Approaching Balanced and Unbalanced Binary Trees: A Comprehensive Guide
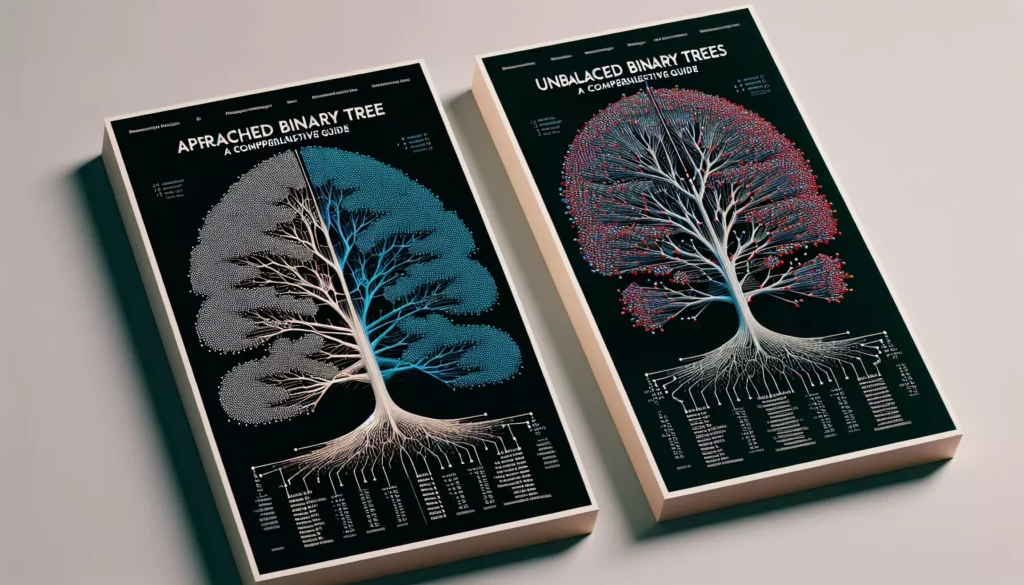
In the world of computer science and programming, data structures play a crucial role in organizing and managing information efficiently. Among these structures, binary trees stand out as versatile and powerful tools for solving various problems. In this comprehensive guide, we’ll dive deep into the realm of balanced and unbalanced binary trees, exploring their characteristics, implementations, and practical applications.
Understanding Binary Trees
Before we delve into the specifics of balanced and unbalanced binary trees, let’s start with a brief overview of binary trees in general.
A binary tree is a hierarchical data structure composed of nodes, where each node has at most two children, typically referred to as the left child and the right child. The topmost node in the tree is called the root, and nodes with no children are called leaves.
Here’s a simple representation of a binary tree node in Python:
class TreeNode:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
Balanced vs. Unbalanced Binary Trees
The distinction between balanced and unbalanced binary trees lies in the distribution of nodes and the overall structure of the tree.
Balanced Binary Trees
A balanced binary tree is one where the heights of the left and right subtrees of every node differ by at most one. This balance ensures that operations like insertion, deletion, and search can be performed efficiently, typically in O(log n) time complexity.
Some common types of balanced binary trees include:
- AVL Trees
- Red-Black Trees
- B-Trees
Unbalanced Binary Trees
An unbalanced binary tree, on the other hand, is one where the heights of the left and right subtrees can differ significantly. In extreme cases, an unbalanced tree can degenerate into a linked list, leading to poor performance for various operations.
Implementing a Balanced Binary Search Tree (AVL Tree)
Let’s implement a balanced binary search tree using the AVL tree algorithm. AVL trees maintain balance by ensuring that the heights of the left and right subtrees of any node differ by at most one.
class AVLNode:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
self.height = 1
class AVLTree:
def __init__(self):
self.root = None
def height(self, node):
if not node:
return 0
return node.height
def balance_factor(self, node):
if not node:
return 0
return self.height(node.left) - self.height(node.right)
def update_height(self, node):
if not node:
return
node.height = 1 + max(self.height(node.left), self.height(node.right))
def rotate_right(self, y):
x = y.left
T2 = x.right
x.right = y
y.left = T2
self.update_height(y)
self.update_height(x)
return x
def rotate_left(self, x):
y = x.right
T2 = y.left
y.left = x
x.right = T2
self.update_height(x)
self.update_height(y)
return y
def insert(self, root, value):
if not root:
return AVLNode(value)
if value < root.value:
root.left = self.insert(root.left, value)
else:
root.right = self.insert(root.right, value)
self.update_height(root)
balance = self.balance_factor(root)
# Left Heavy
if balance > 1:
if value < root.left.value:
return self.rotate_right(root)
else:
root.left = self.rotate_left(root.left)
return self.rotate_right(root)
# Right Heavy
if balance < -1:
if value > root.right.value:
return self.rotate_left(root)
else:
root.right = self.rotate_right(root.right)
return self.rotate_left(root)
return root
def insert_value(self, value):
self.root = self.insert(self.root, value)
This implementation of an AVL tree ensures that the tree remains balanced after each insertion operation. The balance_factor
method calculates the difference in height between the left and right subtrees, and rotations are performed as necessary to maintain balance.
Working with Unbalanced Binary Trees
While balanced trees offer optimal performance for many operations, there are scenarios where unbalanced trees can be useful or even preferable. Let’s explore some aspects of working with unbalanced binary trees.
