Arrays and Lists: Managing Data Collections
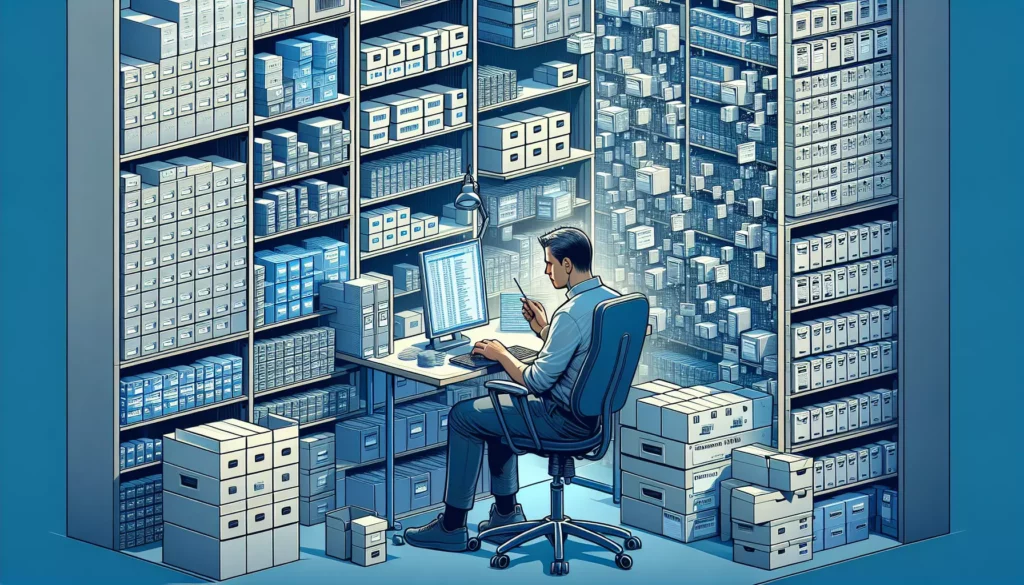
In the world of programming, managing collections of data is a fundamental skill. Whether you’re developing a simple application or preparing for technical interviews at top tech companies, understanding how to work with arrays and lists is crucial. This comprehensive guide will explore these essential data structures, their implementations, and common operations, helping you build a solid foundation for your coding journey.
What Are Arrays and Lists?
Before diving into the specifics, let’s clarify what we mean by arrays and lists:
Arrays
An array is a fixed-size, contiguous block of memory that stores elements of the same data type. Arrays offer constant-time access to elements using their indices but have limitations when it comes to dynamic sizing.
Lists
Lists, on the other hand, are more flexible data structures that can grow or shrink in size. They come in various implementations, such as dynamic arrays (like ArrayList in Java) or linked lists, each with its own strengths and weaknesses.
Arrays: The Foundation of Data Collections
Arrays are one of the most basic and widely used data structures in programming. They offer several advantages:
- Constant-time access to elements (O(1))
- Memory efficiency due to contiguous storage
- Simplicity in implementation and use
Creating and Initializing Arrays
The syntax for creating arrays varies slightly between programming languages. Here are examples in a few popular languages:
Python
# Creating an array (list in Python)
numbers = [1, 2, 3, 4, 5]
# Creating an array of a specific size filled with zeros
zeros = [0] * 5
Java
// Creating an array
int[] numbers = {1, 2, 3, 4, 5};
// Creating an array of a specific size
int[] zeros = new int[5]; // Initializes with default values (0 for int)
JavaScript
// Creating an array
let numbers = [1, 2, 3, 4, 5];
// Creating an array of a specific size filled with a value
let zeros = new Array(5).fill(0);
Accessing and Modifying Array Elements
Accessing elements in an array is straightforward using index notation. Remember that most programming languages use zero-based indexing, meaning the first element is at index 0.
