Approaching Recursive Backtracking Problems: A Comprehensive Guide
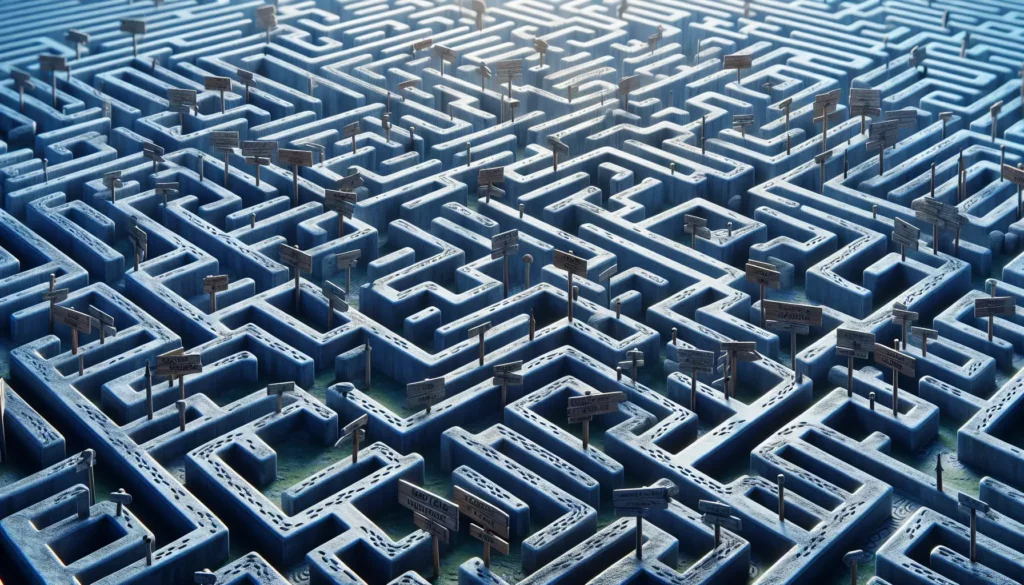
Welcome to our in-depth guide on approaching recursive backtracking problems! If you’re preparing for technical interviews at major tech companies or simply want to enhance your problem-solving skills, understanding recursive backtracking is crucial. This powerful technique is often used to solve complex algorithmic problems, especially those involving combinatorial exploration or optimization.
In this comprehensive blog post, we’ll dive deep into the world of recursive backtracking, exploring its concepts, implementation strategies, and practical applications. By the end of this article, you’ll have a solid foundation for tackling recursive backtracking problems with confidence.
Table of Contents
- Understanding Recursive Backtracking
- Key Components of Recursive Backtracking
- A Problem-Solving Framework
- Common Recursive Backtracking Problems
- Optimization Techniques
- Real-World Applications
- Tips and Tricks for Mastering Recursive Backtracking
- Conclusion
1. Understanding Recursive Backtracking
Recursive backtracking is a problem-solving technique that combines the power of recursion with the ability to undo or “backtrack” from unsuccessful attempts. It’s particularly useful when we need to explore all possible solutions or find an optimal solution in a large search space.
At its core, recursive backtracking involves the following steps:
- Choose a starting point
- Explore a potential solution
- If the solution is valid, continue exploring
- If the solution is invalid or we’ve reached the end, backtrack
- Repeat steps 2-4 until all possibilities are exhausted
The power of this approach lies in its ability to systematically explore all possibilities while efficiently pruning branches that lead to invalid solutions.
2. Key Components of Recursive Backtracking
To effectively implement recursive backtracking, it’s essential to understand its key components:
2.1 Base Case
The base case is the condition that determines when to stop the recursion. It’s crucial to define a clear and correct base case to prevent infinite recursion and ensure the algorithm terminates.
2.2 Recursive Case
The recursive case defines how the problem is broken down into smaller subproblems. It’s where we make choices, explore potential solutions, and call the function recursively.
2.3 Backtracking Mechanism
Backtracking allows us to undo choices and explore alternative paths when we reach an invalid solution or a dead end. This is typically implemented by restoring the state of the problem to what it was before making a choice.
2.4 State Management
Keeping track of the current state of the problem is crucial in recursive backtracking. This may involve maintaining a data structure (e.g., an array or a set) to represent the current partial solution.
2.5 Constraints and Validity Checks
Defining clear constraints and implementing validity checks helps prune the search space by avoiding exploration of invalid paths early on.
3. A Problem-Solving Framework
When approaching recursive backtracking problems, it’s helpful to follow a structured framework. Here’s a step-by-step approach you can use:
3.1 Identify the Problem Type
Determine if the problem is a good fit for recursive backtracking. Look for characteristics such as:
- Combinatorial problems
- Problems requiring exhaustive search
- Optimization problems with constraints
- Problems involving permutations or combinations
3.2 Define the State Space
Clearly define what constitutes a state in your problem. This could be a partial solution, a set of choices made so far, or any other relevant information.
