Are We Overemphasizing Math in Programming? A Balanced Perspective
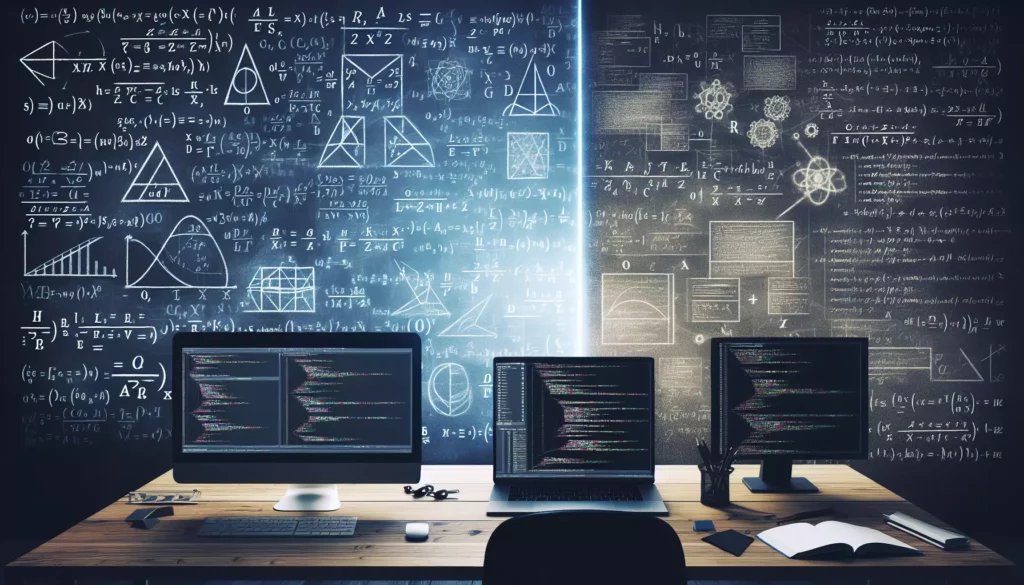
In the world of programming and software development, there’s an ongoing debate about the role of mathematics. Some argue that strong mathematical skills are crucial for success in coding, while others contend that the emphasis on math might be overblown. As we delve into this topic, it’s important to consider both sides of the argument and understand where math truly fits in the programming landscape.
The Traditional View: Math as the Foundation of Programming
Historically, computer science and programming have been closely tied to mathematics. This connection stems from the origins of computing, which were rooted in mathematical concepts and logic. Early computer scientists were often mathematicians, and many fundamental programming concepts have mathematical underpinnings.
Proponents of emphasizing math in programming often cite several reasons:
- Algorithmic Thinking: Mathematics provides a framework for logical thinking and problem-solving, which are essential skills in programming.
- Efficiency: Understanding mathematical concepts can lead to more efficient code and better optimization techniques.
- Advanced Fields: Certain areas of programming, such as machine learning, computer graphics, and cryptography, rely heavily on advanced mathematical concepts.
- Theoretical Computer Science: Many fundamental concepts in computer science, like complexity analysis and formal languages, have strong mathematical foundations.
The Counterargument: Practical Programming vs. Theoretical Mathematics
On the other hand, there’s a growing sentiment that the emphasis on mathematics in programming education might be excessive, especially for many real-world applications. Critics of the math-heavy approach argue:
- Practical Skills Gap: Many day-to-day programming tasks don’t require advanced math, and focusing too much on math might detract from learning practical coding skills.
- Barrier to Entry: Overemphasizing math can discourage potential programmers who may excel in other aspects of coding but struggle with mathematical concepts.
- Changing Landscape: With the rise of high-level programming languages and frameworks, many mathematical operations are abstracted away from the average programmer.
- Diverse Applications: Programming has expanded into various fields where mathematical prowess may be less critical, such as web development or user interface design.
Finding the Balance: The Role of Math in Modern Programming
The reality of math’s importance in programming lies somewhere between these two viewpoints. While it’s true that not all programming tasks require advanced mathematical knowledge, a basic understanding of mathematical concepts can be beneficial for several reasons:
1. Problem-Solving and Logical Thinking
Mathematics teaches structured thinking and problem-solving approaches that are valuable in programming. Concepts like breaking down complex problems into smaller, manageable parts are common in both math and coding.
2. Algorithm Design and Analysis
Understanding basic mathematical concepts can help in designing and analyzing algorithms. For instance, knowledge of logarithms is useful in understanding the time complexity of binary search algorithms.
3. Data Structures
Many data structures have mathematical foundations. Understanding these can lead to more efficient use and implementation of data structures in programming.
4. Specific Programming Domains
Certain areas of programming do require more advanced mathematical knowledge. These include:
- Machine Learning and AI
- Computer Graphics and Game Development
- Scientific Computing
- Cryptography
- Financial Software Development
The Practical Approach: What Math Do Programmers Really Need?
For most programmers, a solid foundation in basic mathematics is sufficient. This typically includes:
- Algebra: Understanding variables, equations, and functions.
- Logic: Boolean algebra and logical operations are fundamental to programming.
- Basic Statistics: Useful for data analysis and understanding probabilistic algorithms.