// Python
numbers = [10, 20, 30, 40, 50]
print(numbers[2]) # Output: 30
numbers[1] = 25 # Modifying an element
print(numbers) # Output: [10, 25, 30, 40, 50]
Common Array Operations
Here are some frequently used operations on arrays:
1. Finding the Length
# Python
length = len(numbers)
// Java
int length = numbers.length;
// JavaScript
let length = numbers.length;
2. Iterating Through an Array
# Python
for num in numbers:
print(num)
// Java
for (int num : numbers) {
System.out.println(num);
}
// JavaScript
numbers.forEach(num => console.log(num));
3. Searching for an Element
# Python
index = numbers.index(30) # Returns the index of the first occurrence
// Java
int index = Arrays.asList(numbers).indexOf(30);
// JavaScript
let index = numbers.indexOf(30);
Lists: Dynamic Data Collections
While arrays are powerful, they have limitations, particularly when it comes to resizing. This is where lists come in handy. Lists offer more flexibility and often provide additional built-in methods for manipulation.
Types of Lists
There are two main types of list implementations:
1. Dynamic Arrays (ArrayList)
Dynamic arrays, like ArrayList in Java or list in Python, use an underlying array that automatically resizes when needed. They offer the same O(1) access time as regular arrays but with the ability to grow.
2. Linked Lists
Linked lists consist of nodes, each containing data and a reference to the next node. They excel at insertions and deletions but have O(n) access time for arbitrary elements.
Creating and Using Lists
Let’s look at how to create and use lists in different languages:
Python (Dynamic List)
# Creating a list
fruits = ['apple', 'banana', 'cherry']
# Adding elements
fruits.append('date')
fruits.insert(1, 'blueberry')
# Removing elements
fruits.remove('banana')
popped = fruits.pop() # Removes and returns the last element
print(fruits) # Output: ['apple', 'blueberry', 'cherry']
Java (ArrayList)
import java.util.ArrayList;
ArrayList<String> fruits = new ArrayList<>();
fruits.add("apple");
fruits.add("banana");
fruits.add("cherry");
fruits.add(1, "blueberry"); // Insert at index 1
fruits.remove("banana");
String last = fruits.remove(fruits.size() - 1); // Remove last element
System.out.println(fruits); // Output: [apple, blueberry]
JavaScript (Array as Dynamic List)
let fruits = ['apple', 'banana', 'cherry'];
fruits.push('date'); // Add to end
fruits.unshift('blueberry'); // Add to beginning
fruits.splice(2, 1); // Remove 'banana'
let last = fruits.pop(); // Remove and return last element
console.log(fruits); // Output: ['blueberry', 'apple', 'cherry']
Common List Operations and Their Time Complexities
Understanding the time complexities of common operations is crucial for efficient programming and interview preparation. Here’s a comparison of operations for dynamic arrays (like ArrayList) and linked lists:
Operation | Dynamic Array | Linked List |
---|---|---|
Access by Index | O(1) | O(n) |
Insert/Remove at Beginning | O(n) | O(1) |
Insert/Remove at End | O(1) amortized | O(1) with tail pointer, O(n) without |
Insert/Remove in Middle | O(n) | O(n) to find + O(1) to change links |
Search | O(n) | O(n) |
Advanced Topics and Techniques
As you progress in your coding journey and prepare for technical interviews, you’ll encounter more advanced concepts related to arrays and lists. Let’s explore some of these topics:
1. Two-Dimensional Arrays (Matrices)
Two-dimensional arrays, or matrices, are arrays of arrays. They’re commonly used to represent grids, tables, or mathematical matrices.
