Approaching Computational Geometry Problems: A Comprehensive Guide
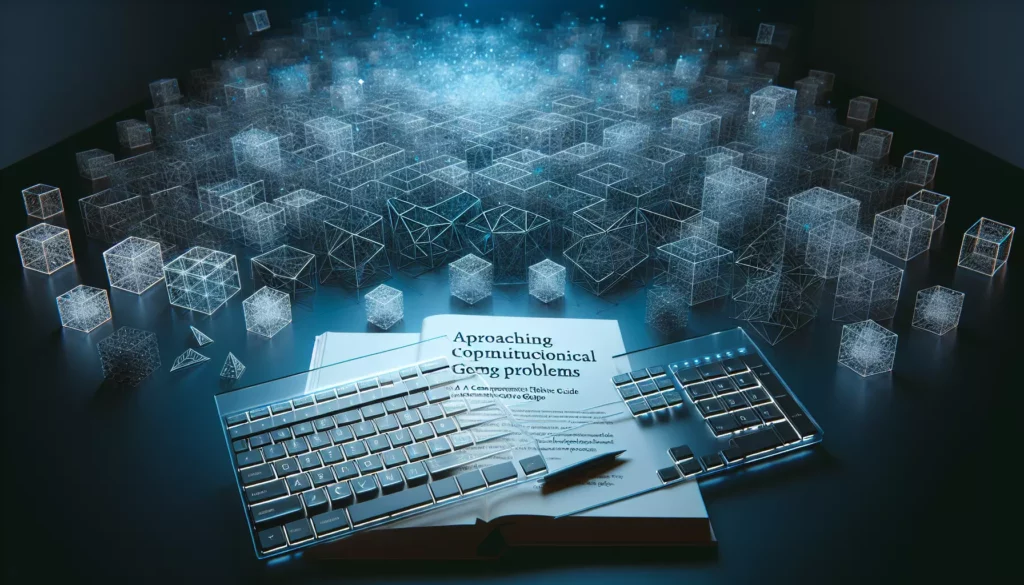
Computational geometry is a fascinating branch of computer science that deals with solving geometric problems algorithmically. It’s a field that combines the precision of mathematics with the efficiency of algorithms, making it crucial for various applications, from computer graphics to robotics. In this comprehensive guide, we’ll explore how to approach computational geometry problems, providing you with the tools and strategies to tackle these challenges effectively.
Understanding Computational Geometry
Before diving into problem-solving strategies, it’s essential to understand what computational geometry entails. Computational geometry focuses on developing efficient algorithms to solve geometric problems. These problems often involve points, lines, polygons, and other geometric shapes in two or three-dimensional space.
Some common areas of study in computational geometry include:
- Convex hulls
- Line segment intersection
- Polygon triangulation
- Voronoi diagrams
- Delaunay triangulations
- Nearest neighbor searches
- Point location
Understanding these concepts is crucial for approaching computational geometry problems effectively.
Key Strategies for Approaching Computational Geometry Problems
1. Visualize the Problem
One of the most important steps in solving computational geometry problems is visualization. Unlike abstract algorithmic problems, geometric problems have a spatial component that can often be better understood through visual representation.
Tips for effective visualization:
- Sketch the problem on paper or use digital drawing tools
- Use different colors to distinguish between various elements
- Consider multiple examples, including edge cases
- Try to identify patterns or symmetries in the geometric arrangement
2. Understand the Geometric Properties
Many computational geometry problems can be solved more efficiently by leveraging fundamental geometric properties. Refresh your knowledge of basic geometric concepts such as:
- Properties of lines, angles, and triangles
- Euclidean distance
- Vector operations
- Coordinate systems (Cartesian, polar)
- Transformations (translation, rotation, scaling)
Understanding these properties can often lead to more elegant and efficient solutions.
3. Break Down Complex Shapes
When dealing with complex geometric shapes, it’s often helpful to break them down into simpler components. For example, a polygon can be decomposed into triangles, or a complex curve might be approximated by a series of line segments.
This approach can simplify the problem and allow you to apply well-known algorithms to these simpler components.
4. Consider Sweeping Techniques
Sweeping is a powerful technique in computational geometry where an imaginary line (or plane in 3D) is moved across the geometric space, processing elements as they are encountered. This technique is particularly useful for problems involving intersections, unions, or other operations on multiple geometric objects.
Examples of problems where sweeping can be effective:
- Finding all intersections among a set of line segments
- Computing the union or intersection of polygons
- Determining if any two line segments in a set intersect
5. Utilize Spatial Data Structures
Efficient data structures are crucial in computational geometry for organizing and querying spatial data. Familiarize yourself with structures such as:
- Quad-trees and Oct-trees
- k-d trees
- R-trees
- Bounding Volume Hierarchies (BVH)
These structures can significantly improve the efficiency of operations like nearest neighbor searches or range queries.
6. Apply Divide-and-Conquer Strategies
Many geometric problems can be solved efficiently using divide-and-conquer algorithms. This approach involves breaking down a problem into smaller subproblems, solving them recursively, and then combining the results.
Examples of geometric problems suited for divide-and-conquer:
- Computing the convex hull of a set of points
- Finding the closest pair of points
- Constructing Voronoi diagrams
7. Consider Incremental Construction
Incremental construction is another powerful technique in computational geometry. It involves building a solution step by step, adding one element at a time and updating the solution accordingly.
This approach can be particularly effective for problems like:
- Constructing Delaunay triangulations
- Building convex hulls
- Creating arrangements of lines or planes
8. Leverage Duality
Duality is a concept in geometry where points and lines (or planes in 3D) can be interchanged. Some problems that are difficult to solve in the primal space become easier when transformed into the dual space.