3.3 Identify Base Cases
Determine the conditions under which the recursion should stop. This could be when a valid solution is found, when all possibilities are exhausted, or when a certain depth is reached.
3.4 Define the Recursive Case
Specify how to break down the problem into smaller subproblems. This involves making choices and recursively exploring them.
3.5 Implement Backtracking Logic
Develop a mechanism to undo choices and explore alternative paths when necessary.
3.6 Add Constraints and Pruning
Implement checks to validate partial solutions and prune invalid paths early in the search process.
3.7 Optimize and Refine
Look for opportunities to optimize your solution, such as memoization, early termination, or intelligent ordering of choices.
4. Common Recursive Backtracking Problems
Let’s explore some classic problems that are often solved using recursive backtracking. For each problem, we’ll provide a brief description and a sample implementation in Python.
4.1 N-Queens Problem
The N-Queens problem involves placing N chess queens on an N×N chessboard so that no two queens threaten each other.
def solve_n_queens(n):
def is_safe(board, row, col):
# Check if a queen can be placed on board[row][col]
# Check this row on left side
for i in range(col):
if board[row][i] == 1:
return False
# Check upper diagonal on left side
for i, j in zip(range(row, -1, -1), range(col, -1, -1)):
if board[i][j] == 1:
return False
# Check lower diagonal on left side
for i, j in zip(range(row, n, 1), range(col, -1, -1)):
if board[i][j] == 1:
return False
return True
def solve(board, col):
# Base case: If all queens are placed, return True
if col >= n:
return True
# Consider this column and try placing this queen in all rows one by one
for i in range(n):
if is_safe(board, i, col):
# Place this queen in board[i][col]
board[i][col] = 1
# Recur to place rest of the queens
if solve(board, col + 1):
return True
# If placing queen in board[i][col] doesn't lead to a solution,
# then remove queen from board[i][col]
board[i][col] = 0
# If the queen can't be placed in any row in this column col, return False
return False
# Initialize the board
board = [[0 for _ in range(n)] for _ in range(n)]
# Start from the first column
if solve(board, 0) == False:
print("Solution does not exist")
return False
# Print the solution
for i in range(n):
for j in range(n):
print(board[i][j], end=" ")
print()
return True
# Test the function
solve_n_queens(4)
4.2 Sudoku Solver
The Sudoku solver aims to fill a 9×9 grid with digits so that each column, row, and 3×3 sub-grid contains all digits from 1 to 9 without repetition.
def solve_sudoku(board):
def find_empty(board):
for i in range(len(board)):
for j in range(len(board[0])):
if board[i][j] == 0:
return (i, j) # row, col
return None
def valid(board, num, pos):
# Check row
for j in range(len(board[0])):
if board[pos[0]][j] == num and pos[1] != j:
return False
# Check column
for i in range(len(board)):
if board[i][pos[1]] == num and pos[0] != i:
return False
# Check 3x3 box
box_x = pos[1] // 3
box_y = pos[0] // 3
for i in range(box_y*3, box_y*3 + 3):
for j in range(box_x*3, box_x*3 + 3):
if board[i][j] == num and (i,j) != pos:
return False
return True
def solve(board):
find = find_empty(board)
if not find:
return True
else:
row, col = find
for i in range(1,10):
if valid(board, i, (row, col)):
board[row][col] = i
if solve(board):
return True
board[row][col] = 0
return False
solve(board)
return board
# Example Sudoku board (0 represents empty cells)
board = [
[5,3,0,0,7,0,0,0,0],
[6,0,0,1,9,5,0,0,0],
[0,9,8,0,0,0,0,6,0],
[8,0,0,0,6,0,0,0,3],
[4,0,0,8,0,3,0,0,1],
[7,0,0,0,2,0,0,0,6],
[0,6,0,0,0,0,2,8,0],
[0,0,0,4,1,9,0,0,5],
[0,0,0,0,8,0,0,7,9]
]
solved_board = solve_sudoku(board)
for row in solved_board:
print(row)
4.3 Permutations
Generate all possible permutations of a given set of elements.
def permutations(arr):
def backtrack(start):
if start == len(arr):
result.append(arr[:])
return
for i in range(start, len(arr)):
arr[start], arr[i] = arr[i], arr[start]
backtrack(start + 1)
arr[start], arr[i] = arr[i], arr[start] # backtrack
result = []
backtrack(0)
return result
# Test the function
print(permutations([1, 2, 3]))
4.4 Subset Sum Problem
Determine if there exists a subset of given numbers that add up to a specific target sum.
def subset_sum(numbers, target):
def backtrack(index, current_sum):
if current_sum == target:
return True
if index == len(numbers) or current_sum > target:
return False
# Include the current number
if backtrack(index + 1, current_sum + numbers[index]):
return True
# Exclude the current number
return backtrack(index + 1, current_sum)
return backtrack(0, 0)
# Test the function
print(subset_sum([3, 34, 4, 12, 5, 2], 9)) # True
print(subset_sum([3, 34, 4, 12, 5, 2], 30)) # False
5. Optimization Techniques
While recursive backtracking is powerful, it can be computationally expensive for large problem spaces. Here are some techniques to optimize your recursive backtracking solutions:
5.1 Memoization
Memoization involves caching the results of expensive function calls to avoid redundant computations. This can significantly improve performance in problems with overlapping subproblems.