- Discrete Mathematics: Concepts like sets, relations, and graphs are often used in programming.
- Basic Calculus: While not always necessary, it can be helpful in understanding certain algorithms and optimization techniques.
The Importance of Context in Programming Education
When discussing the role of math in programming education, it’s crucial to consider the context and goals of the learner. Different career paths and specializations in programming may require varying levels of mathematical proficiency.
Tailoring Education to Career Goals
Educational platforms and coding bootcamps should consider offering tailored paths that align with different career trajectories in programming:
- Web Development: Focus more on practical coding skills, with less emphasis on advanced mathematics.
- Data Science: Include a stronger foundation in statistics and linear algebra.
- Game Development: Emphasize 3D mathematics and physics.
- Systems Programming: Include discrete mathematics and algorithm analysis.
Integrating Math Concepts with Practical Programming
Rather than teaching math in isolation, a more effective approach might be to integrate mathematical concepts within programming courses. This method helps students understand the practical applications of math in coding contexts.
For example:
// Example: Using modular arithmetic in programming
function isEven(number) {
return number % 2 === 0;
}
// This function uses the modulo operator, which is based on the mathematical concept of modular arithmetic
The Role of Algorithmic Thinking
While we may not need to overemphasize advanced mathematics, the importance of algorithmic thinking cannot be overstated. Algorithmic thinking involves breaking down problems, identifying patterns, and developing step-by-step solutions – skills that are crucial in programming and have roots in mathematical reasoning.
Developing Algorithmic Skills Without Heavy Math
Platforms like AlgoCademy focus on developing algorithmic thinking and problem-solving skills, which are essential for programming success. These skills can be cultivated through:
- Puzzle-solving exercises
- Code challenges that focus on logic rather than complex math
- Analyzing and optimizing existing algorithms
- Collaborative problem-solving sessions
The Evolution of Programming Tools and Languages
As programming languages and tools evolve, they often abstract away complex mathematical operations, making certain tasks more accessible to programmers without strong math backgrounds. However, understanding the underlying principles can still be beneficial.
High-Level Abstractions
Modern programming languages and libraries often provide high-level abstractions for complex mathematical operations. For instance:
// Python example using numpy for matrix operations
import numpy as np
matrix1 = np.array([[1, 2], [3, 4]])
matrix2 = np.array([[5, 6], [7, 8]])
result = np.dot(matrix1, matrix2)
print(result)
# This code performs matrix multiplication without requiring the programmer to implement the mathematical operation manually
While these abstractions make complex operations more accessible, understanding the underlying concepts can help in choosing the right tools and optimizing performance when necessary.
The Impact of AI and Machine Learning
The rise of artificial intelligence and machine learning has reignited discussions about the importance of mathematics in programming. These fields often require a deeper understanding of linear algebra, calculus, and statistics.
Balancing AI Knowledge with Practical Skills
For programmers interested in AI and ML, a stronger mathematical foundation is beneficial. However, it’s important to note that many ML frameworks abstract away complex math, allowing developers to implement AI solutions without deep mathematical expertise.
// Example using TensorFlow for a simple neural network
import tensorflow as tf
model = tf.keras.Sequential([
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
# This code creates a neural network without requiring explicit mathematical computations
While this abstraction is powerful, understanding the underlying math can help in tuning models and troubleshooting issues.
The Future of Math in Programming Education
As we look to the future of programming education, it’s clear that a balanced approach is needed. Here are some considerations for educational platforms and institutions:
- Contextual Learning: Teach mathematical concepts in the context of their programming applications.
- Tiered Approach: Offer different levels of mathematical depth based on career paths and personal interests.
- Focus on Fundamentals: Emphasize core mathematical concepts that have broad applications in programming.
- Practical Application: Provide plenty of opportunities to apply mathematical concepts in real-world programming scenarios.
- Interdisciplinary Approach: Encourage connections between mathematics, programming, and other fields to showcase the versatility of these skills.
Conclusion: Striking the Right Balance
In conclusion, while mathematics undoubtedly plays a crucial role in computer science and certain programming specialties, it’s important not to overemphasize it at the expense of other vital skills. The key is to strike a balance that provides a solid foundation in relevant mathematical concepts while focusing on practical programming skills and problem-solving abilities.
For platforms like AlgoCademy, this means:
- Offering a diverse curriculum that caters to different career paths in programming
- Integrating mathematical concepts seamlessly into programming tutorials and challenges
- Focusing on developing strong algorithmic thinking and problem-solving skills
- Providing resources for those who wish to delve deeper into the mathematical aspects of programming
- Emphasizing the practical applications of math in real-world programming scenarios
By adopting this balanced approach, we can ensure that aspiring programmers are well-equipped to tackle the challenges of modern software development, regardless of their mathematical background. The goal should be to make programming accessible to a wide range of individuals while still providing the tools and knowledge necessary for those who wish to explore the more mathematically intensive areas of computer science.
Ultimately, the question isn’t whether we’re overemphasizing math in programming, but rather how we can best integrate mathematical thinking into programming education in a way that enhances problem-solving skills without creating unnecessary barriers. By focusing on relevance, application, and adaptability, we can prepare the next generation of programmers for the diverse and evolving landscape of software development.