Examples where duality can be useful:
- Computing line arrangements
- Solving halfplane intersection problems
- Finding the smallest enclosing circle for a set of points
9. Use Randomization Techniques
Randomized algorithms can often provide simple and efficient solutions to complex geometric problems. These algorithms make random choices during execution, leading to good expected-case performance.
Examples of randomized algorithms in computational geometry:
- Randomized incremental construction for Delaunay triangulations
- Randomized algorithms for linear programming
- Probabilistic methods for nearest neighbor searches
10. Implement Robust Geometric Predicates
Geometric algorithms often rely on basic predicates, such as determining whether a point lies to the left or right of a line, or whether three points are collinear. Implementing these predicates robustly is crucial to avoid numerical errors that can lead to incorrect results.
Consider using exact arithmetic or adaptive precision techniques for critical geometric computations.
Practical Example: Convex Hull Problem
Let’s apply some of these strategies to a classic computational geometry problem: computing the convex hull of a set of points in 2D space.
The convex hull of a set of points is the smallest convex polygon that encloses all the points. It can be visualized as stretching a rubber band around the outermost points of the set.
Approach:
- Visualize the problem: Sketch a set of points and imagine drawing the convex hull around them.
- Consider geometric properties: The vertices of the convex hull will always be points from the original set.
- Apply a divide-and-conquer strategy: We’ll use the Graham scan algorithm, which sorts the points and then processes them in order.
Implementation in Python:
import math
def orientation(p, q, r):
val = (q[1] - p[1]) * (r[0] - q[0]) - (q[0] - p[0]) * (r[1] - q[1])
if val == 0:
return 0 # Collinear
return 1 if val > 0 else 2 # Clockwise or Counterclockwise
def graham_scan(points):
# Find the bottommost point (and leftmost if there are multiple)
bottom_point = min(points, key=lambda p: (p[1], p[0]))
# Sort points based on polar angle with respect to the bottom point
sorted_points = sorted(points, key=lambda p: (math.atan2(p[1] - bottom_point[1], p[0] - bottom_point[0]), p))
stack = []
for point in sorted_points:
while len(stack) > 1 and orientation(stack[-2], stack[-1], point) != 2:
stack.pop()
stack.append(point)
return stack
# Example usage
points = [(0, 3), (2, 2), (1, 1), (2, 1), (3, 0), (0, 0), (3, 3)]
convex_hull = graham_scan(points)
print("Convex Hull:", convex_hull)
This implementation demonstrates several of the strategies we discussed:
- Visualization: The algorithm processes points based on their geometric arrangement.
- Geometric properties: It uses the concept of orientation to determine whether to include a point in the hull.
- Divide-and-conquer: The Graham scan algorithm divides the problem into sorting and hull construction phases.
Common Pitfalls and How to Avoid Them
When approaching computational geometry problems, be aware of these common pitfalls:
1. Floating-Point Precision Errors
Geometric computations often involve floating-point arithmetic, which can lead to precision errors. These errors can accumulate and cause incorrect results, especially in algorithms that make many comparisons.
Solution: Use exact arithmetic libraries or implement robust geometric predicates. For simple cases, you can also use an epsilon value for comparisons.
2. Degenerate Cases
Geometric algorithms can fail when presented with degenerate cases, such as collinear points or overlapping line segments.
Solution: Always consider and test for degenerate cases. Implement special handling for these situations in your algorithms.
3. Incorrect Assumptions About Input
It’s easy to make assumptions about the input, such as no two points having the same coordinates, or all polygons being simple (non-self-intersecting).
Solution: Clearly define the preconditions for your algorithms and handle or report violations of these conditions.
4. Inefficient Algorithms for Large Datasets
Naive implementations of geometric algorithms often have poor performance on large datasets.
Solution: Use appropriate data structures and efficient algorithms. Consider the expected size of your input when choosing an approach.
5. Overlooking Edge Cases
Geometric problems often have numerous edge cases that can be easily overlooked.
Solution: Systematically consider different configurations and edge cases. Use visualization to help identify potential issues.
Advanced Topics in Computational Geometry
As you become more comfortable with basic computational geometry problems, you may want to explore more advanced topics:
1. Higher-Dimensional Geometry
Many geometric algorithms can be extended to higher dimensions, but this often introduces new challenges and complexities.
2. Robust Geometric Computation
Developing algorithms that are numerically robust and can handle degeneracies is an active area of research in computational geometry.
3. Approximation Algorithms
For some geometric problems, exact solutions may be computationally expensive. Approximation algorithms can provide near-optimal solutions more efficiently.
4. Kinetic Data Structures
These are data structures designed to efficiently maintain geometric information about objects in motion.
5. Geometric Optimization
Many real-world problems involve optimizing geometric configurations, such as facility location or path planning.
Conclusion
Approaching computational geometry problems requires a combination of geometric intuition, algorithmic thinking, and careful implementation. By visualizing problems, leveraging geometric properties, and applying appropriate algorithmic techniques, you can develop efficient solutions to complex geometric challenges.
Remember that practice is key to improving your skills in this area. Start with simpler problems and gradually work your way up to more complex ones. Don’t be discouraged if you encounter difficulties – computational geometry can be challenging, but it’s also incredibly rewarding.
As you continue to develop your skills, you’ll find that the strategies and techniques you learn in computational geometry have applications far beyond just solving geometric problems. They can enhance your overall problem-solving abilities and provide valuable insights into algorithm design and analysis.
Keep exploring, keep practicing, and enjoy the beautiful world of computational geometry!