings can be beneficial. Familiarize yourself with:
- Jira: Project management tool for software teams
- Confluence: Team collaboration and knowledge management platform
- Bitbucket: Git-based code hosting and collaboration tool
- Trello: Visual collaboration tool for workflow management
- Bamboo: Continuous integration and deployment server
Understanding these products can help you relate your skills and experiences to Atlassian’s ecosystem. For instance, if you’ve used Jira in your current role, you might discuss how you’ve customized workflows or created plugins to enhance functionality.
7. Soft Skills and Cultural Fit
Atlassian places a high value on cultural fit and soft skills. They look for candidates who embody their company values:
- Open company, no bullshit
- Build with heart and balance
- Don’t #@!% the customer
- Play, as a team
- Be the change you seek
During your interview, be prepared to discuss:
- Your experience working in team environments
- How you handle conflicts or disagreements
- Your approach to problem-solving and innovation
- Examples of how you’ve gone above and beyond for customers or teammates
- Your passion for continuous learning and self-improvement
Remember, Atlassian is looking for more than just technical skills. They want team players who can contribute to their positive and innovative culture.
8. Practice Resources and Mock Interviews
To sharpen your skills for the Atlassian technical interview, consider using these resources:
- LeetCode: Offers a wide range of coding problems, many of which are similar to those used in technical interviews.
- HackerRank: Provides coding challenges and has a specific interview preparation section.
- Pramp: Allows you to practice mock interviews with peers.
- System Design Primer: A GitHub repository with resources for system design topics.
- Atlassian’s Blog: Read about their engineering practices and latest technologies.
Additionally, consider setting up mock interviews with friends or mentors in the industry. Practice explaining your thought process out loud as you solve problems, as this is a crucial skill during the actual interview.
9. What to Expect on Interview Day
On the day of your Atlassian technical interview, you can expect:
- Multiple Rounds: Typically 4-5 interviews, each lasting about an hour.
- Coding Exercises: You’ll likely be asked to code on a whiteboard or in a shared online editor.
- System Design Discussions: For more senior roles, expect to dive into architecture and scalability topics.
- Behavioral Questions: Be prepared to discuss your past experiences and how you handle various situations.
- Q&A Sessions: You’ll have the opportunity to ask questions about the role and the company.
Tips for interview day:
- Arrive early or ensure your video conferencing setup is ready well in advance.
- Bring a notebook and pen for any in-person interviews.
- Stay calm and take your time when solving problems. It’s okay to ask for clarification.
- Think out loud. Interviewers want to understand your thought process.
- If you’re stuck, don’t be afraid to ask for hints. It’s better to make progress with a little help than to be silent.
10. Post-Interview Follow-up
After your Atlassian technical interview:
- Send a Thank You Email: Within 24 hours, send a brief email thanking your interviewers for their time. Mention specific parts of the conversation that you found interesting or valuable.
- Reflect on the Experience: Take notes on the questions you were asked and how you answered them. This can be valuable for future interviews, regardless of the outcome.
- Be Patient: The hiring process can take time, especially for larger companies like Atlassian. If you haven’t heard back within the timeframe they provided, it’s okay to follow up politely with your recruiter.
- Ask for Feedback: If you don’t get the position, it’s perfectly acceptable to ask for constructive feedback. This can help you improve for future opportunities.
Remember, even if you don’t get an offer this time, maintaining a positive relationship with Atlassian can be beneficial for future opportunities.
Conclusion
Preparing for an Atlassian technical interview requires a combination of strong technical skills, problem-solving abilities, and cultural alignment. By focusing on the areas outlined in this guide, you’ll be well-equipped to showcase your talents and potentially land a role at one of the world’s leading software companies.
Remember, the key to success is not just about having the right answers, but also demonstrating your thought process, your ability to learn and adapt, and your passion for technology and teamwork. With thorough preparation and the right mindset, you’ll be ready to tackle whatever challenges the Atlassian interview process may bring.
Good luck with your interview preparation, and may your journey with Atlassian be the next exciting chapter in your career!