# Python
matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
print(matrix[1][2]) # Output: 6
// Java
int[][] matrix = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
System.out.println(matrix[1][2]); // Output: 6
2. Array Slicing
Array slicing allows you to extract a portion of an array. This is particularly useful in Python:
numbers = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
slice1 = numbers[2:5] # [2, 3, 4]
slice2 = numbers[::-1] # Reverse the array: [9, 8, 7, 6, 5, 4, 3, 2, 1, 0]
3. List Comprehensions (Python)
List comprehensions provide a concise way to create lists in Python:
squares = [x**2 for x in range(10)]
evens = [x for x in range(20) if x % 2 == 0]
4. Sorting Arrays and Lists
Most programming languages provide built-in sorting methods:
# Python
numbers.sort() # In-place sorting
sorted_numbers = sorted(numbers) # Returns a new sorted list
// Java
Arrays.sort(numbers); // In-place sorting
List<Integer> list = Arrays.asList(numbers);
Collections.sort(list); // Sorting a list
// JavaScript
numbers.sort((a, b) => a - b); // In-place sorting with custom comparator
5. Binary Search
Binary search is an efficient algorithm for finding an element in a sorted array:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Element not found
6. Dynamic Programming with Arrays
Many dynamic programming problems involve clever use of arrays. For example, the fibonacci sequence can be efficiently computed using an array:
def fibonacci(n):
if n <= 1:
return n
fib = [0] * (n + 1)
fib[1] = 1
for i in range(2, n + 1):
fib[i] = fib[i-1] + fib[i-2]
return fib[n]
Common Interview Questions and Techniques
When preparing for technical interviews, especially for top tech companies, you’ll often encounter problems involving arrays and lists. Here are some common types of questions and techniques to solve them:
1. Two Pointer Technique
This technique is often used to solve array problems efficiently. For example, to reverse an array in-place:
def reverse_array(arr):
left, right = 0, len(arr) - 1
while left < right:
arr[left], arr[right] = arr[right], arr[left]
left += 1
right -= 1
2. Sliding Window
The sliding window technique is useful for problems involving subarrays or subsequences. For example, finding the maximum sum subarray of size k:
def max_sum_subarray(arr, k):
n = len(arr)
if n < k:
return None
window_sum = sum(arr[:k])
max_sum = window_sum
for i in range(k, n):
window_sum = window_sum - arr[i-k] + arr[i]
max_sum = max(max_sum, window_sum)
return max_sum
3. Kadane’s Algorithm
This algorithm is used to find the maximum subarray sum in an array:
def kadanes_algorithm(arr):
max_current = max_global = arr[0]
for i in range(1, len(arr)):
max_current = max(arr[i], max_current + arr[i])
if max_current > max_global:
max_global = max_current
return max_global
4. Dutch National Flag Problem
This problem involves sorting an array of 0s, 1s, and 2s in linear time:
def sort_colors(arr):
low, mid, high = 0, 0, len(arr) - 1
while mid <= high:
if arr[mid] == 0:
arr[low], arr[mid] = arr[mid], arr[low]
low += 1
mid += 1
elif arr[mid] == 1:
mid += 1
else: # arr[mid] == 2
arr[mid], arr[high] = arr[high], arr[mid]
high -= 1
Best Practices and Tips
As you work with arrays and lists, keep these best practices and tips in mind:
- Choose the right data structure: Consider the operations you’ll be performing most frequently and choose between arrays and different types of lists accordingly.
- Be mindful of bounds: Always check array bounds to avoid index out of range errors.
- Use built-in methods: Familiarize yourself with the built-in methods for array and list manipulation in your chosen programming language.
- Consider space complexity: While working with large datasets, be aware of the space complexity of your solutions.
- Practice, practice, practice: Solving diverse problems involving arrays and lists will help you become more proficient and prepare you for technical interviews.
Conclusion
Arrays and lists are fundamental data structures in programming. Mastering their use and understanding the associated algorithms and techniques is crucial for any programmer, especially those preparing for technical interviews at top tech companies. By thoroughly understanding these concepts and practicing regularly, you’ll build a strong foundation for more advanced programming concepts and be well-prepared to tackle complex coding challenges.
Remember, the key to mastery is consistent practice and application. Try implementing the concepts and algorithms discussed in this guide in your preferred programming language. As you progress, challenge yourself with more complex problems and explore how arrays and lists interact with other data structures and algorithms. Happy coding!