from functools import lru_cache
@lru_cache(maxsize=None)
def fibonacci(n):
if n < 2:
return n
return fibonacci(n-1) + fibonacci(n-2)
print(fibonacci(100)) # Fast computation of large Fibonacci numbers
5.2 Pruning
Pruning involves eliminating branches of the search tree that are guaranteed not to lead to a valid solution. This can dramatically reduce the search space.
def n_queens_optimized(n):
def is_safe(board, row, col):
# Check if a queen can be placed on board[row][col]
# We only need to check the left side now
for i in range(col):
if board[row][i] == 1:
return False
if row - i >= 0 and board[row - i][col - i] == 1:
return False
if row + i < n and board[row + i][col - i] == 1:
return False
return True
def solve(board, col):
if col >= n:
return True
for i in range(n):
if is_safe(board, i, col):
board[i][col] = 1
if solve(board, col + 1):
return True
board[i][col] = 0
return False
board = [[0 for _ in range(n)] for _ in range(n)]
if solve(board, 0) == False:
print("Solution does not exist")
return False
for i in range(n):
for j in range(n):
print(board[i][j], end=" ")
print()
return True
n_queens_optimized(8) # Solves 8-Queens problem efficiently
5.3 Intelligent Ordering
Choosing the order in which to explore options can significantly impact performance. Start with choices that are more likely to lead to a solution or that allow for early pruning.
5.4 Iterative Deepening
For problems where the solution depth is unknown, iterative deepening combines the space-efficiency of depth-first search with the completeness of breadth-first search.
def iterative_deepening_dfs(graph, start, goal):
def dfs(node, goal, depth, visited):
if depth == 0:
return node == goal
if node == goal:
return True
if node not in graph:
return False
visited.add(node)
for neighbor in graph[node]:
if neighbor not in visited:
if dfs(neighbor, goal, depth - 1, visited):
return True
visited.remove(node)
return False
max_depth = 0
while True:
visited = set()
if dfs(start, goal, max_depth, visited):
return max_depth
max_depth += 1
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['D', 'E'],
'C': ['F'],
'D': [],
'E': ['F'],
'F': []
}
print(iterative_deepening_dfs(graph, 'A', 'F')) # Output: 2
6. Real-World Applications
Recursive backtracking is not just an academic exercise; it has numerous practical applications in various domains:
6.1 Puzzle Solvers
Many popular puzzles, such as Sudoku, crosswords, and logic puzzles, can be efficiently solved using recursive backtracking algorithms.
6.2 Path Finding
In robotics and game development, recursive backtracking can be used to find paths through mazes or complex terrains.
6.3 Constraint Satisfaction Problems
Many real-world problems, such as scheduling and resource allocation, can be modeled as constraint satisfaction problems and solved using recursive backtracking.
6.4 Combinatorial Optimization
In fields like operations research, recursive backtracking can be used to solve complex optimization problems, such as the traveling salesman problem or job shop scheduling.
6.5 Compiler Design
Recursive backtracking is used in parsing algorithms for programming languages, helping to analyze and interpret code structure.
7. Tips and Tricks for Mastering Recursive Backtracking
To become proficient in solving recursive backtracking problems, consider the following tips:
7.1 Practice Regularly
Solve a variety of recursive backtracking problems to build your intuition and problem-solving skills.
7.2 Visualize the Process
Use diagrams or flowcharts to visualize the recursion tree and backtracking process. This can help you understand the algorithm’s behavior and identify optimization opportunities.
7.3 Start Simple
Begin with a basic implementation and gradually add optimizations. This approach helps ensure correctness before focusing on efficiency.
7.4 Understand the Problem Constraints
Carefully analyze the problem constraints and use them to guide your implementation and optimization efforts.
7.5 Learn from Existing Solutions
Study well-known recursive backtracking algorithms and adapt their strategies to new problems.
7.6 Use Debugging Techniques
Implement logging or visualization tools to help debug complex recursive backtracking algorithms.
8. Conclusion
Recursive backtracking is a powerful problem-solving technique that can tackle a wide range of complex computational problems. By understanding its core principles, mastering common problem patterns, and applying optimization techniques, you’ll be well-equipped to approach challenging algorithmic problems in technical interviews and real-world applications.
Remember that proficiency in recursive backtracking comes with practice. As you work through more problems, you’ll develop a stronger intuition for when and how to apply this technique effectively. Keep exploring, experimenting, and refining your skills, and you’ll soon find yourself confidently solving even the most intricate recursive backtracking challenges.
Happy coding, and may your recursive functions always find their base cases!