Implementing a Simple Binary Search Tree
Here’s an implementation of a basic, potentially unbalanced binary search tree:
class BSTNode:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
class BinarySearchTree:
def __init__(self):
self.root = None
def insert(self, value):
if not self.root:
self.root = BSTNode(value)
else:
self._insert_recursive(self.root, value)
def _insert_recursive(self, node, value):
if value < node.value:
if node.left is None:
node.left = BSTNode(value)
else:
self._insert_recursive(node.left, value)
else:
if node.right is None:
node.right = BSTNode(value)
else:
self._insert_recursive(node.right, value)
def search(self, value):
return self._search_recursive(self.root, value)
def _search_recursive(self, node, value):
if node is None or node.value == value:
return node
if value < node.value:
return self._search_recursive(node.left, value)
return self._search_recursive(node.right, value)
This implementation doesn’t include any balancing mechanisms, so it can become unbalanced depending on the order of insertions.
Advantages of Unbalanced Trees
While balanced trees generally offer better performance, unbalanced trees can have some advantages in certain scenarios:
- Simplicity: Unbalanced trees are often simpler to implement and understand, making them suitable for educational purposes or when simplicity is prioritized over performance.
- Memory Efficiency: Unbalanced trees may use less memory as they don’t need to store additional balancing information.
- Faster Insertions: In cases where the tree remains relatively balanced naturally, unbalanced trees can offer faster insertions as they don’t need to perform balancing operations.
Dealing with Unbalanced Trees
When working with unbalanced trees, it’s important to be aware of potential performance issues and implement strategies to mitigate them:
- Periodic Rebalancing: Implement a mechanism to periodically rebalance the tree, especially after a large number of insertions or deletions.
- Randomization: If possible, randomize the order of insertions to reduce the likelihood of creating highly unbalanced structures.
- Tree Rotation: Implement rotation operations that can be manually applied to improve balance when needed.
Comparing Performance: Balanced vs. Unbalanced
To illustrate the performance difference between balanced and unbalanced binary trees, let’s compare the worst-case time complexities for common operations:
Operation | Balanced Tree | Unbalanced Tree (Worst Case) |
---|---|---|
Insertion | O(log n) | O(n) |
Deletion | O(log n) | O(n) |
Search | O(log n) | O(n) |
As we can see, balanced trees offer significantly better worst-case performance, especially for larger datasets.
Practical Applications
Understanding the characteristics and implementations of balanced and unbalanced binary trees is crucial for solving various programming problems and optimizing algorithms. Let’s explore some practical applications:
1. Database Indexing
Balanced binary trees, particularly B-trees and their variants, are widely used in database systems for efficient indexing. They allow for fast search, insertion, and deletion operations, which are critical for database performance.
2. File Systems
Many file systems use balanced tree structures to organize and manage file and directory information. This allows for quick file lookups and efficient use of storage space.
3. Symbol Tables in Compilers
Compilers often use balanced binary trees to implement symbol tables, which store information about variables, functions, and other program entities. The balanced structure ensures fast lookups during the compilation process.
4. Network Routing Tables
Routing algorithms in computer networks may use tree-like structures to store and search routing information efficiently. Balanced trees can help optimize the lookup process in large routing tables.
5. Game Development
In game development, binary space partitioning (BSP) trees, which can be balanced or unbalanced, are used for tasks like collision detection, visibility determination, and spatial indexing.
Interview Preparation: Common Tree-Related Questions
When preparing for technical interviews, especially for major tech companies, it’s essential to be well-versed in tree-related problems. Here are some common questions and concepts to focus on:
- Tree Traversals: Implement and understand in-order, pre-order, and post-order traversals for binary trees.
- Depth-First Search (DFS) vs. Breadth-First Search (BFS): Know when to use each approach and be able to implement both for tree structures.
- Balanced Tree Implementations: Understand the basics of AVL trees and Red-Black trees, including their balancing mechanisms.
- Lowest Common Ancestor (LCA): Be able to find the LCA of two nodes in a binary tree or binary search tree.
- Tree Serialization and Deserialization: Convert a tree to a string representation and vice versa.
- Path Sum Problems: Solve problems related to finding paths in a tree that sum to a given value.
- Tree Construction: Construct a tree from given traversal sequences (e.g., from inorder and preorder traversals).
- Binary Search Tree Operations: Implement basic BST operations and understand their time complexities.
Conclusion
Mastering the concepts of balanced and unbalanced binary trees is crucial for any aspiring programmer or computer scientist. These data structures form the backbone of many algorithms and systems, from database indexing to compiler design.
By understanding the trade-offs between balanced and unbalanced trees, you’ll be better equipped to choose the right data structure for your specific use case. Balanced trees offer consistent performance guarantees but may require more complex implementation and maintenance. Unbalanced trees, while simpler, can suffer from performance degradation in worst-case scenarios.
As you continue your journey in programming and prepare for technical interviews, make sure to practice implementing both types of trees and solving related problems. This will not only enhance your understanding of these fundamental data structures but also sharpen your problem-solving skills and algorithmic thinking.
Remember, the key to mastering these concepts is consistent practice and application. Try implementing the code examples provided in this guide, experiment with different tree structures, and challenge yourself with increasingly complex tree-related problems. With dedication and perseverance, you’ll be well-prepared to tackle any tree-related challenge that comes your way, whether in a coding interview or a real-world programming task.